using System;
using System.Drawing;
using System.Drawing.Drawing2D;
using System.Windows.Forms;
namespace Net6_GeneralUiWinFrm
{
public class CircularProgressBar : Control
{
private int progress = 0;
private int borderWidth = 20; // 增加的边框宽度
public int Progress
{
get { return progress; }
set
{
progress = Math.Max(0, Math.Min(100, value)); // 确保进度值在0到100之间
Invalidate(); // Causes the control to be redrawn
}
}
protected override void OnPaint(PaintEventArgs e)
{
base.OnPaint(e);
e.Graphics.SmoothingMode = SmoothingMode.AntiAlias;
// Draw background circle
using (Pen pen = new Pen(Color.LightGray, borderWidth))
{
pen.DashStyle = DashStyle.Dot; // 设置点状线条
e.Graphics.DrawEllipse(pen, borderWidth / 2, borderWidth / 2, this.Width - borderWidth, this.Height - borderWidth);
}
// Draw progress arc
using (Pen pen = new Pen(Color.LightGreen, borderWidth)) //lightgreen
{
pen.DashStyle = DashStyle.Solid; // 进度使用实线
// Calculate sweep angle
float sweepAngle = (360f * progress) / 100f;
e.Graphics.DrawArc(pen, borderWidth / 2, borderWidth / 2, this.Width - borderWidth, this.Height - borderWidth, -90, sweepAngle);
}
// Draw progress text
string progressText = $"{progress}%";
using (Font font = new Font("Arial", 12))
using (Brush brush = new SolidBrush(Color.Black))
{
SizeF textSize = e.Graphics.MeasureString(progressText, font);
// Calculate text position
PointF textPoint = new PointF((this.Width - textSize.Width) / 2, (this.Height - textSize.Height) / 2);
e.Graphics.DrawString(progressText, font, brush, textPoint);
}
}
}
}
C# Winform .net6自绘的圆形进度条
最新推荐文章于 2024-08-09 07:19:41 发布
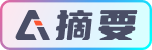