(1)HashMap,LinkedHashMap,TreeMap都属于Map;
(2)Map 主要用于存储键(key)值(value)对,根据键得到值,因此键不允许键重复,但允许值重复。
二、不同点:(1)HashMap里面存入的键值对在取出的时候是随机的,也是我们最常用的一个Map.它根据键的HashCode值存储数据,根据键可
以直接获取它的值,具有很快的访问速度。在Map 中插入、删除和定位元素,HashMap 是最好的选择。
(2)TreeMap取出来的是排序后的键值对。但如果您要按自然顺序或自定义顺序遍历键,那么TreeMap会更好。
(3) LinkedHashMap 是HashMap的一个子类,如果需要输出的顺序和输入的相同,那么用LinkedHashMap可以实现.
(4)HashMap 实际上是一个链表的数组。基于 HashMap 的链表方式实现机制,只要 HashCode() 和 Hash() 方法实现得足够好,能够尽可能地减少冲突的产生,那么对 HashMap 的操作几乎等价于对数组的随机访问操作,具有很好的性能。但是,如果 HashCode() 或者 Hash() 方法实现较差,在大量冲突产生的情况下,HashMap 事实上就退化为几个链表,对 HashMap 的操作等价于遍历链表,此时性能很差。
(5)HashMap 的一个功能缺点是它的无序性,被存入到 HashMap 中的元素,在遍历 HashMap 时,其输出是无序的。如果希望元素保持输入的顺序,可以使用 LinkedHashMap 替代。
(6)LinkedHashMap 继承自 HashMap,具有高效性,同时在 HashMap 的基础上,又在内部增加了一个链表,用以存放元素的顺序。
(7)HashMap 通过 hash 算法可以最快速地进行 Put() 和 Get() 操作。TreeMap 则提供了一种完全不同的 Map 实现。从功能上讲,TreeMap 有着比 HashMap 更为强大的功能,它实现了 SortedMap 接口,这意味着它可以对元素进行排序。TreeMap 的性能略微低于 HashMap。如果在开发中需要对元素进行排序,那么使用 HashMap 便无法实现这种功能,使用 TreeMap 的迭代输出将会以元素顺序进行。LinkedHashMap 是基于元素进入集合的顺序或者被访问的先后顺序排序,TreeMap 则是基于元素的固有顺序 (由 Comparator 或者 Comparable 确定)。
(8)LinkedHashMap 是根据元素增加或者访问的先后顺序进行排序,而 TreeMap 则根据元素的 Key 进行排序。
HashMap与HashTable对比:
(1)基类不同:HashTable基于Dictionary类,而HashMap是基于AbstractMap。Dictionary是什么?它是任何可将键映射到相应值的类的抽象父类,而AbstractMap是基于Map接口的骨干实现,它以最大限度地减少实现此接口所需的工作。
(2)null不同:HashMap可以允许存在一个为null的key和任意个为null的value,但是HashTable中的key和value都不允许为null。
(3)线程安全:HashMap时单线程安全的,Hashtable是多线程安全的。
(4)遍历不同:HashMap仅支持Iterator的遍历方式,Hashtable支持Iterator和Enumeration两种遍历方式。
//map
import java.util.*;
import java.util.Map.Entry;
public class MapTest{
public static void main(String[]args)
{
//(1)HashMap测试
Map<String,String>map=new HashMap<>();
map.put("a","1");
map.put("e","2");
map.put("f","3");
map.put("d","4");
System.out.println("HashMap:");
for(String key: map.keySet())
{
System.out.print(map.get(key)+" ");
}
System.out.println();
