bool b1 = true;
bool b2 = false;
Console.WriteLine(b1 + "_" + b2);
//char(字符)
char ch = 'A';
Console.WriteLine("ch的值为"+ch);
//string(字符串)
string str = "我要认认真真学CSharp";
Console.WriteLine(str);
#endregion
int sbyteSize = sizeof(sbyte);
Console.WriteLine("sbyte所占的字节数为:" + sbyteSize);
int shortSize = sizeof(short);
Console.WriteLine("short所占的字节数为:" + shortSize);
int intSize = sizeof(int);
Console.WriteLine("int所占的字节数为:" + intSize);
int longSize = sizeof(long);
Console.WriteLine("long所占的字节数为:" + longSize);
Console.WriteLine("*********************************************");
int byteSize = sizeof(byte);
Console.WriteLine("byte所占的字节数为:" + byteSize);
int uintSize = sizeof(uint);
Console.WriteLine("uint所占的字节数为:" + uintSize);
int ushortSize = sizeof(ushort);
Console.WriteLine("ushort所占的字节数为:" + ushortSize);
int ulongSize = sizeof(ulong);
Console.WriteLine("ulong所占的字节数为:" + ulongSize);
Console.WriteLine("*********************************************");
int floatSize = sizeof(float);
Console.WriteLine("float所占的字节数为:" + floatSize);
int doubleSize = sizeof(double);
Console.WriteLine("double所占的字节数为:" + doubleSize);
int decimalSize = sizeof(decimal);
Console.WriteLine("decimal所占的字节数为:" + decimalSize);
Console.WriteLine("*********************************************");
int boolSize = sizeof(bool);
Console.WriteLine("bool所占的字节数为:" + boolSize);
int charSize = sizeof(char);
Console.WriteLine("char所占的字节数为:" + charSize);
