scala面向对象
This series is all about the taste of Scala.It is best suitable for all the newbies in Scala.
这个系列是关于Scala的口味的,最适合Scala中的所有新手。
You may also like: Scala Beginner Series (1) : Basics
您可能还会喜欢: Scala入门系列(1):基础知识
In the previous part, we covered:
在上一部分中 ,我们介绍了:
- Values, variables, expressions and types 值,变量,表达式和类型
- Functions and recursion 函数与递归
- The Unit type 单位类型
This article will cover the object oriented nature of Scala language.
本文将介绍Scala语言的面向对象性质。
班级 (Classes)
Scala has the same concept of a class as we have in other languages.
Scala具有与其他语言相同的类概念。
Classes in Scala are blueprints for creating objects. They can contain methods, values, variables, objects, traits and super-classes which are collectively called members. Fields and methods are accessed with the dot operator similar to the other languages.
Scala中的类是用于创建对象的蓝图。 它们可以包含方法,值,变量,对象,特征和超类,它们统称为成员。 与其他语言类似,使用点运算符访问字段和方法。
Also, all fields and methods of classes are public by default, unless we restrict their visibility with the private
and protected
modifiers. We don't have to specify public
access modifier explicitly.
另外,默认情况下,所有类的字段和方法都是公共的,除非我们使用private
和protected
修饰符限制它们的可见性。 我们不必明确指定public
访问修饰符。

A minimal class definition is simply the keyword class
and an identifier. The keyword new
is used to create an instance of the class.
最小的类定义只是关键字class
和标识符。 关键字new
用于创建类的实例。

建设者 (Constructors)
Classes in Scala can take arguments — those are the constructor arguments. When we define a class, we can also define its constructor signature.
Scala中的类可以接受参数-这些是构造函数参数。 当定义一个类时,我们还可以定义其构造函数签名。

Beware that constructor arguments are not class fields . Parameters without val
or var
are private values, visible only within the class. Thus the following code will generate error:
注意构造函数参数 不是类字段。 不带val
或var
参数是私有值,仅在类中可见。 因此,以下代码将产生错误:

Thus in order to promote constructor arguments to class fields, we can put a val
or var
before the argument name.
因此,为了将构造函数参数提升为类字段,我们可以在参数名称前放置val
或var
。

遗产 (Inheritance)
When a class inherits from another, it means it extends another. This lets a class inherit members from the one it extends and provides the code re-usability.
当一个类从另一个继承时,意味着它扩展了另一个。 这使类可以从其扩展的类继承成员,并提供代码可重用性。
To carry out Scala inheritance, we use the keyword extends
:
为了进行Scala继承,我们使用关键字extends
:

In the above example of two classes, Person
class is base class or super-class and Employee
class is derived class or sub-class.
在上面两个类的示例中, Person
类是基类或超类,而Employee
类是派生类或子类。
extends
clause has two effects:
extends
子句具有两个作用:
It makes
Employee
class inherit all non-private members ofPerson
class它使
Employee
类继承了Person
类的所有非私有成员It makes the type
Employee
a subtype of the typePerson
.它使
Employee
类型成为Person
类型的子类型。
Scala also allows only single class inheritance just like Java.
与Java一样,Scala也仅允许单类继承。
Scala has the same subtype polymorphism that we have seen in other statically typed object oriented languages. In subtype polymorphism, instance of subclass can be passed to a base class.
Scala具有与其他静态类型的面向对象语言相同的子类型多态性。 在子类型多态中,子类的实例可以传递给基类。