//(2)LinkedHashMap测试
Map<String,Integer>linkedHashMap=new LinkedHashMap<>();
linkedHashMap.put("a",1);
linkedHashMap.put("b",2);
linkedHashMap.put("f",3);
linkedHashMap.put("d",4);
System.out.println("LinkedHashMap:");
for(String key : linkedHashMap.keySet())
{
System.out.print(linkedHashMap.get(key)+" ");
}
System.out.println();
//(3)TreeMap测试
Map<String,Integer>treeMap=new TreeMap<>();
treeMap.put("a",1);
treeMap.put("e",2);
treeMap.put("f",3);
treeMap.put("d",4);
System.out.println("TreeMap:");
for(String key:treeMap.keySet())
{
System.out.print(treeMap.get(key)+" ");
}
System.out.println();
//(4)HashTable测试
Hashtable<String,Integer>hashtable=new Hashtable<>();
hashtable.put("a",1);
hashtable.put("f",2);
hashtable.put("b",3);
hashtable.put("c",4);
System.out.println("Hashtable:");
//(1)遍历方式
System.out.println(hashtable.toString());
//(2)foreach方式
for(String key:hashtable.keySet())
{
System.out.print(hashtable.get(key)+" ");
}
System.out.println();
//(3)迭代器模式
Iterator<String> iterator=hashtable.keySet().iterator();
while(iterator.hasNext())
{
String key=(String)iterator.next();
int value=hashtable.get(key);
System.out.print(value+" ");
}
System.out.println();
//(4)迭代器的第二种方式
Iterator<Entry<String,Integer>>iterator2=hashtable.entrySet().iterator();
while(iterator2.hasNext())
{
Map.Entry<String,Integer>entry=iterator2.next();
String key=entry.getKey();
int value=entry.getValue();
System.out.print(value+" ");
}
System.out.println();
//(5)通过枚举的方式
Enumeration<Integer>enumeration=hashtable.elements();
while(enumeration.hasMoreElements())
{
System.out.print(enumeration.nextElement()+" ");
}
System.out.println();
//(6)枚举方式的第二种
Enumeration<String>enumeration2=hashtable.keys();
while(enumeration2.hasMoreElements())
{
String key=enumeration2.nextElement();
System.out.print(hashtable.get(key)+" ");
}
}
}
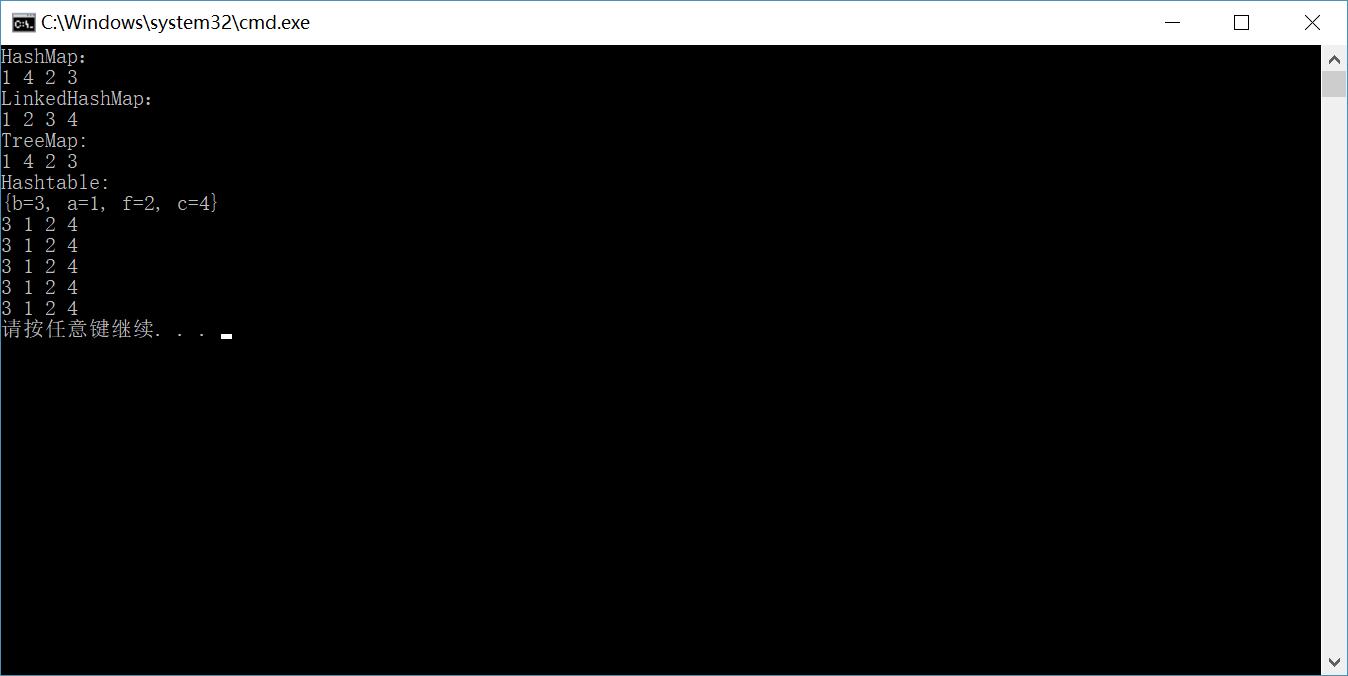