模糊图像恢复内容
I was watching a video by Grant Sanderson (aka 3Blue1Brown) about convolutions, image processing, and the Julia programming language (awesome language!) when someone asked this question.
当有人问这个问题时,我正在看格randint·桑德森(Grant Sanderson,又名3Blue1Brown)的一段有关卷积,图像处理和Julia编程语言(真棒语言!)的视频。
He answered (correctly) that he didn’t think so, because we’d be losing information. However, I thought a little more about the question and figured that it would actually be possible if the output image had the same size as the input one! This way, the output has enough pixels/information to recover the original one.
他(正确)回答说他不这么认为,因为我们会丢失信息。 但是,我对这个问题进行了更多思考,并认为如果输出图像的大小与输入图像的大小相同,则实际上是可能的! 这样,输出具有足够的像素/信息来恢复原始像素/信息。
First, I should start by explaining what a convolution is and how it can be used to blur an image. If you want a thorough explanation, I suggest the video above, but if you want a quick one, I got you. So, a convolution is a mathematical operation that, when applied to images, can be seen as a filter applied to it.
首先,我应该首先解释什么是卷积以及如何使用卷积来模糊图像。 如果您想进行详尽的解释,建议您使用上面的视频,但是如果您想获得一个快速的解释,我可以帮助您。 因此,卷积是一种数学运算,当应用于图像时,可以看作是应用于其的过滤器。
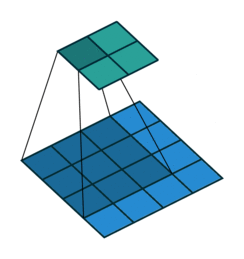
In this animation, we can see an example of a convolution of an image with a filter/kernel. The original image would be the blue matrix, the kernel the sliding dark blue matrix, and the output the greenish-blue matrix.
在此动画中,我们可以看到图像与滤镜/内核卷积的示例。 原始图像将是蓝色矩阵,内核将是滑动的深蓝色矩阵,输出将是蓝绿色矩阵。
The convolution is obtained by multiplying the overlapping kernel and image and summing the products. The following equations may help: given an image x and a kernel k, the result of the convolution will be y,
卷积是通过将重叠的核与图像相乘并对其乘积求和而获得的。 以下等式可能会有所帮助:给定图像x和内核k ,卷积的结果将为y ,
![convolution(x[4, 4], k[3, 3]) = y[2, 2]](https://img-blog.csdnimg.cn/img_convert/370e9cae40c98d574b022e6419eda043.png)
with
与

If you already knew how convolutions on images worked, maybe this system of equations isn’t too scary. If you didn’t, don’t worry, you won’t have to remember it, that’s the program job! A useful representation is to interpret the convolution as a matrix multiplication, which isn’t too difficult to write from the equations above:
如果您已经知道图像上的卷积是如何工作的,也许这个方程组并不太吓人。 如果没有,不用担心,您将不必记住它,这是程序的工作! 一个有用的表示形式是将卷积解释为矩阵乘法,从上面的等式可以很容易地写出:
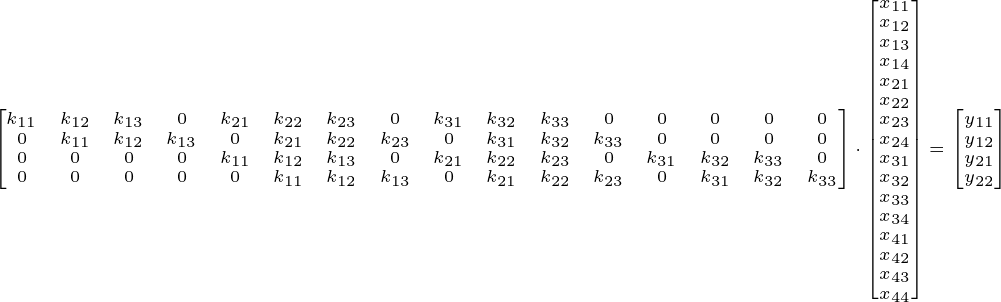
equivalent to the matrix equation
等效于矩阵方程
![A[4 x 16] x[16 x 1] = y[4 x 1]](https://img-blog.csdnimg.cn/img_convert/f7c399a5095ff30e85560118b08d51dd.png)
With this representation, it seems that knowing A and y, then x could be calculated by solving the equation above. However, since A has more columns than rows, the system is said to be undetermined, which means that we can’t obtain only one solution.
