ios graphql
Did you ever feel frustrated when working with a REST API, because the endpoints didn’t give you the data you needed for the views in your app? Getting the right information from the server either required multiple requests or you had to bug the backend developers to adjust the API? Worry no more — it’s GraphQL and Apollo to the rescue!
使用REST API时,您是否曾经感到沮丧吗,因为端点没有为您提供应用程序视图所需的数据? 从服务器获取正确的信息要么需要多个请求,要么就不得不调试后端开发人员来调整API? 不再担心-可以通过GraphQL和Apollo进行救援!
GraphQL is a new API design paradigm open-sourced by Facebook in 2015, but has been powering their mobile apps since 2012. It eliminates many of the inefficiencies with today’s REST API. In contrast to REST, GraphQL APIs only expose a single endpoint and the consumer of the API can specify precisely what data they need.
GraphQL是Facebook在2015年开源的一种新API设计范式,但自2012年以来一直为他们的移动应用程序提供动力。它消除了当今REST API的许多低效率问题。 与REST相比,GraphQL API仅公开一个端点,并且该API的使用者可以精确地指定所需的数据。
iOS Apollo安装 (iOS Apollo Installation)
Let’s get started by installing apollo client framework & peer graphql dependencies:1) Cd your xcworkspace file path2) pod ‘Apollo’3) pod install
让我们从安装apollo客户端框架和对等graphql依赖关系开始:1)cd xcworkspace文件路径2)pod'Apollo'3)pod安装
添加代码生成构建步骤 (Adding a code generation build step)
In order to invoke apollo
as part of the Xcode build process, create a build step that runs before "Compile Sources".
为了在Xcode构建过程中调用apollo
,请创建一个在“编译源”之前运行的构建步骤。
- On your application targets’ “Build Phases” settings tab, click the “+” icon and choose “New Run Script Phase”. Create a Run Script, change its name to “Generate Apollo GraphQL API” and drag it just above “Compile Sources”. Then add the following contents to the script area below the shell: 在应用程序目标的“构建阶段”设置选项卡上,单击“ +”图标,然后选择“新建运行脚本阶段”。 创建一个运行脚本,将其名称更改为“ Generate Apollo GraphQL API”,并将其拖到“ Compile Sources”上方。 然后将以下内容添加到外壳下面的脚本区域:
SCRIPT_PATH=”${PODS_ROOT}/Apollo/scripts”cd “${SRCROOT}/${TARGET_NAME}”“${SCRIPT_PATH}”/run-bundled-codegen.sh codegen:generate — target=swift — includes=./**/*.graphql — localSchemaFile=”schema.json” API.swift
SCRIPT_PATH =” $ {PODS_ROOT} / Apollo / scripts” cd “ $ {SRCROOT} / $ {TARGET_NAME}”“ $ {SCRIPT_PATH}” / run-bundled-codegen.sh codegen:generate — target = swift — includes =。/ ** / *。graphql — localSchemaFile =” schema.json” API.swift
schema.json : Name of schema fileAPI.swift : It will be auto generated class after running above script.
