Shell Script is a program designed to run in the Unix/Linux shell. We can write a particular set of commands as a script and run it to perform some tasks.
Shell Script是一个旨在在Unix / Linux shell中运行的程序 。 我们可以将一组特定的命令作为脚本编写并运行以执行一些任务。
We can run Unix/Linux command on Windows, Mac using a Bash (Bash is a command-line interpreter or a shell) like Git Bash or any other program. We can ls
to list content in a directory, touch
to create files.
我们可以在Windows,Mac上使用Gash Bash之类的Bash(Bash是命令行解释器或Shell)运行Unix / Linux命令。 我们可以ls
列出目录中的内容, touch
创建文件。
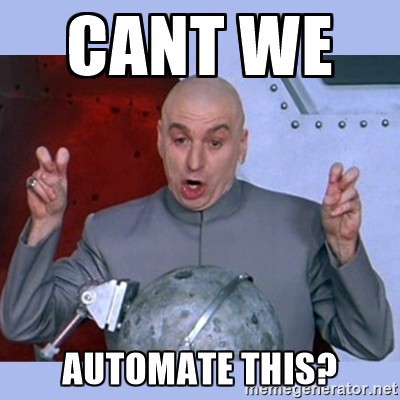
So, let’s create a file with .sh extension to write some script and open it with your favorite text editor. Also, open up the terminal or Git Bash if you are using windows.
因此,让我们创建一个扩展名为.sh的文件以编写一些脚本,然后使用您喜欢的文本编辑器将其打开。 另外,如果使用Windows,请打开终端或Git Bash。
First, we have to make our script executable. Unless if we run it, we’ll get permission denied error. To do that run chmod +x testscript.sh
command in the terminal. By this command, we change the mode to executable (we give the permission to execute).
首先,我们必须使脚本可执行。 除非我们运行它,否则会出现权限拒绝错误。 为此,请在终端中运行chmod +x testscript.sh
命令。 通过此命令,我们将模式更改为可执行文件(我们授予执行权限)。
To run the script, run the command ./testscript.sh
in the terminal. It will not give you any output because the file is still empty.
要运行脚本, ./testscript.sh
在终端中运行命令./testscript.sh
。 因为文件仍然为空,它不会提供任何输出。
In our script (testscript.sh), first we have to declare the dialect, I’m using Bash. In the terminal run the command which bash
to find the location of bash in your computer. In the top of your script type #! <BASH_LOCATION>
to declare the bash.
在脚本(testscript.sh)中,首先我们必须声明方言,我正在使用Bash。 在终端中,运行命令which bash
来查找bash在计算机中的位置。 在脚本顶部,输入#! <BASH_LOCATION>
#! <BASH_LOCATION>
声明bash。
Now let’s write a simple script as below,
现在让我们编写一个如下的简单脚本,
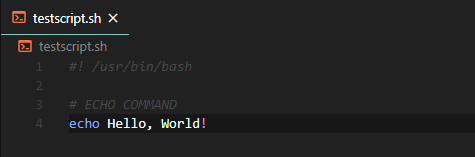
In line 1, I have declared the Bash. Line 3, it’s a comment. Line 4, echo
command will display “Hello, World!” in terminal.
在第1行中,我声明了Bash。 第3行,这是一条评论。 第4行, echo
命令将显示“ Hello,World!” 在终端。
Now run the script by ./testscript.sh
in Git Bash or terminal and you’ll see the output.
现在,在Git Bash或终端中通过./testscript.sh
运行脚本,您将看到输出。
So, let’s move on. I’m going to explain the basic stuff in Shell Scripts using the following gist,
因此,让我们继续前进。 我将使用以下要点解释Shell脚本中的基本内容,
#! /usr/bin/bash
# ECHO COMMAND
echo Hello, World!
# VARIABLES
NAME="Osusara"
echo "My name is $NAME"
# INPUTS
read -p "Enter a number: " NUMBER
echo "Your number is $NUMBER"
# CONDITIONALs
if [ "$NUMBER" == 1 ]
then
echo "Number is 1"
elif [ "$NUMBER" == 2 ]
then
echo "Number is 2"
else
echo "Number is not 1 or 2"
fi
# CASE STATEMENTs
read -p "Are you a human? Y/n: " ANSWER
case "$ANSWER" in
[yY] | [yY][eE][sS])
echo "You are not an Alien"
;;
[nN] | [nN][oO])
echo "You are not a human"
;;
*)
echo "Please enter y or n"
;;
esac
# LOOPS
# FOR LOOP
NUMBERS="12 31 25 16"
for NUMBER in $NUMBERS
do
echo "Number $NUMBER"
done
# WHILE LOOP
NUMBER=1
while [ $NUMBER -lt 10 ]
do
echo "Number is $NUMBER"
((NUMBER++))
done
# FUNCTIONS
function hello() {
echo "Hello, World!"