}
}
}
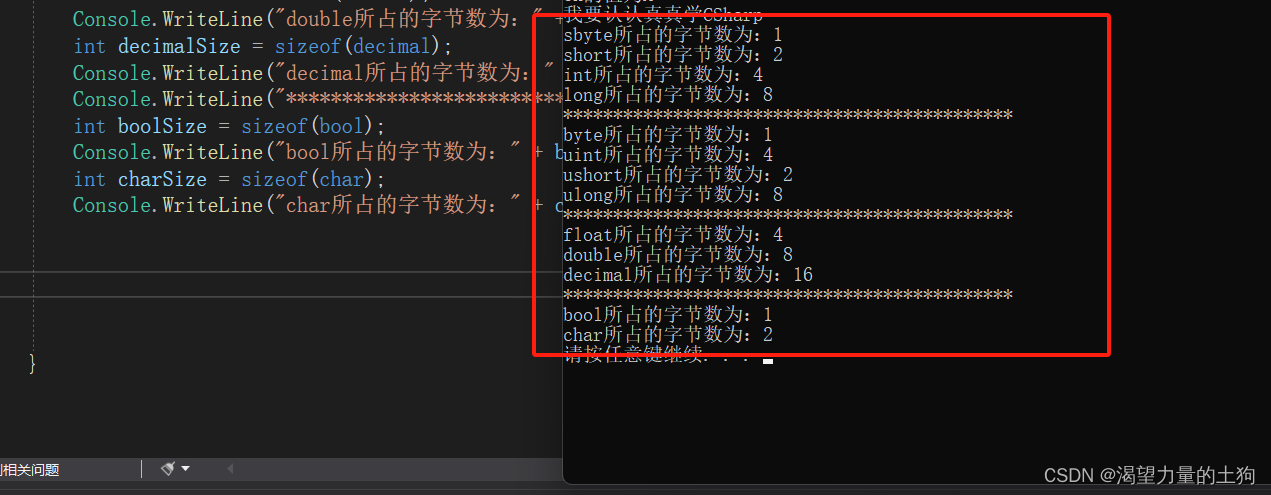
## 常量
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CSharp入门_常量
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine(“常量”);
//常量使用关键字const
const int c = 1;
Console.WriteLine(“c的值为”+c);
//常量特点:必须初始化,不可以更改
//使用常量的场景:声明一些常用的不变的量,如π
}
}
}
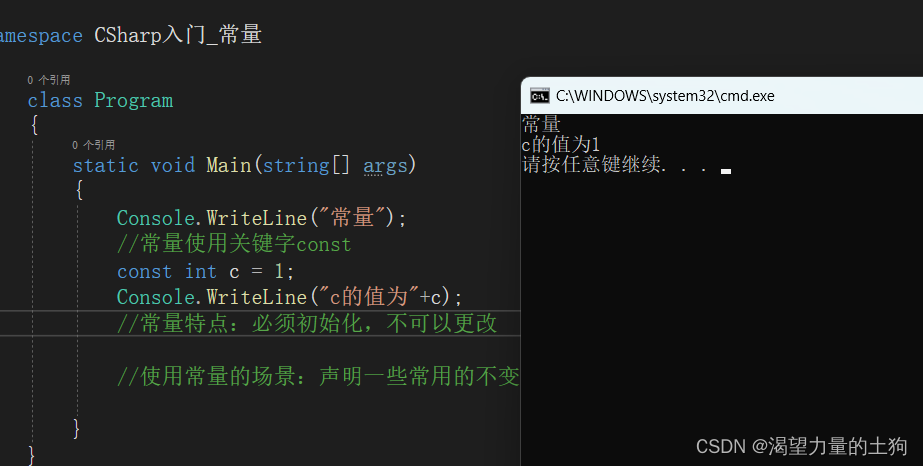
## 转义字符
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CSharp入门_转义字符
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine(“转义字符”);
/*
* 转义字符的使用
*/
//单引号 ’
string str1 = “‘哈哈哈’”;
Console.WriteLine("str1 = "+str1);
//双引号 "
string str2 = ““CSharp太好学啦!””;
Console.WriteLine("str2 = " + str2);
//换行 \n
string str3 = ““CSharp\n太好学啦!””;
Console.WriteLine("str3 = " + str3);
//斜杠 \
string str4 = ““CSharp\太好学啦!””;
Console.WriteLine("str4 = " + str4);
/*
* 下面的是不太常用的转义字符
*
*/
//制表符 \t (空一个tab键)
string str5 = "我要好好学\t编程";
Console.WriteLine("str5 = " + str5);
//光标退格 \b (光标退一格进行覆盖)
string str6 = "我要好好学\b编程";
Console.WriteLine("str6 = " + str6);
//警报音 \a 这里会有个警报音响起
string str7 = "\a";
Console.WriteLine(str7);
/*
取消转义字符 使用@
*/
string str8 = @"\n\\";
Console.WriteLine("str8 = "+str8);
}
}
}
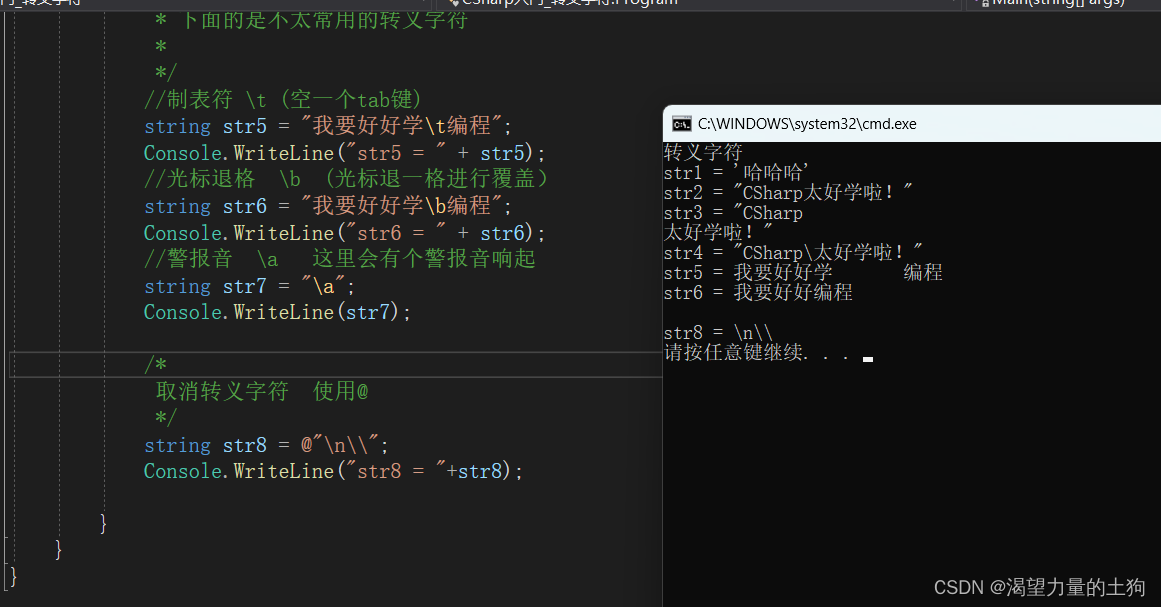
## 隐式转换
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CSharp入门_隐式转换
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine(“隐式转换”);
//大范围装小范围,记住这个就可以
long L = 5;
int i = 1;
L = i;
Console.WriteLine("L = "+L);
//一般都是这个规律,但是浮点型中decimal不能隐式转换为float和double
