SWIFT-STRING(SWIFT — STRINGS)
串 (String)
String is a collection of characters. In Swift, a series of characters are represented by String type. Let’s have a quick look into an example below:
字符串是字符的集合。 在Swift中,一系列字符由String类型表示。 让我们快速浏览以下示例:
import UIKit
//String created using String instance
let someString = String("Hello, world!")
print("String is: \(someString)")
//String is: Hello, world!
Now we will look into string literals.
现在,我们将研究字符串文字。
字符串字面量 (String Literal)
String literal is the sequence of characters enclosed in double quote. This can also be used for initializing the string. When a variable is initialized with string literal, Swift compiler infer it as String
type. Let look at few examples:
字符串文字是用双引号引起来的字符序列。 这也可以用于初始化字符串。 当使用字符串文字初始化变量时,Swift编译器将其推断为String
类型。 让我们看几个例子:
//String created using String literal
let stringUsingLiteral = "Hello, world!"
print("String is: \(stringUsingLiteral)")
//String is: Hello, world!
In above example, “Hello, world!” is the string literal assigned to stringUsingLiteral
variable. Swift infer this as String
.
在上面的示例中,“您好,世界! ”是分配给stringUsingLiteral
变量的字符串文字。 Swift将其推断为String
。
String literal is the sequence of characters enclosed in double quote.
字符串文字是用双引号引起来的字符序列。
Now as you seen in the above example, you may raise few questions like, Can we use as much number of character as we wish in the string literal? Can string literal be multi-line sentences also, like paragraphs? Can string literal provides the basic sentence formatting?
现在,如您在上面的示例中看到的那样,您可能会提出一些问题,例如,我们可以在字符串文字中使用所需数量的字符吗? 字符串文字也可以像段落一样是多行句子吗? 字符串文字可以提供基本的句子格式吗?
In short, can we write a story as string literal and assign it to a string variable???
简而言之,我们可以将故事写为字符串文字并将其分配给字符串变量吗???
Answer is YES! You can. Surprised?!!! Just read further and see these string literal features provided by Swift. Swift provides Multiline String Literals for this.
答案是肯定的! 您可以。 惊讶吗?! 只需进一步阅读,即可查看Swift提供的这些字符串文字功能。 Swift为此提供了多行字符串文字。
多行字符串文字: (Multiline String Literals:)
三重引号—“”” (Three Double Quote — “””)
Multiline string literal is a string or the sequence of strings spanned over multiple lines and enclosed between three double quotation marks delimiter.
多行字符串文字是跨越多行并包含在三个双引号定界符之间的字符串或字符串序列。
Wait!!! what? Just earlier we have seen that string literals are enclosed between just a double quote, then why THREE DOUBLE QUOTATION MARK now? Yes, multiline string literals needs three double quotation marks. Let’s take a look at the example.
等待!!! 什么? 刚才我们已经看到字符串文字被括在双引号之间,那么为什么现在要加三个双引号呢? 是的,多行字符串文字需要三个双引号。 让我们看一个例子。
let storyStringLiteral = """
A lion was once sleeping in the jungle when a mouse
started running up and down his body just for fun.
This disturbed the lion’s sleep, and he woke up quite angry.
"""
print(storyStringLiteral)
/*
A lion was once sleeping in the jungle when a mouse
started running up and down his body just for fun.
This disturbed the lion’s sleep, and he woke up quite angry.
*/
As you can see above there are the story is written in three lines — Line 2, 3 and 4. And we can see that it has been printed in three separate lines also — Line 10, 11 and 12.
正如您在上面看到的,故事是用三行写成的-第2、3和4行。我们可以看到它也已经印刷在三行中了-第10、11和12行。
Multiline string literal is spanned over multiple lines, enclosed in three double quotes.
多行字符串文字跨越多行,并用三个双引号引起来。
新线概念 (New Line Concept)
Now a point to be noted quickly is that, a multi-line string literal content must not begin on the line which contains the start delimiter. And in same way closing delimiter should not be on the line same line in which content ends, it must be after the line on which content ends.
