一 JSP是什么?
jsp 页面允许在标准的HTML 页面中包含Java代码
目标:是支持表现和业务逻辑的分离.
表现:由前端人员实现.
业务逻辑: 有后台程序员处理
二JSP页面的运行原理
JSP-页面---->翻译成.Java文件--->编译成.class文件;
JSP在翻译成.Java文件时 会将Java小脚本中的代码直接编译到 service 方法中;
HTML标签 是由 out对象 写回到浏览器中;
需求:使用JSP完成登录
只使用JSP
登录成功 去往 成功页面 并显示欢迎 xxx
登录失败 显示失败信息
登录的JSP<%@page import="org.apache.jasper.tagplugins.jstl.core.If"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<%--
<%
//取出错误信息
String msg =(String)request.getAttribute("msg");
if(msg!=null){
out.print(msg);
}
%>
--%>
<%--利用EL表达式 回显数据 --%>
${msg }
<form action="/sh-web-newjsp/dologin.jsp" method="post">
用户名:<input type="text" name="username"/><br/>
密码:<input type="text" name="password"/><br/>
<input type="submit" value="提交"/>
</form>
</body>
</html>
处理登录逻辑的JSP
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<%
String username = (String)request.getParameter("username");
String password = (String)request.getParameter("password");
//处理逻辑
//请求分发
if(username.equals("afd")&&password.equals("123")){
//成功 请求重定向
session.setAttribute("username", username);
response.sendRedirect("/sh-web-newjsp/success.jsp");
}else{
//失败
request.setAttribute("msg", "账号和密码失败,请重新登录");
request.getRequestDispatcher("/login.jsp").forward(request, response);
}
%>
</body>
</html>
登录成功的JSP
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
欢迎:
<%
String usernsme =(String) session.getAttribute("username");
out.write(usernsme);
%>
进入本网站
</body>
</html>
三 JSP的五种元素
5.声明标签
3个指令标签:
1.page<%@ 引入头文件%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
pageEncoding 设置本页面的编码格式
contentType 相当于 response.setContentType
<%@ page session= "true" %>
session 开关默认开启状态的,在JSP编译成servlet时 系统会生成 session对象 ,当设置成false是 session就不会被创建;
<%@ page errorPage="/3.jsp" %>
当本页面错误时 跳转到另一个页面
<%@ page isErrorPage="true"%>
isErrorPage默认是关闭的 开启后可以使用exception对象获取错误信息
以下测试 isErrorPage
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page session= "false" %>
<%--session 开关 默认开启状态的
关上时 编译.Java文件系统不会为你创建 session
--%>
<%@ page errorPage="/3.jsp" %>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<%
//session.getAttribute("123");
int num = 10/0;
%>
</body>
</html>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%@ page isErrorPage="true"%>
<%-- isErrorPage默认是关闭的 开启后可以使用exception对象获取错误信息 --%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
服务器正在维护升级中....
