1. 混合使用 Path、Query 和请求体参数
from fastapi import FastAPI, Path
from typing import Optional
from pydantic import BaseModel
app = FastAPI()
class Item1(BaseModel):
name: str
price: float
description: Optional[str] = None
tax: Optional[float]
@app.put("/items/{item_id}")
async def update_item(
*,
item_id: int = Path(..., title="id of item to get", ge=0, le=1000),
q: Optional[str] = None,
item: Optional[Item1] = None,
):
res = {"item_id": item_id}
if q:
res.update({"q": q})
if item:
res.update({"item": item})
return res
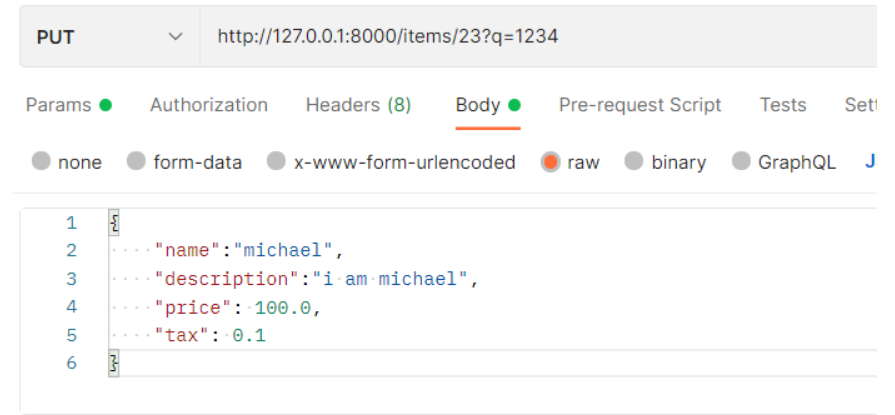
2. 多个请求体参数
from pydantic import BaseModel
class Item(BaseModel):
name: str
price: float
description: Optional[str] = None
tax: Optional[float]