现在,需要快速注意的一点是,多行字符串文字内容一定不能在包含开始定界符的行上开始。 并且以同样的方式,结束定界符不应位于内容结束的同一行上,而必须位于内容结束的行之后。
Read above lines again!
再次阅读以上内容!
In above example, can’t we start writing the story content from the line 1 itself? And, can’t we put end delimiter in same line in which story ends? NO, we can’t. If we do, the compiler will throw error. See example below:
在上面的示例中,我们不能从第1行本身开始编写故事内容吗? 而且,我们不能将结束定界符放在故事结束的同一行吗? 不,我们不能。 如果这样做,编译器将抛出错误。 请参见下面的示例:

So what happened to the blank spaces in the line 1 and will line 5? Will that also be included in the string literal or not? NO, the delimiters lines are not included in the string literals. So line 1 and line 5 will won’t be considered while the string operations.
那么第1行和第5行的空白发生了什么? 是否还会将其包含在字符串文字中? 不,分隔符行不包含在字符串文字中。 因此,在进行字符串操作时将不考虑第1行和第5行。
Multiline string literals content must begin on a new line and closing delimiter must begin on a new line. Delimiter lines are not included in the string literals operations.
多行字符串文字内容必须在新行开始,并且结束定界符必须在新行开始。 分隔符行不包括在字符串文字操作中。
避免换行 (Avoiding Line Breaks)
Let’s look at example below first,
我们先来看下面的例子
let quote = """
"It takes many good deeds to build a good reputation,
and only one bad one to lose it."
"""
print(quote)
/*
"It takes many good deeds to build a good reputation,
and only one bad one to lose it."
*/
In above example, you can see that the quoted line is actually written in two lines. This has been done by introducing new line after word “reputation,”. But don’t you think a quote looks good in single line. So how do we achieve that? That can be achieved by writing backslash “\” after word “reputation,” in above example. See below:
在上面的示例中,您可以看到带引号的行实际上写成两行。 这是通过在“声誉”一词之后引入新行来完成的。 但是,您不认为单行引号看起来不错。 那么我们如何实现呢? 在上述示例中,可以通过在单词“ reputation”之后写反斜杠“ \”来实现。 见下文:
let quote = """
"It takes many good deeds to build a good reputation,\
and only one bad one to lose it."
"""
print(quote)
/*
"It takes many good deeds to build a good reputation, and only one bad one to lose it."
*/
In above example you can see that, when backslash is introduced at the end of Line 2, then the whole statement is printed in single line (Line 9).
在上面的示例中,您可以看到,当在第2行的末尾引入反斜杠时,整个语句将打印在一行(第9行)中。
This is useful in cases when for better readability purposes, you may write shorter lines in source code inside the multiline string literals. For doing this you must have used line break after few words itself. But you may not want the line break in the string value or when it is displayed, in those cases you can use backslash at the end of the lines.
当出于更好的可读性目的而在多行字符串文字内的源代码中编写较短的行时,这很有用。 为此,您必须在几句话之后使用换行符。 但是,您可能不希望在字符串值中或在显示字符串值时出现换行符,在这种情况下,可以在行尾使用反斜杠。
But what to do if the multiline string literals itself have backslash in the string content? Think! We will discuss later in this reading.
但是,如果多行字符串文字本身在字符串内容中带有反斜杠,该怎么办? 认为! 我们将在本阅读后面讨论。
When source code includes a line break inside of a multiline string literal, that line break also appears in real string’s value. If you want to avoid the line break then use the backslash ‘\’ at the end of those lines.
当源代码在多行字符串文字中包含换行符时,该换行符也会出现在实际字符串的值中。 如果要避免换行,请在这些行的末尾使用反斜杠“ \”。
引入空白行 (Introducing Blank Line)
Suppose you are writing a short story inside a multiline string literals, and you want to introduce blank line between two paragraphs, how you will achieve this? It’s simple, just introduce blank line in the source, it will replicate in the string value also. Look at the below example.
