- 设置控件的四边圆角
UIView *subView = [[UIView alloc] initWithFrame:CGRectMake(100, 100, 100, 100)];
subView.backgroundColor = [UIColor cyanColor];
subView.layer.cornerRadius = 20;
subView.layer.masksToBounds = YES;
[self.view addSubview:subView];
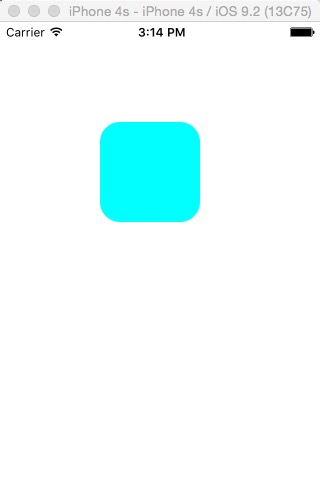
- 设置控件的自定义哪边的圆角
UIView *subView = [[UIView alloc] initWithFrame:CGRectMake(100, 100, 100, 100)];
subView.backgroundColor = [UIColor cyanColor];
/**参数解释
rect: 控件的frame
corners: 圆角的点
UIRectCornerTopLeft = 1 << 0,
UIRectCornerTopRight = 1 << 1,
UIRectCornerBottomLeft = 1 << 2,
UIRectCornerBottomRight = 1 << 3,
cornerRadii: 圆角弧度
*/
UIBezierPath *path = [UIBezierPath bezierPathWithRoundedRect:subView.bounds byRoundingCorners:UIRectCornerTopLeft | UIRectCornerBottomRight cornerRadii:CGSizeMake(20, 20)];
CAShapeLayer *layer = [[CAShapeLayer alloc] init];
layer.frame = subView.bounds;
layer.path = path.CGPath;
subView.layer.mask = layer;
[self.view addSubview:subView];
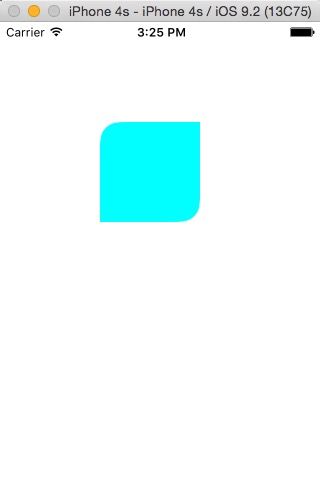
- 设置富文本
NSShadow *shadow = [[NSShadow alloc] init];
shadow.shadowColor = [UIColor purpleColor];
shadow.shadowBlurRadius = 10;
NSDictionary * dic = @{NSFontAttributeName:[UIFont fontWithName:@"AmericanTypewriter" size:20],
NSForegroundColorAttributeName:[UIColor redColor],
NSUnderlineStyleAttributeName:@(NSUnderlineStyleSingle),
NSShadowAttributeName:shadow
};
NSMutableAttributedString * attributeStr = [[NSMutableAttributedString alloc] initWithString:@"2222我是一个富文本,zheli很多属性,666I just di it。66666清除属性."];
[attributeStr setAttributes:dic range:NSMakeRange(0, attributeStr.length)];
[attributeStr addAttribute:NSFontAttributeName value:[UIFont systemFontOfSize:30] range:NSMakeRange(9, 10)];
[attributeStr addAttribute:NSForegroundColorAttributeName value:[UIColor cyanColor] range:NSMakeRange(13, 13)];
NSDictionary * dicAdd = @{NSBackgroundColorAttributeName:[UIColor yellowColor],NSLigatureAttributeName:@1};
[attributeStr addAttributes:dicAdd range:NSMakeRange(19, 13)];
[attributeStr removeAttribute:NSFontAttributeName range:NSMakeRange(32, 9)];
UILabel * label = [[UILabel alloc] initWithFrame:CGRectMake(100, 100, 200, 400)];
label.numberOfLines = 0;
label.attributedText = attributeStr;
[self.view addSubview:label];
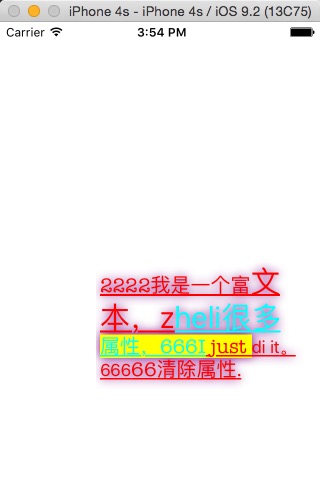
- 是否包含表情字符
+ (BOOL)isContainsEmoji:(NSString *)string
{
__block BOOL isEomji = NO;
[string enumerateSubstringsInRange:NSMakeRange(0, [string length]) options:NSStringEnumerationByComposedCharacterSequences usingBlock:
^(NSString *substring, NSRange substringRange, NSRange enclosingRange, BOOL *stop) {
const unichar hs = [substring characterAtIndex:0];
if (0xd800 <= hs && hs <= 0xdbff) {
if (substring.length > 1) {
const unichar ls = [substring characterAtIndex:1];
const int uc = ((hs - 0xd800) * 0x400) + (ls - 0xdc00) + 0x10000;
if (0x1d000 <= uc && uc <= 0x1f77f) {
isEomji = YES;
}
}
} else if (substring.length > 1) {
const unichar ls = [substring characterAtIndex:1];
if (ls == 0x20e3) {
isEomji = YES;
}
} else {
if (0x2100 <= hs && hs <= 0x27ff && hs != 0x263b) {
isEomji = YES;
} else if (0x2B05 <= hs && hs <= 0x2b07) {
isEomji = YES;
} else if (0x2934 <= hs && hs <= 0x2935) {
isEomji = YES;
} else if (0x3297 <= hs && hs <= 0x3299) {
isEomji = YES;