<%=
exception.getMessage()
%>
</body>
</html>
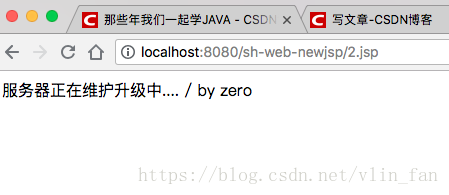
2. Include 包含
<%@ Include file = "包含哪个页面"%>
包含分为两种
静态包含
<%-- <%@ include file="/5.jsp" %>--%>
编译成.Java文件时 直接把两个个界面编译成一个.Java文件
输出了两套HTML标签;
动态包含
当读取到动态包含时 才会把动态包含的那个页面编译成.Java文件 此时编译成的是两个.Java文件
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<%--静态包含 --%>
<%-- <%@ include file="/5.jsp" %>--%>
<%--动态包含 --%>
<%--<jsp:include page="/5.jsp"></jsp:include> --%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
吾问无为谓无无无无无无无无无无
</body>
</html>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
---坎坎坷坷扩扩扩扩扩扩扩扩扩扩扩扩扩扩
</body>
</html>

3.taglib
可以引入标准标签库(c标签)
<%--
引入标准标签库
prefix 使用标签时起的别名
--%>
<%@taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %>
二.6个动作标签
<JSP:include> 上面的动态包含
<JSP:forward> 请求转发
<JSP:param> 请求中的参数
<jsp:useBean> 创建对象
<jsp:setProperty> 设置对象的
<jsp:getProperty>
6.jsp
<jsp:forward page="/7.jsp">
<jsp:param value="hero" name="username"/>
<jsp:param value="5201314" name="password"/>
</jsp:forward>
7.jsp
<body>
我是7 哈哈哈
<%=request.getParameter("username") %>
<%=request.getParameter("password") %>
</body>
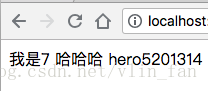
<jsp:useBean>
创建一个user对象
<%@page import="com.lanou3g.User"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<%
User user = new User();
user.setUsername("wanglong");
user.setPassword("123");
%>
<%=user.getUsername() %>
<%--使用useBean 创建对象
id 表示创建的这个对象的名字
class 表示使用哪个类来创建对象
--%>
<jsp:useBean id="user1" class="com.lanou3g.User"/>
<%--
name表示要赋值哪个对象
property 表示给对象的哪个属性赋值
value 表示赋的值
--%>
<jsp:setProperty property="username" name="user1" value="kuner"/>
<jsp:setProperty property="password" name="user1" value="10086"/>
<%--获取对象 --%>
<jsp:getProperty property="username" name="user1"/>
<jsp:getProperty property="password" name="user1"/>
</body>
</html>

三.9个内置对象
request
response
session
application ServletContext 全局域
exception
page 表示本类对象
config
out
pageContext 作用范围 指当前页面 域对象
域对象的作用范围
pageContext < request< session< ServletContext
1.pageContext 本类对象
<body>
<%
//pageContext 域对象
// 作用范围 :只能当前页面访问到
//可以获取(操作)所有的域对象
pageContext.setAttribute("sex", "女");
%>
<%=pageContext.getAttribute("sex") %>
</body>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<%
//利用PageContext 向各个域中保存值
//参数三表示向哪个域中存值
//pageContext.setAttribute("www", "pageContext",PageContext.PAGE_SCOPE);
//pageContext.setAttribute("www", "request", PageContext.REQUEST_SCOPE);
//想当于 request.setAttribute("www", request);
//pageContext.setAttribute("www", "session", PageContext.SESSION_SCOPE);
pageContext.setAttribute("www", "servletContext", PageContext.APPLICATION_SCOPE);
%>
<%--进行全域查找 从域范围由小到大查找 --%>
<%=pageContext.findAttribute("www") %>
<%=pageContext.findAttribute("www") %>
</body></html>
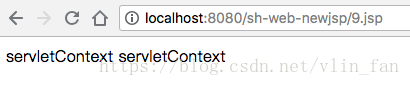
pageContext.findAttteribute 在查找值得时候从域范围由小到大查找 找到后就不在查询;
EL 表达式
el表达式 判断空值
<%@page import="java.util.ArrayList"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<!-- EL表达式 对空值的判断 -->
<%
String str1 = null;
request.setAttribute("str1", str1);
String str2 ="";
request.setAttribute("str2", str2);
String str3 ="wanglong";
request.setAttribute("str3", str3);
ArrayList<String> list1 = new ArrayList<String>();
