ios并发
Concurrency is achieved when the app is capable to run multiple tasks at the same time. Latest A13 Bionic comes with 6 cores which means that it has the capacity to execute 6 tasks at a time. Yes, one core can execute at-most one task at at time. To learn more about the cores, please go through Cores
当应用程序能够同时运行多个任务时,就可以实现并发。 最新的A13 Bionic具有6个内核,这意味着它具有一次执行6个任务的能力。 是的,一个核心一次最多可以执行一项任务。 要了解有关内核的更多信息,请遍历内核
All the latest iphones has the capacity to run multiple tasks at a time which improves the performance of the app. But how does it do that? Let’s understand this in this post.
所有最新的iPhone均具有一次运行多个任务的能力,从而提高了应用程序的性能。 但是它是怎么做到的呢? 让我们在这篇文章中了解这一点。
When we tap on the app icon on the home screen on our phone, a program gets executed by our operating system (OS will have all the addresses and meta data to launch the program after the app gets installed). Then this program creates process to start the execution which starts with the initial point(in iOS it’s called main.m). During the lifetime of this process, it may creates many threads (smallest sequence of instructions to be executed by the cores). Let’s understand these terminologies first -
当我们点击手机主屏幕上的应用程序图标时,程序将由我们的操作系统执行(安装该应用程序后,操作系统将具有所有地址和元数据以启动该程序)。 然后,此程序创建一个过程以开始执行,该过程从初始点开始(在iOS中称为main.m)。 在此过程的整个生命周期中,它可能会创建许多线程(内核将执行的最小指令序列)。 首先让我们了解这些术语-
线 (Thread)
In very simple words, it’s a smallest sequence of instructions which gets executed by the cores available on the device.
简单来说,这是由设备上可用内核执行的最小指令序列。
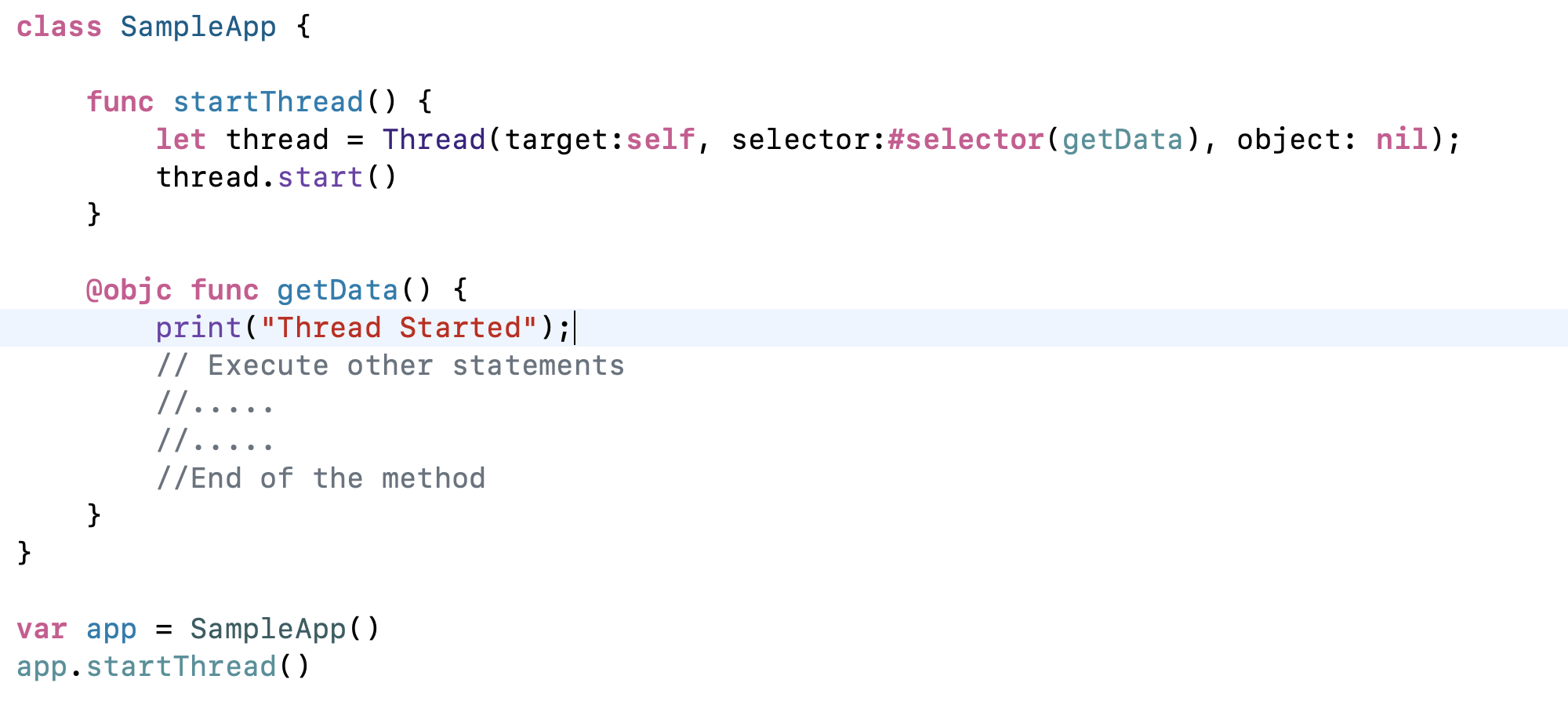
Above code shows how we create a seperate thread to execute some code in getData method. As the scope of this thread is at the method level, it will be destroyed by the OS as soon as it completes getData()
上面的代码展示了我们如何创建一个单独的线程来执行getData方法中的某些代码。 由于此线程的作用域在方法级别,因此一旦完成getData() ,它将被操作系统销毁。
The app starts with Main Thread which executes all the UI level instructions and also manages some background threads to execute background tasks. I would highly recommend you to go through Threads in deep as it will help you understand how iOS schedules and execute tasks on multiple cores at a time. To read more about Threads please go through- Threads