假设您在多行字符串文字中写了一个短篇小说,并且想在两段之间引入空白行,您将如何实现? 很简单,只需在源代码中引入空白行,它也会在字符串值中复制。 看下面的例子。
let story = """
A lion was once sleeping in the jungle when a mouse started running up
and down his body just for fun. This disturbed the lion’s sleep,
and he woke up quite angry. He was about to eat the mouse when
the mouse desperately requested the lion to set him free.
“I promise you, I will be of great help to you someday if you save me.”
The lion laughed at the mouse’s confidence and let him go.
One day, a few hunters came into the forest and took the lion with them.
They tied him up against a tree. The lion was struggling to get out and
started to whimper. Soon, the mouse walked past and noticed the lion in trouble.
Quickly, he ran and gnawed on the ropes to set the lion free.
Both of them sped off into the jungle.
"""
print(story)
/*
A lion was once sleeping in the jungle when a mouse started running up
and down his body just for fun. This disturbed the lion’s sleep,
and he woke up quite angry. He was about to eat the mouse when
the mouse desperately requested the lion to set him free.
“I promise you, I will be of great help to you someday if you save me.”
The lion laughed at the mouse’s confidence and let him go.
One day, a few hunters came into the forest and took the lion with them.
They tied him up against a tree. The lion was struggling to get out and
started to whimper. Soon, the mouse walked past and noticed the lion in trouble.
Quickly, he ran and gnawed on the ropes to set the lion free.
Both of them sped off into the jungle.
A lion was once sleeping in the jungle when a mouse
started running up and down his body just for fun.
This disturbed the lion’s sleep, and he woke up quite angry.
*/
