程序员过度用脑会脑部疾病吗
I still remember when I landed my first job out of university, how amazing and overwhelming it felt. I was eager to absorb every bit of knowledge and be the best at my craft but soon realized that ‘perfect’ code does not exist.
我仍然记得当我从大学毕业后才找到第一份工作时,感觉多么令人惊奇和压倒性。 我渴望吸收所有知识,并尽我所能,但很快意识到“完美”的代码不存在。
I started noticing myself stuck for hours, sometimes days in a situation that I refer to as ‘code paralysis’. If you are an over-thinker like myself, you may have even come across a similar situation where you found yourself stuck analyzing the situations and its parameters rather than acting upon it; its referred to as ‘analysis paralysis’.
我开始注意到自己被困了几个小时,有时甚至是几天,在我称之为“代码瘫痪”的情况下。 如果您像我一样过于思想,那么您甚至可能遇到过类似的情况,您发现自己陷于分析情况及其参数,而不是对之采取行动; 它称为“分析瘫痪”。
Code paralysis is a situation of impaired productivity due to overthinking in the context of software development.
由于软件开发过程中的过度思考,代码瘫痪是生产力下降的情况。
问题 (The problem)
Code paralysis is a common phenomenon that can occur over a wide range of decision-making situations; they can range from big architectural decisions, to simply writing a small conditional statement.
代码瘫痪是一种常见现象,可能会在广泛的决策情况下发生; 它们的范围从大型的体系结构决策到简单地编写小的条件语句。
Let us consider the following task: you are provided with an array of Account
and asked to write a function that set the state
of all Account
with a negative balance to 'inactive'
and return an array of the updated ones.
让我们考虑以下任务:向您提供一个Account
数组,并要求您编写一个函数,该函数将负余额的所有Account
的state
设置为'inactive'
并返回一个更新的数组。
class Account {
constructor (balance, state) {
this.balance = balance;
this.state = state;
}
}
A possible solution that you may come up with is:
您可能想出的一种可能的解决方案是:
function deactivateAccounts (accounts) {
const updatedAccounts = [];
accounts.forEach(account => {
if (account.balance < 0) {
account.state = 'inactive';
updatedAccounts.push(account);
}
});
return updatedAccounts;
}
It works as intended but how about we filter
the array first and then update the Account
to the proper state
:
它可以按预期工作,但是我们如何首先filter
数组,然后将Account
更新到正确的state
:
function deactivateAccounts (accounts) {
return accounts
.filter(account => account.balance < 0)
.map(account => {
account.state = 'inactive';
return account;
});
}
But wait, why are we iterating twice over when we can reduce
it instead:
但是,等等,为什么我们可以reduce
迭代次数呢?
function deactivateAccounts (accounts) {
return accounts.reduce((accumulator, account) => {
if (account.balance < 0) {
account.state = 'inactive';
accumulator.push(account);
}
return accumulator;
}, []);
}
Looking great! But what if the function is called with an array of Account
consisting of million entries? In this situation, we are far better off using a native for
loop…
看起来很棒! 但是,如果用包含数百万个条目的Account
数组调用该函数怎么办? 在这种情况下,我们最好使用本机的for
循环…
Though this may be a very simple task, it can escalate very quickly in an overthinker’s mind. The art of writing code is never black and white, and the correctness of the implementation varies on multiple factors such as:
尽管这可能是一个非常简单的任务,但在思想者的脑海中它可以Swift升级。 编写代码的技巧从来都不是黑白的,并且实现的正确性取决于多种因素,例如:
- Codebase patterns 代码库模式
- Language-specific idioms 语言特定的习语
- The balance between readability and performance 可读性与性能之间的平衡
解决方案” (The “solution”)
Alright, so how do we deal with it?
好吧,那么我们该如何处理呢?
