Spongebob is already tired trying to reason his weird actions and calculations, so he simply asked you to find all pairs of n and m, such that there are exactly x distinct squares in the table consisting of n rows and m columns. For example, in a 3 × 5 table there are 15squares with side one, 8 squares with side two and 3 squares with side three. The total number of distinct squares in a 3 × 5 table is15 + 8 + 3 = 26.
The first line of the input contains a single integer x (1 ≤ x ≤ 1018) — the number of squares inside the tables Spongebob is interested in.
First print a single integer k — the number of tables with exactly x distinct squares inside.
Then print k pairs of integers describing the tables. Print the pairs in the order of increasing n, and in case of equality — in the order of increasing m.
26
6 1 26 2 9 3 5 5 3 9 2 26 1
2
2 1 2 2 1
8
4 1 8 2 3 3 2 8 1
In a 1 × 2 table there are 2 1 × 1 squares. So, 2 distinct squares in total.
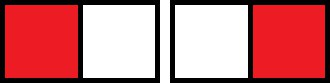
In a 2 × 3 table there are 6 1 × 1 squares and 2 2 × 2 squares. That is equal to 8 squares in total.
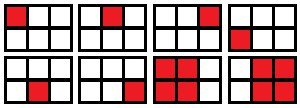
题意是给定一个X,问那些矩形中含有的正方形总数等于X。
这题当时没时间做了,(太弱。。。)后面补的。
官方题解:
第一点:n*m里面的正方形数量就是sum((n-i)*(m-i)),i从1到n-1啊。。。在纸上画几次就明白了。
第二点:从1到n的平方和等于n(n+1)(2n+1)/6。。。
然后就是枚举n,求m。
代码:
#pragma warning(disable:4996)
#include <iostream>
#include <algorithm>
#include <cmath>
#include <vector>
#include <string>
#include <cstring>
#include <map>
using namespace std;
typedef long long ll;
const int maxn = 2000005;
ll x;
ll a[maxn];
ll b[maxn];
int main()
{
//freopen("i.txt", "r", stdin);
//freopen("o.txt", "w", stdout);
int flag;
ll i, len, num, n, m, temp;
cin >> x;
flag = -1;
num = 0;
len = 2 * pow((double)x, ((double)1 / (double)3));
for (i = 1; i <= len+1; i++)
{
temp = 6 * x + i*i*i - i;
n = i*i + i;
if ((temp % (3 * n) == 0) && (i <= temp / (3 * n)))
{
a[num] = i;
b[num] = temp / (3 * n);
if (a[num] == b[num])
{
flag = num;
}
num++;
}
}
if (flag == -1)
{
cout << num * 2 << endl;
for (i = 0; i < num; i++)
{
cout << a[i] << " " << b[i] << endl;
}
for (i = num-1; i >= 0; i--)
{
cout << b[i] << " " << a[i] << endl;
}
}
else
{
cout << num * 2 - 1 << endl;
for (i = 0; i < num; i++)
{
cout << a[i] << " " << b[i] << endl;
}
for (i = num - 1; i >= 0; i--)
{
if (flag == i)
continue;
cout << b[i] << " " << a[i] << endl;
}
}
//system("pause");
return 0;
}