swift编码
问题陈述 (Problem statement)
Nowadays writing code is not a big deal but sometimes writing well-organized & performant code is a tedious task even if you have proper coding guidelines in place. But still, you or maybe your teammates jump or forget to adhere to the in-place coding guidelines. What if we have an automated system, a library, that imposes all the defined rules in your working project that validates each of the code you write in your working project. If you or your team member go off the guidelines. They will immediately be intimated with appropriate flags (with warnings or errors). No, I am not talking some imaginary stuff, it’s doable we have many open-source libraries once we can include them to our project. Our job becomes easy, that library will keep validating the coding rules/guidelines.
如今,编写代码已不是什么大问题,但有时即使编写适当的编码指南,编写井井有条的高性能代码也是一项繁琐的工作。 但是,您或您的队友还是跳起来或忘记遵守就地编码准则。 如果我们有一个自动化系统(一个库),该库在您的工作项目中强加了所有已定义的规则,该规则将验证您在工作项目中编写的每个代码。 如果您或您的团队成员不遵守准则。 它们将立即被适当的标记(带有警告或错误)提示。 不,我不是在谈论一些虚构的东西,一旦我们可以将它们包含到我们的项目中,那么我们有很多开源库是可行的。 我们的工作变得很容易,该库将继续验证编码规则/准则。
Now, You got me, Yes, I am talking about Swiftlint (https://github.com/realm/SwiftLint). It’s a great library that enforces the Swift style and conventions. It provides over 75 rules and the best part of this library is, you can modify define your own custom rule. Also, you can modify the existing rules as per your requirement. Is not it exciting? In this article, I will talk about how to use it, modify, and add our own custom rules. Without wasting a moment, let’s jump to the implementation.
现在,您明白了,是的,我在谈论Swiftlint ( https://github.com/realm/SwiftLint )。 这是一个强大的库,可强制执行Swift样式和约定。 它提供了75多个规则,该库的最佳组成部分是,您可以修改定义自己的自定义规则。 另外,您可以根据需要修改现有规则。 这不令人兴奋吗? 在本文中,我将讨论如何使用它,修改和添加我们自己的自定义规则。 不用浪费时间,让我们跳到实现。
如何安装和使用 (How to Install and use it)
We can use this library in various ways. Some of the ways are listed below
我们可以通过多种方式使用该库。 下面列出了一些方法
Using Homebrew, to install it using the hombrew, run the below command from your mac terminal
brew install swiftlint
使用 Homebrew ,要使用hombrew安装它,请从mac终端
brew install swiftlint
运行以下命令Using CocoaPods, add the following line to your Podfile and run the pod install command from mac terminal
pod `SwiftLint`
使用 的CocoaPods ,下面一行添加到您的Podfile和运行荚从MAC终端安装命令
pod `SwiftLint`
There are many other ways we can install it in our project, go through the documentation (https://github.com/realm/SwiftLint#installation) and install as per your wish. But the recommended way is to install it using Pod since it supports installing a pinned version rather than simply the latest and which is the case with Homebrew.
我们还有许多其他方法可以将其安装到项目中,请查阅文档( https://github.com/realm/SwiftLint#installation )并按照您的意愿进行安装。 但是推荐的方法是使用Pod进行安装,因为它支持安装固定版本,而不是简单地安装最新版本,Homebrew就是这种情况。
与您的Xcode项目集成 (Integrate with your Xcode project)
In order to integrate SwiftLint with Xcode project target to get warnings and errors displayed in the Xcode, you just need to add a new Run Script Phase with the following script
为了将SwiftLint与Xcode项目目标集成在一起 ,以获取在Xcode中显示的警告和错误,您只需要使用以下脚本添加一个新的Run Script Phase
If you are not sure, how to add it follow the instructions below.
如果不确定,请按照以下说明进行添加。
建立 (Build)
Once you are done with Run Script and builds your Xcode project, you might notice a couple of warnings as attached screenshot.