//特殊类型如bool,char,string之间不存在隐式转换,但是char可以隐式转换为整数
//(因为char取值范围是0-65535,其对应的数字 其实是一个ascll码,一个数字就是一个对应关系)
//无符号的不能装有符号的,但是有符号的可以装无符号的,因为无符号数没有负数,而有符号数则有负数
//即有符号数不能隐式转换为无符号数,无符号数可以隐式转换为有符号数(要注意范围是涵盖的,大装小)
uint ui = 4;
L = ui;
Console.WriteLine("L = " +L );
//浮点数可以装任何类型的 整型
float f = L;
Console.WriteLine("f = " + f);
//总结:
//double一>f1oat一>所有整形(无符号、有符号)
//decimal一>所有整形(无符号、有符号)
//整数不能去装浮点数:浮点数不能隐式转换为整数
//总结隐式转换规则
//高精度(大范围)装低精度(小范围)
//double一 > f1oat一 > 整数(无符号、有符号)一 > char
//decimal一 > 整数(无符号、有符号)一 > char
//string和boo1不参与隐式转换规则的
}
}
}
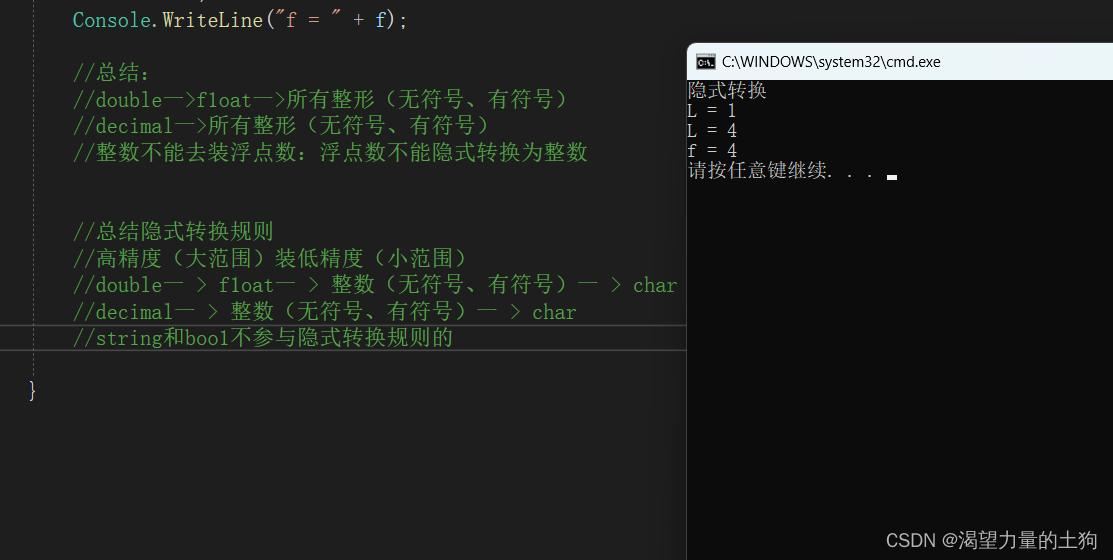
## 显示转换
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CSharp入门_显示转换
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine(“显示转换”);
//1、强制类型转换 将高精度类型强制转换为低精度类型
//语法:变量类型 变量名 = (强转的类型)变量;
//需要注意:精度和范围
short st = 1;
int i = 5;
st = (short)i;
Console.WriteLine("st的值为:" + st);
//如果我们范围超过就会出问题
short so = 2;
int x = 40000;
so = (short)x;
Console.WriteLine("so的值为:" + so);
//注意:在强制类型转换的时候一定要注意范围,在强制转换浮点型的时候会出现精度变低的情况,要注意
//浮点数强转成整形时会直接抛弃掉小数点后面的小数
double b = 1.5999999;
i = (int)b;
Console.WriteLine("i的值为:" + i);
//2、Parse转换 (将字符串类型转换为对应的类型)字符串必须能够转换为对应类型,不然会报错,还有范围
//语法:变量类型.Parse("字符串");
int i2 = int.Parse("123");
Console.WriteLine("i2的值为:" + i2);
//3、Convert转换
//作用:
//更准确的将各个类型之间进行相互转换
//语法:Convert.To目标类型(变量或常量)
//注意:填写的变量或常量必须正确否则出错
int i3 = Convert.ToInt32("1234");
Console.WriteLine("i3的值为:" + i3);
