Time Limit: 6000/2000 MS (Java/Others) Memory Limit: 32768/32768 K (Java/Others)
Total Submission(s): 3477 Accepted Submission(s): 1504
Total Submission(s): 3477 Accepted Submission(s): 1504
Problem Description
Somewhere deep in the Czech Technical University buildings, there are laboratories for examining mechanical and electrical properties of various materials. In one of yesterday’s presentations, you have seen how was one of the laboratories changed into a new multimedia lab. But there are still others, serving to their original purposes.
In this task, you are to write software for a robot that handles samples in such a laboratory. Imagine there are material samples lined up on a running belt. The samples have different heights, which may cause troubles to the next processing unit. To eliminate such troubles, we need to sort the samples by their height into the ascending order.
Reordering is done by a mechanical robot arm, which is able to pick up any number of consecutive samples and turn them round, such that their mutual order is reversed. In other words, one robot operation can reverse the order of samples on positions between A and B.
A possible way to sort the samples is to find the position of the smallest one (P1) and reverse the order between positions 1 and P1, which causes the smallest sample to become first. Then we find the second one on position P and reverse the order between 2 and P2. Then the third sample is located etc.
The picture shows a simple example of 6 samples. The smallest one is on the 4th position, therefore, the robot arm reverses the first 4 samples. The second smallest sample is the last one, so the next robot operation will reverse the order of five samples on positions 2–6. The third step will be to reverse the samples 3–4, etc.
Your task is to find the correct sequence of reversal operations that will sort the samples using the above algorithm. If there are more samples with the same height, their mutual order must be preserved: the one that was given first in the initial order must be placed before the others in the final order too.
In this task, you are to write software for a robot that handles samples in such a laboratory. Imagine there are material samples lined up on a running belt. The samples have different heights, which may cause troubles to the next processing unit. To eliminate such troubles, we need to sort the samples by their height into the ascending order.
Reordering is done by a mechanical robot arm, which is able to pick up any number of consecutive samples and turn them round, such that their mutual order is reversed. In other words, one robot operation can reverse the order of samples on positions between A and B.
A possible way to sort the samples is to find the position of the smallest one (P1) and reverse the order between positions 1 and P1, which causes the smallest sample to become first. Then we find the second one on position P and reverse the order between 2 and P2. Then the third sample is located etc.
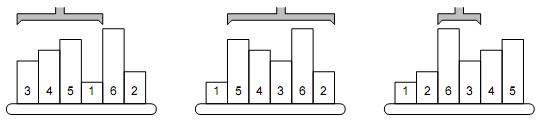
The picture shows a simple example of 6 samples. The smallest one is on the 4th position, therefore, the robot arm reverses the first 4 samples. The second smallest sample is the last one, so the next robot operation will reverse the order of five samples on positions 2–6. The third step will be to reverse the samples 3–4, etc.
Your task is to find the correct sequence of reversal operations that will sort the samples using the above algorithm. If there are more samples with the same height, their mutual order must be preserved: the one that was given first in the initial order must be placed before the others in the final order too.
Input
The input consists of several scenarios. Each scenario is described by two lines. The first line contains one integer number N , the number of samples, 1 ≤ N ≤ 100 000. The second line lists exactly N space-separated positive integers, they specify the heights of individual samples and their initial order.
The last scenario is followed by a line containing zero.
The last scenario is followed by a line containing zero.
Output
For each scenario, output one line with exactly N integers P1 , P1 , . . . PN ,separated by a space.
Each Pi must be an integer (1 ≤ Pi ≤ N ) giving the position of the i-th sample just before the i-th reversal operation.
Note that if a sample is already on its correct position Pi , you should output the number Pi anyway, indicating that the “interval between Pi and Pi ” (a single sample) should be reversed.
Each Pi must be an integer (1 ≤ Pi ≤ N ) giving the position of the i-th sample just before the i-th reversal operation.