该应用程序从执行所有UI级别指令的主线程开始,并且还管理一些后台线程以执行后台任务。 我强烈建议您深入了解Threads,因为它将帮助您了解iOS如何一次在多个内核上调度和执行任务。 要了解有关线程的更多信息,请浏览-线程
处理(Process)
A process is the one which creates and manages all the threads required to execute a program. Consider a facebook app on our iphone. On the OS level, it’s stored as a program and when we launch it, it creates a process which in-turn creates threads to run our instructions. So a process can have multiple threads running a the same time and in a similar way, a program can have multiple processes running at the same time.
进程是创建和管理执行程序所需的所有线程的进程。 考虑在我们的iPhone上使用Facebook应用程序。 在操作系统级别,它存储为程序,当我们启动它时,它会创建一个过程,该过程又会创建线程来运行我们的指令。 因此,一个进程可以有多个线程同时运行,并且以类似的方式,一个程序可以有多个进程在同一时间运行。
So the finals words are — A process creates or managess one or more threads to execute the instructions. A program creates or manages one or more process to start and run the program till it’s completion.
因此,最后的词是-进程创建或管理一个或多个线程来执行指令。 程序创建或管理一个或多个过程以启动和运行该程序,直到完成为止。
An iOS app is a program which, when launched, creates a process that creates and manages threads to execute the instructions in our code. Our app comes with One Main Thread that executes all the UI level tasks and mostly runs on high performance cores (every processor has some high performance cores). iOS gives us options to create other threads to run our code in background to acheive better performance because if those background tasks are run on the same main thread, our UI will get unresponsive for the time the other tasks get executed.
iOS应用程序是一个程序,启动后会创建一个进程,该进程创建和管理线程以执行我们代码中的指令。 我们的应用程序带有一个执行所有UI级别任务的主线程,并且主要在高性能内核上运行(每个处理器都有一些高性能内核)。 iOS提供了创建其他线程的选项,以便在后台运行我们的代码以实现更好的性能,因为如果这些后台任务在同一主线程上运行,则在执行其他任务时,我们的UI将无响应。
iOS gives some high level abstractions too to create and handle threads. Example are — GCD (Grand Central Dispatch), Operation Queues.