//Convert转换时会四舍五入,精度比强制类型转换高
i3 = Convert.ToInt32(1.688f);
Console.WriteLine("i3的值为:" + i3);
//对于bool也可以转化为int
i3 = Convert.ToInt32(true);
Console.WriteLine("i3的值为:" + i3);
i3 = Convert.ToInt32(false);
Console.WriteLine("i3的值为:" + i3);
float f1 = Convert.ToSingle(12.5);
bool b1 = Convert.ToBoolean("true");
//4、其他类型转string
//变量.ToString();
String str3 = 1.ToString();
Console.WriteLine("str3的值为:" + str3);
String str4 = 1.5f.ToString();
Console.WriteLine("str4的值为:" + str4);
Console.WriteLine("666"+1 +true);//相对于每个都调用了tostring
}
}
}
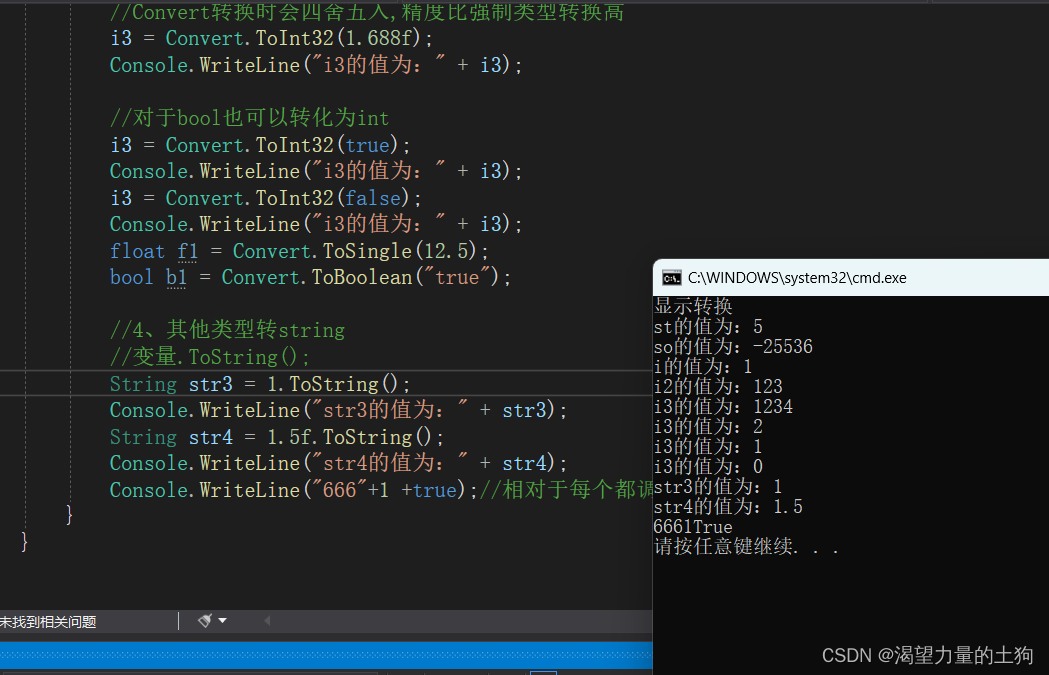
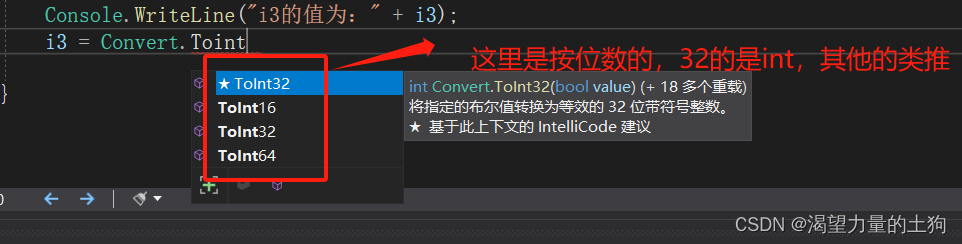
## 异常捕获
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CSharp入门_异常捕获
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine(“异常捕获”);
#region 作用
//异常捕获作用:避免代码错误造成程序卡死
//string str = Console.ReadLine();
// int i = int.Parse(str);//比如这里的str如果输入的范围大于int就会卡死
#endregion
#region 语法
try
{
//希望进行异常捕获的代码块
//如果try中的代码块出现异常就进入catch
}
catch(Exception e)//可以通过e得到具体的报错信息(可以不写)
{
//如果异常进行的动作
}
finally//可选
{
//不管有没有出错,都会执行其中的代码
}
#endregion
#region 测试
try
{
string str2 = Console.ReadLine();
int i2= int.Parse(str2);
Console.WriteLine(i2);
}
catch
{
Console.WriteLine("请输入合法的数字!");
}
finally
{
Console.WriteLine("执行完毕!");
}
#endregion
}
}
}
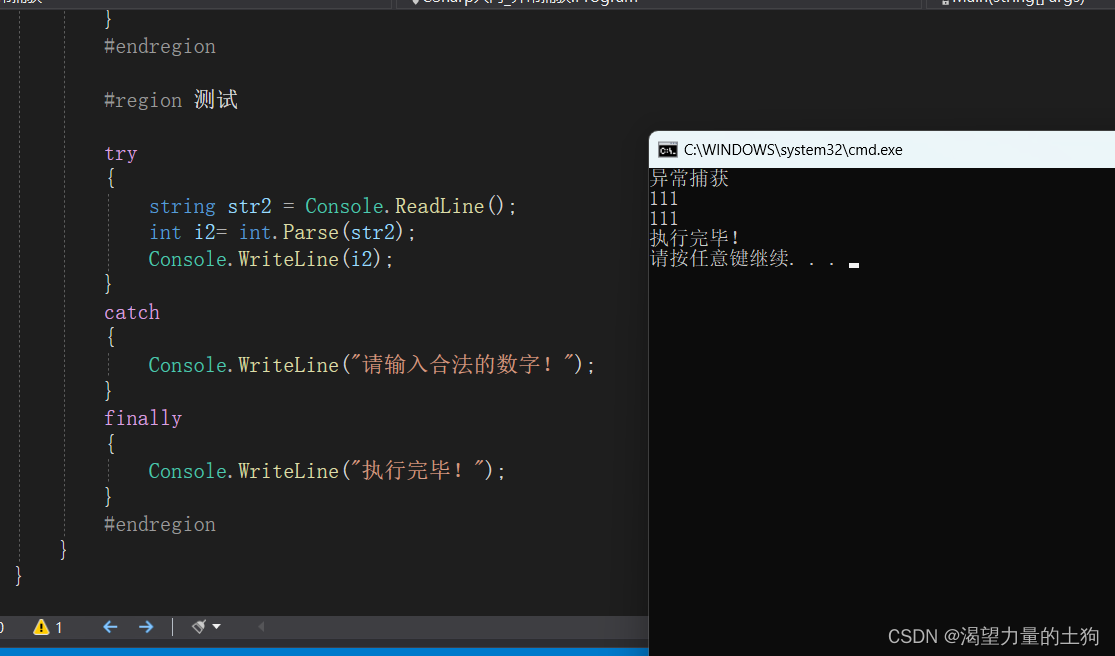如果输入不合法:
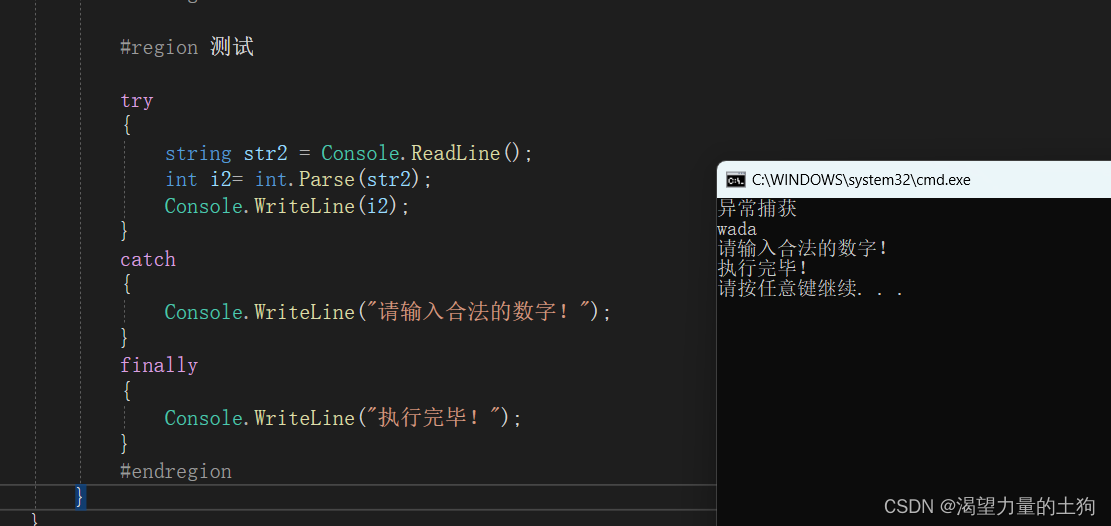
## 运算符
### 算术运算符
| 一元运算符 | 描述 | 实例 |
| --- | --- | --- |
| ++ | 增量运算符 | ++a 输出 11 |
