本文涵盖的主题 (Topics Covered in This Article)
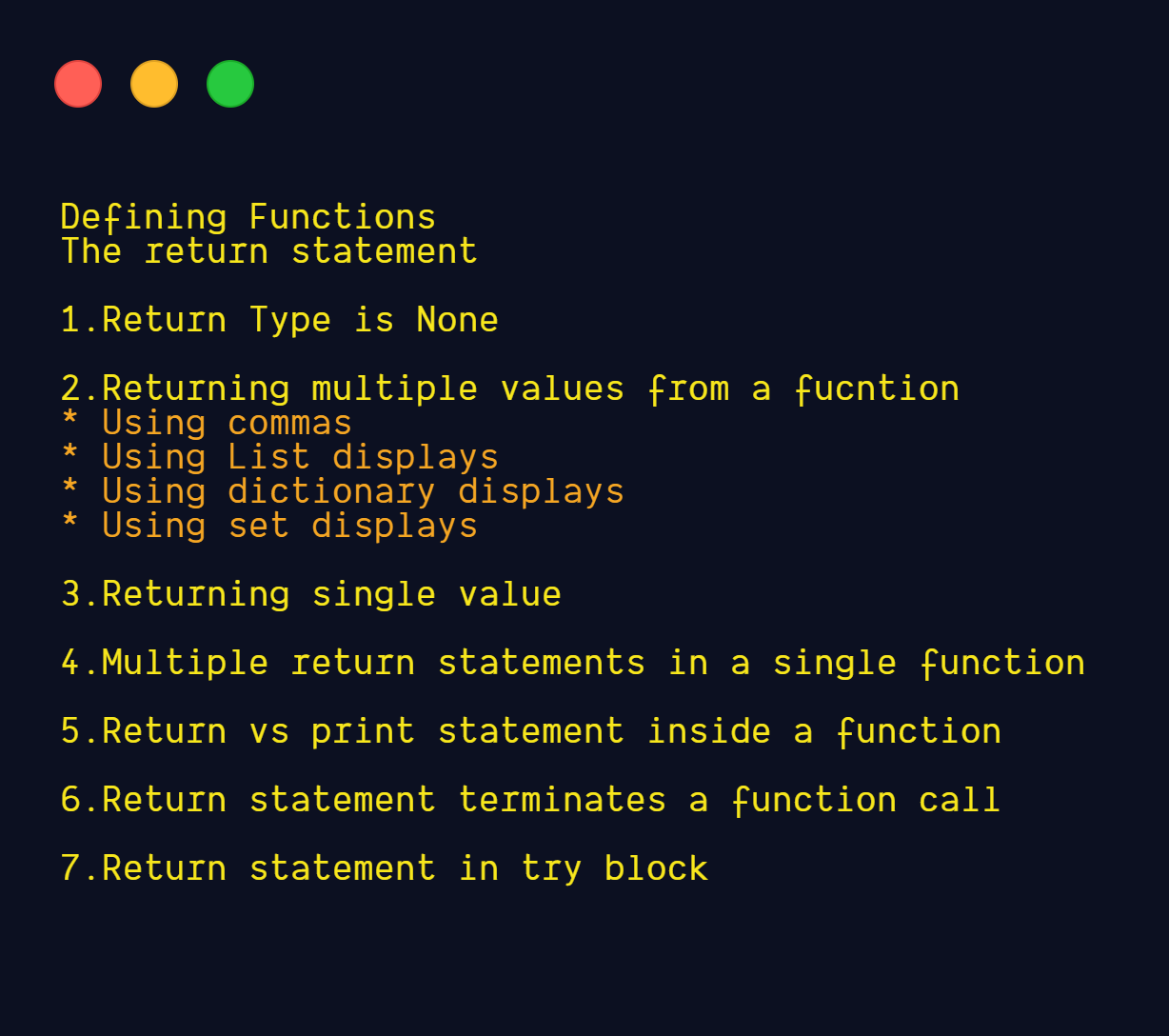
定义功能 (Defining Functions)
Function definition starts with keyword def
followed by the function name and a parenthesized list of formal parameters. The statements that form the body of the function start at the next line and must be indented.
