欢迎关注“程序杂货铺”公众号,里面有精彩内容,欢迎大家收看^_^
设计模式笔记
1、单例模式
定义:
确保一个类只有一个实例,并提供一个全局访问点。
类图:
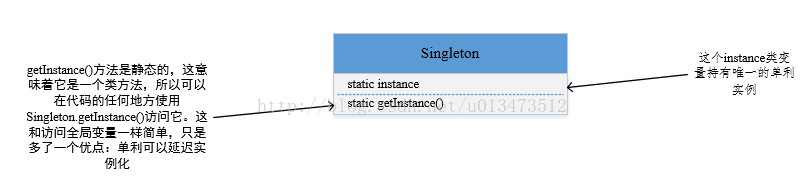
源代码:
public class Singleton {
private static Singleton instance = null;
private Singleton(){
}
public static Singleton getInstance() {
if(instance == null){
synchronized (Singleton.class) {
instance = new Singleton();
}
}
return instance;
}
}
2、工厂模式
定义:
工厂模式定义了一个创建对象的接口,由子类决定要实例化的类是哪一个。工厂方法让类把实例化推迟到子类。
(1). 简单工厂模式
类图:
源代码:
public abstract class Product {
public void method1() {
}
public void method2() {
}
}
public class Product_A extends Product{
public Product_A() {
}
public void method1() {
System.out.println("this is method1 in Product_A");
}
public void method2() {
System.out.println("this is method2 in Product_A");
}
}
public class Product_B extends Product{
public Product_B() {
}
public void method1() {
System.out.println("this is method1 in Product_B");
}
public void method2() {
System.out.println("this is method2 in Product_B");
}
}
public class SimpleFactory {
public Product createProduct(String type) {
Product product = null;
if(type.equals("Product_A")){
product = new Product_A();
}else if (type.equals("Product_B")) {
product = new Product_B();
}
return product;
}
}
(2). 抽象工厂模式
类图:
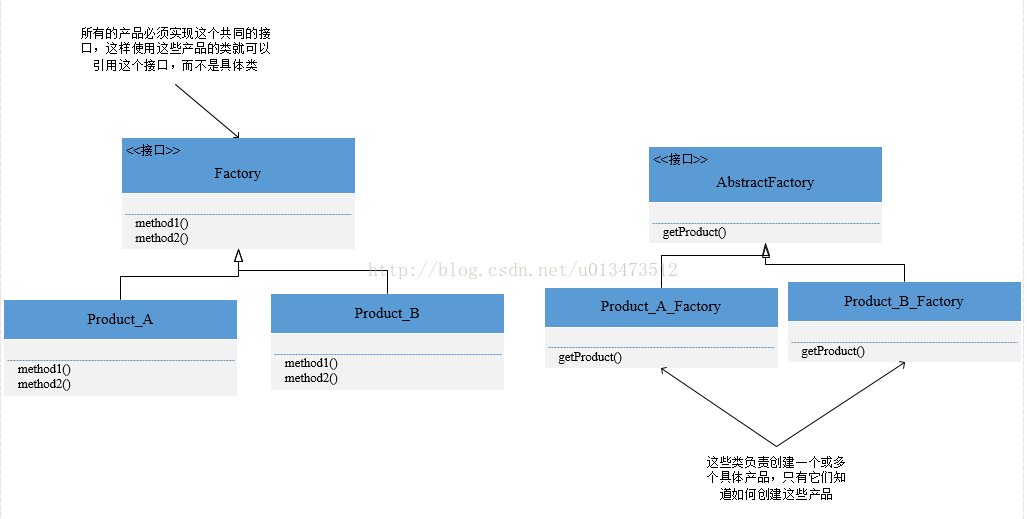
源代码:
public interface Product {
public void method1();
public void method2();
}
public class Product_A implements Product {
@Override
public void method1() {
System.out.println("this is method1 in Product_A");
}
@Override
public void method2() {
System.out.println("this is method2 in Product_A");
}
}
public class Product_B implements Product {
@Override
public void method1() {