schema.json:模式文件的名称API.swift:运行上述脚本后将自动生成的类。
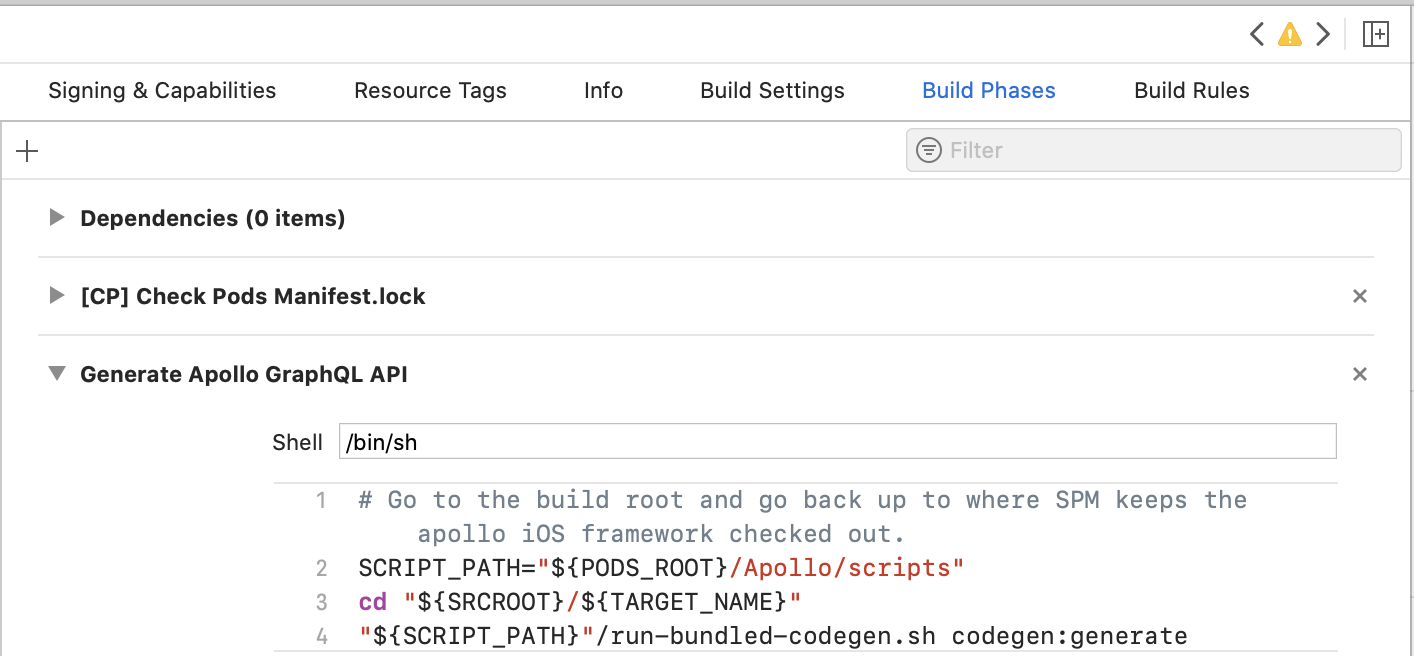
将架构文件添加到目标目录 (Adding a schema file to your target directory)
You’ll have to copy or download a schema to your target directory before generating code.
在生成代码之前,您必须将模式复制或下载到目标目录。
Apollo iOS requires a GraphQL schema file as input to the code generation process. A schema file is a JSON file that contains the results of an an introspection query. Conventionally this file is called schema.json
Apollo iOS需要GraphQL模式文件作为代码生成过程的输入。 模式文件是一个JSON文件,其中包含自省查询的结果。 按照惯例,此文件称为schema.json
To download schema.json
, you need to use the id_token from auth0 and run this in your terminal
要下载schema.json
,您需要使用auth0中的id_token并在终端中运行它
apollo schema:download — endpoint=http://hasura.io/learn/graphql — header=”Authorization: Bearer <token>”
apollo模式:下载—端点= http://hasura.io/learn/graphql —标头=“授权:承载<令牌>”
使用查询或突变创建.graphql文件 (Create .graphql files with your queries or mutations)
Apollo iOS generates code from queries and mutations contained in .graphql
files in your target.
Apollo iOS通过目标中.graphql
文件中包含的查询和变异来生成代码。
A useful convention is to colocate queries, mutations or fragments with the Swift code that uses them by creating <name>.graphql
next to <name>.swift
.
一个有用的约定是通过在<name>.graphql
旁边创建<name>.graphql
来将查询,变异或片段与使用它们的Swift代码并<name>.swift
。
If you have the Xcode add-ons installed, you can use the Xcode companion view to show a .swift
file and the corresponding .graphql
file side by side.