函数定义以关键字def
开头,后跟函数名称和带括号的形式参数列表。 构成函数主体的语句从下一行开始,并且必须缩进。
def fname(parameters):
statement(s)
The function may contain return
or yield
statements.If a function has a yield
statement, it is known as a generator function.
该函数可能包含return
或yield
语句。如果一个函数有一个 yield
语句,称为生成器函数 。
“退货”声明 (The ‘return’ Statement)
The return
statement terminates the function call and returns a value to the caller.
的 return
语句终止函数调用并向调用方返回一个值。
The return
statement without an expression argument returns None
, and a function without a return
statement also returns None
.
没有表达式参数的return
语句返回None
,而没有return
语句的函数也返回 None
。
Syntax:
句法:
"return" [expresion_list]
1.返回类型为None (1. Return Type Is None)
Example 1:
范例1:
If there is no return
statement inside the functions, it returns None
.
如果函数内部没有return
语句,则返回None
。
def add():passprint (add())#Output:None
Example 2:
范例2:
If the return
statement is not reached in the function, it returns None
.Here, in this example, the condition c>10
is not satisfied, so it returns None
.
如果未在函数中return
语句,则返回None
。在此示例中,不满足条件c>10
,因此返回None
。
def add(a,b):
c=a+bif c>10:return c
print (add(1,2))#Output:None
Example 3:
范例3:
def add(a,b):
c=a+b
print (add(1,2))#Output:None
2.从函数返回多个值 (2. Returning multiple values from a function)
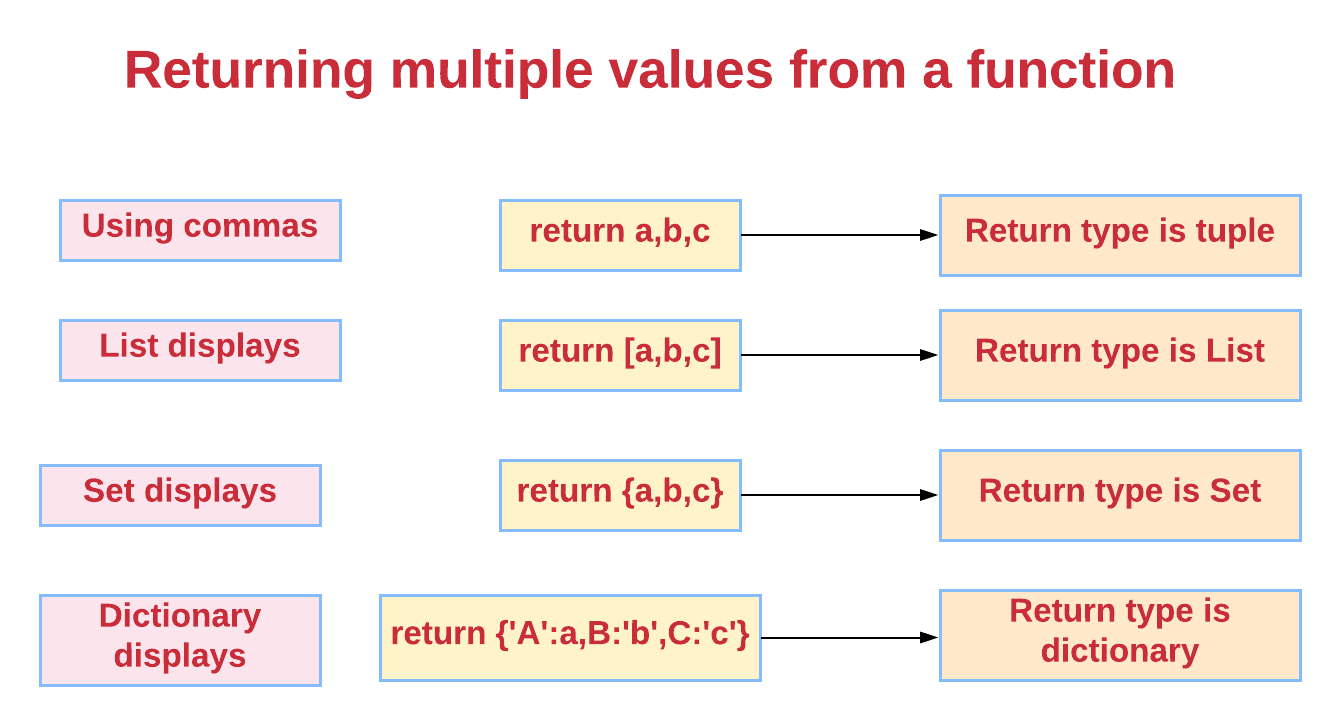
Returning multiple values using commas
使用逗号返回多个值
If the expression list in the return
statement contains at least one comma, except when part of a list or set display, it returns a tuple. The length of the tuple is the number of expressions in the list. The expressions are evaluated from left to right.