System.out.println("this is method1 in Product_B");
}
@Override
public void method2() {
System.out.println("this is method2 in Product_B");
}
}
public interface AbstractFactory {
public Product getProduct();
}
public class Product_A_Factoty implements AbstractFactory {
@Override
public Product getProduct() {
return new Product_A();
}
}
public class Product_B_Factory implements AbstractFactory {
@Override
public Product getProduct() {
return new Product_B();
}
}
3、策略模式
定义:
策略模式定义了算法族,分别将其封装起来,让它们之间可以相互替换,此模式让算法的变化独立于使用算法的客户。
类图:
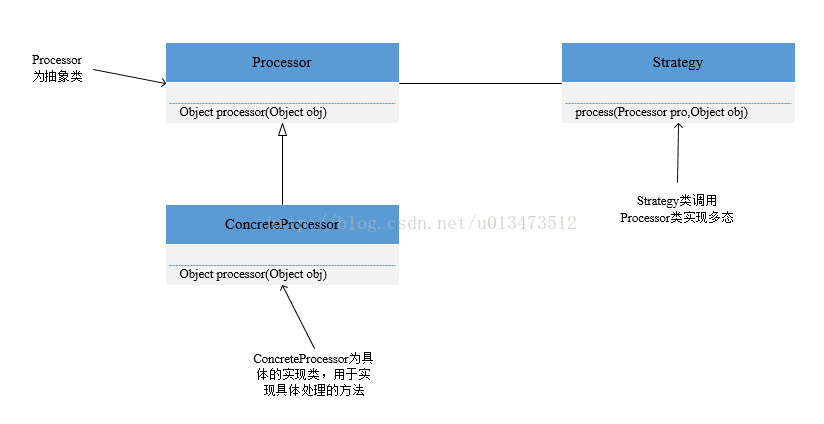
源代码:
public abstract class Processor {
public Object process(Object obj){
return obj;
}
}
/**
* ConcreteProcessor
* */
public class Upcase extends Processor{
public String process(Object obj){
return ((String)obj).toUpperCase();
}
}
/**
* ConcreteProcessor
* */
public class Downcase extends Processor{
public String process(Object obj){
return ((String)obj).toLowerCase();
}
}
public class Strategy {
public void process(Processor p,Object obj) {
System.out.println(p.process(obj));
}
}
4、观察者模式
定义:
观察者模式定义了对象之间的一对多依赖,这样一来,当一个对象改变状态时,它的所有依赖者都会收到通知并自动更新。
类图:
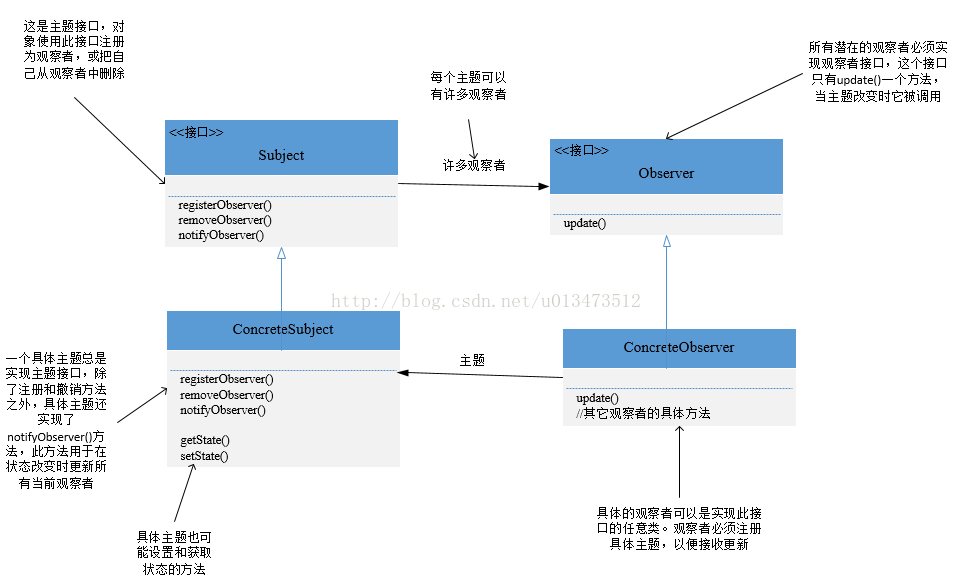
源代码:
public interface Subject {
public void registerObserver(Observer o);
public void removeObserver(Observer o);
public void notifyObserver(); //当主题状态改变时,该方法会被调用,以通知所有的观察者
}
public class ConcreteSubject implements Subject {
private List<Observer> observers;
private String parameter;
public ConcreteSubject() {
this.observers = new ArrayList<>();
}
@Override
public void registerObserver(Observer o) {
this.observers.add(o);
}
@Override
public void removeObserver(Observer o) {