You can see that the blank line introduced at Line 8. And when string is printed, the same blank is introduced at Line 25.
您会看到在第8行引入了空白行。当打印字符串时,在第25行引入了相同的空白。
Blank line in source replicates in the multiline string value also.
源中的空白行也将复制多行字符串值。
线缩进 (Line Indentation)
Now we will see how we can indent the multiline strings. There are multiple cases, let first start with adding few whitespaces at the beginning of the line. If you write whitespaces before the starting of the line, then that whitespace is included in the string value.
现在,我们将看到如何缩进多行字符串。 有多种情况,让我们首先从在行首添加几个空格开始。 如果在行首之前写空格,则该空格将包含在字符串值中。

In above example, you can see that whitespaces in first line are included in the string value.
在上面的示例中,您可以看到字符串值中包含第一行中的空格。
Whitespaces before the starting of the line is included in the multiline string literal value.
多行字符串文字值中包含行开头之前的空格。
The next question is how to indent whole multiline string? Well, this can be done by indenting the closing delimiter appropriately. Any space on the left of the closing delimiter is ignored in the string value. Let’s look into multiple examples below:
下一个问题是如何缩进整个多行字符串? 好吧,这可以通过适当地缩进闭合定界符来实现。 字符串值中忽略右定界符左侧的任何空格。 让我们看下面的多个示例:
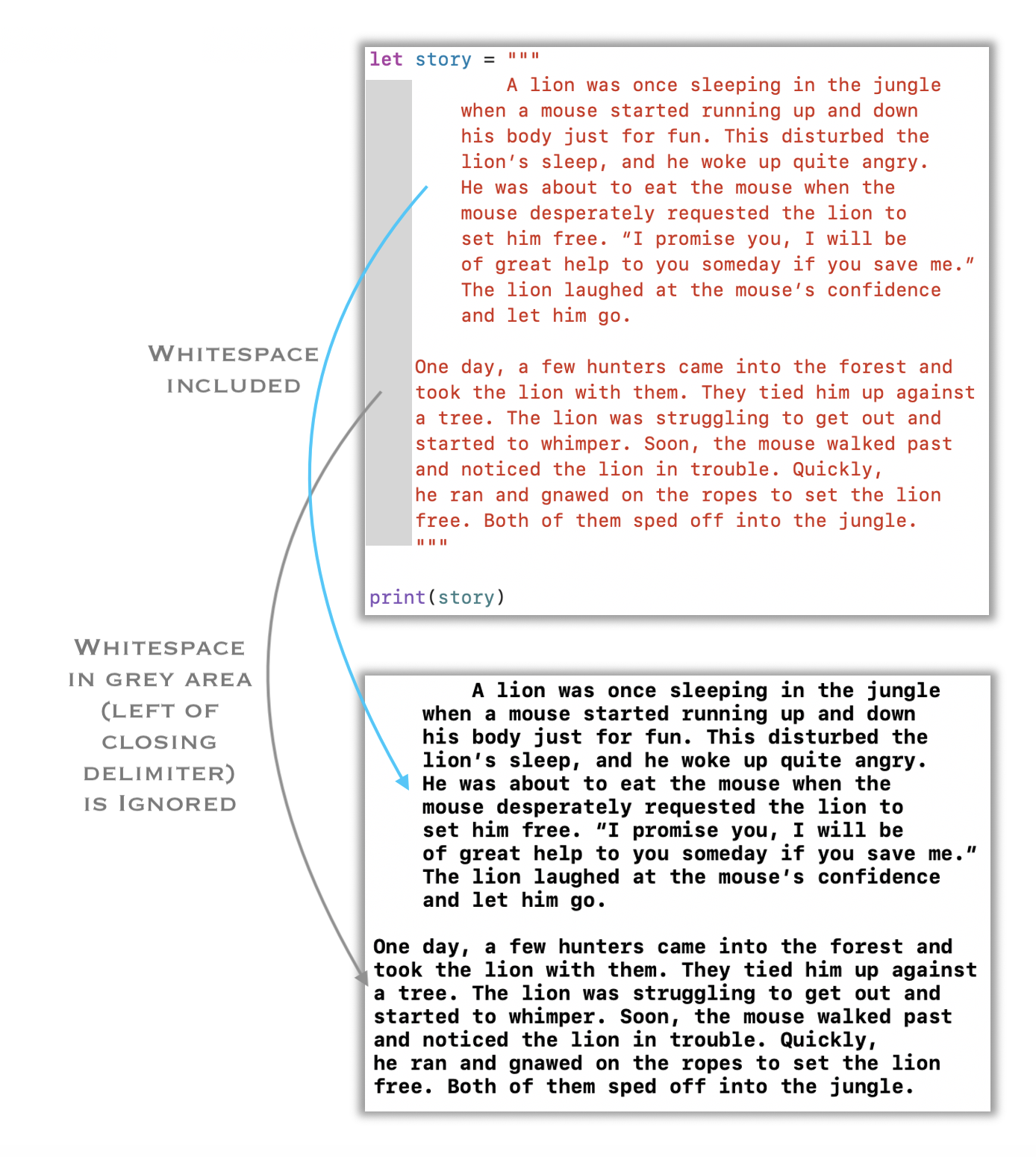
In above example you can see that any whitespace before closing delimiter, (grey area) is ignored while string is displayed. But any space along with or after closing delimiter is included in the string value and it is shown when string is displayed.
在上面的示例中,您可以看到在显示字符串时,在关闭定界符(灰色区域)之前的所有空格都将被忽略。 但是,在字符串值中包括与结束定界符一起或之后的任何空格,并且在显示字符串时会显示该空格。
Any guess, what will happen in below case, when closing delimiter is itself after the multiline string literal sentences?
任何猜想,在多行字符串文字句子后面关闭分隔符本身时,在以下情况下会发生什么?