如果return
语句中的表达式列表包含至少一个逗号(除非是列表或集合显示的一部分),则它返回一个元组。 元组的长度是列表中表达式的数量。 表达式从左到右计算。
The return type is a tuple.
返回类型是一个元组。
def add(a,b):
result=a+breturn a,b,result
print (add(1,2))#Output:(1,2,3)
The return
statement having a single expression with a trailing comma returns a tuple.
具有单个表达式和后缀逗号的return
语句返回一个元组。
def add(a,b):
result=a+breturn result,
print (add(1,2))#Output:(3,)
Returning multiple values using list displays
使用列表显示返回多个值
A list display yields a new list object, the contents being specified by a list of expressions. When a comma-separated list of expressions is supplied, its elements are evaluated from left to right and placed into the list object in that order. The return type is a list.
列表显示将产生一个新的列表对象,其内容由表达式列表指定。 提供逗号分隔的表达式列表时,将按从左到右的顺序评估其元素并将其按顺序放入列表对象中。 返回类型是一个列表。
def calc(a,b):
sum=a+b
dif=a-b
mul=a*b
div=a/breturn [sum,dif,mul,div]
print (calc(5,4))#Output:[9, 1, 20, 1.25]
Returning multiple values using dictionary displays
使用字典显示返回多个值
A dictionary display yields a new dictionary object. When a comma-separated sequence of expressions in the key/value pairs is supplied, each key object is used as a key into the dictionary to store the corresponding value.Return type is a dictionary.
词典显示将产生一个新的词典对象。 提供键/值对中的逗号分隔的表达式序列时,每个键对象都用作字典中的键以存储相应的值。 返回类型是字典。
def calc(a,b):
sum=a+b
dif=a-b
mul=a*b
div=a/breturn {"Addition":sum,"Subtraction":dif,"Multiplication":mul,"Division":div}
print (calc(5,4))#Output:{'Addition': 9, 'Subtraction': 1, 'Multiplication': 20, 'Division': 1.25}
Returning multiple values using set displays
使用设置显示返回多个值
A set display yields a new mutable set object, the contents being specified by either a sequence of expressions or a comprehension. When a comma-separated list of expressions is supplied, its elements are evaluated from left to right and added to the set object. The return type is a set.
集合显示将产生一个新的可变集合对象,其内容由一系列表达式或理解来指定。 提供逗号分隔的表达式列表时,将从左到右评估其元素并将其添加到set对象中。 返回类型是一个集合。
def calc(a,b):
sum=a+b
dif=a-b
mul=a*b
div=a/breturn {sum,dif,mul,div}
print (calc(5,4))#Output:{9, 20, 1, 1.25}
3.从函数返回单个值 (3. Returning a single value from a function)
The return statement having a single expression without a trailing comma doesn’t create a tuple; it returns the value of that expression.
只有一个表达式且不带逗号的return语句不会创建元组。 它返回该表达式的值。
def add(a,b):
result=a+breturn result
print (add(1,2))#Output:3print (add("Hello ","Python"))#Output:Hello Pythonprint (add([1,2],[3,4]))#Output:[1, 2, 3, 4]print (add((1,2),(3,4)))#Output:(1, 2, 3, 4)
4.单个函数中有多个“ return”语句 (4. Multiple ‘return’ statements in a single function)
The function call is terminated when one of the return statements is reached.
