源代码
调试环境:
Ubuntu14.04
内核版本:3.13.0-32
代码参考《LINUX设备驱动程序》第三章 字符设备驱动程序
功能:向字符设备写入字符串;从字符设备读出字符串
scull.c
#include <linux/init.h>
#include <linux/module.h>
#include <linux/fs.h>
#include <linux/slab.h>
#include <linux/errno.h>
#include <linux/types.h>
#include <linux/cdev.h>
#include <asm/uaccess.h>
#include "scull.h"
int scull_major = SCULL_MAJOR;
int scull_minor = 0;
module_param(scull_major, int, S_IRUGO);
module_param(scull_minor, int, S_IRUGO);
struct scull_dev *scull_device;
int scull_trim(struct scull_dev *dev)
{
if (dev)
{
if (dev->data)
{
kfree(dev->data);
}
dev->data = NULL;
dev->size = 0;
}
return 0;
}
int scull_open(struct inode *inode, struct file *filp)
{
struct scull_dev *dev;
dev = container_of(inode->i_cdev, struct scull_dev, cdev);
filp->private_data = dev;
if ((filp->f_flags & O_ACCMODE) == O_WRONLY)
{
if (down_interruptible(&dev->sem))
{
return -ERESTARTSYS;
}
scull_trim(dev);
up(&dev->sem);
}
return 0;
}
int scull_release(struct inode *inode, struct file *filp)
{
return 0;
}
ssize_t scull_read(struct file *filp, char __user *buf, size_t count, loff_t *f_pos)
{
struct scull_dev *dev = filp->private_data;
ssize_t retval = 0;
if (down_interruptible(&dev->sem))
{
return -ERESTARTSYS;
}
if (*f_pos >= dev->size)
{
goto out;
}
if (*f_pos + count > dev->size)
{
count = dev->size - *f_pos;
}
if (!dev->data)
{
goto out;
}
if (copy_to_user(buf, dev->data + *f_pos, count))
{
retval = -EFAULT;
goto out;
}
*f_pos += count;
retval = count;
out:
up(&dev->sem);
return retval;
}
ssize_t scull_write(struct file *filp, const char __user *buf, size_t count, loff_t *f_pos)
{
struct scull_dev *dev = filp->private_data;
ssize_t retval = -ENOMEM;
if (down_interruptible(&dev->sem))
{
return -ERESTARTSYS;
}
if (!dev->data)
{
dev->data = kmalloc(SCULL_BUFFER_SIZE, GFP_KERNEL);
if (!dev->data)
{
goto out;
}
memset(dev->data, 0, SCULL_BUFFER_SIZE);
}
if (count > SCULL_BUFFER_SIZE - dev->size)
{
count = SCULL_BUFFER_SIZE - dev->size;
}
if (copy_from_user(dev->data + dev->size, buf, count))
{
retval = -EFAULT;
goto out;
}
dev->size += count;
retval = count;
out:
up(&dev->sem);
return retval;
}
loff_t scull_llseek(struct file *filp, loff_t off, int whence)
{
struct scull_dev *dev = filp->private_data;
loff_t newpos;
switch(whence)
{
case 0:
newpos = off;
break;
case 1:
newpos = filp->f_pos + off;
break;
case 2:
newpos = dev->size + off;
break;
default:
return -EINVAL;
}
if (newpos < 0)
{
return -EINVAL;
}
filp->f_pos = newpos;
return newpos;
}
struct file_operations scull_fops = {
.owner = THIS_MODULE,
.llseek = scull_llseek,
.read = scull_read,