通过这种表示,似乎知道A和y ,则x可以通过求解上面的公式来计算。 但是,由于A的列多于行,因此该系统尚未确定,这意味着我们不能仅获得一个解决方案。
I started by saying that, to be possible to reverse a convolution, the input and output sizes would have to be the same. In matrix form, this would correspond to A being square (same number of rows and columns), allowing us to invert it and calculate x as
我首先说,要想反转卷积,输入和输出大小必须相同。 在矩阵形式中,这将对应于A为正方形(行和列的数目相同),从而我们可以将其求逆并将x计算为
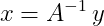
Now, our input is 4x4 and the output is 2x2. How can we obtain an output with the same size as the input? One way would be to add a padding to the input image, for example, 0-padding,
现在,我们的输入为4x4,输出为2x2。 我们如何获得与输入大小相同的输出? 一种方法是在输入图像中添加填充,例如0填充,
![convolution(x[4, 4], k[3, 3] with 0-padding of 1) = y[3, 3]](https://img-blog.csdnimg.cn/img_convert/5fa87f29c32eaa30980b14c128c3cc5a.png)
This way, the output would be 4x4 like the original input. In detail, for this simple case of a convolution with padding, the output dimensions can be calculated as,
这样,输出将像原始输入一样为4x4。 详细地说,对于这种带有填充的卷积的简单情况,可以将输出尺寸计算为:

If we want the input and output to have the same size, then the padding has to be,
如果我们希望输入和输出具有相同的大小,则填充必须为

which yields an important condition: the kernel size has to be odd since the padding is an integer value.
这产生了一个重要条件:内核大小必须为奇数,因为填充是整数值。
This convolution can also be represented as a matrix multiplication as the one above, but I’ll spare you the boredom of reading it since the dimensions would be much larger. One would write the equations of the convolution associated with each entry of y and then structure it as a matrix multiplication as above.
这种卷积也可以表示为上述矩阵的乘积,但是我会不厌其烦地阅读它,因为尺寸会大得多。 可以写出与y的每个项相关的卷积方程,然后将其构造为如上所述的矩阵乘法。
![A[16 x 16] x[16 x 1] = y[16 x 1]](https://img-blog.csdnimg.cn/img_convert/347f7a599403265bd7944e0bec46271d.png)
Notice that despite the padded input being 6x6, corresponding to 36 elements, only 4x4 of those elements are unique and unknown variables. Therefore, x in the equation can be only 16x1, instead of 36x1.
请注意,尽管填充的输入为6x6(对应于36个元素),但这些元素中只有4x4是唯一变量和未知变量。 因此, 等式中的x只能是16x1,而不是36x1。
To solve for x and reverse the convolution, one only needs to know A and y. To construct A, one needs to know the kernel used for the convolution and the type of padding used.
要求解x并逆转卷积,只需知道A和y。 要构造A,需要知道用于卷积的内核和所使用的填充类型。
Now, how could this be used?
现在,如何使用?
An image can be blurred by doing a convolution. For example, a Gaussian Blur is obtained by convolving an image with a kernel/filter that has a Gaussian distribution with the largest value at the center and values that sum to 1. An example would be the kernel
通过卷积可以使图像模糊。 例如,高斯模糊是通过将图像与内核/过滤器卷积而得到的,该内核/过滤器的中心具有高斯分布,其中心处的最大值最大,其值之和为1。
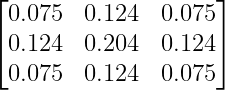
I did a simple implementation of this problem in Julia to check that it is, in fact possible, to reverse the convolution, which you can review and use at the link below.
我在Julia中对此问题进行了简单的实现,以检查实际上是否可以逆转卷积,您可以在下面的链接中进行检查和使用。
So I started by blurring an image with a Gaussian Blur. I did a convolution of the original one with a Gaussian kernel and used replicate padding (values outside the original image are set to the nearest border value, instead of 0).