At compile time, compiler only knows that we are calling demo()
method of Element
object. But at run time, the most derived method i.e. demo()
method of ArrayElement
is called. A derived class can, of course, override their super class methods.
在编译时,编译器仅知道我们正在调用Element
对象的demo()
方法。 但是在运行时,将调用派生程度最高的方法,即ArrayElement
demo()
方法。 派生类当然可以覆盖其超类方法。
抽象类 (Abstract Classes)
Scala also has a concept of an abstract class that is similar to Java’s abstract class, which contains both abstract and non-abstract methods and cannot support multiple inheritances. They can’t be instantiated as well.
Scala还具有一个抽象类的概念,该概念类似于Java的抽象类,该类包含抽象方法和非抽象方法,并且不支持多个继承。 它们也无法实例化。
To implement Scala abstract class, we use the keyword abstract
against its declaration:
为了实现Scala抽象类,我们针对其声明使用关键字abstract
:
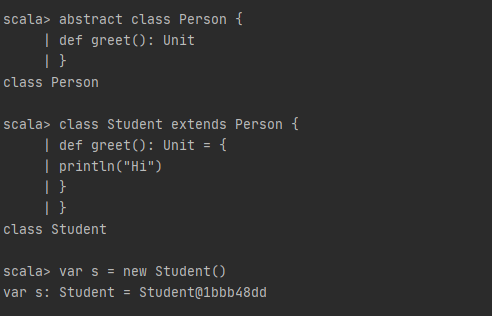
It is also mandatory for a child to implement all abstract methods of the parent class. Abstract methods of abstract class are those methods which do not contain any implementation.
子项也必须实现父类的所有抽象方法。 抽象类的抽象方法是那些不包含任何实现的方法。
特质 (Traits)
Traits in Scala are similar to Java’s Interfaces. Classes and objects can extend traits, but traits cannot be instantiated and therefore have no parameters.
Scala中的特性类似于Java的Interfaces。 类和对象可以扩展特征,但是特征不能被实例化,因此没有参数。
Traits are like partially implemented interfaces. It may contain abstract and non-abstract methods. It is possible that all methods are abstract, but it should have at least one abstract method.
特性就像部分实现的接口。 它可能包含抽象和非抽象方法。 可能所有方法都是抽象的,但它至少应具有一个抽象方法。
Classes which extend the traits have to implement the abstract methods of the trait, but need not to implement the concrete methods of the trait.
扩展特征的类必须实现特征的抽象方法,但不必实现特征的具体方法。
To define trait, we use the trait
keyword:
要定义特征,我们使用trait
关键字:

Scala has single-class inheritance and multi-trait mixing. It is possible to extend any number of Scala traits with a class or with an abstract class.
Scala具有单类继承和多特征混合。 一个类或一个抽象类可以扩展任意数量的Scala特征。

匿名类 (Anonymous Classes)
Scala also has the concept of anonymous classes much like Java.
Scala还具有类似于Java的匿名类的概念。
We already saw how to implement parent class’s declared methods. A less formal way to provide the implementation for a parent class’s methods is with an anonymous class, a non-reusable and nameless class definition.
我们已经看到了如何实现父类的声明方法。 为父类的方法提供实现的一种不太正式的方法是使用匿名类,不可重用和无名的类定义。
To define a one-time anonymous class, instantiate the parent (and potentially abstract) class and follow the class name and parameters with curly braces containing the implementation.
要定义一次性匿名类,请实例化父类(并可能是抽象类),并使用包含实现的花括号跟随类名和参数。

The result is an instance that does extend the given parent class with a one-time implementation, but can be used like an instance from a traditional class definition.
结果是一个实例确实通过一次性实现扩展了给定的父类,但可以像传统类定义中的实例一样使用。
To the compiler, anonymous class looks like this:
对于编译器,匿名类如下所示:

With anonymous classes, the compiler does a lot of work behind the scenes. This allows us to abstract that complexity away from our code.
对于匿名类,编译器在后台进行了大量工作。 这使我们可以从代码中抽象出这种复杂性。
单例对象 (Singleton Objects)
There is no idea of static
in Scala, instead we have singleton objects.
Scala中没有static
概念,相反,我们有单例对象。
An object is a class that has exactly one instance. It is created lazily when it is referenced, like a lazy val. The methods and fields declared inside singleton object are globally accessible, we don’t need an object to access them. We can import them from anywhere in the program. Also, we can’t pass parameters to its primary constructor.
对象是仅具有一个实例的类。 它在引用时是惰性创建的,例如惰性val。 在单例对象中声明的方法和字段可全局访问,我们不需要对象即可访问它们。 我们可以从程序中的任何位置导入它们。 另外,我们不能将参数传递给它的主要构造函数。
A singleton object is declared using the object
keyword:
使用object
关键字声明单例对象:

A singleton object can extend classes and traits. It also provides an entry for program execution. Without such an object, the code compiles but produces no output.
单例对象可以扩展类和特征。 它还提供了用于程序执行的条目。 没有这样的对象,代码将编译但不产生任何输出。
伴侣对象 (Companion Objects)
When a singleton object is named the same as a class, it is called a companion object, and the class is called companion class. The companion class-object pair has to be in a single source file. Either member of the pair can access its companion’s private members. We use a companion object for methods and values which are not specific to instances of the companion class.
当单例对象的名称与类相同时,它称为伴随对象,而该类称为伴随类。 配套的类-对象对必须位于单个源文件中。 该对中的任何一个成员都可以访问其同伴的私人成员。 我们将伴随对象用于并非特定于伴随类实例的方法和值。