this.observers.remove(o);
}
@Override
public void notifyObserver() {
int i,n;
n = this.observers.size();
for(i = 0;i < n;i++){
Observer observer = this.observers.get(i);
observer.update(parameter);
}
}
public void setParameter(String parameter) {
this.parameter = parameter;
notifyObserver();
}
}
public interface Observer {
public void update(String parameter);
}
public class ConcreteObserver implements Observer {
private String parameter;
private Subject subject;
public ConcreteObserver(Subject subject) {
this.subject = subject;
this.subject.registerObserver(this);
}
@Override
public void update(String parameter) {
this.parameter = parameter;
display();
}
public void display() {
System.out.println("Current state : " + parameter);
}
}
5、装饰者模式
定义:
动态地将责任附加到对象上。若要扩展功能,装饰着提供了比继承更有弹性的替代方法。
类图:
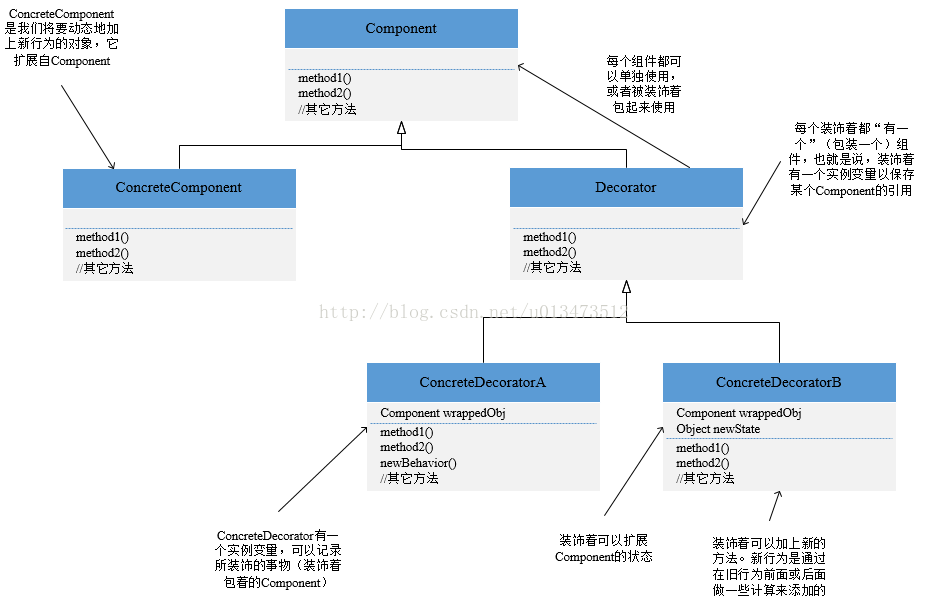
源代码:
public abstract class Component {
protected String description = "Component";
public String getDescription() {
return description;
}
}
public class ConcreteComponent_A extends Component{
public ConcreteComponent_A() {
description = "ConcreteComponent_A";
}
public String getDescription() {
return description;
}
}
public class ConcreteComponent_B extends Component{
public ConcreteComponent_B() {
description = "ConcreteComponent_B";
}
public String getDescription() {
return description;
}
}
public abstract class Decorator extends Component{
public abstract String getDescription();
}
public class ConcreteDecorator_A extends Decorator{
Component component;
public ConcreteDecorator_A(Component component) {
this.component = component;
}
@Override
public String getDescription() {