iOS也提供了一些高级抽象来创建和处理线程。 例如-GCD (中央调度),操作队列。
大中央派遣(GCD) (Grand Central Dispatch (GCD))
It’s similiar C’s libdispatch library. It’s purpose is to queue up tasks (piece of code e.g. method or closure) that can be run in parallel by the available cores. GCD is built upon the Threads API so it does all the work the same way but we don’t have to worry about creating and managing Threads, which saves a lot of effort and time. Pass a closure and GCD will take care of executing it with the attached thread.We can enqueue n number of tasks to a queue but they are executed FIFO(First in First Out). These queues will have a pool of threads attached managed by the OS.
这是类似C的libdispatch库。 目的是使可以由可用内核并行运行的任务(代码段,例如方法或闭包)排队。 GCD是基于Threads API构建的,因此它以相同的方式完成所有工作,但是我们不必担心创建和管理Threads,从而节省了大量的精力和时间。 传递一个闭包,GCD将负责通过连接的线程执行它。我们可以将n个任务排队到一个队列中,但是它们以FIFO(先进先出)的方式执行。 这些队列将具有由操作系统管理的附加线程池。
Serial Queues have one thread attached and Concurrent queues can have as many threads as the system has resources for to run tasks in parallel.
串行队列附加了一个线程,并且并行队列可以具有与系统具有并行运行任务资源一样多的线程。
Even GCD start execution of the tasks in FIFO but it doesn’t ensure that the tasks will finish in the order we submit them, rather this depends on the task’s complexity and the time it can take.
甚至GCD都开始在FIFO中执行任务,但是它不能确保任务按我们提交的顺序完成,而是取决于任务的复杂性和所需的时间。
There’re two types of Queues that iOS provides to execute our tasks in parallel
iOS提供了两种类型的队列来并行执行我们的任务
- Serial Queues串行队列
- Concurrent Queues并发队列
串行队列(Serial Queues)
Serial queues has only single thread associated with them and thus allows a single task to be executed at a given time. Our App runs on a Serial Queue which is called Main Queue. All the UI level operations run on this queue and if we execute any other task in the same queue, our UI might get unresponsive until the other tasks gets completed. So it’s highly recommended to use main queue only for UI related stuff.
串行队列只有一个与之关联的线程,因此允许在给定时间执行单个任务。 我们的应用程序在称为主队列的串行队列上运行。 所有UI级别的操作都在此队列上运行,如果我们在同一队列中执行任何其他任务,则在其他任务完成之前,我们的UI可能会变得无响应。 因此,强烈建议仅将主队列用于与UI相关的内容。
并发队列 (Concurrent Queues)
A concurrent queue, on the other hand, is able to utilize as many threads as the system has resources for. Threads will be created and released as necessary on a concurrent queue.
另一方面,并发队列可以利用系统拥有资源的线程数。 将根据需要在并发队列上创建和释放线程。
Note: While you can tell iOS that you’d like to use a concurrent queue, remember that there is no guarantee that more than one task will run at a time. If your iOS device is completely bogged down and your app is competing for resources, it may only be capable of running a single task.