| -- | 减量运算符 | --a 输出 9 |
| + | 一元加运算符 | +a 输出 10 |
| - | 一元减运算符 | -a 输出 -10 |
| 二元运算符 | 描述 | 实例 |
| --- | --- | --- |
| \* | 乘法运算符 | a \* b 输出 200 |
| / | 除法运算符 | b / a 输出 2 |
| % | 余数运算符 | b % a 输出 0 |
| + | 加法运算符 | b + a 输出 30 |
| - | 减法运算符 | b - a 输出 10 |
### 布尔逻辑运算符
| 布尔运算符 | 描述 | 实例 |
| --- | --- | --- |
| ! | 逻辑非运算符 | !a 为 False |
| & | 逻辑与运算符 | a & b 为 False |
| | | 逻辑或运算符 | a | b 为 True |
| ^ | 逻辑异或运算符 | a ^ b 为 True |
| && | 条件逻辑与运算符 | a && b 为 False |
| || | 条件逻辑或运算符 | a || b 为 True |
### 关系运算符
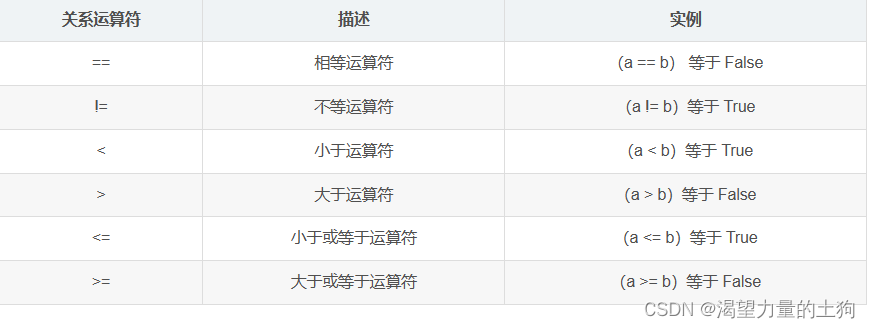
### 位运算符
| 位运算符 | 描述 | 实例 |
| --- | --- | --- |
| ~ | 按位求补运算符 | ~a 等于 -2 |
| << | 左移位运算符 | a << 2 等于 4 |
| >> | 右移位运算符 | a >> 2 等于 0 |
| >>> | 无符号右移运算符 | a >>> 2 等于 0 |
| & | 逻辑与运算符 | a & b 等于 0 |
| ^ | 逻辑异或运算符 | a ^ b 等于 3 |
| | | 逻辑或运算符 | a | b 等于 3 |
### 其他运算符
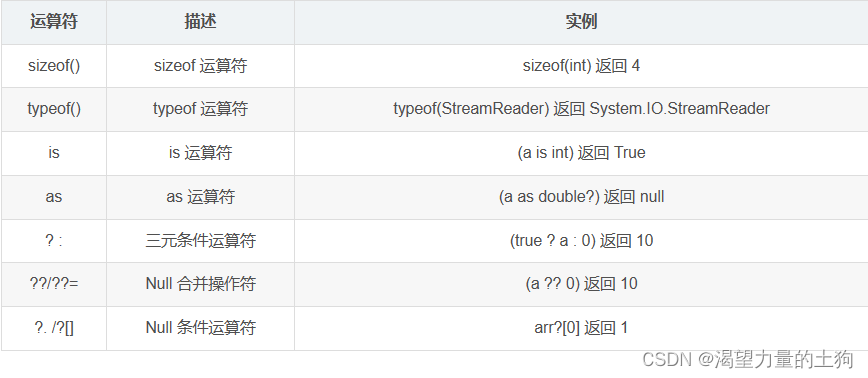
## 字符串拼接
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CSharp入门_字符串拼接
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine(“字符串拼接”);
//1、用+号进行字符串拼接
string str = “123”;
Console.WriteLine(str+“456”);
str = str + 1;
Console.WriteLine(str);
str = "123";
str += "1" + 4 + true;
Console.WriteLine(str);
str += 1 + 2 + 3 + 4;
Console.WriteLine(str);
str += "" + 1 + 2 + 3;
Console.WriteLine(str);
//2、使用string.Format();
//string.Format("待拼接的内容",内容1,内容2,.....)
//拼接内容中的固定规则
//想要被拼接的内容用占位符替代{数字}数字:n依次往后
string str3;
str3 = string.Format("我爱{0}, 我今年{1}岁, 我想好好学习{2}", "学习",18, "C#");