一旦完成了运行脚本并构建了Xcode项目,您可能会注意到一些警告,如所附的屏幕截图所示。
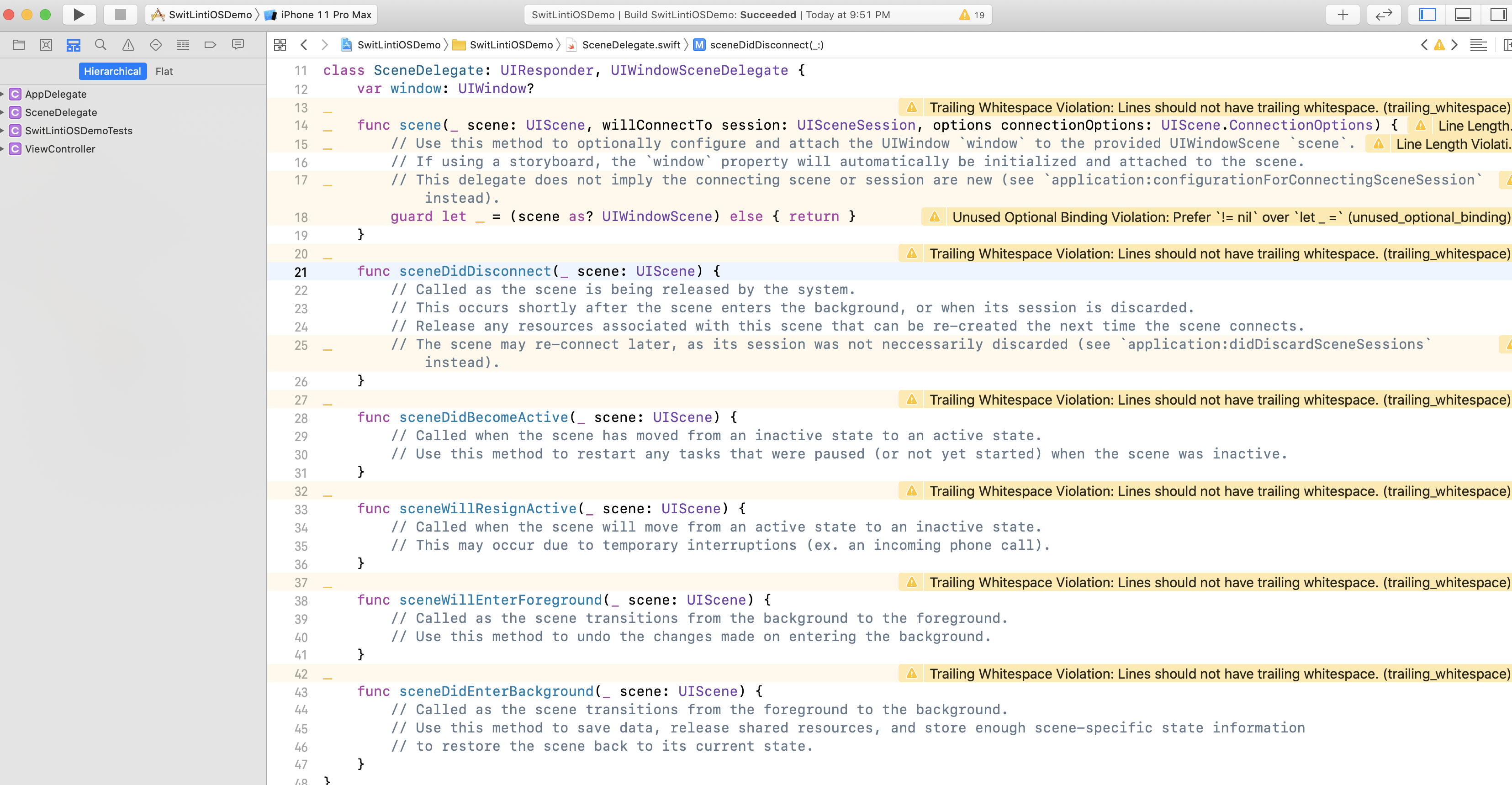
规则与配置 (Rules and Configuration)
We can configure SwiftLint rules as per our need by adding a .swiftlint.yml
file from the directory we run SwiftLint from. Let’s first talk about the configuration parameters.
我们可以根据需要配置SwiftLint规则,方法是从运行SwiftLint的目录中添加.swiftlint.yml
文件。 让我们首先讨论配置参数。
opt_in_rules
are disabled by default (i.e., you have to explicitly enable them in your configuration file). These are the rules that can have many false positives, a rule that is too slow or a rule that is not in general consensus or is only useful in some cases (e.g.force_unwrapping
).opt_in_rules
默认情况下处于禁用状态(即,您必须在配置文件中显式启用它们)。 这些规则可能有很多误报,太慢的规则或没有达成共识或仅在某些情况下有用的规则(例如force_unwrapping
)。disabled_rules
: Disable rules from the default enabled set.disabled_rules
:从默认启用集中禁用规则。whitelist_rules
: Only the rules specified in this list will be enabled. Can not be specified alongsidedisabled_rules
oropt_in_rules
.Also when we create a new custom rule, we have to place it under the whitelist_rules.whitelist_rules
:仅启用此列表中指定的规则。 不能靠指定disabled_rules
或opt_in_rules
。还有,当我们创建一个新的自定义规则,我们必须把它放在whitelist_rules下。analyzer_rules
: This is an entirely separate list of rules that are only run by theanalyze
command. All analyzer rules are opt-in, so this is the only configurable rule list (there is no disabled/whitelist equivalent).analyzer_rules
:这是一个完全独立的规则列表,仅由analyze
命令运行。 所有分析器规则均已选择加入 ,因此这是唯一可配置的规则列表(没有等效的禁用/白名单)。
To know which rule is placed under the opt_in_rules, correctable (auto correctable by running swiftlint autocorrect command), enabled in your configuration, kind, and configuration (whether configured as a warning or an error). Run the swiftlint rules command. Once you run the command it will print the details similar to the given screenshot.
