一、演示效果
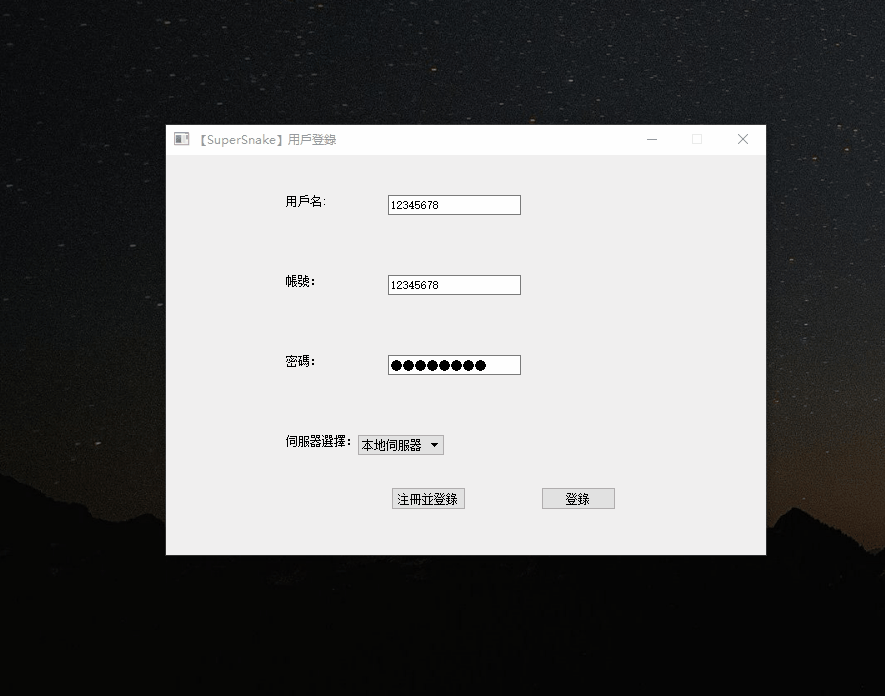
二、核心代码
#include "Food.h"
#include <QTime>
#include <time.h>
#include "Snake.h"
Food::Food(int foodSize):foodSize(foodSize)
{
coordinate.x = -1;
coordinate.y = -1;
qsrand(time(NULL));
}
void Food::createFood(int map_row,int map_col,vector<Point> snakeCoords){
int foodX = qrand()%map_col*foodSize;
int foodY = qrand()%map_row*foodSize;
while(foodX == coordinate.x && foodY == coordinate.y){
foodX = qrand()%map_col*foodSize;
foodY = qrand()%map_row*foodSize;
}
while(1){
bool flag = true;
for(auto& e:snakeCoords){
if(e.x == foodX && e.y == foodY){
foodX = qrand()%map_col*foodSize;
foodY = qrand()%map_row*foodSize;
flag = false;
break;
}
}
if(flag)break;
}
coordinate.x = foodX;
coordinate.y = foodY;
}
Point Food::getCoor()const{
return this->coordinate;
}
#include "MapScene.h"
#include <QDebug>
#include <QMessageBox>
#include <QPushButton>
#include <vector>
#include <QPainter>
#include "data.h"
#include <QJsonDocument>
#include <QJsonObject>
#include "User.h"
#include <QDateTime>
#include "NetworkManager.h"
MapScene::MapScene(QWidget *parent,int row,int col,Snake* snake,int speed) : BaseScene(parent),row(row),col(col),snake(snake),speed(speed)
{
QFile cssFile;
cssFile.setFileName("./css/mapScene.css");
cssFile.open(QIODevice::ReadOnly);
QString styleSheet = cssFile.readAll();
cssFile.close();
this->setStyleSheet(styleSheet);
int mapWidth = col*snake->getSize(),mapHeight = row*snake->getSize();
int controlBarHeight = 50;
this->setFixedSize(mapWidth,mapHeight+controlBarHeight);
this->setWindowTitle(u8"【SuperSnake】遊戲界面");
this->initControlBar(mapWidth,mapHeight,controlBarHeight);
this->initMap();
QMessageBox::information(this,u8"提示",u8"【操作說明】使用W、A、S、D改變蛇的運動方向(注:WASD對應上下左右)");
connect(gameTimer,&QTimer::timeout,this,&MapScene::onGameRunning);
}
bool MapScene::updateRankList(){
QFile rankListFile;
rankListFile.setFileName(rankListPath);
rankListFile.open(QIODevice::ReadOnly);
QByteArray rankListData = rankListFile.readAll();
QJsonDocument jsonDoc = QJsonDocument::fromJson(rankListData);
QJsonObject jsonObj = jsonDoc.object();
QString userId = User::getCurrentUserId();
QString userName = User::getCurrentUserName();
QString speed = QString::number(this->speed);
QDateTime current_date_time =QDateTime::currentDateTime();
QString current_date =current_date_time.toString("yyyy-MM-dd");
QJsonObject speedObj = jsonObj[speed].toObject();
if(!speedObj.contains(userId)){
QJsonObject newRankRecord;
newRankRecord.insert("userName",userName);
newRankRecord.insert("maxScore",score);
newRankRecord.insert("date",current_date);
speedObj.insert(userId,newRankRecord);
}else{
int maxScore = speedObj[userId].toObject()["maxScore"].toInt();
if(score<=maxScore){
rankListFile.close();
return false;
}
QJsonObject userIdObj = speedObj[userId].toObject();
userIdObj["maxScore"] = score;
userIdObj["date"] = current_date;
speedObj[userId] = userIdObj;
}
rankListFile.close();
rankListFile.open(QIODevice::WriteOnly);