class User(BaseModel):
username: str
full_name: Optional[str] = None
@app.put("/items/{item_id}")
async def update_item(item_id: int, item: Item, user: User):
res = {"item_id" : item_id, "item" : item, "user": user}
return res
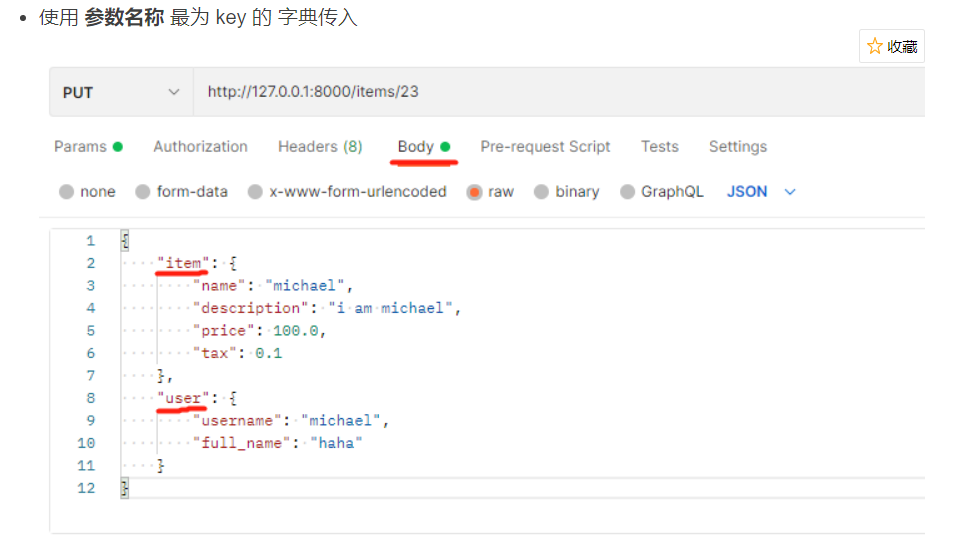
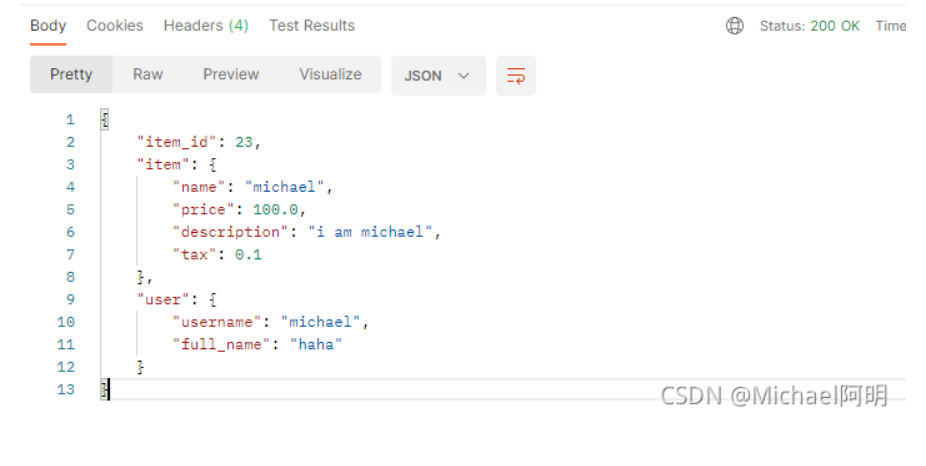
3. 请求体中的单一值
传参时,varname : type = Body(...),如果不这么写,会被作为查询参数 ?varname=xxx
from fastapi import Body
class Item(BaseModel):
name: str
price: float
description: Optional[str] = None
tax: Optional[float]
class User(BaseModel):
username: str
full_name: Optional[str] = None
@app.put("/items/{item_id}")
async def update_item(item_id: int, item: Item, user: User, importance : int = Body(...)):