Note that if a sample is already on its correct position Pi , you should output the number Pi anyway, indicating that the “interval between Pi and Pi ” (a single sample) should be reversed.
Sample Input
6 3 4 5 1 6 2 4 3 3 2 1 0
Sample Output
4 6 4 5 6 6 4 2 4 4
Source
Recommend
这题网上给出的大多数解答都有问题啊,直接访问下边的节点上边的标记可能并没有上传过来,但是居然能A掉。。真神奇
#include <cstdio>
#include <cstring>
#include <algorithm>
#include <iostream>
#define MAXN 100010
#define Key_value ch[ch[root][1]][0]
using namespace std;
int pre[MAXN],ch[MAXN][2],root,tot,n,q;
int size[MAXN],add[MAXN];
long long sum[MAXN];
struct thing
{
int r,v;
} a[MAXN];
bool camp(thing a,thing b)
{
if(a.v == b.v) return a.r < b.r;
return a.v < b.v;
}
long long min(long long a,long long b)
{
if(a > b) return b;
return a;
}
void NewNode(int &r,int father,int num)
{
r = num;
pre[r] = father;
size[r] = 1;
add[r] = 0;
ch[r][0] = ch[r][1] = 0;
}
void Update_Swap(int r,int ADD)
{
if(r == 0) return;
add[r] = (add[r] + ADD)%2;
swap(ch[r][0],ch[r][1]);
}
void push_up(int r)
{
size[r] = size[ch[r][0]] + size[ch[r][1]] + 1;
}
void push_down(int r)
{
if(add[r])
{
Update_Swap(ch[r][0],add[r]);
Update_Swap(ch[r][1],add[r]);
add[r] = 0;
}
}
void Build(int &x,int l,int r,int father)
{
if(l > r) return;
int mid = (l+r) >> 1;
NewNode(x,father,mid);
Build(ch[x][0],l,mid-1,x);
Build(ch[x][1],mid+1,r,x);
push_up(x);
}
void Init()
{
root = tot = 0;
for(int i = 1;i <= n;i++)
{
scanf("%d",&a[i].v);
a[i].r = i;
}
sort(a+1,a+1+n,camp);
Build(root,1,n,0);
}
void Rotate(int x,int kind)
{
int y = pre[x];
push_down(y);
push_down(x);
ch[y][!kind] = ch[x][kind];
pre[ch[x][kind]] = y;
if(pre[y]) ch[pre[y]][ch[pre[y]][1] == y] = x;
pre[x] = pre[y];
ch[x][kind] = y;
pre[y] = x;
push_up(y);
}
void Splay(int r,int goal)
{
if(r == goal) return;
push_down(r);
while(pre[r] != goal)
{
if(pre[pre[r]] == goal) Rotate(r,ch[pre[r]][0] == r);
else
{
int y = pre[r];
int kind = ch[pre[y]][0] == y;
if(ch[y][kind] == r)
{
Rotate(r,!kind);
Rotate(r,kind);
}
else
{
Rotate(y,kind);
Rotate(r,kind);
}
}
}
push_up(r);
if(goal == 0) root = r;
}
int Find(int r)
{
push_down(r);
while(ch[r][0])
{
r = ch[r][0];
push_down(r);
}
return r;
}
void Detele(int &root)
{
if(ch[root][1]*ch[root][0] == 0)
{
root = ch[root][ch[root][0] == 0];
pre[root] = 0;
return;
}
Splay(Find(ch[root][1]),root);
ch[ch[root][1]][0] = ch[root][0];
pre[ch[root][0]] = ch[root][1];
root = ch[root][1];
pre[root] = 0;
push_up(root);
return;
}
int main()
{
while(scanf("%d",&n) && n)
{
Init();
for(int i = 1;i <= n;i++)
{
Splay(a[i].r,0);
Update_Swap(ch[root][0],1);
if(i < n) printf("%d ",size[ch[root][0]]+i);
else printf("%d\n",n);
Detele(root);
}
}
}