#include "cstdio"
#include "queue"
#define MAX 20
#define INF 999
using namespace std;
int n;
int w[MAX];
int bestx[MAX];
int c;
int r[MAX];
struct bnode
{
struct bnode *parent;
int left;
};
struct heapNode
{
struct bnode *b;
int level;
int uweight;
bool operator < (const heapNode &hn) const
{
return uweight < hn.uweight;
}
};
priority_queue<heapNode> pq;
void enHeap(int uw, bnode *parent, int isLeft, int i)
{
bnode *b = (bnode *)malloc(sizeof(bnode));
b->parent = parent;
b->left = isLeft;
heapNode hn;
hn.level = i;
hn.uweight = uw;
hn.b = b;
pq.push(hn);
}
void init(int n1, int c1, int weight[])
{
n = n1;
c = c1;
int i;
for(i=1; i<=n; i++)
w[i] = weight[i];
r[n] = 0;
for(i=n-1; i>0; i--)
r[i] = w[i+1] + r[i+1];
}
int maxLoading()
{
bnode *e = NULL;
heapNode hn;
int i = 1;
int ew = 0;
while(i!=n+1)
{
if(ew+w[i]<=c)
enHeap(ew+w[i]+r[i], e, 1, i+1);
enHeap(ew+r[i], e, 0, i+1);
hn = pq.top();
pq.pop();
ew = hn.uweight - r[i];
e = hn.b;
i = hn.level;
}
for(i=n; i>0; i--)
{
bestx[i] = e->left;
e = e->parent;
}
return ew;
}
int main()
{
int n1 = 4;
int c1 = 70;
int weight[] = {0, 20, 10, 26, 15};
init(n1, c1, weight);
printf("船载重为:%d\n", c1);
int sum = maxLoading();
printf("集装箱重量为:\n");
int i;
for(i=1; i<=n; i++)
printf("%d ", w[i]);
printf("\n最优载重为:%d\n", sum);
return 0;
}
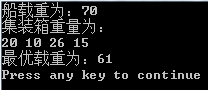