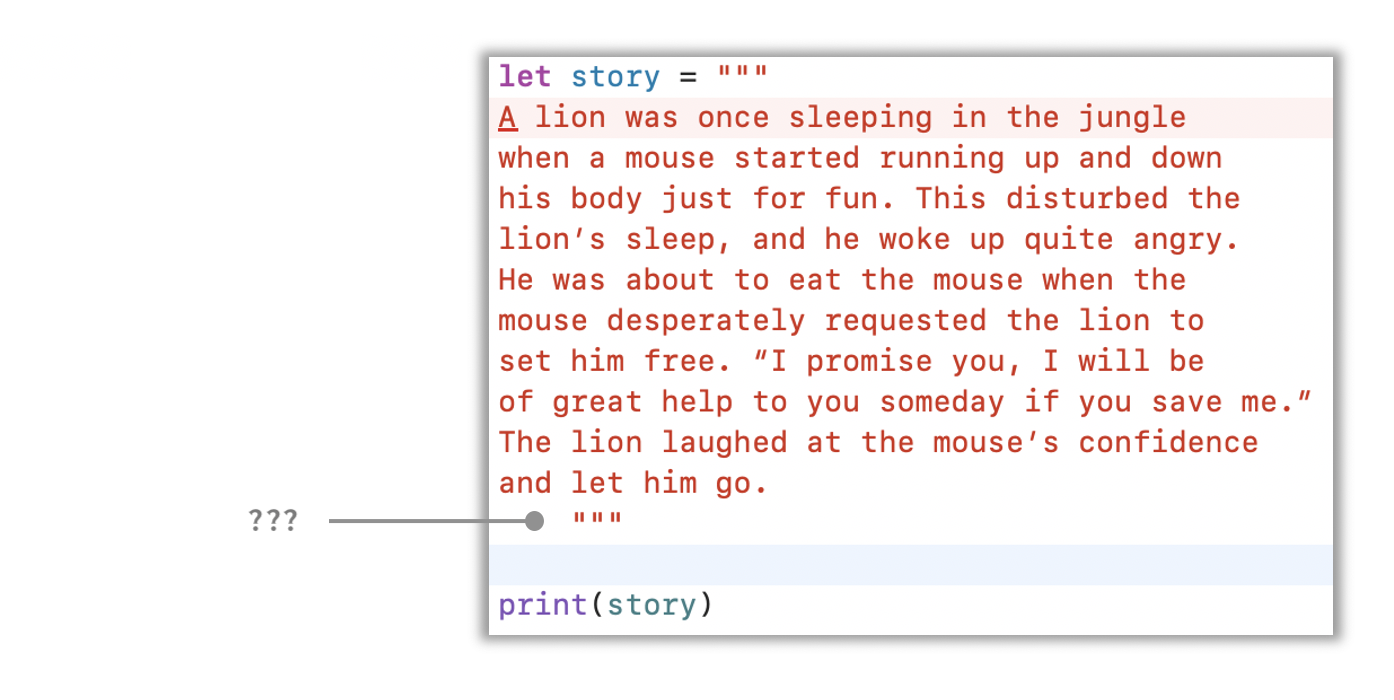
You can’t do this. Compiler will throw below error!
你做不到编译器将抛出以下错误!

Whitespaces before the closing delimiter are ignored for all the other lines. But whitespaces along or after are included.
对于其他所有行,在结束定界符之前的空格将被忽略。 但是,包括前后的空格。
That’s all for the string literals! But don’t go anywhere we are not done yet. Let’s approach towards the special characters in the String literals.
字符串文字就这些了! 但是不要走到我们还没有完成的地方。 让我们着眼于String文字中的特殊字符。
特殊字符 (Special Characters)
There are certain characters which has special effects when included in the string literals. A list of special characters in Swift listed below.