res = {"item_id" : item_id, "item" : item, "user": user}
return res
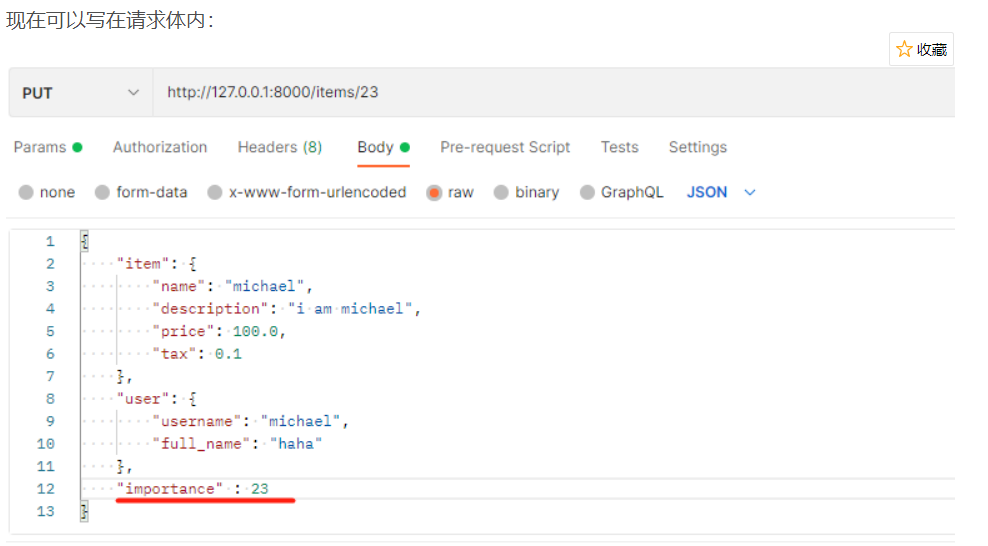
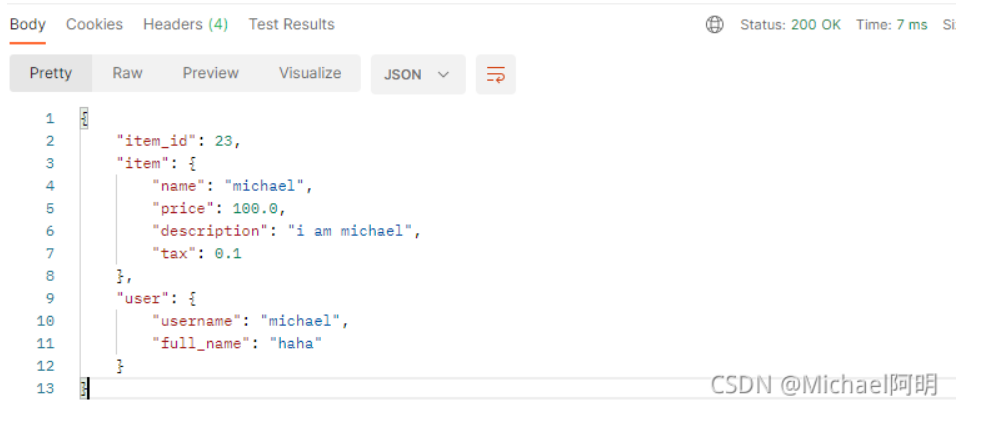
4. 多个请求体参数和查询参数
由于默认情况下单一值被解释为查询参数,因此你不必显式地添加 Query,你可以仅执行操作:q: str = None
5. 嵌入单个请求体参数
如果你只有一个请求体参数
@app.put("/items/{item_id}")
async def update_item(item_id: int, item: Item):
res = {"item_id" : item_id, "item" : item}
return res
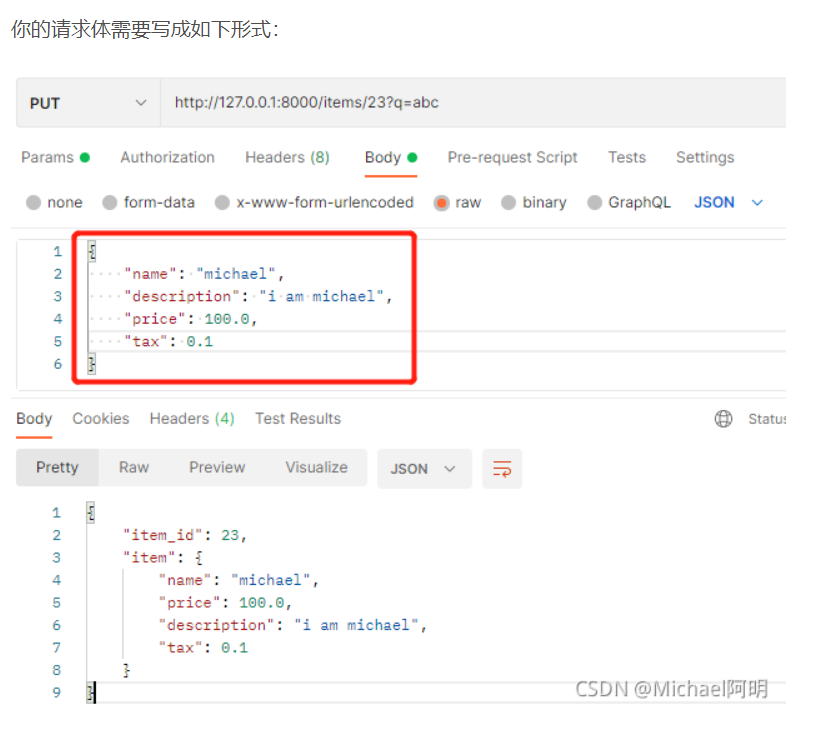
如果你想写成 带 key 的 json 形式,添加一个传入参数 embed,item: Item = Body(..., embed=True)
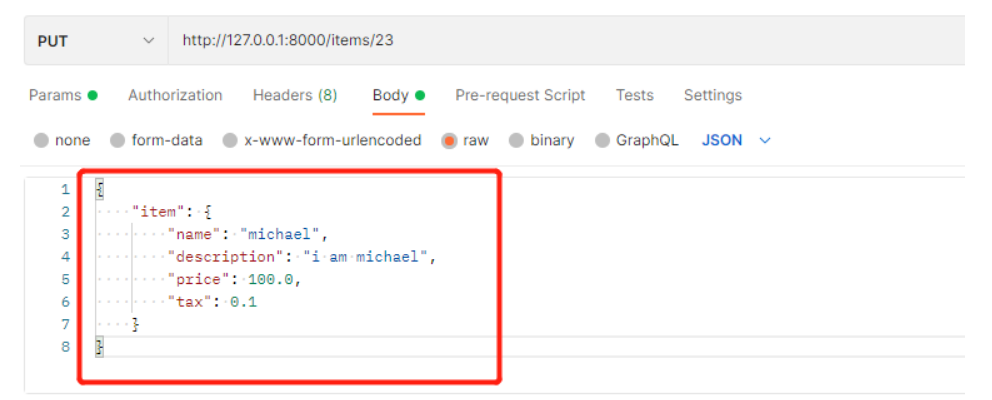
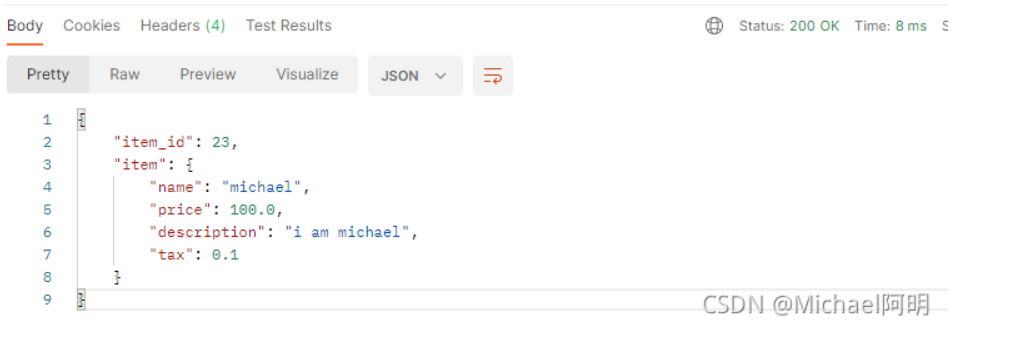
6. 字段
可以使用 Pydantic 的 Field 在 Pydantic 模型内部声明校验和元数据
from fastapi import FastAPI, Path, Body
from typing import Optional