return component.getDescription() + ", ConcreteDecorator_A";
}
}
public class ConcreteDecorator_B extends Decorator{
Component component;
public ConcreteDecorator_B(Component component) {
this.component = component;
}
@Override
public String getDescription() {
return component.getDescription() + ", ConcreteDecorator_B";
}
}
6、命令模式
定义:
将“请求”封装成对象,以便使用不同的请求、队列或日志来参数化其对象。命令模式也支持可撤销的操作。
类图:
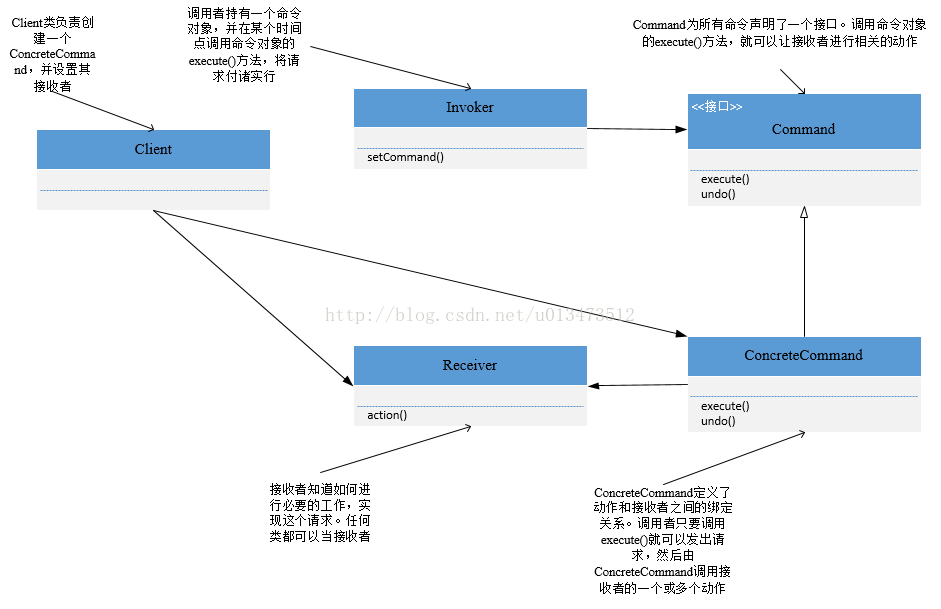
源代码:
public interface Command {
public void execute();
}
/**
* 起到ConcreteCommand的作用
* */
public class LightOnCommand implements Command {
private Light light;
public LightOnCommand(Light light) {
this.light = light;
}
@Override
public void execute() {
light.on();
}
}
/**
* 起到ConcreteCommand的作用
* */
public class LightOffCommand implements Command {
private Light light;
public LightOffCommand(Light light) {
this.light = light;
}
@Override
public void execute() {
light.off();
}
}
/**
* 起到Receiver的作用
* */
public class Light {
private boolean light;
private String name;
public Light(String name) {
this.name = name;
}
public void on() {
light = true;
}
public void off() {
light = false;
}
@Override
public String toString() {
return "Light [name = " + name + ", is = " + light + "]";
}
}
public class Invoker {
private Command[] onCommands;
private Command[] offCommands;
public Invoker() {
onCommands = new Command[2];
offCommands = new Command[2];
for(int i = 0;i < 2;i++){
onCommands[i] = null;
offCommands[i] = null;
}
}
public void setCommand(int index,Command on,Command off) {
onCommands[index] = on;
offCommands[index] = off;
}
public void onButtonWasPushed(int index) {
onCommands[index].execute();
}
