django视图函数
If you have only used function-based generic views in your Django project so far and find out that they are limited, you should think class-based view as a fresh approach to extending those limitations in more complex projects.
如果到目前为止,您仅在Django项目中使用了基于函数的通用视图,并且发现它们是有限的,则应将基于类的视图视为在更复杂的项目中扩展这些限制的一种新方法。
Class-based view
基于类的视图
Class-based view is an alternate way of function-based view. In the beginning, there was only function-based view in Django: “Django passed your function an HttpRequest and expected back an HttpResponse.”
基于类的视图是基于函数的视图的另一种方式。 最初,Django中只有一个基于函数的视图:“ Django将函数传递给HttpRequest并期望返回HttpResponse。”
Function-based views are obviously useful for simple applications but there is no way to customize or extend them so developers needed an alternative approach.
基于函数的视图显然对简单的应用程序有用,但是无法自定义或扩展它们,因此开发人员需要一种替代方法。
The reason class-based view is way more flexible and extendible than function-based views is Django uses mixins and toolkit of base classes to build class-based views.
基于类的视图比基于函数的视图更加灵活和可扩展的原因是Django使用混合类和基类的工具包来构建基于类的视图。
Using class-based views can seem like more complex because same thing can be achieved by function-based method more easily. But on the other hand, without them more advanced designs would be limited.
使用基于类的视图似乎更加复杂,因为通过基于函数的方法可以更轻松地实现相同的事情。 但是另一方面,没有它们,更高级的设计将受到限制。
Implementation of class-based view:
实现基于类的视图:
Example of function-based views:
基于函数的视图示例:
from django.http import HttpResponsedef my_view(request):if request.method == 'GET':# <view logic>return HttpResponse('result')
( 摘自文档 )
Example of class-based views:
基于类的视图的示例:
from django.http import HttpResponsefrom django.views import Viewclass MyView(View):def get(self, request):# <view logic>return HttpResponse('result')
( 摘自文档 )
Now because urls.py expecting from you a function that returns an Http response;above example would have raised an error. But class-based views have an as_view()
the method which returns a function that can be called when a request arrives for a URL pattern you have determined for a particular page.
现在,因为urls.py期望您返回一个返回Http响应的函数 ;上面的示例将引发一个错误。 但是基于类的视图具有as_view()
方法,该方法返回一个函数 ,当到达针对您为特定页面确定的URL模式的请求时,可以调用该函数 。
response = MyView.as_view()(request)
after setup()
to initialize its attributes anddispatch()
to determine whether request method is GET or POST, it returns an HTTP response. But this cycle is more like backstage play. Let’s move on
在setup()
初始化其属性并且dispatch()
确定请求方法是GET还是POST之后,它返回一个HTTP响应。 但是这个周期更像是后台播放。 让我们继续
In order to set this up, in urls.py should be:
为了进行设置,在urls.py中应该是:
urlpatterns = [
path('index', MyView.as_view(),name="index"),
]
instead of:
代替:
urlpatterns = [
path('index', views.index,name="index"),
]
Since it is returning an Http response like a function-based view, it is possible to use template responses in class view such as to render, redirect, HttpResponseRedirect and so on.
由于它像基于函数的视图一样返回Http响应,因此可以在类视图中使用模板响应,例如渲染,重定向,HttpResponseRedirect等。
类属性 (Class Attributes)
There are two ways to set class attributes;
有两种设置类属性的方法:
- Python way of subclassing Python的子类化方式
class StartingView(View):
startwith = "Hello, World!"def get(self, request):return HttpResponse(self.startwith)
2. Setting class attributes as a keyword to the as_view() function in urls.py
2.在urls.py中将类属性设置为as_view()函数的关键字
urlpatterns = [
path('about/', StartingView.as_view(startwith="Hello,World!")),
]
Second way is a one-time configuration while in the subclassing method, attributes can be set multiple times.
第二种方法是一次性配置,而在子类化方法中,可以多次设置属性。
- In first way; you can also decorate your view in urls.py like this: 第一种方式 您还可以像这样在urls.py中装饰视图:
urlpatterns = [
path('index/', login_required(TemplateView.as_view(template_name="secret.html"))),
path('vote/', permission_required('polls.can_vote')(VoteView.as_view())),
]
Conclusion
结论
I have tried to explain the usage of class-based view however I honestly do prefer function-based views for simple cases, especially if I don’t have to repeat the same code. Here is one example:
我试图解释基于类的视图的用法,但是老实说,在简单情况下,我确实更喜欢基于函数的视图,特别是在不必重复相同代码的情况下。 这是一个例子:
Let’s say you want to add today’s date for your code. In function-based view you can simply write:
假设您要为代码添加今天的日期。 在基于函数的视图中,您可以简单地编写:
'today': date.today()
With a Class view, on the other hand, what you have to add is this:
另一方面,在“类”视图中,您需要添加的内容是:
def get_context_data(self, **kwargs):
context = super().get_context_data(**kwargs)
context['today'] = date.today()return context
As a result, class-based can be hard and complex in most of the situations wherein some situations it is extremely helpful.