注意:虽然您可以告诉iOS您想使用并发队列,但是请记住,不能保证一次运行多个任务。 如果您的iOS设备完全停滞不前,而您的应用程序正在争夺资源,则它可能只能运行一项任务。
串行队列示例 (Example of a Serial Queue)
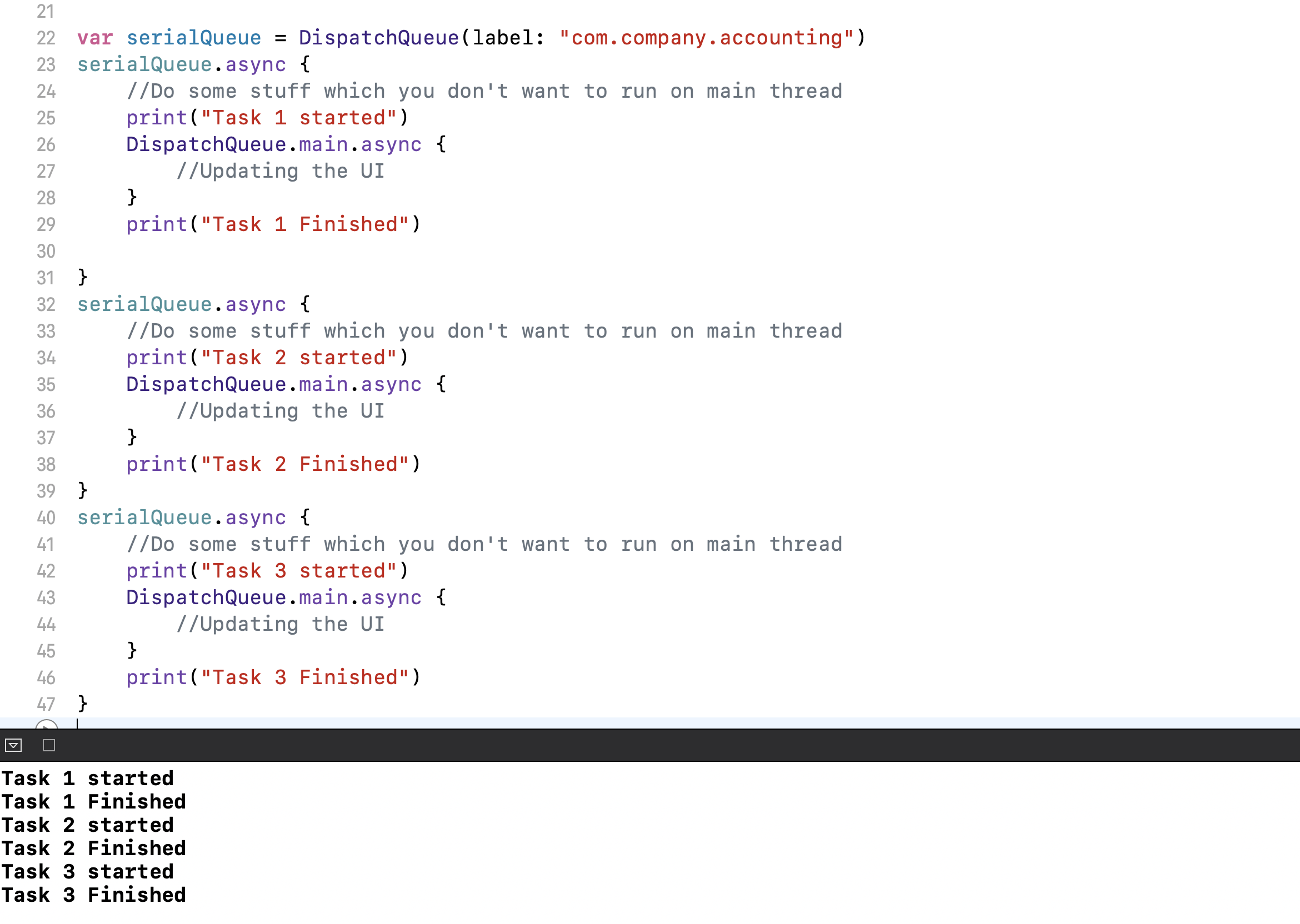
As you can see, three blocks of code are asynchrounously enqueued in the serial queue and the result shows that the second tasks start only when the first is finished and same goes for 2nd and 3rd.