要知道哪个规则位于opt_in_rules下 , 可纠正(可由 运行swiftlint autocorrect命令),并在您的配置,种类和配置中 启用 (无论配置为警告还是错误) 。 运行swiftlint Rules命令。 一旦运行命令,它将打印与给定屏幕截图类似的详细信息。
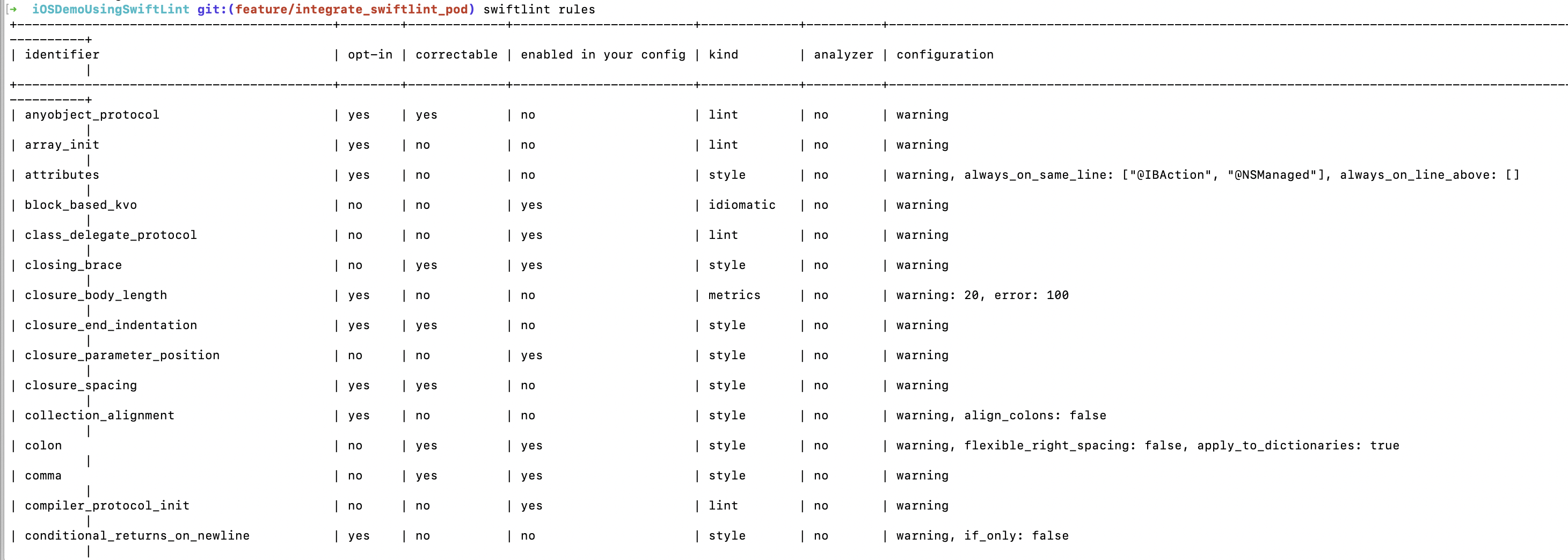
I am attaching the .swiftlint.yml which gives you a better understanding of rules configuration.
我附上了.swiftlint.yml 使您更好地了解规则配置。
如何修改或禁用规则? (How to modify or disable a rule?)
Sometimes, default configured rules do not suit our requirements. We may require to update rule definition or we may require to exclude some of the rules for some classes or specific folders.
有时,默认配置的规则不符合我们的要求。 我们可能需要更新规则定义,或者可能需要排除某些类别或特定文件夹的某些规则。
Disable rule at the project levelDisabling a rule at the project level is not a good idea though sometimes we may need to disable it. We just need to place that particular rule in the disabled_rules
category. Make sure you have the .swiftlint.yml placed inside your project folder.