public void offButtonWasPushed(int index) {
offCommands[index].execute();
}
@Override
public String toString() {
String str = "";
for(int i = 0;i < onCommands.length;i++){
str += "index "+i+" : "+onCommands[i].getClass().getName()+" "+offCommands[i].getClass().getName()+"\n";
}
return str;
}
}
7、适配器模式
定义:
将一个类的接口,转换成客户期望的另一个接口。适配器让原本接口不兼容的类可以合作无间。
类图:
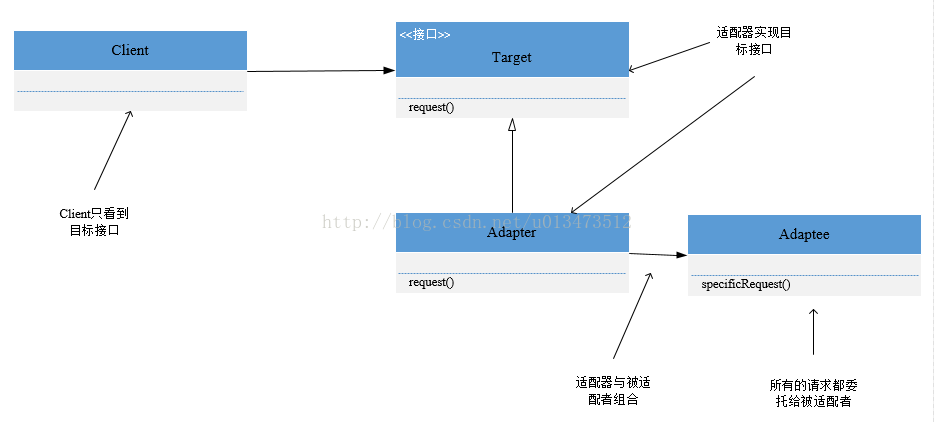
源代码:
public interface Target {
public void request();
}
public class RealTarget implements Target {
@Override
public void request() {
System.out.println("this is real do.");
}
}
public class Adaptee {
public void doing() {
System.out.println("this is Adaptee_do.");
}
}
public class Adapter implements Target {
private Adaptee adaptee;
public Adapter(Adaptee adaptee) {
this.adaptee = adaptee;
}
@Override
public void request() {
adaptee.doing();
}
}
8、外观模式
定义:
提供了一个统一的接口,用来访问子系统中的一群接口。外观定义了一个高层接口,让子系统更容易使用。
类图:
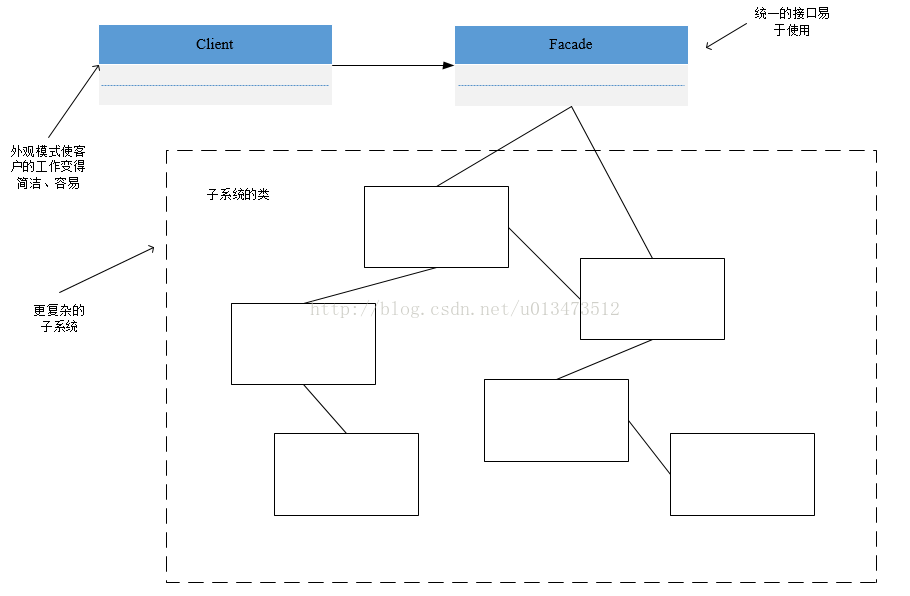
9、模板方法模式
定义:
在一个方法中定义一个算法的骨架,而将一些步骤延迟到子类中。模板方法使得子类可以在不改变算法结构的情况下,重新定义算法中的某些步骤。
类图:
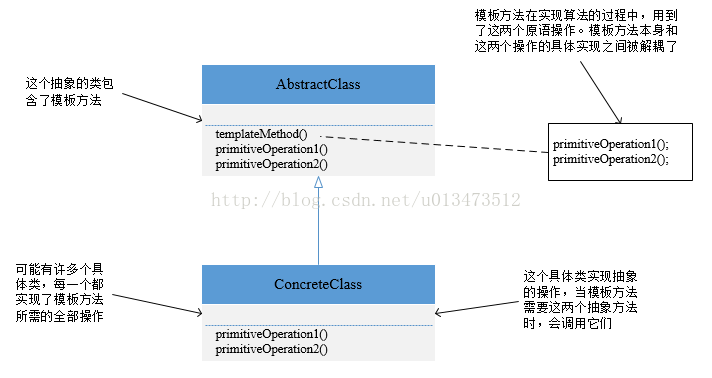
源代码:
public abstract class AbstractClass {
final void templateMethod(){
primitiveOperation1();
primitiveOperation2();
}
abstract void primitiveOperation1();
abstract void primitiveOperation2();
}
public class ConcreteClass_A extends AbstractClass{
@Override
void primitiveOperation1() {
System.out.println("this is primitiveOperation1 in ConcreteClass_A");
}
@Override
void primitiveOperation2() {
System.out.println("this is primitiveOperation2 in ConcreteClass_A");
}
}
public class ConcreteClass_B extends AbstractClass{
@Override
void primitiveOperation1() {