} else if (hs == 0xa9 || hs == 0xae || hs == 0x303d || hs == 0x3030 || hs == 0x2b55 || hs == 0x2b1c || hs == 0x2b1b || hs == 0x2b50|| hs == 0x231a ) {
isEomji = YES;
}
}
}];
return isEomji;
}
- 获取文本内容是否含有电话号码
+ (NSMutableArray *)getMobileByStr:(NSString *)strContent
{
NSMutableArray *arrUrl = [NSMutableArray array];
if(strContent && strContent.length > 0)
{
NSString *regulaStr = @"\\d{7,23}";
NSRegularExpression *regex = [NSRegularExpression regularExpressionWithPattern:regulaStr
options:NSRegularExpressionCaseInsensitive error:nil];
NSArray *arrayOfAllMatches = [regex matchesInString:strContent
options:0
range:NSMakeRange(0, [strContent length])];
for (NSTextCheckingResult *match in arrayOfAllMatches)
{
NSString* substringForMatch = [strContent substringWithRange:match.range];
[arrUrl addObject:substringForMatch];
}
}
return arrUrl;
}
- 获取文本内容是否含有链接
+ (NSMutableArray *)getUrlByStr:(NSString *)strContent
{
NSMutableArray *arrUrl = [NSMutableArray array];
if(strContent && strContent.length > 0)
{
NSString *regulaStr = @"((http[s]{0,1}|ftp)://[a-zA-Z0-9\\.\\-]+\\.([a-zA-Z]{2,4})(:\\d+)?(/[a-zA-Z0-9\\.\\-~!@#$%^&*+?:_/=<>]*)?)|(www.[a-zA-Z0-9\\.\\-]+\\.([a-zA-Z]{2,4})(:\\d+)?(/[a-zA-Z0-9\\.\\-~!@#$%^&*+?:_/=<>]*)?)|([a-zA-Z0-9\\.\\-]+\\.([a-zA-Z]{2,4})(:\\d+)?(/[a-zA-Z0-9\\.\\-~!@#$%^&*+?:_/=<>]*)?)";
NSRegularExpression *regex = [NSRegularExpression regularExpressionWithPattern:regulaStr
options:NSRegularExpressionCaseInsensitive error:nil];
NSArray *arrayOfAllMatches = [regex matchesInString:strContent
options:0
range:NSMakeRange(0, [strContent length])];
for (NSTextCheckingResult *match in arrayOfAllMatches)
{
NSString* substringForMatch = [strContent substringWithRange:match.range];
[arrUrl addObject:substringForMatch];
}
}
return arrUrl;
}
- 获取设备类型
typedef NS_ENUM(NSInteger,DeviceType) {
DeviceTypeFor5SAndAbove = 1,
DeviceTypeFor6,
DeviceTypeFor6p,
};
+(DeviceType)getDeviceType
{
DeviceType type;
CGRect bounds = [[UIScreen mainScreen] bounds];
CGFloat height=bounds.size.height;
CGFloat scale=[UIScreen mainScreen].scale;
if(height < 667){
type = DeviceTypeFor5SAndAbove;
}
else if(scale < 2.9){
type = DeviceTypeFor6;
}
else{
type = DeviceTypeFor6p;
}
return type;
}
- 计算指定时间与当前的时间差
/**
* 计算指定时间与当前的时间差
*
* @param compareDateString 时间字符串(格式:2013-3-2 8:28:53)
*
* @return (比如,3分钟前,1个小时前,2015年1月10日)
*
* ADD BY ZHANGZH
*/
+ (NSString *)compareCurrentTime:(NSString *)compareDateString
{
if(compareDateString.length > 23){
compareDateString = [compareDateString substringToIndex:23];
}
NSDateFormatter *formatter = [[NSDateFormatter alloc] init];
[formatter setDateFormat:@"yyyy-MM-dd'T'HH:mm:ss"];
NSDate *compareDate = [formatter dateFromString:compareDateString];
if(compareDate==nil)
{
[formatter setDateFormat:@"yyyy-MM-dd'T'HH:mm:ss.SSS"];
compareDate= [formatter dateFromString:compareDateString];;
}
NSTimeInterval timeInterval=[compareDate timeIntervalSinceNow];
timeInterval=-timeInterval;
long temp=0;
NSString *compareResult;
if(timeInterval<60)
{
compareResult=[NSString stringWithFormat:@"1分钟前"];
}
else if((temp=timeInterval/60)<60)
{
compareResult=[NSString stringWithFormat:@"%i分钟前",(int)temp];
}
else if((temp=temp/60)<24)
{
compareResult=[NSString stringWithFormat:@"%i小时前",(int)temp];
}
else
{
compareResult = @"一天前";
}
return compareResult;
}