.write = scull_write,
.open = scull_open,
.release = scull_release,
};
void scull_cleanup_module(void)
{
dev_t devno = MKDEV(scull_major, scull_minor);
if (scull_device)
{
scull_trim(scull_device);
cdev_del(&scull_device->cdev);
kfree(scull_device);
}
unregister_chrdev_region(devno, 1);
}
static void scull_setup_cdev(struct scull_dev *dev)
{
int err, devno = MKDEV(scull_major, scull_minor);
cdev_init(&dev->cdev, &scull_fops);
dev->cdev.owner = THIS_MODULE;
dev->cdev.ops = &scull_fops;
err = cdev_add(&dev->cdev, devno, 1);
if (err)
{
printk(KERN_NOTICE "Error %d adding scull", err);
}
}
static int __init scull_init_module(void)
{
int result;
dev_t dev = 0;
if (scull_major)
{
dev = MKDEV(scull_major, scull_minor);
result = register_chrdev_region(dev, 1, "scull");
}
else
{
result = alloc_chrdev_region(&dev, scull_minor, 1, "scull");
scull_major = MAJOR(dev);
}
if (result < 0)
{
printk(KERN_WARNING "scull: can't get major %d\n", scull_major);
return result;
}
scull_device = kmalloc(sizeof(struct scull_dev), GFP_KERNEL);
if (!scull_device)
{
result = -ENOMEM;
goto fail;
}
memset(scull_device, 0, sizeof(struct scull_dev));
//源代码中使用init_MUTEX(&scull_device->sem)函数,此函数被新内核版本废除
//故使用sema_init(&scull_device->sem,1)代替,达到相同效果
sema_init(&scull_device->sem,1);
scull_setup_cdev(scull_device);
return 0;
fail:
scull_cleanup_module();
return result;
}
module_init(scull_init_module);
module_exit(scull_cleanup_module);
MODULE_LICENSE("GPL");
scull.h
#ifndef _SCULL_H
#define _SCULL_H
#define SCULL_MAJOR 0
#define SCULL_BUFFER_SIZE PAGE_SIZE
struct scull_dev {
char *data;
unsigned long size;
struct semaphore sem;
struct cdev cdev;
};
#endif
Makefile
obj-m += scull.o
CURRENT_PATH:=$(shell pwd)
LINUX_KERNEL:=$(shell uname -r)
LINUX_KERNEL_PATH:=/lib/modules/$(LINUX_KERNEL)/build
all:
make -C $(LINUX_KERNEL_PATH) M=$(CURRENT_PATH) modules
clean:
make -C $(LINUX_KERNEL_PATH) M=$(CURRENT_PATH) clean
编译源代码
-
编译驱动程序代码,即执行 make 命令,编译结果如图。
-
依次执行以下命令,安装字符设备驱动程序
-
设备字符驱动程序安装成功
测试
向设备驱动程序scull中写入“hello,linux”,并从设备驱动程序中读出来。(如图)
sudo su //获取系统超级权限
echo hello,linux > scull //向字符设备驱动程序中写入字符串“hello,linux”
cat scull //读出写入的字符
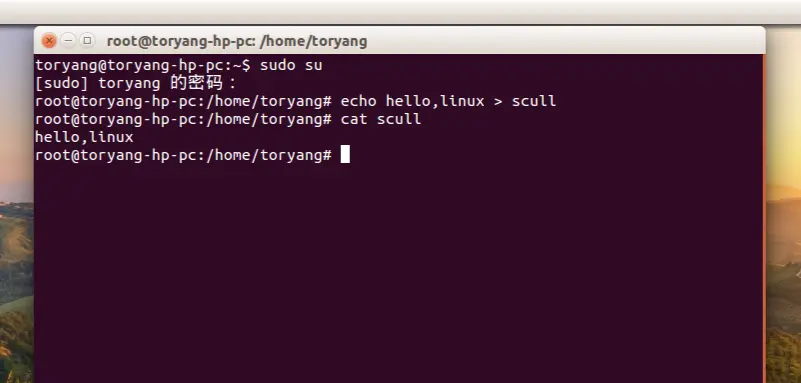
后记
本次实验是我第一次深入地了解Linux系统,确实学到了很多东西。以前虽然也在用linux,知道一些Ubuntu下的基本命令,但是那时只是将linux当做windows的代替品来用,只知道一味地使用linux下的应用程序软件做开发,而不是真正地使用linux。
本次实验在做源码编译和设备驱动程序编写的同时,了解到了一些linux操作系统的底层知识,更加体会到了linux和window在一些方面地差异。
在接下来使用linux系统的时候我会不断地深入了解该系统,学习真正使用linux操作系统。
文/Torang(简书作者)
原文链接:http://www.jianshu.com/p/5f22f179bf8f
著作权归作者所有,转载请联系作者获得授权,并标注“简书作者”。
原文链接:http://www.jianshu.com/p/5f22f179bf8f
著作权归作者所有,转载请联系作者获得授权,并标注“简书作者”。