如您所见,三个代码块被非同步地排队在串行队列中,结果表明,第二个任务仅在第一个任务完成时才开始,而第二个任务和第三个任务都去了。
并发队列示例 (Example of a Concurrent Queue)
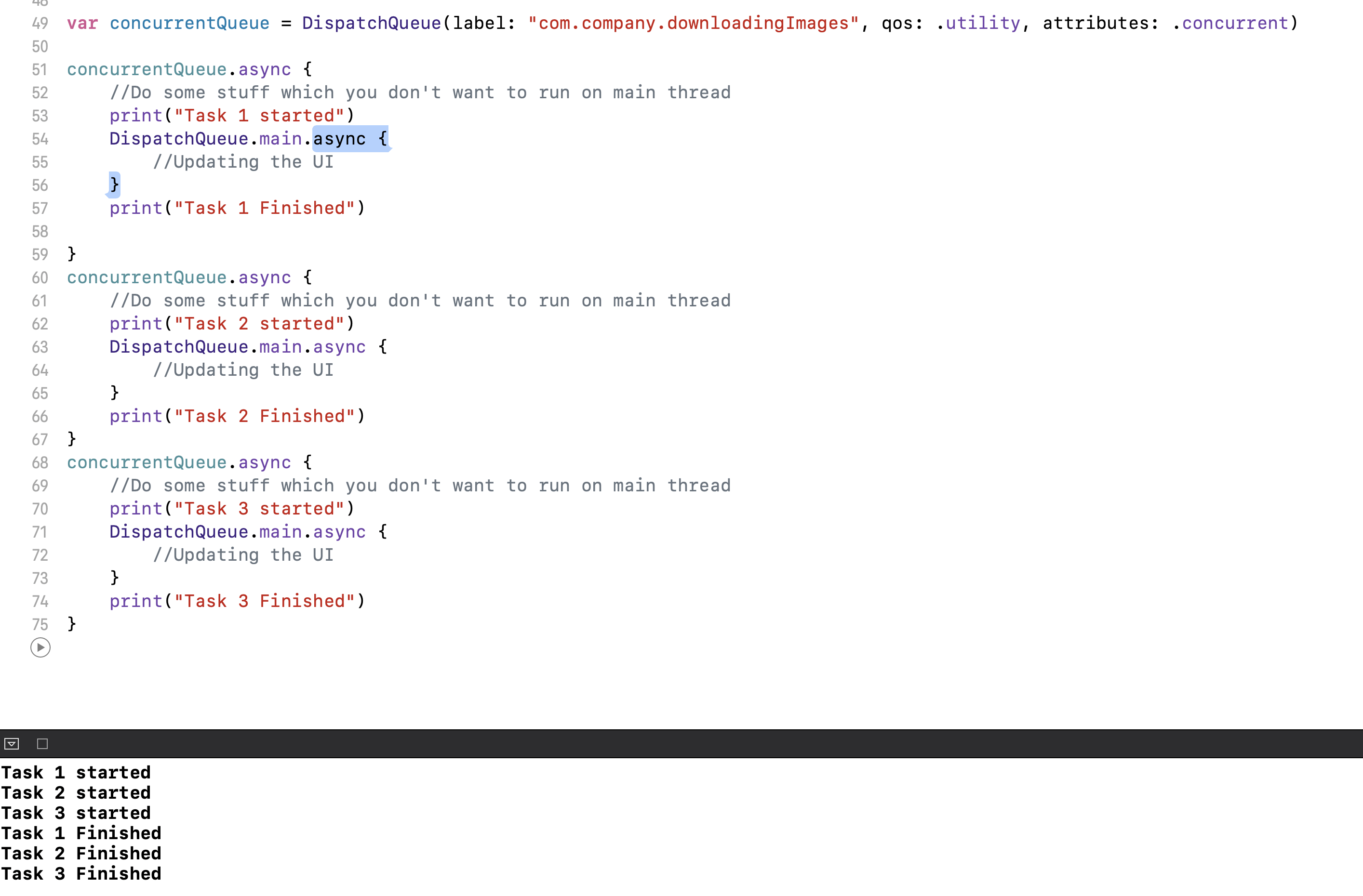
In this example, a we enqueue three blocks to execute and the results shows that the 2nd and 3rd tasks starts executing before even the first is finished. This means that the task will be executed concurrently on different cores.