from pydantic import BaseModel, Field
app = FastAPI()
class Item(BaseModel):
name: str
price: float = Field(..., gt=0, description="price must be greater than 0")
description: Optional[str] = Field(None, title="description of item", max_length=30)
tax: Optional[float] = None
@app.put("/items/{item_id}")
async def update_item(item_id: int, item: Item = Body(..., embed=True)):
res = {"item_id" : item_id, "item" : item}
return res
Field 的工作方式和 Query、Path 和 Body 相同,包括它们的参数等等也完全相同
注意,from pydantic import Field
7. 嵌套模型
7.1 List 字段
将一个属性定义为拥有子元素的类型,如 list
class Item(BaseModel):
name: str
price: float = Field(..., gt=0, description="price must be greater than 0")
description: Optional[str] = Field(None, title="description of item", max_length=30)
tax: Optional[float] = None
tags: list = [] # 没有声明元素类型
具有子类型的 List,from typing import List
tags: List[str] = []
7.2 子模型作为类型
from fastapi import FastAPI, Path, Body
from typing import Optional, List, Set
from pydantic import BaseModel, Field
app = FastAPI()
class Image(BaseModel):
url:str
name: str
class Item(BaseModel):
name: str
price: float = Field(..., gt=0, description="price must be greater than 0")
description: Optional[str] = Field(None, title="description of item", max_length=30)
tax: Optional[float] = None
tags: Set[str] = []
image: Optional[Image] = None
@app.put("/items/{item_id}")
async def update_item(item_id: int, item: Item = Body(..., embed=True)):