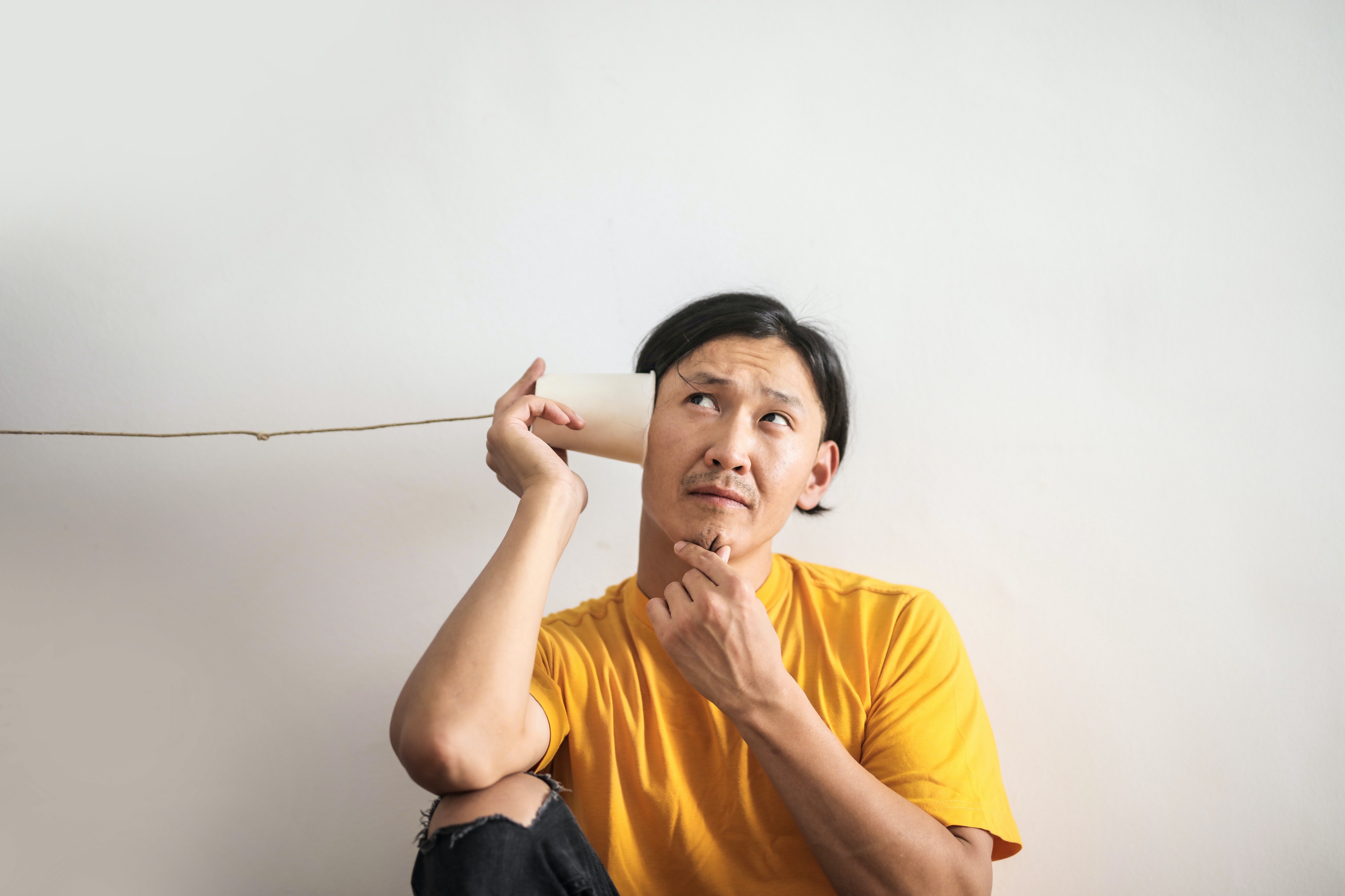
The bad news is that there is no way around it. It still is a constant struggle for me to this day. But I have developed a simple framework to help me deal with the situation as soon as I catch myself stuck in this mental loop. I tried to keep it keep as simple as possible or I know that I will never stick to it, here we go:
坏消息是没有办法解决。 直到今天,对我而言,这仍然是不断的斗争。 但是,我开发了一个简单的框架,可以帮助我在陷入这种心理循环时立即处理这种情况。 我试图使其保持尽可能的简单,否则我将永远坚持下去,那就开始吧:
1.有一个明确的目标 (1. Have a clear goal)
Oftentimes we don’t know how to build a feature or service because we don’t know what needs to be built in the first place. Be sure you have all the requirements and that you fully understand them. This is also a good opportunity to put on your ‘user hat’ and ask yourself:
通常,我们不知道如何构建功能或服务,因为我们一开始就不知道需要构建什么。 确保您具有所有要求,并且您完全了解它们。 这也是戴上“用户帽子”并问自己的好机会:
- Does the solution solves the problem and satisfies the user requirements that were initially imposed? 解决方案是否解决了问题并满足了最初提出的用户要求?
- Is this the most impactful solution given the time constraints? 鉴于时间限制,这是否是最具影响力的解决方案?
2.使它工作,无论如何! (2. Make it work, no matter what!)
No seriously, pretend that no one is ever going to read your code and write out whatever comes to mind to make it as functional as possible. As soon as the test cases pass, or the minimum functionality works, stop and walk away from your laptop!
没错,假装没有人会阅读您的代码并写出想出的任何东西来使其尽可能发挥作用。 一旦测试用例通过或最低功能正常运行,请停止并离开笔记本电脑!