I have entered :paste
mode here because I am working in the REPL
. Since companions need to be in same source file, so :paste
mode in REPL
allows companions to be defined together.
我在这里进入:paste
模式,因为我正在REPL
工作。 由于伴随文件必须位于同一源文件中,因此REPL
:paste
模式允许一起定义伴随文件。
static
members in Java are modeled as ordinary members of a companion object in Scala.
Java中的static
成员在Scala中被建模为伴随对象的普通成员。
申请方法 (The Apply Method)
Scala classes as well as Scala objects, both offer a default method that we name as the apply
method. We sometimes call it an injector method. We can also invoke this method without a name. Let me show you what I mean:
Scala类以及Scala对象都提供了一个默认方法,我们将其命名为apply
方法。 我们有时将其称为注入器方法。 我们也可以不带名称地调用此方法。 让我告诉你我的意思:

Remember, apply
is just a special function that lets us call the parent object directly, like a function. It has nothing to do with object orientation, classes, or constructors in the slightest.
记住, apply
是一个特殊的函数,它使我们可以像函数一样直接调用父对象。 它与面向对象,类或构造函数无关。
We can use apply in companion objects as factory methods. The idea of the factory method is to construct class instances without new
.
我们可以在伙伴对象中使用apply作为工厂方法。 factory方法的想法是在没有new
情况下构造类实例。

案例分类 (Case Classes)
Scala case classes are like regular classes with a few key differences which we will go over. When the compiler sees the case
keyword in front of a class
, it generates code for us, with the following benefits:
Scala案例类就像常规类一样,有一些关键的区别,我们将继续介绍。 当编译器在class
前面看到case
关键字时,它将为我们生成代码,具有以下好处:
- Scala case classes are immutable by default and decomposable through pattern matching. 默认情况下,Scala case类是不可变的,可通过模式匹配将其分解。
It does not use
new
keyword to instantiate object. This is because case classes have anapply
method by default which takes care of object construction.它不使用
new
关键字实例化对象。 这是因为案例类默认情况下具有一个apply
方法,该方法负责对象的构造。

An
unapply
method is generated, which lets us to use case classes in more ways inmatch
expressions.一个
unapply
生成的方法,它可以让我们在以多种方式使用case类match
的表达式。A default
toString
method is generated, which is helpful for debugging.生成默认的
toString
方法,这对调试很有帮助。equals
andhashCode
methods are generated, which let us compare objects and easily use them as keys in maps. It is used to check the value equality of all individual member fields instead of just checking the reference equality of the objects.会生成
equals
和hashCode
方法,这使我们可以比较对象并将它们轻松用作地图中的键。 它用于检查所有单个成员字段的值相等性,而不仅仅是检查对象的引用相等性。

We can create a shallow copy of an instance of a case class simply by using the
copy
method. We can also change the constructor arguments.我们可以简单地使用
copy
方法创建案例类实例的浅表副本。 我们还可以更改构造函数参数。

All the parameters listed in the case class are public and
val
by default. It is possible to usevar
in case classes but this is discouraged.默认情况下,案例类中列出的所有参数均为public和
val
。 在案例类中可以使用var
,但是不建议这样做。

案例对象 (Case objects)
A case object
is like an object
, but just like a case class has more features than a regular class, a case object has more features than a regular object. Its features include:
case object
就像一个object
,但是就像案例类具有比常规类更多的功能一样,案例对象具有比常规对象更多的功能。 其功能包括:
- It’s serializable 可序列化
It has a default
hashCode
implementation它具有默认的
hashCode
实现It has an improved
toString
implementation它具有改进的
toString
实现
The biggest advantage of case classes and case objects is that they support pattern matching. Pattern matching is a major feature of functional programming languages, and Scala’s case classes and objects provide a simple way to implement pattern matching in match expressions and other areas.
案例类和案例对象的最大优点是它们支持模式匹配。 模式匹配是功能编程语言的主要功能,Scala的案例类和对象提供了一种在匹配表达式和其他区域中实现模式匹配的简单方法。
You may also like: Pattern Match Anything in Scala
您可能还会喜欢: 模式匹配Scala中的所有内容
敬请关注… (Stay Tuned…)
Stay tuned for our next part of Scala Beginner Series where we will cover the functional nature of Scala. You don’t want to miss it!
请继续关注我们的Scala入门系列下一部分,我们将介绍Scala的功能本质。 你不想错过它!
翻译自: https://levelup.gitconnected.com/scala-beginner-series-2-object-oriented-scala-4e2496ec2e9f
scala面向对象