res = {"item_id" : item_id, "item" : item}
return res
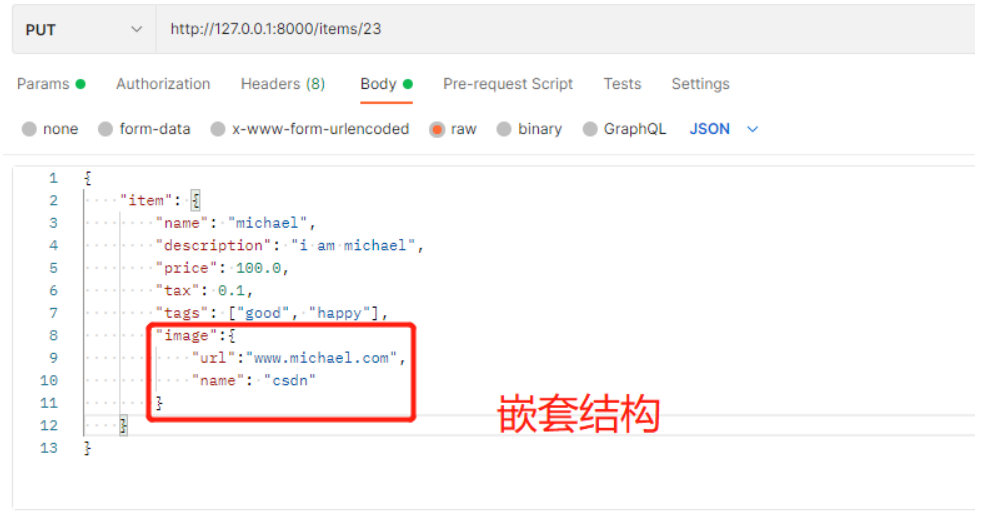
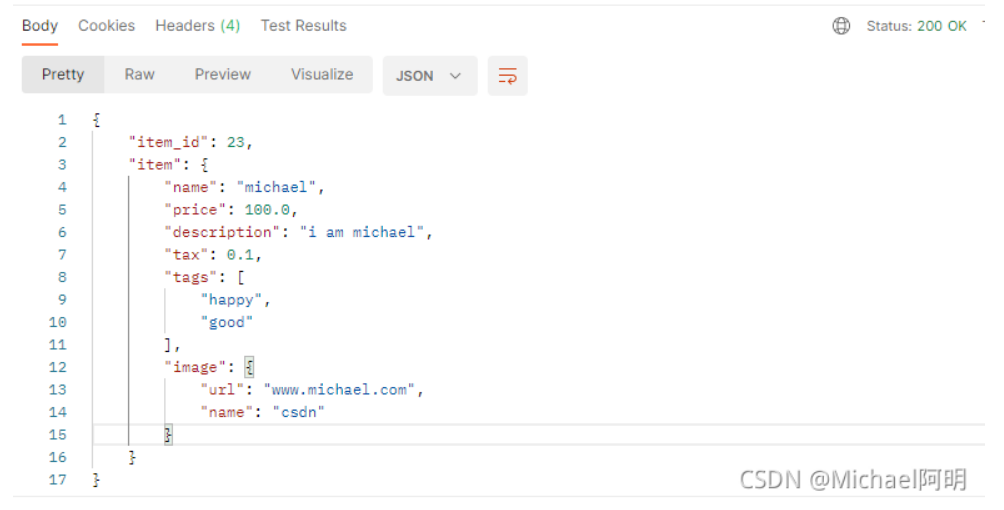
8. 特殊类型校验
HttpUrl,检查是不是有效的 URL
from pydantic import BaseModel, Field, HttpUrl
app = FastAPI()
class Image(BaseModel):
url: HttpUrl
name: str
则上面的输入应改的地方 "url":"http://www.michael.com",否则不是有效的 URL
9. 带有一组子模型的属性
更改为 image: Optional[List[Image]] = None
输入需要改为
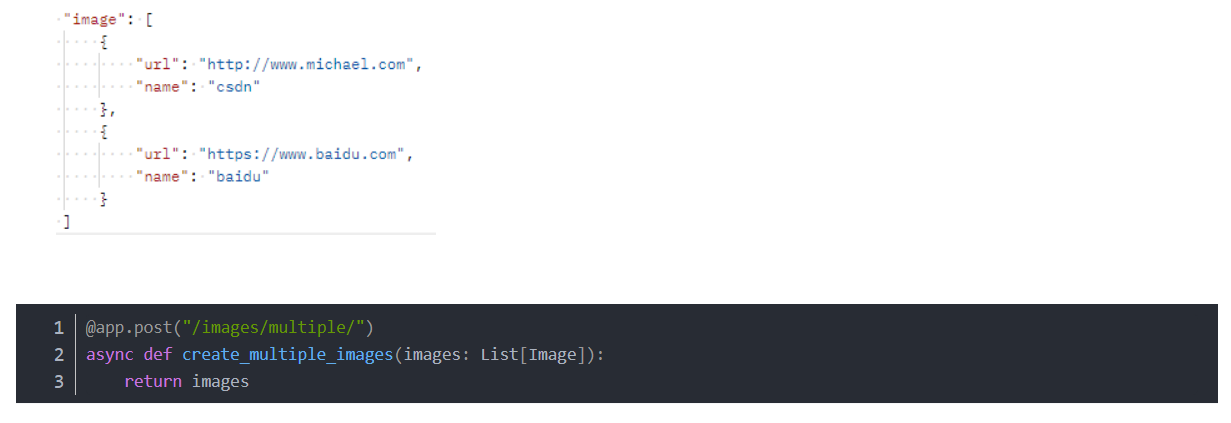
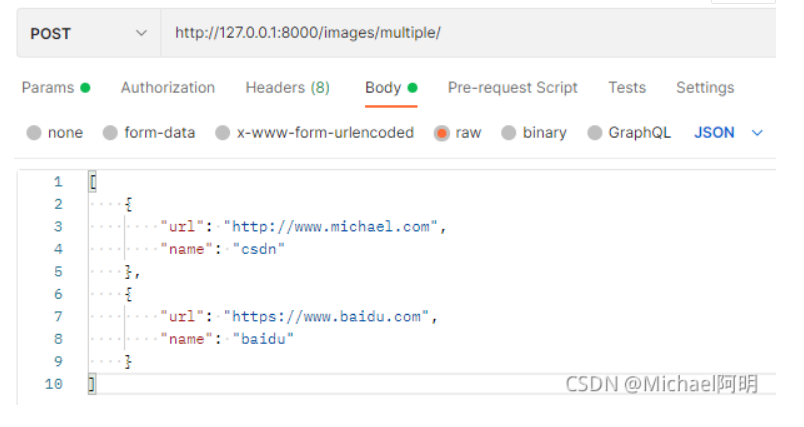
10. 任意 dict 构成的请求体
from typing import Optional, List, Set, Dict
@app.post("/index-weights/")
async def create_index_weights(weights: Dict[int, float]):
# key 为 int, value 为浮点
return weights
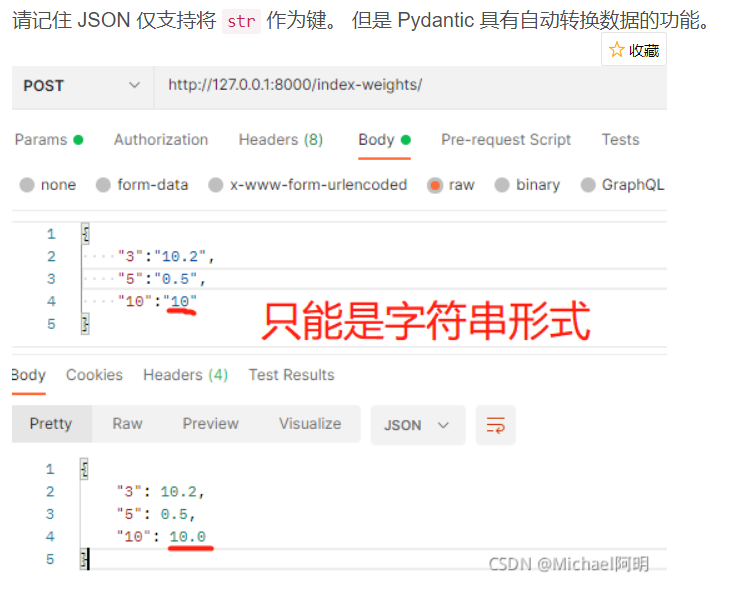