3.开始重构 (3. Start refactoring)
This is the hardest step in my opinion and code refactoring deserves an entire article, or book, or even an entire library on its own. Here is my general thought process when tackling the refactoring process represented as a state diagram:
在我看来,这是最难的一步,代码重构值得一整篇文章或一本书,甚至是一个完整的库。 这是我处理以状态图表示的重构过程时的一般思考过程:
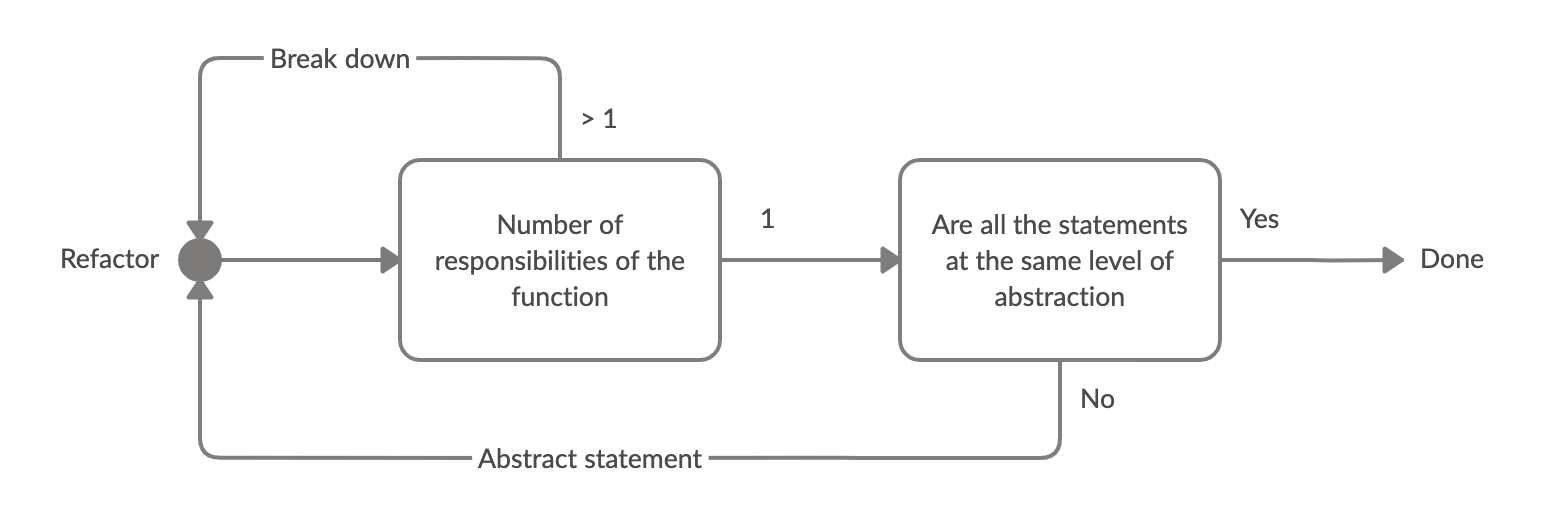
Note that this is not a “one size fits all” scenario, but rather a good entry point if you are not sure of where to start.
请注意,这不是“一刀切”的情况,但是如果您不确定从哪里开始,那是一个很好的切入点。
There are plenty of software design principles, but personally, the most important and practical one is the single-responsibility principle (SRP).
有很多软件设计原则,但就个人而言,最重要和最实用的原则是单一职责原则 (SRP)。
Functions should do one thing. They should do it well. They should do it only.
函数应该做一件事。 他们应该做好。 他们只能这样做。
— Uncle Bob
—鲍勃叔叔
SRP helps to keep your functions small and manageable. Now let us try to refactor the code from our previous example by navigating through the state diagram.
SRP有助于使您的功能小巧且易于管理。 现在,让我们通过浏览状态图来尝试重构先前示例中的代码。
The first step is to ask ourselves: How many responsibilities does the deactivateAccounts
function have?
第一步是自问: deactivateAccounts
函数有多少职责?
The answer is 2:
答案是2 :
Filter out
Account
with negative balances.过滤出余额为负的
Account
。Set their
state
to"inactive"
.将其
state
设置为"inactive"
。
As indicated our first course of action is to “Break down” deactivateAccounts
into two pieces as follows:
如所示,我们的第一个行动方案是“分解” deactivateAccounts
分为以下两个部分:
function filterByNegativeBalance (accounts) {
return accounts.filter(account => account.balance < 0);
}
function deactivateAccounts (accounts) {
return accounts.map(account => {
account.state = 'inactive';
return account;
});
}
Notice how deactivateAccounts
is now going to deactivate all Account
regardless of their balance
. This is intentional, as someone who might be calling deactivateAccounts
from a different module will never expect it to only deactivate negative Account
. A more descriptive and accurate name for the main function is deactivateNegativeAccounts
.
