在某网站下载某些图片时,经常会出现图片格式是webp的情况,所以正好初学python的时候用当前知识写了一个简单的批量转换图片的程序。
主程序部分
import os
from PIL import Image
from time import time
from threading import Thread
from tqdm import tqdm
import re
def split_str(string): # 输入字符串切分
pattern = r'[,,]+'
return re.split(pattern, string)
# 转换函数
def image_converter(source_dir, old_suffix, new_suffix, delete_original):
start_time = time()
if not old_suffix.startswith('.'):
old_suffix = '.' + old_suffix # 旧的扩展名
if not new_suffix.startswith('.'):
new_suffix = '.' + new_suffix # 新的扩展名
new_dir = os.path.join(os.path.dirname(source_dir), 'finished-' + os.path.basename(source_dir)) # 在同级创建完成后的文件夹
converted_count = 0
if not os.path.exists(new_dir): # 目录不存在就创建
os.makedirs(new_dir)
# 获取待转换图像的路径列表
file_paths = []
for root, dirs, files in os.walk(source_dir):
for file in files:
file_path = os.path.join(root, file)
if os.path.splitext(file_path)[1] == old_suffix:
file_paths.append(file_path)
def convert_image(file_path):
img = Image.open(file_path)
new_file_path = os.path.join(new_dir, os.path.splitext(
os.path.basename(file_path))[0] + new_suffix)
img.save(new_file_path)
if delete_original:
os.remove(file_path)
nonlocal converted_count
converted_count += 1
# 创建线程列表
threads = []
for file_path in file_paths:
t = Thread(target=convert_image, args=(file_path,))
threads.append(t)
# 启动线程
for t in threads:
t.start()
# 显示进度条
for t in tqdm(threads):
t.join()
end_time = time()
print(
f'成功转换了{converted_count}张图片.\n转换后的目录为: {new_dir}\n程序运行时间为: {end_time - start_time:.2f}秒')
def run():
source_dir, old_suffix, new_suffix = split_str(input(
'请输入原图片目录,旧扩展名和新扩展名(用逗号分隔):\n'))
delete_original = input('是否要删除原图?请输入 y 或 n: ').lower() == 'y'
if source_dir.startswith('"'):
source_dir = source_dir.strip('"')
image_converter(source_dir, old_suffix, new_suffix, delete_original)
if __name__ == '__main__':
run()
实验输入结果
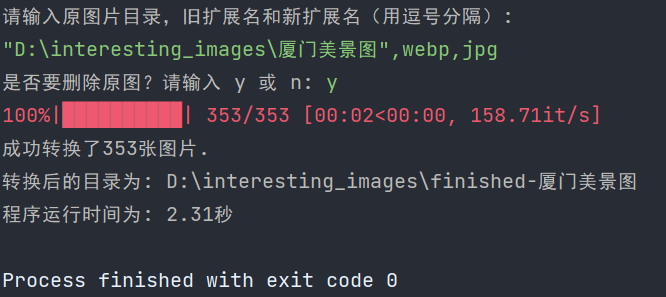