当到达返回语句之一时,函数调用终止。
def num(a):if a%2==0:return "Even Number"
else:return "Odd Number"print (num(5))#Output:Odd Numberprint (num(30))#Output:Even Number
5.函数中的“ return”和“ print”语句 (5. ‘return’ vs. ‘print’ statements inside a function)
The return
statement will terminate the function call and return the value to the caller.
return
语句将终止函数调用,并将值返回给调用方。
The print
statement will just print the value. We can’t assign that result to another variable or pass the result to another function.
的 print
语句将只显示该值。 我们不能将该结果分配给另一个变量或将结果传递给另一个函数。
Example: Assigning return value to a variable and also passing as an argument to another function
示例:将返回值分配给变量,并将其作为参数传递给另一个函数
def add(a,b):
r1=a+breturn r1def sub(c,d):
r2=c-dreturn r2#We can assign the return value to a variabler3=add(3,4)
print (r3)#Output:7#We can pass the return value to another function also.print (sub(r3,5))#Output:2
Example: Functions with the print
statement
示例:带有 print
语句的 函数
def add(a,b):
r1=a+b
print (r1)add(3,4) #Output:7
#We can't assign the return value to a variabler3=add(3,4)
print (r3)#Output:None
6.'return'语句终止函数调用 (6. ‘return’ statement terminates the function call)
The return
statement terminates the current function call with the expression list (or None
) as the return
value. The statements after the return
statement inside the function are not executed.
return
语句以表达式列表(或None
)作为return
值终止当前函数调用。 函数内部return
语句之后的语句不执行。
def add(a,b):
result=a+breturn result
print ("After return statement")
print (add(3,4) )#Output:7
In the above example, the return
statement will terminate the function call, so the print
statement is not executed.
在上面的示例中, return
语句将终止函数调用,因此不会执行print
语句。
def add(a,b):
result=a+b
print (result)
print ("Addition is done")
add(3,4)'''
Output:
7
Addition is done
'''
In the above example, both the print
statements are executed.
在上面的示例中,两个print
语句均被执行。
7.在“ try”块中的“ return”语句 (7. ’return’ statement in ‘try’ block)
“When
return
passes control out of atry
statement with afinally
clause, thatfinally
clause is executed before really leaving the function.” — Python documentation“当
return
将控制从带有finally
子句的try
语句中移出时,将在真正离开函数之前执行finally
子句。” — Python文档
Example: In the below example, the function call will return after executing the finally
clause.
示例:在下面的示例中,函数调用将在执行 finally
子句 后返回 。
def add(a,b):try:
result=a+breturn resultexcept Exception as e:return efinally:
print ("Finally done")
print (add(3,4))'''
Output:
Finally done
7
'''
If an exception is raised, the function call will return after executing the finally
clause.
如果引发异常,则函数将在执行finally
子句后返回。
def add(a,b):try:
result=a+breturn resultexcept Exception as e:return efinally:
print ("Finally done")
print (add({'a':1},{'b':2}))'''
Output:
Finally done
unsupported operand type(s) for +: 'dict' and 'dict'
'''
结论 (Conclusion)
The
return
statement occurs only inside a function definition.return
语句仅在函数定义内出现。The
return
statement will terminate the function call and returns the value.return
语句将终止函数调用并返回值。The
return
statement can return multiple values also. Thereturn
value can be used as an argument to another function also.return
语句也可以返回多个值。return
值也可以用作另一个函数的参数。If the
return
statement is not in the function or if it is not reached, it will returnNone
.如果
return
语句不在函数中或未到达,则将返回None
。The function can have multiple
return
statements also. The function call is terminated when one of thereturn
statements is reached.该函数还可以具有多个
return
语句。 当到达return
语句之一时,函数调用终止。
I hope that you have found this article helpful. Thanks for reading!
希望本文对您有所帮助。 谢谢阅读!