Console.WriteLine(str3);
str3 = string.Format("{0}{1}{2}", 1, true, false);
Console.WriteLine(str3);
//3、控制台打印
Console.WriteLine("A{0}B{1}C{2}", 1, true, false);
//注意:后面的内容可以比前面多,但是不能比前面少,否则会报错,还有占位符数字要从0开始
}
}
}
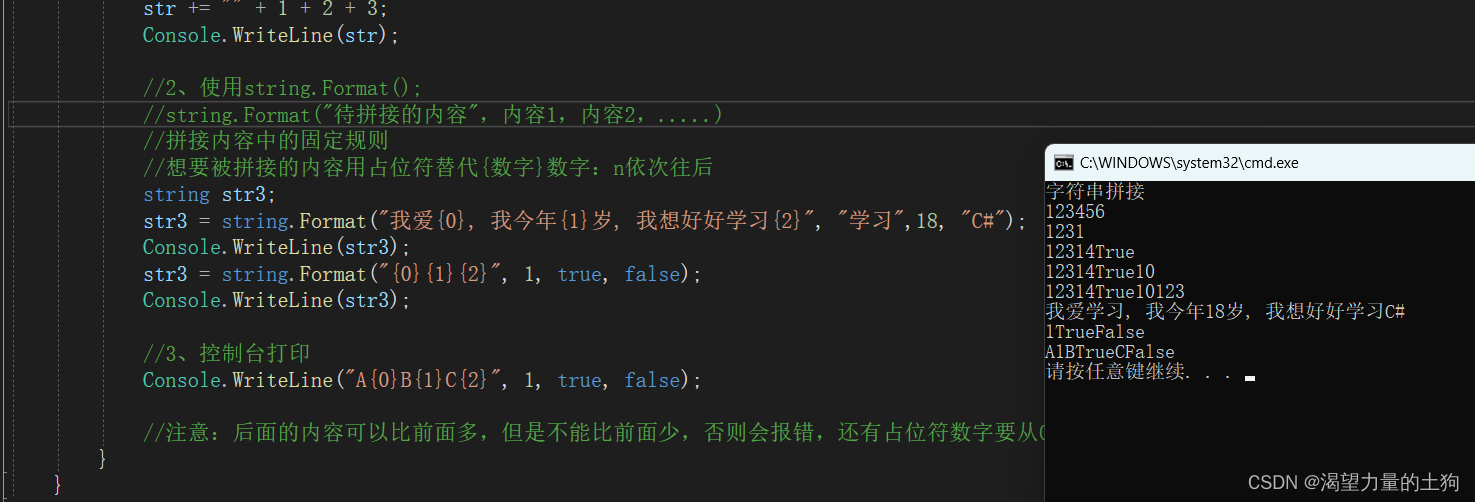
## 条件分支语句
### if...else 语句
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CSharp入门_条件分支语句
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine(“条件分支语句”);
//if语句
int i = 0;
int flag = 0;
if (flag == 0)
{
i = 100;
flag = 1;
}
Console.WriteLine(i);
//if...else
if (flag == 1)
{
i = 200;
}
else
{
i = 1;
}
Console.WriteLine(i);
//再举一个
Console.WriteLine("请输入性别:"+"输入男或者女");
string sex = Console.ReadLine();
if (sex == "男")
{
Console.WriteLine("我是男生");
}
else
{
Console.WriteLine("我是女生");
}
}
}
}
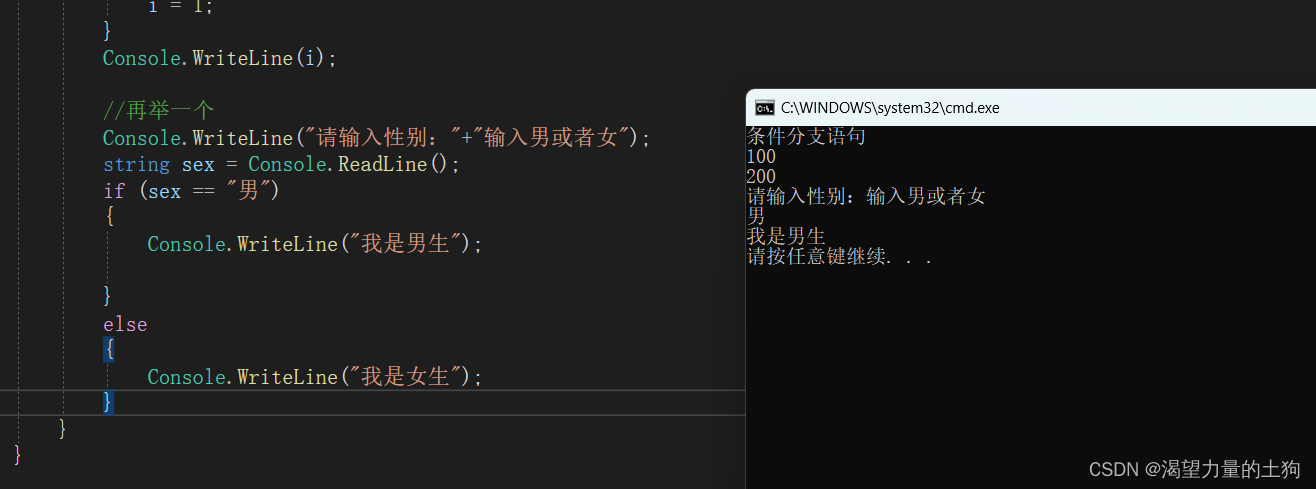
### switch语句
//switch语句
int a = 3;
switch (a)
{
case 1: //条件只能是常量
Console.WriteLine("a = 1");
break;
case 2:
Console.WriteLine("a = 2");
break;
case 3:
Console.WriteLine("a = 3");
break;
default:
Console.WriteLine("什么条件都不满足,执行default语句");
break;
}
//switch也支持自定义常量
int b = 2;
const int x = 2;
switch (b)
{
case 1: //条件只能是常量
Console.WriteLine("b = 1");
break;
case x:
Console.WriteLine("b = 2");
break;
case 3:
Console.WriteLine("b = 3");
break;
default:
Console.WriteLine("什么条件都不满足,执行default语句");
break;
}
//同时需要注意的是switch会进行贯穿
int c = 1;
switch (c)
{
case 1: //条件只能是常量
case 2:
case 3:
Console.WriteLine("c = 3"); //C#的贯穿和java不一样,java的在case后面还可以进行执行语句,而C#后面只能像上述所写
//贯穿到第一个break,所以结果是c = 3
break;
default:
Console.WriteLine("什么条件都不满足,执行default语句");
break;
}
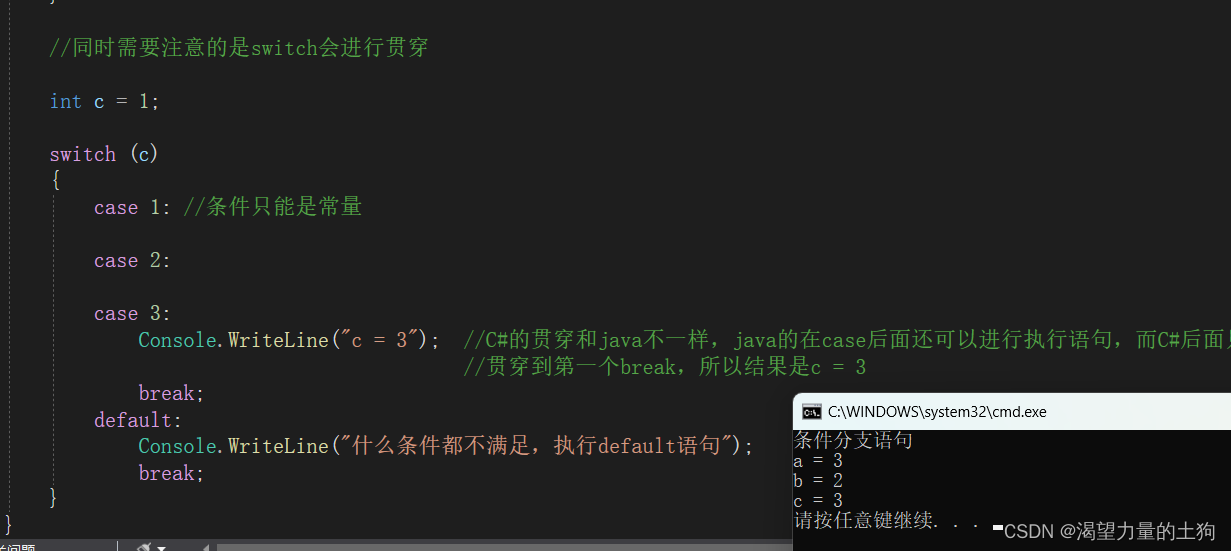
## 循环语句
### while语句
写循环语句的时候一般要避免写死循环(条件一直为真) 还会造成程序卡死
但是有时候也会用到死循环
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CSharp入门_循环语句
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine(“循环语句”);
//while语句
//while (true)
//{
// Console.WriteLine("这是一个死循环");
//}
//巧用死循环
string password = "123456";
while (true)
{
Console.WriteLine("请用户输入密码:");
string str = Console.ReadLine();
if (str == password)
{
Console.WriteLine("登录成功!");
break;
}
}
}
}
}
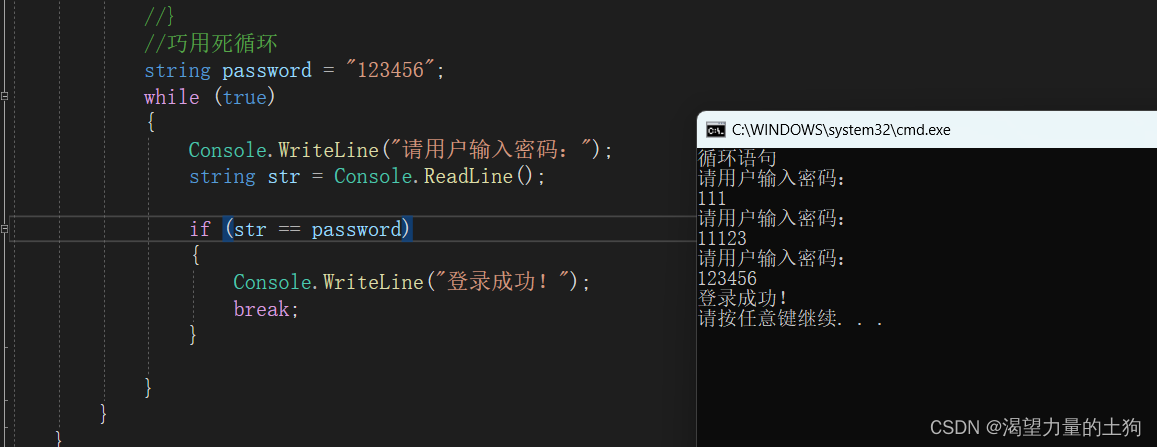
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace CSharp入门_循环语句
{
class Program
{
static void Main(string[] args)
{
Console.WriteLine(“循环语句”);
//while语句
//while (true)
//{
// Console.WriteLine("这是一个死循环");
//}
//巧用死循环
string password = "123456";
while (true)
{
Console.WriteLine("请用户输入密码:");
string str = Console.ReadLine();
if (str == password)
{
Console.WriteLine("登录成功!");
break;//跳出循环
}
}
int i = 0;
while (i < 10)
{
i++;
}
Console.WriteLine("i = "+i);
}
}
}
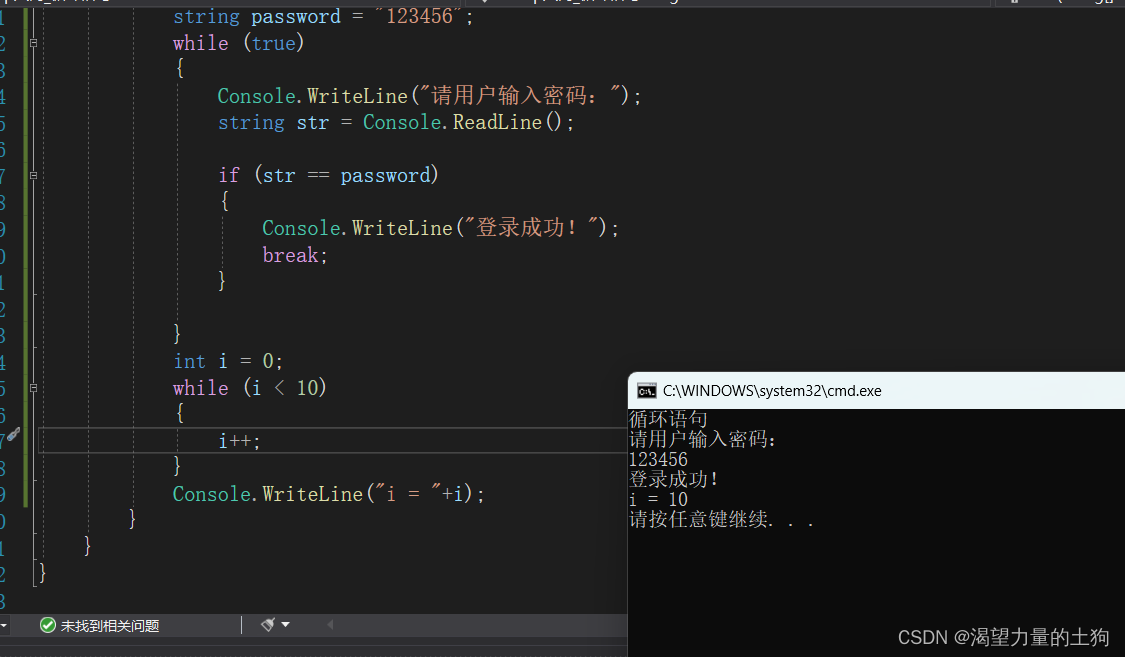
//打印1到20之间的奇数
int index = 0;
while (index < 20)
{
++index;
//什么样的数是奇数
//不能被2整除的数一>%
if (index % 2 == 0)
continue; //回到循环开始,继续执行
Console.WriteLine(index);
}
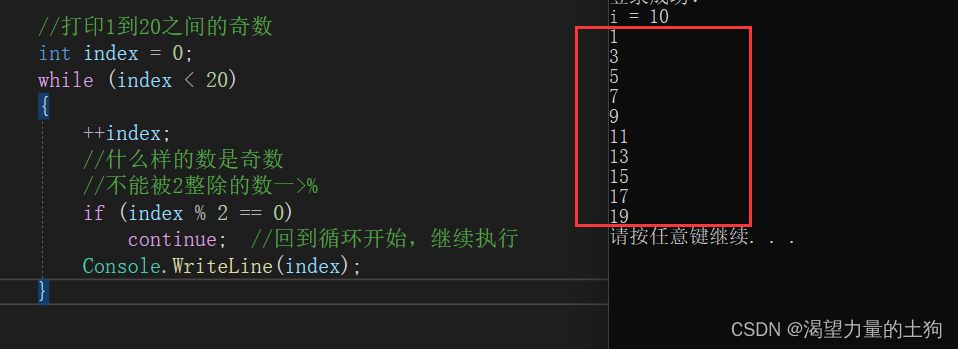