请注意, deactivateAccounts
现在将如何停用所有Account
而不管其balance
如何。 这是有意为之的,因为可能会从其他模块调用deactivateAccounts
永远不会希望它仅停用否定的Account
。 主要函数的一个更具描述性和准确性的名称是deactivateNegativeAccounts
。
function deactivateNegativeAccounts (accounts) {
const negativeAccounts = filterByNegativeBalance(accounts);
return deactivateAccounts(negativeAccounts);
}
Back to the state diagram: How many responsibilities does each function have? Now the answer is one each:
回到状态图:每个功能有多少职责? 现在答案是一个:
filterByNegativeBalance
is only filtering the accounts bybalance
.filterByNegativeBalance
仅按balance
过滤帐户。deactivateAccounts
is only updating thestate
.deactivateAccounts
仅更新state
。
Sweet! we can move on to the next step: Are all the statements at the same level of abstraction?
甜! 我们可以继续下一步:所有语句是否处于相同的抽象级别?
filterByNegativeBalance
we are simply calling agetter
onbalance
, so we are good here.filterByNegativeBalance
我们只是在balance
上调用getter
,所以我们在这里很好。deactivateAccounts
does this function really need to know how to anAccount
should be deactivated? Not really, so we should abstract thestate
update and encapsulate it in the classAccount
.deactivateAccounts
这个功能真的需要知道如何停用一个Account
吗? 并非如此,因此我们应该抽象state
更新并将其封装在Account
类中。
function deactivateAccounts (accounts) {
return accounts.map(account => account.deactivate());
}
Here is the complete refactored code:
这是完整的重构代码:
class Account {
constructor (balance, state) {
this.balance = balance;
this.state = state;
},
deactivate () {
this.state = 'inactive';
return this;
}
}
function deactivateNegativeAccounts (accounts) {
const negativeAccounts = filterByNegativeBalance(accounts);
return deactivateAccounts(negativeAccounts);
}
function filterByNegativeBalance (accounts) {
return accounts.filter(account => account.balance < 0);
}
function deactivateAccounts (accounts) {
return accounts.map(account => account.deactivate());
}
Done! As mentioned previously, refactoring code is a huge topic and if you want to learn more about it, I highly recommend “Clean Code” by Robert C. Martin.
做完了! 如前所述,重构代码是一个巨大的话题,如果您想了解更多信息,我强烈推荐Robert C. Martin的“ Clean Code”。
4.寻求反馈 (4. Ask for feedback)
Ready or not, don’t be afraid to reach out. If you have the opportunity to work in a great company, then everybody in your team, especially your manager will want you to succeed. It is in their best interest. Still unsure? Present your CL as a “draft”, they don’t need to know that it is, in fact, your finished work :)
准备好与否,不要害怕伸出手。 如果您有机会在一家出色的公司工作,那么团队中的每个人,特别是您的经理都希望您成功。 这符合他们的最大利益。 还不确定吗? 将您的CL呈现为“草稿”,他们不需要知道它实际上就是您完成的工作:)
5.重复 (5. Repeat)
Do a few iterations of step #3 and #4, the more complex the problem the more iterations it requires. But it is very important to set a hard limit on the number of iterations and to time-box your steps to ensure that you don’t get stuck in an infinite refactoring loop. This will help bring balance between getting sh*t done and pushing clean and readable code.
对步骤3和#4进行几次迭代,问题越复杂,则需要进行的迭代越多。 但是,对迭代次数设置硬性限制并设置步骤时间以确保您不会陷入无限重构循环中,这一点非常重要。 这将有助于在完成与推送干净且可读的代码之间取得平衡。
仅此而已! (And that is all!)
One more thing, go for a 5-min walk at least once in the process. I mean it, get up and walk away from your desk! There is some biology involved here, walking increases blood flow to the brain and allows your mind to reset and come back with fresh perspectives.
还有一件事,在此过程中至少步行5分钟。 我是认真的,站起来离开办公桌! 此处涉及一些生物学,步行会增加流向大脑的血液,并使您的思想重新设置并以崭新的视角返回。
This little framework has helped me tremendously against code paralysis throughout my career and still is, and I hope it does to you as well. Thanks for reading!
这个小框架在我的整个职业生涯中一直为我提供了极大的帮助,以防止代码瘫痪,现在仍然如此,我希望它对你也有帮助。 谢谢阅读!
翻译自: https://levelup.gitconnected.com/how-to-deal-with-overthinking-as-a-programmer-5e8b53c08742
程序员过度用脑会脑部疾病吗