System.out.println("this is primitiveOperation1 in ConcreteClass_B");
}
@Override
void primitiveOperation2() {
System.out.println("this is primitiveOperation2 in ConcreteClass_B");
}
}
10、迭代器模式
定义:
提供一种方法顺序访问一个聚合对象中的各个元素,而又不暴露其内部的表示。
作用:
迭代器模式让我们能游走于聚合内的每一个元素,而又不暴露其内部的表示。将游走的任务放在迭代器上,而不是聚合上,这样简化了聚合的接口和实现,也让责任各得其所。
类图:
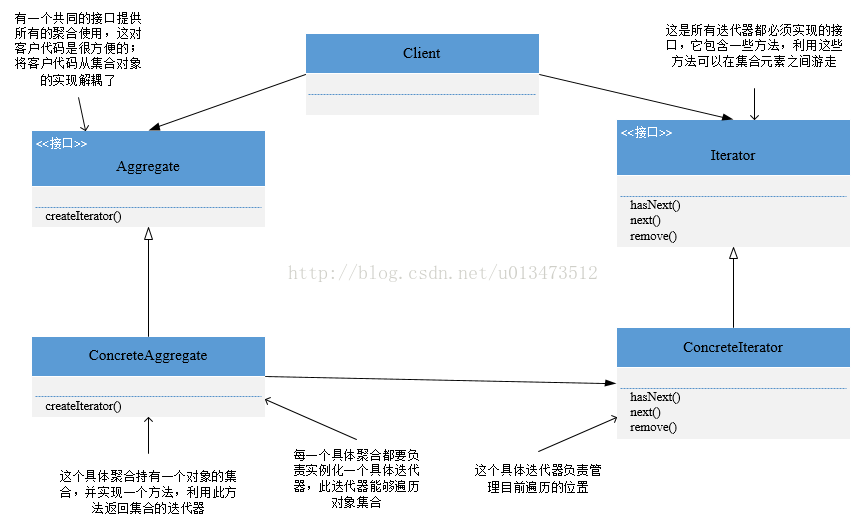
源代码:
public interface Aggregate {
public Iterator createIterator(); //我们使用java.util.Iterator作为Iterator类
}
public class ConcreteAggregate implements Aggregate {
List list;
public ConcreteAggregate() {
this.list = new ArrayList();
init();
}
public void init() {
addItem("first");
addItem("second");
addItem("third");
}
public void addItem(String parameter) {
list.add(parameter);
}
@Override
public Iterator createIterator() {
return list.iterator();
}
}
11、组合模式
定义:
允许将对象组合成树形结构来表现“整体/部分”层次结构。
作用:
组合能让客户以一致的方式处理个别对象以及对象组合。组合模式让我们能用树形方式创建对象的结构,树中包含了组合以及个别的对象。使用组合结构,我们能把相同的操作应用在组合和个别对象上。换句话说,在大多数情况下,我们可以忽略对象组合和个别对象之间的差异。
类图:
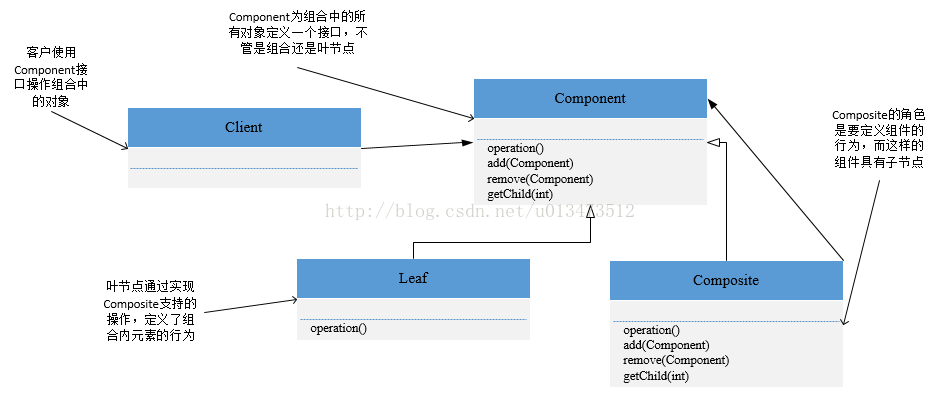
源代码:
public abstract class Component {
public void add(Component component) {
throw new UnsupportedOperationException();
}
public void remove(Component component) {
throw new UnsupportedOperationException();
}
public Component getChild(int i) {
throw new UnsupportedOperationException();
}
public void operation() {
}
}
public class Leaf extends Component {
String description;
public Leaf(String description) {
this.description = description;
}
public void operation() {
System.out.println("this is Leaf " + description + " operation.");
}
}
public class Composite extends Component{
List<Component> components;
String description;