包含在字符串文字中的某些字符具有特殊效果。 下面列出了Swift中的特殊字符列表。
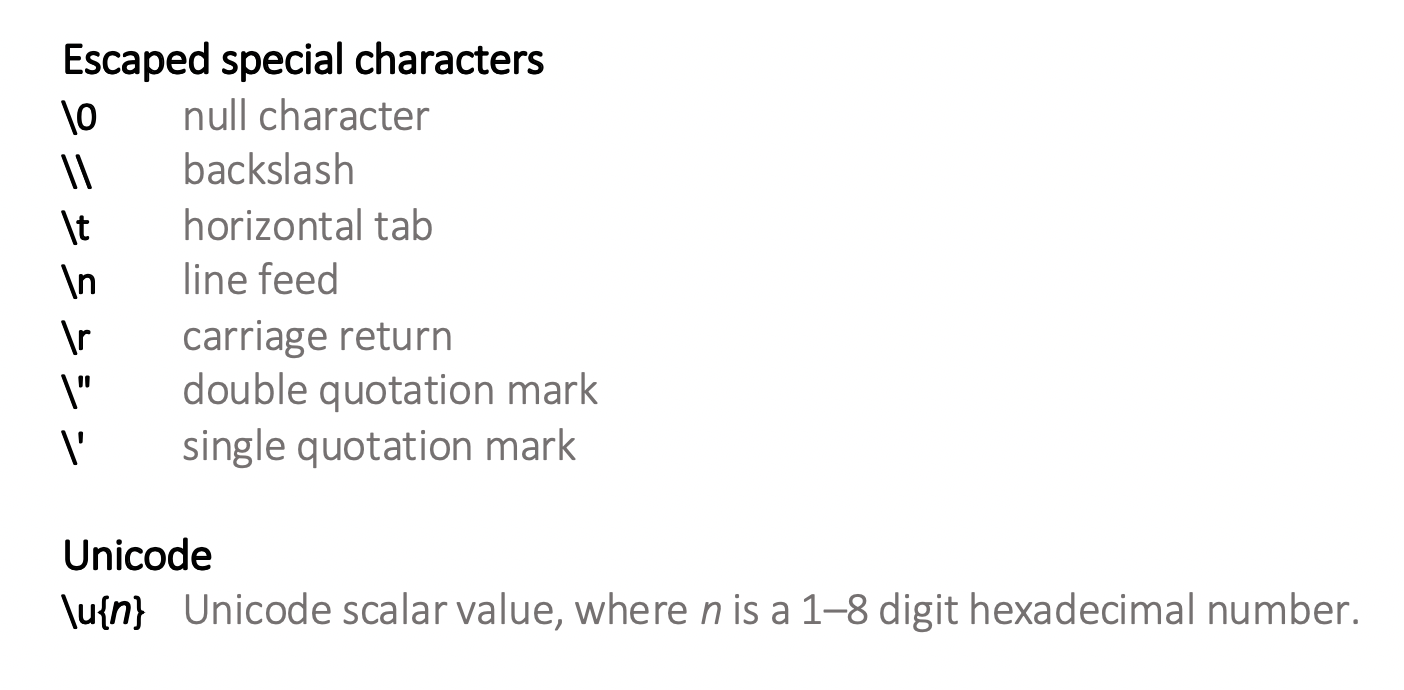
Now let’s see the effects of these special characters when included in the string literals. This is bit long example, but a minute of patience will make us gain a lot. Please go through this.
现在,让我们看看包含在字符串文字中的这些特殊字符的效果。 这是一个很长的例子,但是一分钟的耐心会让我们受益匪浅。 请通过这个。
//ESCAPED SPECIAL CHARACTERS
/*
\0 - Null Character do not occupie any space.
There is no space between two pipes in below string
even though there is a null character in between those two pipes.
*/
let nullCharString = "This string contains a null character between next two pipes |\0|"
print("\(nullCharString)")
//----------------------------------------------------------------
//This string contains a null character between next two pipes ||
//----------------------------------------------------------------
/*
\0 - Another example with 4 null characters.
But none are printed or occupied any space.
*/
let multipleNullCharString = "This string contains 4 null character between next two pipes |\0\0\0\0|"
print("\(multipleNullCharString)")
//----------------------------------------------------------------
//This string contains 4 null character between next two pipes ||
//----------------------------------------------------------------
/*
Backslash: \\ - It is normally used as escape sequence in the string literals.
So in order to print backslash itself, a more backslash is prefixed as escape sequence.
*/
let backSlashString = "This string has a backslash \\ here."
print(backSlashString)
//----------------------------------------------------------------
//This string has a backslash \ here.
//----------------------------------------------------------------
/*
Horizontal tab: \t - It adds the space of a tab whereever it is used in string.
