继承映射
When generating a database using JPA, one of the most important things we have to do is mapping inheritances. In this post, you are going to learn some basic things that we have to know when mapping inheritance.
使用JPA生成数据库时,我们要做的最重要的事情之一就是映射继承。 在本文中,您将学习映射继承时必须了解的一些基本知识。
For the ScholarX project, I had to add JPA annotations to the spring-boot project in order to generate the MySQL database. The models had already been created for the entities. To initiate the task, I was given a class diagram. Otherwise, it might be so hard to find data types, relationships, etc. Click here if you wanna find out what a class diagram is.
对于ScholarX项目,我必须将JPA批注添加到spring-boot项目中,以便生成MySQL数据库。 已经为实体创建了模型。 为了启动任务,给了我一个类图。 否则,可能很难找到数据类型,关系等。如果您想了解类图是什么,请单击此处 。
Okay then… back to the topic. JPA has several inheritance strategies.
好吧……回到主题。 JPA有几种继承策略。
MappedSuperclass
映射超类
- Single Table 单桌
- Joined Table 联接表
- Table-Per-Class 每类表
If you have checked our class diagram you may notice that we have a base modal called “BaseScholarxModel” for all entities. And there’s another parent called “EnrolledUser” extended by the base modal. We don’t need tables for these two models. Therefore I had to make use of those different strategies mentioned above.
如果您检查了我们的类图,您可能会注意到我们为所有实体提供了一个名为“ BaseScholarxModel”的基本模式。 基本模态扩展了另一个父级“ EnrolledUser”。 这两个模型我们不需要表格。 因此,我不得不利用上述那些不同的策略。
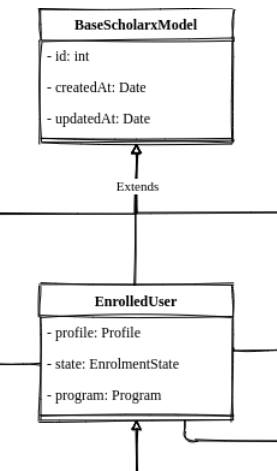
映射超类 (MappedSuperclass)
This is one of the easiest inheritance strategies. We only have to add @MappedSuperclass
annotation to above the class. But keep in mind that we don't use the @Entity
annotation here as we don't need a separate table for the base model. However, we can only have one @MappedSuperclass
. In my case, you can see that there are two parent classes which should not have tables in the hierarchy. But we can use this annotation for the topmost parent.
这是最简单的继承策略之一。 我们只需要在类上方添加@MappedSuperclass
批注。 但请记住,我们在这里不使用@Entity
批注,因为我们不需要为基础模型提供单独的表。 但是,我们只能有一个@MappedSuperclass
。 就我而言,您可以看到有两个父类在层次结构中不应该包含表。 但是我们可以将此注释用于最高父级。
MappedSuperclass inheritance
映射超类继承
(In “AcadeMiX” which was one of our other projects, we had only one base model. So, there we could use only the MappedSuperclass strategy)
(在我们的其他项目之一“ AcadeMiX”中,我们只有一个基本模型。因此,我们只能使用MappedSuperclass策略)
每类表 (Table-Per-Class)
This is very similar to the previous strategy. But this strategy defines entities for parent classes also. However, it doesn’t generate tables for parent classes. And it creates separate tables for each model extended by the parent. To use this, we just have to put @Inheritance(strategy = InheritanceType.TABLE_PER_CLASS)
above the class to define the inheritance type.
这与以前的策略非常相似。 但是这种策略也为父类定义了实体。 但是,它不会为父类生成表。 并且为父级扩展的每个模型创建单独的表。 要使用此功能,我们只需要在类上方放置@Inheritance(strategy = InheritanceType.TABLE_PER_CLASS)
即可定义继承类型。
This was the perfect solution for my case.
这是我的案例的完美解决方案。
Table-per-class inheritance strategy
每类表继承策略
So far we have talked about two inheritance strategies. Two more to go on. I’ll briefly explain those.
到目前为止,我们已经讨论了两种继承策略。 还有两个要继续。 我将简要解释这些。
单桌 (Single Table)
This is the default inheritance strategy. So if you hope to use this, you have to put just @Inheritance
annotation above the class. or you can define the strategy as SINGLE_TABLE. This creates a single table for each class hierarchy. To differentiate between objects, a discriminator value column is generated. It can be customized also. In my opinion, this strategy makes things complicated.
这是默认的继承策略。 因此,如果您希望使用它,则只需在类上方放置@Inheritance
批注。 或者您可以将策略定义为SINGLE_TABLE。 这将为每个类层次结构创建一个表。 为了区分对象,将生成一个鉴别值列。 也可以定制。 我认为,这种策略使事情变得复杂。
@Entity@Inheritance(strategy = InheritanceType.SINGLE_TABLE)
public class Parent {private long id;
// getters and setters
}
联接表 (Joined Table)
In this strategy, a table is created for the parent object. and Child Tables are created with a foreign key to the parent. so we have to join tables if we want to get an entity. You can define this kind of inheritance as shown below.
在此策略中,将为父对象创建一个表。 和子表是使用父级的外键创建的。 因此,如果要获取实体,则必须联接表。 您可以如下定义此类继承。
Parent class:
家长班:
@Entity@Inheritance(strategy = InheritanceType.JOINED)
public class Vehicle{
private long id;// getters and setters
}
Child class:
子班:
@Entity@PrimaryKeyJoinColumn(name = “busId”)
public class Bus extends Vehicle{
// code
}
So I have briefly described JPA(Hibernate) inheritance strategies. See my pull request on this task by clicking here. Contribute to SEF. If you are new to programming we have a lot of good first issues in our GitHub repos (Especially in sef-site). Just put a comment there if you like to work on an issue.
因此,我已经简要描述了JPA(Hibernate)继承策略。 单击此处,查看我对此任务的请求。 为SEF做贡献。 如果您是编程新手,我们的GitHub存储库(尤其是在sef-site中 )有很多很好的第一期问题。 如果您想解决某个问题,只需在此处发表评论即可。
And… That’s all for today. Hope you have learned something new. Or maybe you have found a solution for something which you were struggling with. Thanks for reading!! Bye!
而且...今天就这些。 希望你学到了新东西。 或者,也许您找到了自己正在苦苦挣扎的解决方案。 谢谢阅读!! 再见!
翻译自: https://levelup.gitconnected.com/inheritance-mapping-with-jpa-hibernate-338f0c0b8450
继承映射