Time Limit: 3000MS | Memory Limit: Unknown | 64bit IO Format: %lld & %llu |
Description
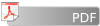
This puzzle consists of two wheels. Both wheels can rotate both clock and counter-clockwise. They contain 21 coloured pieces, 10 of which are rounded triangles and 11 of which are separators. Figure 1 shows the final position of each one of the pieces. Note that to perform a one step rotation you must turn the wheel until you have advanced a triangle and a separator.
Figure 1. Final puzzle configuration
Your job is to write a program that reads the puzzle configuration and prints the minimum sequence of movements required to reach the final position. We will use the following integer values to encode each type of piece:
0 | grey separator |
1 | yellow triangle |
2 | yellow separator |
3 | cyan triangle |
4 | cyan separator |
5 | violet triangle |
6 | violet separator |
7 | green triangle |
8 | green separator |
9 | red triangle |
10 | red separator |
A puzzle configuration will be described using 24 integers, the first 12 to describe the left wheel configuration and the last 12 for the right wheel. The first integer represents the bottom right separator of the left wheel and the next eleven integers describe the left wheel clockwise. The thirteenth integer represents the bottom left separator of right wheel and the next eleven integers describe the right wheel counter-clockwise.
The final position is therefore encoded like:
If for instance we rotate the left wheel clockwise one position from the final configuration (as shown in Figure 2) the puzzle configuration would be encoded like:
Figure 2. The puzzle after rotating the left wheel on step clockwise from the final configuration.
Input
Input for your program consists of several puzzles. The first line of the input will contain an integer n specifying the number of puzzles. There will then be n lines each containing 24 integers separated with one white space, describing the initial puzzle configuration as explained above.
Output
For each configuration your program should output one line with just one number representing the solution. Each movement is encoded using one digit from 1 to 4 in the following way:
1 | Left Wheel Clockwise rotation |
2 | Right Wheel Clockwise rotation |
3 | Left Wheel Counter-Clockwise rotation |
4 | Right Wheel Counter-Clockwise rotation |
No space should be printed between each digit. Since multiple solutions could be found, you should print the solution that is encoded as the smallest number. The solution will never require more than 16 movements.
If no solution is found you should print ``NO SOLUTION WAS FOUND IN 16 STEPS". If you are given the final position you should print ``PUZZLE ALREADY SOLVED".
Sample Input
3 0 3 4 3 0 5 6 5 0 1 2 1 0 7 8 7 0 9 10 9 0 1 2 1 0 3 4 5 0 3 6 5 0 1 2 1 0 7 8 7 0 9 10 9 0 1 2 1 0 9 4 3 0 5 6 5 0 1 2 1 0 7 8 7 0 9 10 3 0 1 2 1
Sample Output
PUZZLE ALREADY SOLVED 1434332334332323 NO SOLUTION WAS FOUND IN 16 STEPS
题意:如上图,给出一个初始状态,问在16步之内能否转到终态,输出路径。
思路:16太大,像这样知道初态和终态的可以使用双向bfs求解。
代码:
#include <iostream>
#include <functional>
#include <cstdio>
#include <cstring>
#include <algorithm>
#include <cmath>
#include <string>
#include <map>
#include <stack>
#include <vector>
#include <set>
#include <queue>
#pragma comment (linker,"/STACK:102400000,102400000")
#define pi acos(-1.0)
#define eps 1e-6
#define lson rt<<1,l,mid
#define rson rt<<1|1,mid+1,r
#define FRE(i,a,b) for(i = a; i <= b; i++)
#define FREE(i,a,b) for(i = a; i >= b; i--)
#define FRL(i,a,b) for(i = a; i < b; i++)
#define FRLL(i,a,b) for(i = a; i > b; i--)
#define mem(t, v) memset ((t) , v, sizeof(t))
#define sf(n) scanf("%d", &n)
#define sff(a,b) scanf("%d %d", &a, &b)
#define sfff(a,b,c) scanf("%d %d %d", &a, &b, &c)
#define pf printf
#define DBG pf("Hi\n")
typedef long long ll;
using namespace std;
#define INF 0x3f3f3f3f
#define mod 1000000009
const int maxn = 1005;
const int MAXN = 2005;
const int MAXM = 200010;
const int N = 1005;
struct Node
{
int step;
string g;
string path;
};
int flag1,flag2;
string p1,p2;
map<string,string>Hash1;
map<string,int>Hash2;
string goal="034305650121078709*90121";
void change(string &s,int w)
{
string ss=s;
if (w==1)
{
for (int i=0;i<12;i++)
s[(i+2+12)%12]=ss[i];
s[23]=s[11]; s[22]=s[10] ; s[21]=s[9];
}
else if (w==2)
{
for (int i=0;i<12;i++)
s[(i-2+12)%12+12]=ss[i+12];
s[11]=s[23]; s[10]=s[22]; s[9]=s[21];
}
else if (w==3)
{
for (int i=0;i<12;i++)
s[(i-2+12)%12]=ss[i];
s[23]=s[11]; s[22]=s[10] ; s[21]=s[9];
}
else
{
for (int i=0;i<12;i++)
s[(i+2+12)%12+12]=ss[i+12];
s[11]=s[23] ; s[10]=s[22] ; s[9]=s[21];
}
}
void backbfs()
{
// printf("***\n");
Hash2.clear();
Node st,now;
st.path="";
st.g=goal;
st.step=0;
Hash2[goal]=1;
queue<Node>Q;
Q.push(st);
while (!Q.empty())
{
st=Q.front();
Q.pop();
if (st.step>8) return ;
if (Hash1.find(st.g)!=Hash1.end())
{
p1=Hash1[st.g];
p2=st.path;
flag2=1;
return ;
}
for (int i=1;i<=4;i++)
{
now.g=st.g;
now.step=st.step+1;
change(now.g,i);
if (Hash2[now.g]==0)
{
Hash2[now.g]=1;
now.path=st.path+(char)(i+'0');
Q.push(now);
}
}
}
}
void bfs(string s)
{
Node st,now;
Hash1.clear();
st.g=s;
st.path="";
st.step=0;
Hash1[s]="#";
queue<Node>Q;
Q.push(st);
while (!Q.empty())
{
st=Q.front();
Q.pop();
if (st.g==goal)
{
p1=st.path;
flag1=1;
return ;
}
if (st.step>=8)
{
backbfs();
return ;
}
for (int i=1;i<=4;i++)
{
now.g=st.g;
now.step=st.step+1;
change(now.g,i);
if (Hash1.find(now.g)==Hash1.end())
{
now.path=st.path+(char)(i+'0');
Hash1[now.g]=now.path;
// cout<<now.path<<endl;
Q.push(now);
}
}
}
}
int main()
{
#ifndef ONLINE_JUDGE
freopen("C:/Users/lyf/Desktop/IN.txt","r",stdin);
#endif
int i,j,x,t;
scanf("%d",&t);
while (t--)
{
string s="";
for (i=0;i<24;i++)
{
scanf("%d",&x);
if (x<10) s=s+(char)(x+'0');
else s=s+"*";
}
if (s==goal)
{
printf("PUZZLE ALREADY SOLVED\n");
continue;
}
flag1=flag2=0;
bfs(s);
if (flag1){
cout<<p1<<endl;
}
else if (flag2)
{
cout<<p1;
int len=p2.length();
for (i=len-1;i>=0;i--)
{
if (p2[i]=='1') printf("3");
else if (p2[i]=='2') printf("4");
else if (p2[i]=='3') printf("1");
else printf("2");
}
printf("\n");
}
else
printf("NO SOLUTION WAS FOUND IN 16 STEPS\n");
}
return 0;
}