*/
let hozTabString = "There is space of horizontal tab between two cats here 🐱\t🐱"
print("\(hozTabString)")
//----------------------------------------------------------------
//There is space of horizontal tab between two cats here 🐱 🐱
//----------------------------------------------------------------
/*
Line Feed: \n - It moves the cursor to the next new line.
*/
let lineFeedString = "Hello!\nHere is an example of text that uses \nline feed special character."
print("\(lineFeedString)")
//----------------------------------------------------------------
//Hello!
//Here is an example of text that uses
//line feed special character.
//----------------------------------------------------------------
/*
Carraige Return: \r - It moves the cursor to the beginning of the new line.
*/
let carriageReturnString = "Hello!\rHere is an example of text that uses \rcarraige return special character."
print("\(carriageReturnString)")
//----------------------------------------------------------------
//Hello!
//Here is an example of text that uses
//carraige return special character.
//----------------------------------------------------------------
/*
Double Quote: \" - It is normally denotes the string literals.
So a backslash is prefixed to include the double quote as part of the string.
*/
let doubleQuoteString = "\"This is a double quoted string.\""
print("\(doubleQuoteString)")
//----------------------------------------------------------------
//"This is a double quoted string."
//----------------------------------------------------------------
/*
Single quote: \' - It in swift do not have any special significane
but it is included as special character as per Apple's Swift document.
So a backslash is prefixed to include the double quote as part of the string.
It can be ignored and included in string without backslash.
*/
let singleQuoteString = "This is a \'single quoted\' string."
print("\(singleQuoteString)")
//----------------------------------------------------------------
//This is a 'single quoted' string.
//----------------------------------------------------------------
/*
Single quote: \' - Single quote can be printed without the backslash escape sequence.
*/
let anotherSingleQuoteStr = "This is another 'single quoted' string without escape sequence."
print("\(anotherSingleQuoteStr)")
//----------------------------------------------------------------
//This is another 'single quoted' string without escape sequence.
//----------------------------------------------------------------
//UNICODE
/*
Below string represents few animals using Unicode.
*/
let animalsString = " Monkey \u{1F412} \n Dog \u{1F415} \n Tiger \u{1F405}"
print("\(animalsString)")
//----------------------------------------------------------------
// Monkey 🐒
// Dog 🐕
// Tiger 🐅
//----------------------------------------------------------------
As promised, let’s see how a backslash is included in the string literals. Have a look at the Line 31, that explain everything itself.
按照承诺,让我们看看字符串文字中如何包含反斜杠。 看一下Line 31,它解释了所有内容。
Now the next obvious question is, what if we want to remove the effect of these special characters when included in the string literals? Use Extended String Delimiters for this.
现在,下一个明显的问题是,如果要删除包含在字符串文字中的这些特殊字符的影响,该怎么办? 为此使用扩展字符串定界符。
扩展字符串定界符 (Extended String Delimiters)
You can place a string literal within extended delimiters to include special characters in a string without invoking their special effects. For this you have to place the string within quotation marks and surround that with # sign. Let see the examples how to achieve this.
您可以在扩展定界符中放置字符串文字,以在字符串中包含特殊字符,而无需调用其特殊效果。 为此,您必须将字符串放在引号内,并用#号将其引起来。 让我们看示例如何实现这一点。
let oneDelimiterString = #"This string has a line feed here \n wihch has no effect."#
print("\(oneDelimiterString)")
//----------------------------------------------------------------
//This string has a line feed here \n wihch has no effect.
//----------------------------------------------------------------
let twoDelimiterString = ##"This string has a line feed here \n wihch has no effect."##
print("\(twoDelimiterString)")
//----------------------------------------------------------------
//This string has a line feed here \n wihch has no effect.
//----------------------------------------------------------------
let multiDelimiterString = ###"This string has a line feed here \n wihch has no effect."###
print("\(multiDelimiterString)")
//----------------------------------------------------------------
//This string has a line feed here \n wihch has no effect.
//----------------------------------------------------------------
You can see in above example that we have placed the # sign at the start and the end of the string. And this has removed the effect of \n in the line. As a result the line didn’t break.