结果,在大多数情况下,基于类的学习可能会非常困难和复杂,而在某些情况下它非常有用。
First of all, it helps with the DRY code principle ( Don’t Repeat Yourself) Because once you write your class view, you can use it anywhere.
首先,它有助于DRY代码原理(不要重复自己),因为一旦编写了类视图,便可以在任何地方使用它。
If you do want to integrate your code with Javascript ( or will be using Django Rest Framework) then you will most likely use more class-based views.
如果您确实想将代码与Javascript集成在一起(或将使用Django Rest Framework),则很可能会使用更多基于类的视图。
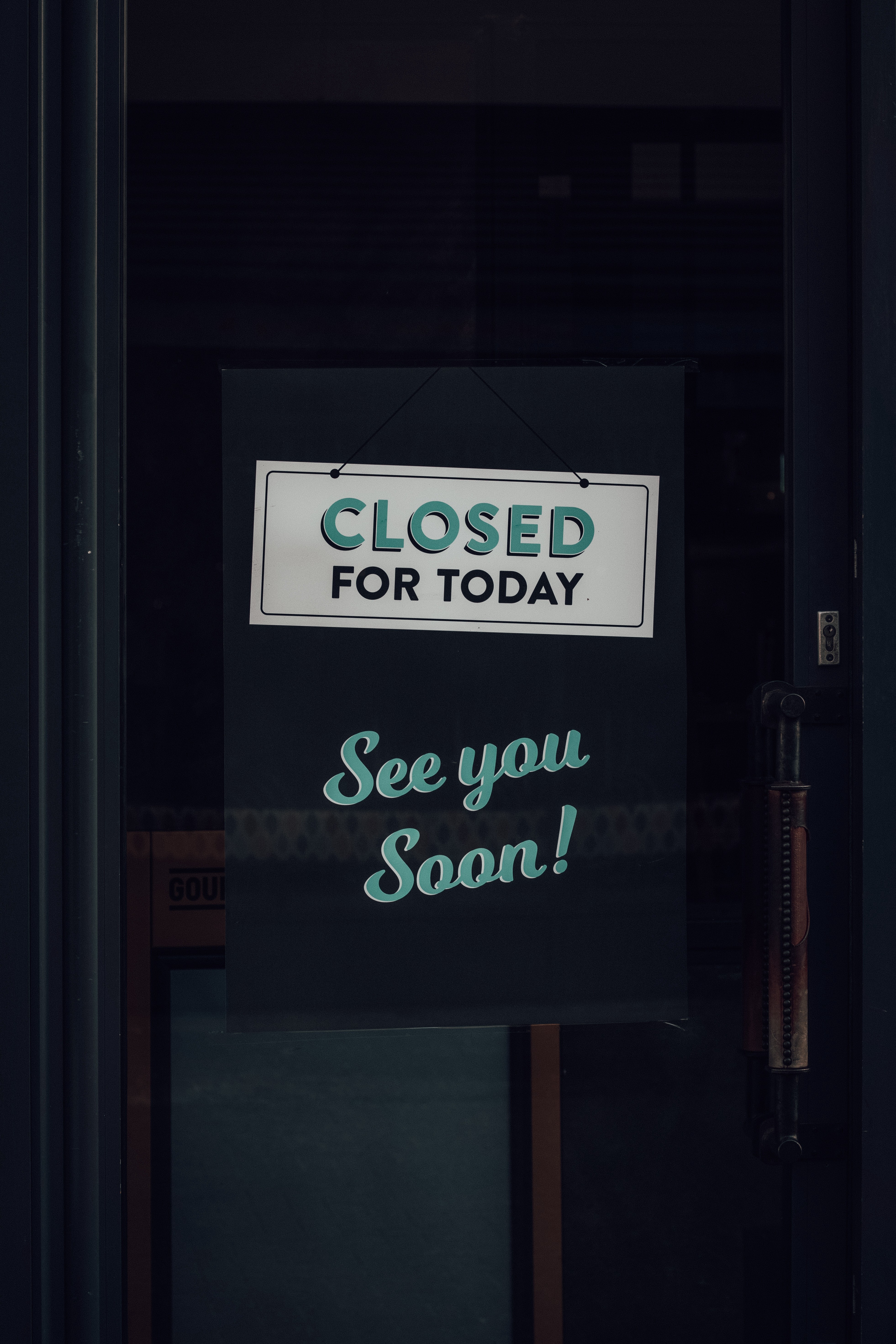
django视图函数