1.1 异常概述与异常体系结构
- 在Java语言中,将程序执行中发生的不正常情况称为“异常”
- 分类:编译时异常和运行时异常
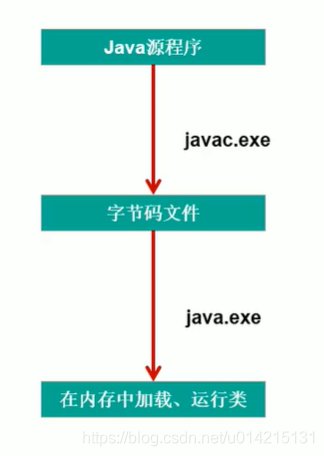
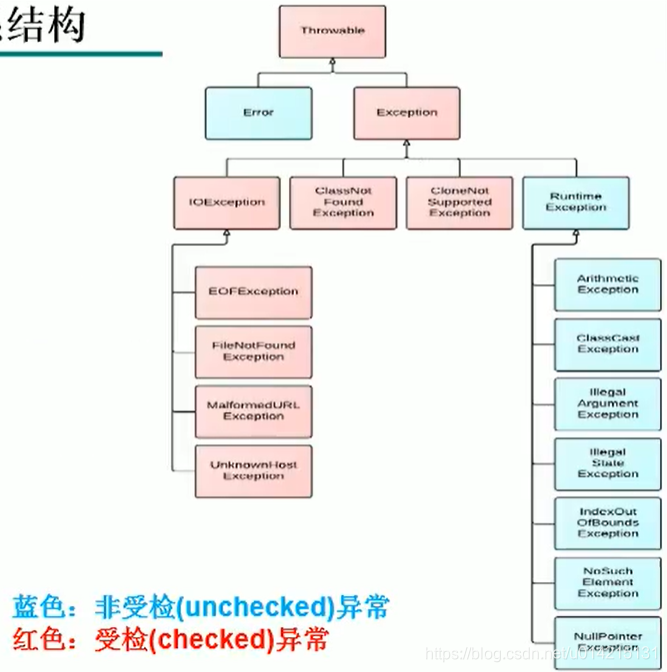
1.2 常见异常
package demo;
import java.util.Date;
import java.util.Scanner;
import org.junit.Test;
public class ExceptionTest {
@Test
public void test1() {
int[] arr = null;
System.out.println(arr[2]);
String str = "abc";
System.out.println(str.charAt(5));
Object o = new Date();
String str1 = (String) o;
String str2 = "abc";
Integer.parseInt(str);
int i = 10 / 0;
Scanner scanner = new Scanner(System.in);
int nextInt = scanner.nextInt();
int[] arr1 = new int[10];
System.out.println(arr1[20]);
}
}
1.3 异常处理机制一:try-catch-finally
- Java提供的是异常处理的抓抛模型
- Java的执行过程中如出现异常,会生成一个异常类对象,该异常对象将被提交给Java运行时系统,这个过程称为抛出(thorw)异常
package demo;
import org.junit.Test;
public class ExceptionTest1 {
@Test
public void test1() {
String str = "123";
str = "abc";
try {
int num = Integer.parseInt(str);
System.out.println("hello-1");
} catch (NumberFormatException e) {
System.out.println(e.getMessage());
e.printStackTrace();
System.out.println("出现异常了");
}
System.out.println("hello-2");
}
}
package demo;
import org.junit.Test;
public class FinallyTest {
@Test
public void test1() {
int a = 10;
int b = 0;
try {
System.out.println(a / b);
} catch (Exception e) {
e.printStackTrace();
int[] arr = new int[10];
System.out.println(arr[10]);
} finally {
System.out.println("hello");
}
}
}
1.4 异常处理机制二:throws
package demo;
import java.io.File;
import java.io.FileInputStream;
import java.io.FileNotFoundException;
import java.io.IOException;
public class ExceptionTest2 {
public void method1() throws IOException {
File file = new File("hello.txt");
FileInputStream fis = new FileInputStream(file);
int read = fis.read();
while (read != -1) {
System.out.println(read);
read = fis.read();
}
}
}
1.5 手动抛出异常:throw
package demo;
public class StudentTest {
public static void main(String[] args) {
try {
Student student = new Student();
student.regist(-1001);
System.out.println(student);
} catch (Exception e) {
System.out.println(e.getMessage());
}
}
}
class Student {
private int id;
public void regist(int id) throws Exception {
if (id > 0) {
this.id = id;
} else {
throw new Exception("输入的数据非法");
}
}
@Override
public String toString() {
return "Student [id=" + id + "]";
}
}
1.6 用户自定义异常类
package demo;
public class MyException extends RuntimeException{
static final long serialVersionUID = -70348975766939L;
public MyException() {
}
public MyException(String msg) {
super(msg);
}
}