您可以在上面的示例中看到,我们在字符串的开头和结尾放置了#号。 这就消除了行中\ n的影响。 结果,线路没有中断。
One other thing to be noticed is different strings has different number of delimiter sign, #. Yes, You can use any number of delimiter sign (#), but the number of delimiter sign should always be same at the start and the end of the string. It may be one, two, three, multiple in numbers.
需要注意的另一件事是不同的字符串具有不同数量的定界符#。 是的,您可以使用任意数量的定界符(#),但是定界符的数量在字符串的开头和结尾应始终相同。 它可以是一,二,三,倍数。
You can use any number of delimiter sign (#), but the number of delimiter sign should always be same at the start and the end of the string.
您可以使用任意数量的定界符(#),但是定界符的数量在字符串的开头和结尾应始终相同。
What happen if we have multiple special characters in a string literals, but we just want to remove the effect for few of them not all? In this case use extended delimiters for the string and add the delimiters in between the special character for which you want to have the effects!
如果我们在字符串文字中有多个特殊字符,但是我们只想删除其中一部分(不是全部)的效果,会发生什么? 在这种情况下,请对字符串使用扩展定界符,并在要产生效果的特殊字符之间添加定界符!
let exDelimiteIgnoredrstring = ##"""
This string has a line feed here \n wihch has no effect.\##nBut this is in new line.
There are two dog here also but in below line separated by tab.\##n 🐕\##t🐕
"""##
print("\(exDelimiteIgnoredrstring)")
//----------------------------------------------------------------
//This string has a line feed here \n wihch has no effect.
//But this is in new line.
//There are two dog here also but in below line separated by tab.
// 🐕 🐕
//----------------------------------------------------------------
In above example you can see that whenever ## is used in between the \n like — \##n and in \t like — \##t, then in these cases special characters has its effects in the string literal, rather than printing as plain characters. One thing need to be remembered is that the number of # in between special characters should be same as at the start and end of the string literal.
在上面的示例中,您可以看到,只要在\ n(如\\ ## n)和\ t(如\\ ## t)之间使用##,那么在这些情况下,特殊字符会在字符串文字中产生影响,而不是在打印为普通字符。 需要记住的一件事是,特殊字符之间的#数目应与字符串文字的开头和结尾处的数目相同。
Special characters have effects when included in the string literals. This effect can be removed by using Extended string delimiters.
包含在字符串文字中的特殊字符会产生影响。 可以使用扩展字符串定界符来消除此影响。
That’s all for now!
目前为止就这样了!
结论 (Conclusions)
- String literal is the sequence of characters enclosed in double quote.字符串文字是用双引号引起来的字符序列。
- Multiline string literal is spanned over multiple lines, enclosed in three double quotes. 多行字符串文字跨越多行,并用三个双引号引起来。
- Multiline string literals content must begin on a new line and closing delimiter must begin on a new line. Delimiter lines are not included in the string literals operations. 多行字符串文字内容必须从新行开始,并且结束定界符必须从新行开始。 分隔符行不包括在字符串文字操作中。
- Line break can be avoided in multiline string literal using the backslash ‘\’ at the end of the lines. 可以在行尾使用反斜杠“ \”来避免在多行字符串文字中出现换行。
- Blank line in source replicates in the multiline string value also. 源中的空白行也将复制多行字符串值。
- Whitespaces before the starting of the line is included in the multiline string literal value. 多行字符串文字值中包含行开头之前的空格。
- Whitespaces before the closing delimiter of multiline string are ignored for all the other lines. But whitespaces along or after are included. 对于所有其他行,多行字符串的结束定界符之前的空格将被忽略。 但是,包括前后的空格。
- Special characters have effects when included in the string literals. This effect can be removed by using Extended string delimiters. 包含在字符串文字中的特殊字符会产生影响。 可以使用扩展字符串定界符来消除此影响。
Thank You!
谢谢!
I hope you have enjoyed going through this!
希望您喜欢这个过程!
翻译自: https://medium.com/@srideo.prasad/string-literals-in-swift-6e0e02a41e26