jsonObj[speed] = speedObj;
jsonDoc.setObject(jsonObj);
rankListFile.write(jsonDoc.toJson());
rankListFile.close();
return true;
}
bool MapScene::updateRankList(QString url){
QByteArray rankListData = NetworkManager::get(url);
QJsonDocument jsonDoc = QJsonDocument::fromJson(rankListData);
QJsonObject jsonObj = jsonDoc.object();
QString userId = User::getCurrentUserId();
QString userName = User::getCurrentUserName();
QString speed = QString::number(this->speed);
QDateTime current_date_time = QDateTime::currentDateTime();
QString current_date =current_date_time.toString("yyyy-MM-dd");
QJsonObject speedObj = jsonObj[speed].toObject();
if(!speedObj.contains(userId)){
QJsonObject newRankRecord;
newRankRecord.insert("userName",userName);
newRankRecord.insert("maxScore",score);
newRankRecord.insert("date",current_date);
speedObj.insert(userId,newRankRecord);
}else{
int maxScore = speedObj[userId].toObject()["maxScore"].toInt();
if(score<=maxScore){
return false;
}
QJsonObject userIdObj = speedObj[userId].toObject();
userIdObj["maxScore"] = score;
userIdObj["date"] = current_date;
speedObj[userId] = userIdObj;
}
jsonObj[speed] = speedObj;
jsonDoc.setObject(jsonObj);
NetworkManager::put(url,jsonDoc);
return true;
}
void MapScene::onGameRunning(){
snake->move();
moveFlag = true;
int snakeSize = snake->getSize();
std::vector<Point> snakeCoords = snake->getCoords();
Point foodCoord = food->getCoor();
int snakeNum = snake->getNum();
if(isSnakeEat(snakeCoords,foodCoord)){
snake->addNum();
food->createFood(this->row,this->col,snakeCoords);
score+=100;
scoreLabel->setText(QString(u8"分數:%1").arg(score));
scoreLabel->adjustSize();
snake->setSnakeColor(QColor(rand()%256,rand()%256,rand()%256));
}
if(isSnakeDead(snakeCoords,snakeSize,snakeNum)){
gameTimer->stop();
QString server = User::getCurrentServer();
NetworkManager nw;
bool ret;
if(server == "local"){
ret = updateRankList();
}
else{
nw.createLoadDialog();
ret = updateRankList(webJsonUrl_RL);
}
QString resultStr = QString(u8"你的分數為:%1").arg(score);
if(!ret){
resultStr+=" >>排行榜沒有任何改變@@<<";
}else{
resultStr+=" >>排行榜已更新^.^<<";
}
this->initMap();
if(server == u8"web")nw.closeLoadDialog();
QMessageBox::information(this,u8"遊戲結束",resultStr);
}
update();
}
void MapScene::initControlBar(int mapWidth,int mapHeight,int controlBarHeight){
QPushButton* startGameBtn = new QPushButton(u8"開始遊戲",this);
startGameBtn->setFont(QFont(u8"Adobe 楷体 Std R",14));
startGameBtn->adjustSize();
startGameBtn->move(mapWidth*0.03,mapHeight+controlBarHeight/2-startGameBtn->height()/2);
connect(startGameBtn,&QPushButton::clicked,[this](){
gameTimer->start();
});
QPushButton* pauseGameBtn = new QPushButton(u8"暫停遊戲",this);
pauseGameBtn->setFont(QFont(u8"Adobe 楷体 Std R",14));
pauseGameBtn->adjustSize();
pauseGameBtn->move(mapWidth*0.05+startGameBtn->width()+10,mapHeight+controlBarHeight/2-pauseGameBtn->height()/2);
connect(pauseGameBtn,&QPushButton::clicked,[this](){
gameTimer->stop();
});
QPushButton* backBtn = new QPushButton(u8"返回",this);