}
hello
function myAge() {
echo "I'm $1 and I'm $2 years old"
}
myAge "Osusara" "24"
# CREATE FOLDER AND WRITE TO A FILE
mkdir hello
touch "hello/world.txt"
echo "Hello, World!" >> "hello/world.txt"
echo "Created hello/world.txt"
变数 (Variables)
In shell scripts, we can declare a variable and assign a value as shown in the example code.
在shell脚本中,我们可以声明一个变量并分配一个值,如示例代码所示。
输入项 (Inputs)
In the code example, it takes user input and assigns it to variable NUMBER. Then echo it. -p
means prompt.
在代码示例中,它接受用户输入并将其分配给变量NUMBER。 然后回声它。 -p
表示提示。
条件语句 (Conditional Statements)
These are similar to if-else statements in other programming languages. You can understand the syntax from the code example. If statements must end with fi
in scripts (backward of if).
这些类似于其他编程语言中的if-else语句。 您可以从代码示例中了解语法。 在脚本中,if语句必须以fi
结尾(if的后向)。
Also, we can use comparisons for conditions as below.
另外,我们可以对以下条件进行比较。
X -eq Y (true if X and Y are equal)
X -ne Y (true if X and Y are not equal)
X -gt Y (true if X is grater than Y)
X -gt Y (true if X is grater than or equal Y)
X -lt Y (true if X is less than Y)
X -lt Y (true if X is less than or equal Y)# EXAMPLE
X=2
Y=1
if [ $X -gt $Y ]
then
echo "$X is greater than $Y"
fi
There is another type of condition. It is File Conditions.
还有另一种情况。 这是文件条件。
-d file (true if the provided string is a directory)
-e file (true if the file is exists)
-f file (true if the provided string is a file)
-g file (true if the group id is set on a file)
-r file (true if the file is readable)
-s file (true if the file has a non-zero size)
-u (true if the user id is set on a file)
-w (true if the file is writable)
-x (true if the file is an executable)# EXAMPLE
FILE="test.txt"
if [ -f "$FILE" ]
then
echo "$FILE is a file"
else
echo "$FILE is not a file"
fi
案例陈述 (Case Statements)
In the script What I have created is just a simple case statement with user input. First, read the user input then according to that echo a text. This is similar to Switch Case in other programming languages.
在脚本中,我创建的只是带有用户输入的简单case语句。 首先,读取用户输入,然后根据该回显文本。 这类似于其他编程语言中的Switch Case。
[yY] | [yY][eE][sS])
this line checks if the user input either “y” or “Y” or either “yes” or “YES”. ;;
is similar to break where mark the end of the statement. *)
is the default statement that executes if the input doesn’t match with any case. And case statements must end with esac
(backward of case).
[yY] | [yY][eE][sS])
[yY] | [yY][eE][sS])
此行检查用户输入的是“ y”还是“ Y”,还是“ yes”或“ YES”。 ;;
类似于在语句结束处标记break 。 *)
是输入与任何大小写都不匹配时执行的默认语句。 并且case语句必须以esac
结尾(大小写落后)。
循环 (Loops)
First, one is a simple for loop, which loops through the numbers and echo the.
首先,一个简单的for循环会循环遍历数字并回显。
The second example is a while loop, which runs while the NUMBER is less than 10. ((NUMBER++))
increments the value of NUMBER one by one.
第二个示例是while循环,它在NUMBER小于10时运行。 ((NUMBER++))
逐一递增NUMBER的值。
功能 (Functions)
In the code example, I have written two functions. The first one is a simple function. Very similar to functions in other programming languages.
在代码示例中,我编写了两个函数。 第一个是简单的功能。 与其他编程语言中的功能非常相似。
The second function has parameters. We don’t declare them in brackets like other languages. In shell scripts, we use positional parameters. As in the example. We declare the parameters inside the function as $1, $2 … and when we call the function we should assign values for each parameter as myAge “Osusara” “24”
in the example.
第二个函数具有参数。 我们不会像其他语言一样在方括号中声明它们。 在shell脚本中,我们使用位置参数。 就像在此样品中。 我们在函数内将参数声明为$ 1,$ 2…,并且在调用函数时,应在示例中为每个参数指定值myAge “Osusara” “24”
。
创建文件夹并写入文件 (Create folder and write to a file)
As in the code, we can write shell commands. To create folders, files, write to files, etc.
就像在代码中一样,我们可以编写shell命令。 创建文件夹,文件,写入文件等。
So, that’s it for this article. Hope this will help you to get the basic idea of shell scripts.
因此,本文仅此而已。 希望这将帮助您了解shell脚本的基本概念。
Happy Coding Folks 👽
快乐的编码民间 👽
翻译自: https://medium.com/@osusarakammalawatta/shell-scripting-quick-beginners-guide-d973f3389e22