public Composite(String description) {
components = new ArrayList<>();
this.description = description;
}
public void add(Component component) {
components.add(component);
}
public void remove(Component component) {
components.remove(component);
}
public Component getChild(int i) {
return components.get(i);
}
public void operation() {
System.out.println("this is Composite " + description + " operation.");
}
}
12、状态模式
定义:
允许对象在内部状态改变时改变它的行为,对象看起来好像修改了它的类。
类图:
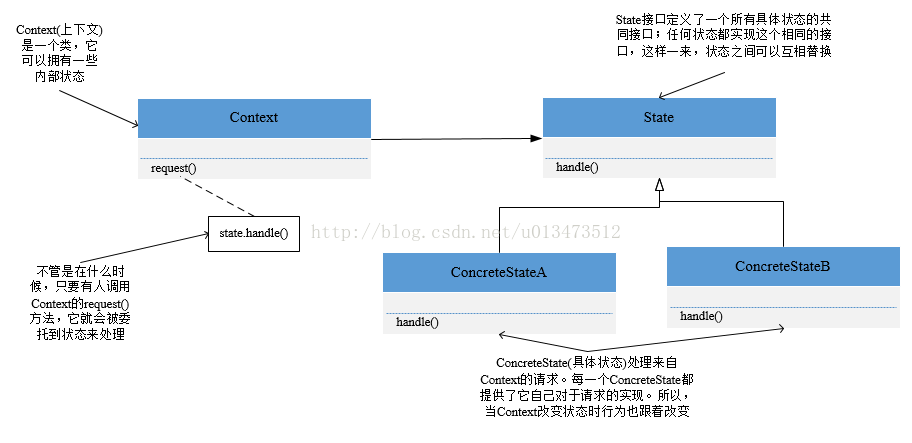
源代码:
public interface State {
public void handle();
}
public class OpenState implements State {
@Override
public void handle() {
System.out.println("It's opening!");
}
}
public class CloseState implements State {
@Override
public void handle() {
System.out.println("It's closed!");
}
}
public class Context {
private State openState;
private State closeState;
public Context() {
openState = new OpenState();
closeState = new CloseState();
}
public void open() {
openState.handle();
}
public void close() {
closeState.handle();
}
}
13、代理模式
定义:
为另一个对象提供一个替身或占位符以控制对这个对象的访问。
作用:
使用代理模式创建代表(representative)对象,让代表对象控制某对象的访问,被代理的对象可以是远程的对象、创建开销大的对象或需要安全控制的对象。
类图:
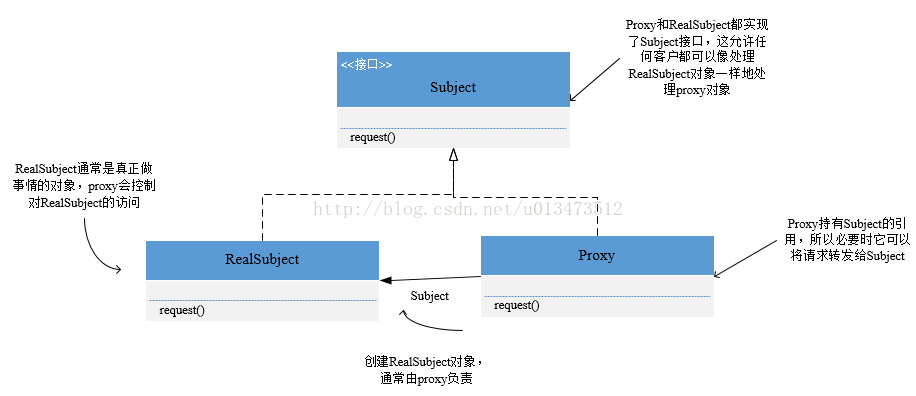
源代码:
public interface Subject {
public void request();
}
class RealSubject implements Subject {
@Override
public void request() {
System.out.println("this is request in RealSubject.");
}
}
public class Proxy implements Subject {
Subject subject;
public Proxy() {
subject = new RealSubject();
}
@Override
public void request() {
subject.request();
}
}
具体源码及类图详见http://download.csdn.net/detail/u013473512/9803167
欢迎关注“程序杂货铺”公众号,里面有精彩内容,欢迎大家收看^_^