在此示例中,我们排队三个要执行的块,结果显示第2个和第3个任务甚至在第一个任务完成之前就开始执行。 这意味着任务将在不同的内核上同时执行。
I can’t cover GCD in full in this post, probably will write a separate one just to explain GCD and it’s terminologies. If you’re eager to learn more, go through the official documention for the same- Queues
我不能在这篇文章中完整地介绍GCD,可能会写一个单独的文章来解释GCD及其术语。 如果你渴望了解更多信息,补办各项─正式机制的文档队列
异步并不意味着并发(Asynchronous doesn’t mean concurrent)
WOn the other hand, categorizing something as serial versus concurrent identifies whether the queue has a single thread or multiple threads available to it. If you think about it, submitting three asynchronous tasks to a serial queue means that each task has to completely finish before the next task is able to start as there is only one thread available.In other words, a task being synchronous or not speaks to the source of the task. Being serial or concurrent speaks to the destination of the task.
另一方面,将事物分类为串行还是并发可以识别队列是具有一个线程还是多个线程。 如果考虑一下,将三个异步任务提交到串行队列意味着每个任务必须完全完成才能启动下一个任务,因为只有一个线程可用。任务的来源。 串行或并发表明任务的目的地。
运作方式 (Operations)
Just like GCD, Operations and OperationQueue are the highest level of abstraction to achieve concurrency in our app. Operations are fully-functional classes that can be submitted to an OperationQueue, just like you’d submit a closure of work to a DispatchQueue for GCD. Because they’re classes and can contain variables, you gain the ability to know what state the operation is in at any given point.
与GCD一样,Operations和OperationQueue是实现应用程序中并发性的最高抽象级别。 操作是可以提交给OperationQueue的功能齐全的类,就像您将工作结案提交给GCD的DispatchQueue一样。 因为它们是类并且可以包含变量,所以您可以知道操作在任何给定点处于什么状态。
At a time, a operation can be in any of these state-
一次操作可以处于以下任何一种状态-
isReady
准备好了
isExecuting
正在执行
isCancelled
取消
isFinished
已完成
Each state’s name explain the current status of the operation.
每个州的名称都说明了该操作的当前状态。
Point to note here is, if an operation is in isExecuting state and we set isCancelled to true that doesn’t mean that the operation will be immediately cancelled. It’ll just set the property to isCancelled.
这里要注意的是,如果某个操作处于isExecuting状态,并且我们将isCancelled设置为true,则并不意味着该操作将立即被取消。 只需将属性设置为isCancelled。
Operations provide greater control over your tasks as you can now handle such common needs as cancelling the task, reporting the state of the task, wrapping asynchronous tasks into an operation and specifying dependencies between various tasks. I’m not going in deep about operations but you can read about them right here- OperationQueues
操作现在可以更好地控制任务,因为您现在可以处理诸如取消任务,报告任务状态,将异步任务包装到操作中以及指定各种任务之间的依存关系等常见需求。 我不会去深约操作,但你可以看到他们的权利这里- OperationQueues
结论 (Conclusion)
To make performant and powerful apps, Concurrent programming becomes the most important subject to learn. Even though iOS provides higher level abstractions like GCD and Operations, it becomes really important to know how and when to use them. I would suggest everyone if they are new to iOS development, to go through GCD, Operations in detail and also learn how and when these abstractions work best. It’s equally important to learn how the threading works underneath to understand the low level execution for making better decisions. There’re other important terminologies like Semaphores, Groups and Locks which we should know but I’m keeping them for another post.
为了使性能和功能强大的应用程序,并发编程成为最重要的学习主题。 即使iOS提供了更高级别的抽象(如GCD和操作),了解如何以及何时使用它们也变得非常重要。 我会建议每个人,如果他们是iOS开发的新手,请仔细阅读GCD,Operations,并学习如何以及何时最好地使用这些抽象。 同样重要的是,了解线程在下面的工作方式,以了解低级执行以做出更好的决策。 我们还应该知道其他一些重要的术语,例如信号量,组和锁,但我将其保留在另一篇文章中。
Thanks For Reading 😀
感谢您的阅读😀
Bye 👋
再见👋
翻译自: https://medium.com/analytics-vidhya/understand-terminologies-in-concurrency-ios-f945beee88e2
ios并发