request.setAttribute("list1", list1);
ArrayList<String> list2 = new ArrayList<String>();
list2.add("aaa");
list2.add("bbb");
request.setAttribute("list2", list2);
//根据从域中取出来的值,来让单选框默认选择哪个
String name = "nv";
request.setAttribute("name", name);
%>
<!--
测试el表达式对空值的显示结果
不管null还是没有内容 都不会报错
真正有值才会显示(容错性高)
-->
${str1 }
${str2 }
${str3 }
${list1 }
${list2 }
<!-- 判断空值的方法 关键词 empty -->
${empty str1 }
${empty str2 }
${empty str3 }
${empty list1 }
${empty list2 }
<!-- 支持三目表达式 -->
${empty str3?"我是前面的":"我是后面的" }<br>
男<input type="radio" name = "sex" value="nan" ${name=="nan"?"checked='checked'":""} /><br/>
女<input type="radio" name = "sex" value="nv" ${name=="nv"?"checked='checked'":""}/>
</body>
</html>
el表达式 获取对象的属性值
<%@page import="com.lanou3g.City"%>
<%@page import="java.util.HashMap"%>
<%@page import="java.util.ArrayList"%>
<%@page import="com.lanou3g.User"%>
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<!--
EL表达式 获取对象的属性值
JavaBean导航(对象导航)
-->
<%
City city = new City();
city.setAddress("sh");
User u1 = new User();
u1.setUsername("wanglong");
u1.setPassword("123");
u1.setCity(city);
request.setAttribute("u1", u1);
//创建list 保存2个值 保存到域中
ArrayList<String> list = new ArrayList<String>();
list.add("aaa");
list.add("bbb");
request.setAttribute("l1", list);
//创建一个map 保存2个值 保存到域中
HashMap<String,String> map = new HashMap<String,String>();
map.put("a", "aaaaa");
map.put("b", "bbbbb");
map.put("c", "ccccc");
request.setAttribute("m1", map);
%>
<!--
内部相当于 调用getUsername()方法
用点能获取的用中括号都能获取
用中括号能获取的不一定能用点获取
-->
${u1.username }
${u1["username"] }
${u1['username'] }
${u1.city.address }
${u1["city"]["address"] }
${l1[0] }
${l1[1] }
${m1["a"] }
${m1["b"] }
${m1["c"] }
</body>
</html>
el表达式: 隐式对象
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<!--
EL表达式 隐式对象
注意:隐式对象在EL表达式中书写
-->
<%
//解决乱码
response.setContentType("text/html; charset=UTF-8");
request.setCharacterEncoding("UTF-8");
//利用pageContext 对象 向各个域中保存值
pageContext.setAttribute("xxx", "REQUEST", PageContext.REQUEST_SCOPE);
pageContext.setAttribute("xxx", "SESSION", PageContext.SESSION_SCOPE);
pageContext.setAttribute("xxx", "PAGE", PageContext.PAGE_SCOPE);
pageContext.setAttribute("xxx", "APPLICATION", PageContext.APPLICATION_SCOPE);
%>
<!-- 使用隐式对象 指定域找对应的值 -->
${requestScope.xxx }
${sessionScope.xxx }
${pageScope.xxx }
${applicationScope.xxx }
<form action="${pageContext.request.contextPath }/13.jsp" method="post"><br/>
账号:<input type="text" name="username"/><br/>
密码: <input type="text" name="password"/><br/>
唱歌<input type="checkbox" name="hobby" value="sing"/><br/>
踢球<input type="checkbox" name="hobby" value="ball"/><br/>
<input type="submit" value="提交"/><br/>
</form>
</body>
</html>
el表达式 :获取表单数据
<%@ page language="java" contentType="text/html; charset=UTF-8"
pageEncoding="UTF-8"%>
<!DOCTYPE html PUBLIC "-//W3C//DTD HTML 4.01 Transitional//EN" "http://www.w3.org/TR/html4/loose.dtd">
<html>
<head>
<meta http-equiv="Content-Type" content="text/html; charset=UTF-8">
<title>Insert title here</title>
</head>
<body>
<!-- 获取表单提交的数据 -->
<%
request.setCharacterEncoding("UTF-8");
%>
${param.username }
${param.password }
${paramValues.hobby[0] }
</body>
</html>