### do while语句 (使用较少)
do while语句和while的不同在于do while是先执行一次再进行判断,其他基本一致。
**自我介绍一下,小编13年上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。**
**深知大多数Python工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!**
**因此收集整理了一份《2024年Python开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。**
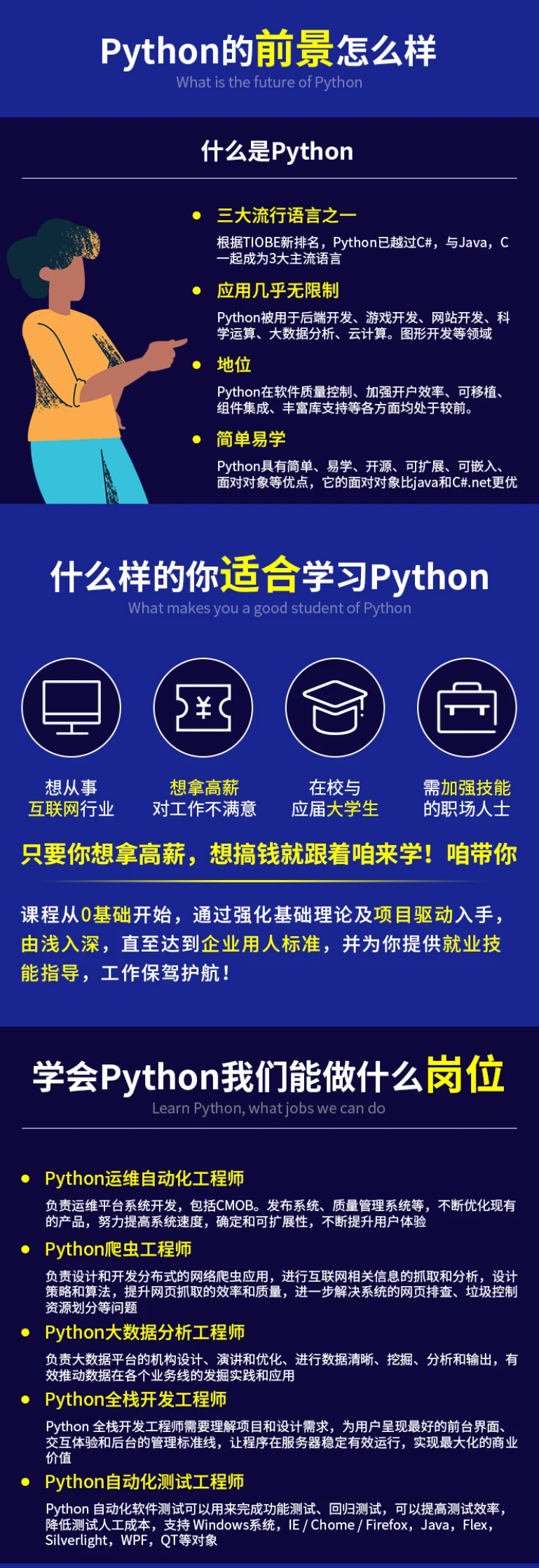
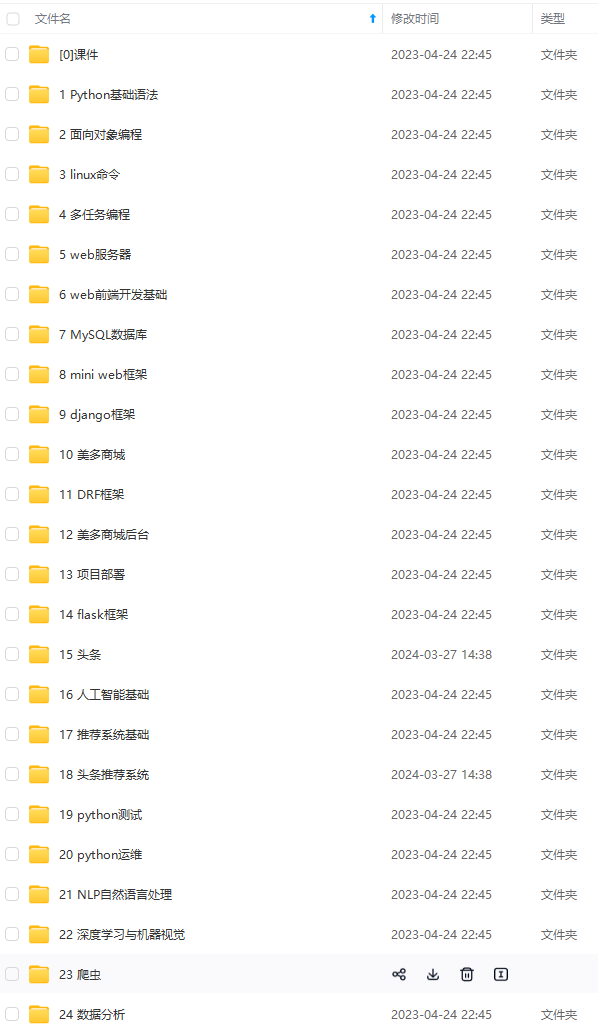
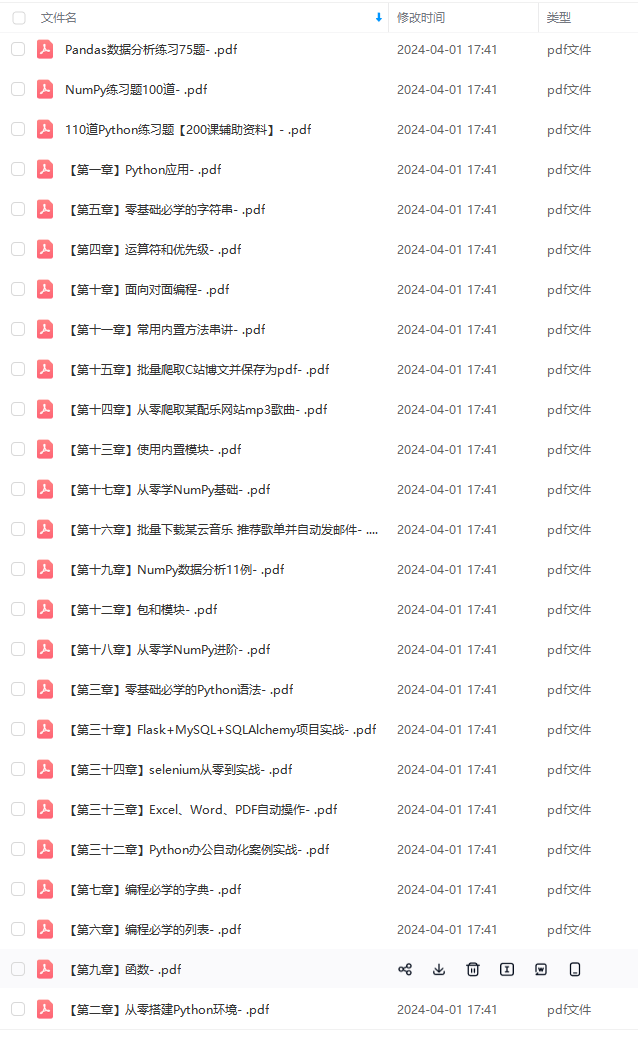
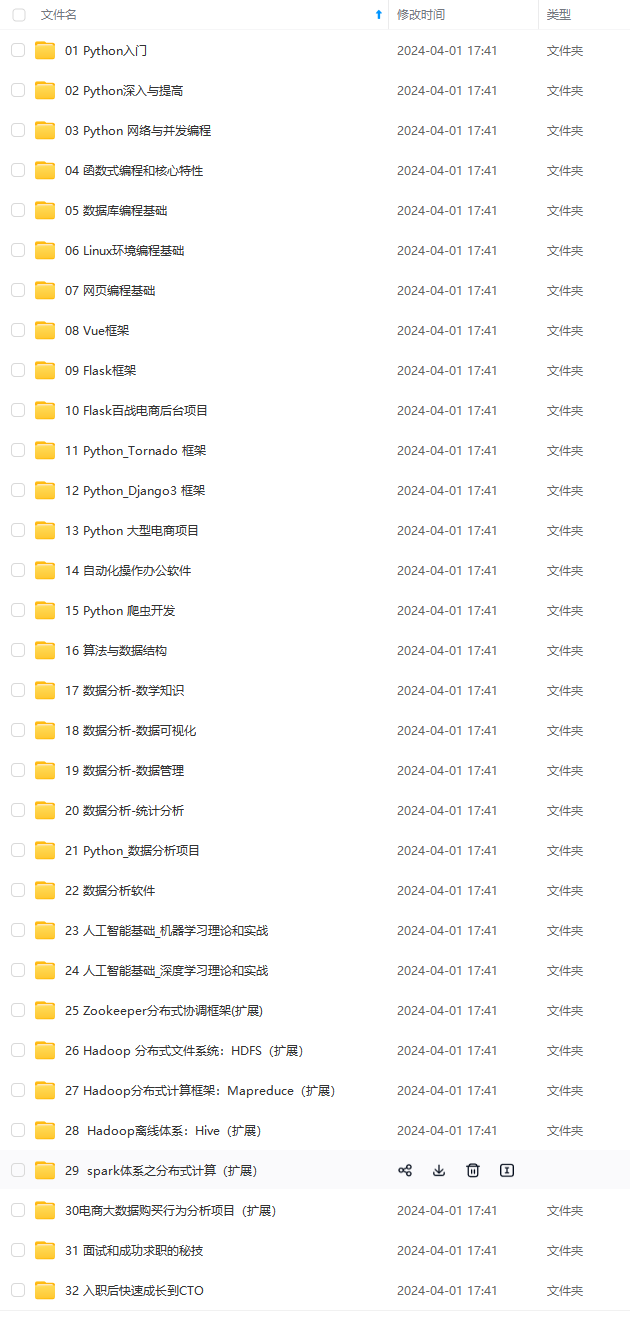
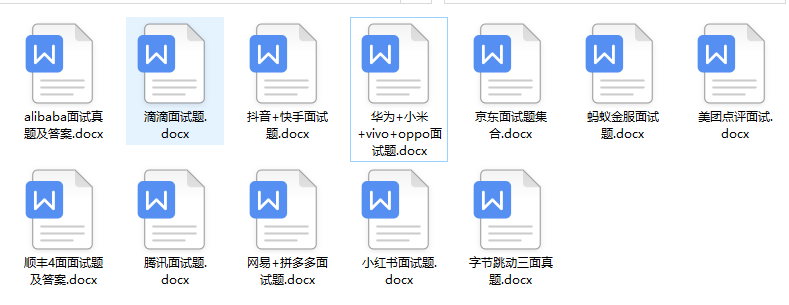
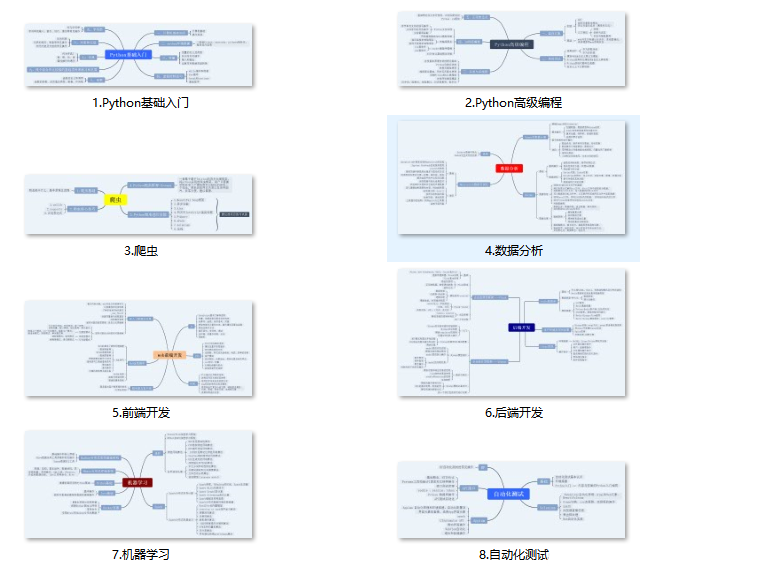
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上前端开发知识点,真正体系化!**
**由于文件比较大,这里只是将部分目录大纲截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且后续会持续更新**
**如果你觉得这些内容对你有帮助,可以扫码获取!!!(备注:Python)**
上海交大毕业,曾经在小公司待过,也去过华为、OPPO等大厂,18年进入阿里一直到现在。**
**深知大多数Python工程师,想要提升技能,往往是自己摸索成长或者是报班学习,但对于培训机构动则几千的学费,着实压力不小。自己不成体系的自学效果低效又漫长,而且极易碰到天花板技术停滞不前!**
**因此收集整理了一份《2024年Python开发全套学习资料》,初衷也很简单,就是希望能够帮助到想自学提升又不知道该从何学起的朋友,同时减轻大家的负担。**
[外链图片转存中...(img-REiClee8-1713550437154)]
[外链图片转存中...(img-VxAxmv4x-1713550437155)]
[外链图片转存中...(img-2sZ76uvn-1713550437155)]
[外链图片转存中...(img-JVf2J7c4-1713550437156)]
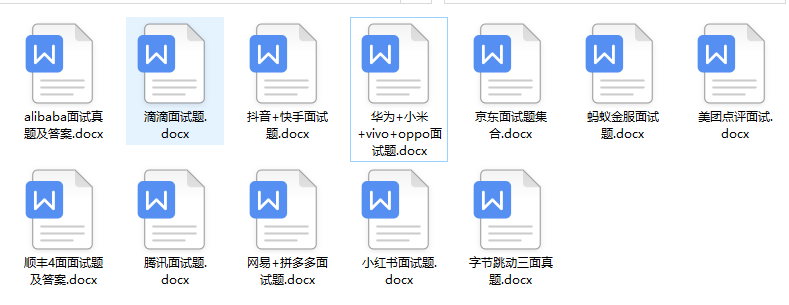
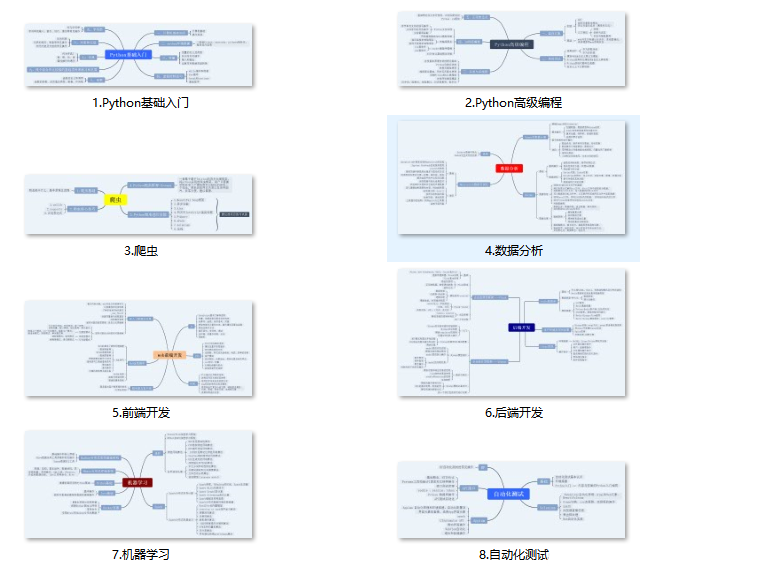
**既有适合小白学习的零基础资料,也有适合3年以上经验的小伙伴深入学习提升的进阶课程,基本涵盖了95%以上前端开发知识点,真正体系化!**
**由于文件比较大,这里只是将部分目录大纲截图出来,每个节点里面都包含大厂面经、学习笔记、源码讲义、实战项目、讲解视频,并且后续会持续更新**
**如果你觉得这些内容对你有帮助,可以扫码获取!!!(备注:Python)**
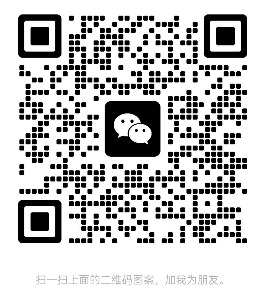