在项目级别禁用规则在项目级别禁用规则不是一个好主意,尽管有时我们可能需要禁用它。 我们只需要将该特定规则放在disabled_rules
类别。 确保将.swiftlint.yml放置在项目文件夹中。
Disable rule within a source fileWe can disable the rules within source file with the given format// swiftlint:disable <rule1> [<rule2> <rule3>...]
This way rules will be disabled until the end of the file or until the linter sees a matching enable comment like as below// swiftlint:enable <rule1> [<rule2> <rule3>...]
在源文件中禁用规则我们可以在给定格式的源文件中禁用规则// swiftlint:disable <rule1> [<rule2> <rule3>...]
这样,规则将被禁用,直到文件结尾或直到// swiftlint:enable <rule1> [<rule2> <rule3>...]
看到匹配的启用注释,如下所示// swiftlint:enable <rule1> [<rule2> <rule3>...]
Or if we want to we can use all
keyword and it will disable all rules until the linter sees a matching enable comment// swiftlint:disable all
// swiftlint:enable all
或者,如果我们愿意,可以使用all
关键字,它将禁用所有规则,直到// swiftlint:disable all
看到匹配的启用注释// swiftlint:disable all
// swiftlint:enable all
As you can see in the attached screenshot below. I have disabled the identifier name rule for constant i. It is not getting any violation triggered. But for constant j identifier name violation is triggered. Because as soon the linter sees the enable rule command. It enabled the rule again for the subsequent lines.
正如您在下面的屏幕截图中所见。 我已禁用 常数 i 的 标识符名称 规则 。 它没有触发任何违规。 但是对于常数 j, 会触发标识符名称冲突。 因为linter很快会看到enable rule命令。 它再次为后续行启用了该规则。
如何添加自定义规则? (How to add a custom rule?)
SwiftLint provides an easy way to add a custom rule. In order to add a custom rule, we need to define custom regex-based rules in our swiftlint.yml configuration.
SwiftLint提供了一种添加自定义规则的简便方法。 为了添加自定义规则,我们需要在swiftlint.yml配置中定义基于自定义正则表达式的规则。
For example, we have a use case where we don’t want to apply the conditinal_return_on_newline for guard statement but want to keep it applicable for the rest of the conditional statements. we can create a custom which skips the validation for guard statement but validate the rest of the conditional statements.
例如,我们有一个用例,我们不想将conditinal_return_on_newline用于保护语句,但希望使其适用于其余条件语句。 我们可以创建一个自定义,该自定义跳过对保护语句的验证,但对其余条件语句进行验证。
Disable the
conditional_returns_on_newline
the rule for the whole project. Put this rule under disabled_rules in the configuration file对整个项目 禁用
conditional_returns_on_newline
规则。 将此规则放在 配置文件中的 disabled_rules 下Define a custom regex-based rules in your configuration
在配置中定义基于自定义正则表达式的规则
Add a newly created rule in
whitelist_rules
the section of .swiftlint.yml在
whitelist_rules
添加新创建的规则 的 .swiftlint.yml部分
The possible .swiftlint.yml configuration file will look like an attached screenshot.
可能的.swiftlint.yml配置文件看起来像所附的屏幕截图。
Note- When we need to add a new rule which is not provided in the swiftlint rules. Then form a valid regex, define it under the custom_rules ( in .swiftlint.yml) with all required parameters, and add it to the whitelist_rules section.
注意: 当我们需要添加 swiftlint 规则中 未提供的 新 规则时。 然后形成一个有效的 正则表达式 , 使用所有必需的参数 在 custom_rules (在 .swiftlint.yml中) 下定义它 ,并将其添加到 whitelist_rules 部分。
回顾 (Recap)
SwiftLint helps us to enforce swift coding styles and conventions. Also, allow us to modify existing rules, disable or add a new custom rule as per our requirements. This was a small insight into SwiftLint, I would suggest you dive into it to use in better way.
SwiftLint帮助我们实施快速的编码样式和约定。 此外,允许我们根据我们的要求修改现有规则,禁用或添加新的自定义规则。 这只是对SwiftLint的一小部分见解,建议您深入研究一下以更好地使用它。
I hope you like this article. Suggestion and feedback are most welcomeEnjoy ✌️😊
希望您喜欢这篇文章。 欢迎提出建议和反馈享受En️😊
翻译自: https://medium.com/@kamarshad/how-to-enforce-the-swift-coding-styles-conventions-d13d70babb71
swift编码