如果安装了Xcode加载项,则可以使用Xcode伴随视图.swift
显示.swift
文件和相应的.graphql
文件。
创建阿波罗客户端 (Create apollo client)
Write your query or mutation inside .graphql file , like this:
在.graphql文件中编写查询或变异,如下所示:
mutation registerUser ($userDetail:UserInput!){createUser(user:$userDetail){idtokenfnamelnameemailbio}}
变异registerUser($ userDetail:UserInput!){createUser(user:$ userDetail){idtokenfnamelnameemailbio}}
//MARK:- Call When Need To Register User With An App
// MARK: -需要注册用户时调用
func registerUser(completion:@escaping (Bool,String?)->()) {let apollo = ApolloClient(url: URL(string: “Your graphql url")!)let sendDict = UserInput(email:”abc@test.com”,fname:”test”, lname: “app”,password:”1234567")apollo.perform(mutation:RegisterUserMutation.init(userDetail:sendDict)) { result inswitch result {case .success(let graphQLResult):if(graphQLResult.errors?.count == nil){completion(true,””)}else{completion(false,graphQLResult.errors?.first?.message)}breakcase .failure(let error):NSLog(“Error while create user: \(error.localizedDescription)”)completion(false,error.localizedDescription)}}}
func registerUser(completion: @escaping (Bool,String?)->()){ let apollo = ApolloClient(url:URL(string:“您的graphql url”)!) let sendDict = UserInput(email:” abc @ test。 com”,fname:“ test”,lname:“ app”,密码:“ 1234567”)apollo.perform(mutation:RegisterUserMutation.init(userDetail:sendDict)){结果inswitch结果{ case .success( let graphQLResult): if (graphQLResult.errors?.count == nil ){completion( true ,”“)} 否则 {completion( false ,graphQLResult.errors?.first?.message)} breakcase .failure( let error):NSLog(” Error while创建用户:\(error.localizedDescription)”)completion( false ,error.localizedDescription)}}}
Mutation is used to save a data in graphql database.
变异用于将数据保存在graphql数据库中。
Let’s take an example of query, it is used to get a data from graphql db.
让我们以查询为例,它用于从graphql db获取数据。
query AllHuntingTypes {selectHuntingTypes {types{ titleid}categories{titleid}}}
查询AllHuntingTypes {selectHuntingTypes {types {titleid} categories {titleid}}}
//MARK:- Call When Need To Fetch All Categories
// MARK: -需要获取所有类别时调用
apollo.fetch(query:AllHuntingTypesQuery.init()){ result inswitch result {case .success(let graphQLResult):if(graphQLResult.errors?.count == nil){self.arrHuntingTypes = graphQLResult.data?.selectHuntingTypes?.types as?[AllHuntingTypesQuery.Data.SelectHuntingType.Type]self.arrHuntingCat = graphQLResult.data?.selectHuntingTypes?.categories as? [AllHuntingTypesQuery.Data.SelectHuntingType.Category]completion(true,””)}else{completion(false,graphQLResult.errors?.first?.message)}breakcase .failure(let error):NSLog(“Error while create user: \(error.localizedDescription)”)completion(false,error.localizedDescription)}}}
apollo.fetch(query:AllHuntingTypesQuery.init()){结果切换结果{ case .success( let graphQLResult): if (graphQLResult.errors?.count == nil ){ self .arrHuntingTypes = graphQLResult.data?.selectHuntingTypes?。类型为 [[AllHuntingTypesQuery.Data.SelectHuntingType.Type] self .arrHuntingCat = graphQLResult.data?.selectHuntingTypes?.categories 作为 ? [AllHuntingTypesQuery.Data.SelectHuntingType.Category] completion( true ,””)} 其他 {completion( false ,graphQLResult.errors?.first?.message)} breakcase .failure( let error):NSLog(“创建用户时出错: \(error.localizedDescription)”)completion( false ,error.localizedDescription)}}}
加起来 (Summing Up)
GraphQL is a modern way to communicate with your server. It can replace the commonly used REST API approach. With GraphQL, you communicate with the back end via just one endpoint using declarative syntax.
GraphQL是与服务器通信的现代方式。 它可以替代常用的REST API方法。 使用GraphQL,您可以使用声明性语法通过一个端点与后端进行通信。
Using GraphQL in your Swift applications for iOS, iPadOS, watchOS, macOS, and tvOS is easy with the Apollo framework. It’s officially supported by the GraphQL community, and lately, it has been well maintained and supported.
使用Apollo框架,可以轻松地在iOS,iPadOS,watchOS,macOS和tvOS的Swift应用程序中使用GraphQL。 它得到了GraphQL社区的正式支持,并且最近得到了很好的维护和支持。
链接 (Links)
Official iOS GraphQL documentation and repository
翻译自: https://medium.com/@sharmadeeksha30/implementation-of-graphql-in-ios-d89de176ef4c
ios graphql