backBtn->setFont(QFont(u8"Adobe 楷体 Std R",14));
backBtn->adjustSize();
backBtn->move(mapWidth*0.95-backBtn->width(),mapHeight+controlBarHeight/2-backBtn->height()/2);
connect(backBtn,&QPushButton::clicked,[this](){
gameTimer->stop();
this->close();
emit backToSettingScene();
});
scoreLabel = new QLabel(u8"分數:0",this);
scoreLabel->setFont(QFont(u8"Adobe 楷体 Std R",14));
scoreLabel->adjustSize();
scoreLabel->move(mapWidth*0.05+startGameBtn->width()+pauseGameBtn->width()+20,mapHeight+controlBarHeight/2-scoreLabel->height()/2);
}
void MapScene::drawSnake(QPainter& painter,std::vector<Point>& snakeCoords,int snakeNum,int snakeSize){
QColor snakeColor = snake->getSnakeColor();
painter.setPen(QPen(snakeColor));
painter.setBrush(QBrush(snakeColor));
for(int i = 0;i<snakeNum;i++){
painter.drawRect(snakeCoords[i].x,snakeCoords[i].y,snakeSize,snakeSize);
}
}
void MapScene::drawFood(QPainter& painter,int snakeSize){
painter.setPen(QColor());
QBrush brush(QColor(255,255,0));;
painter.setBrush(brush);
Point foodCoor = food->getCoor();
painter.drawEllipse(foodCoor.x,foodCoor.y,snakeSize,snakeSize);
}
bool MapScene::isSnakeDead(std::vector<Point>& snakeCoords,int& snakeSize,int& snakeNum){
if(snakeCoords[0].x<0 || snakeCoords[0].x>=this->col*snakeSize || snakeCoords[0].y<0 || snakeCoords[0].y>=this->row*snakeSize )return true;
for(int i = 1;i < snakeNum;i++){
if(snakeCoords[0].x == snakeCoords[i].x && snakeCoords[0].y == snakeCoords[i].y )return true;
}
return false;
}
bool MapScene::isSnakeEat(std::vector<Point>& snakeCoords,Point& foodCoord){
if(snakeCoords[0].x == foodCoord.x && snakeCoords[0].y == foodCoord.y)return true;
return false;
}
void MapScene::paintEvent(QPaintEvent* event){
QPainter painter(this);
int snakeSize = snake->getSize();
std::vector<Point> snakeCoords = snake->getCoords();
int snakeNum = snake->getNum();
painter.drawLine(0,row*snakeSize,col*snakeSize,row*snakeSize);
drawFood(painter,snakeSize);
drawSnake(painter,snakeCoords,snakeNum,snakeSize);
}
void MapScene::changeSnakeDir(QKeyEvent* event){
int snakeDir = snake->getDir();
switch(event->key()){
case Qt::Key_W:
if(snakeDir!=DOWN){
snake->setDir(UP);
moveFlag = false;
}
break;
case Qt::Key_A:
if(snakeDir!=RIGHT){
snake->setDir(LEFT);
moveFlag = false;
}
break;
case Qt::Key_S:
if(snakeDir!=UP){
snake->setDir(DOWN);
moveFlag = false;
}
break;
case Qt::Key_D:
if(snakeDir!=LEFT){
snake->setDir(RIGHT);
moveFlag = false;
}
break;
}
}
void MapScene::keyPressEvent(QKeyEvent* event){
if(!moveFlag)return;
changeSnakeDir(event);
}
void MapScene::initMap(){
if(!gameTimer)gameTimer = new QTimer(this);
gameTimer->setInterval(100/speed);
if(!food)food = new Food(snake->getSize());
food->createFood(this->row,this->col,snake->getCoords());
snake->init();
score = 0;
scoreLabel->setText(QString(u8"分數:%1").arg(score));
scoreLabel->adjustSize();
}
MapScene::~MapScene(){
if(food!=nullptr){
delete food;
food = nullptr;
}
}
三、下载链接
https://download.csdn.net/download/u013083044/89656909