因此,我首先使用高斯模糊对图像进行模糊处理。 我用高斯内核对原始图像进行了卷积,并使用了复制填充(原始图像之外的值设置为最接近的边界值,而不是0)。
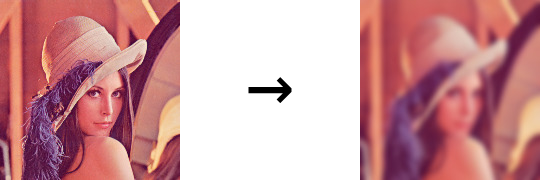
Since I knew the kernel used, I was able to construct the matrix A and then solve for x. The results are as expected: the reconstructed image is exactly equal to the original one.
因为我知道所使用的内核,所以我能够构造矩阵A ,然后求解x 。 结果符合预期:重建的图像与原始图像完全相同。
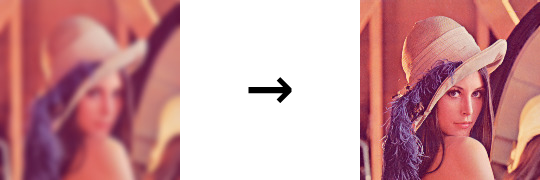
Feel free to check the implementation linked above and verify that the original image was not used in the reconstruction.
随时检查上面链接的实现,并验证重建中未使用原始图像。
Now, this 100% reconstruction is only possible because the kernel and padding used are known. What happens if we use a kernel that is not exactly the same as the one used to blur the original image?
现在,仅因为已知使用的内核和填充,才有可能进行100%的重构。 如果我们使用的内核与用于模糊原始图像的内核不完全相同,会发生什么?
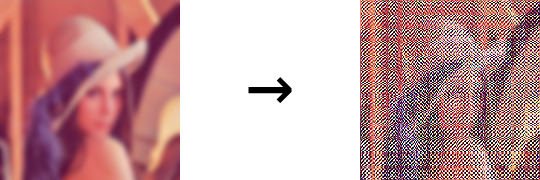
And what if we assume 0-padding when replicate padding was used?
如果假设使用重复填充时填充为0,该怎么办?
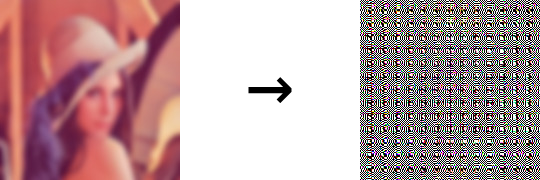
As we can see, if we don’t know the kernel and padding used, then we’re not able to reconstruct the original image. In this sense, it can almost be seen as an encryption problem: if we know the “key”, then we’re able to reconstruct the original message without any loss or additional noise.
如我们所见,如果我们不知道所使用的内核和填充,那么我们将无法重建原始图像。 从这个意义上讲,它几乎可以看作是一个加密问题:如果我们知道“密钥”,那么我们便能够重建原始消息而不会造成任何损失或额外的噪音。
Reconstructing the original image is also a very expensive task since matrix A grows very fast depending on the size of the original image. If the original image was 4x4, then A would be 16x16 — the number of elements scale with N².
重建原始图像也是一项非常昂贵的任务,因为矩阵A会根据原始图像的大小快速增长。 如果原始图像为4x4,则A将为16x16-元素数量以N²缩放。
Hope you liked this brief explanation and found it interesting. I surely did and it was a very good way to learn more about Julia, convolutions, image processing, and linear algebra. If you have any questions and/or find any error, feel free to share.
希望您喜欢这个简短的说明,并发现它很有趣。 我确实做到了,这是一种很好的了解Julia,卷积,图像处理和线性代数的好方法。 如果您有任何疑问和/或发现任何错误,请随时分享。
As a goodbye note, I think we don’t need to worry about people reversing our blurred photos, for now.
再见,我认为我们现在不必担心人们会颠倒我们模糊的照片。
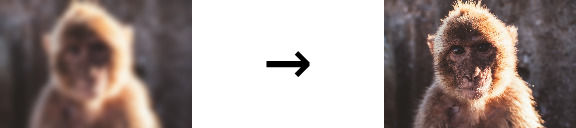
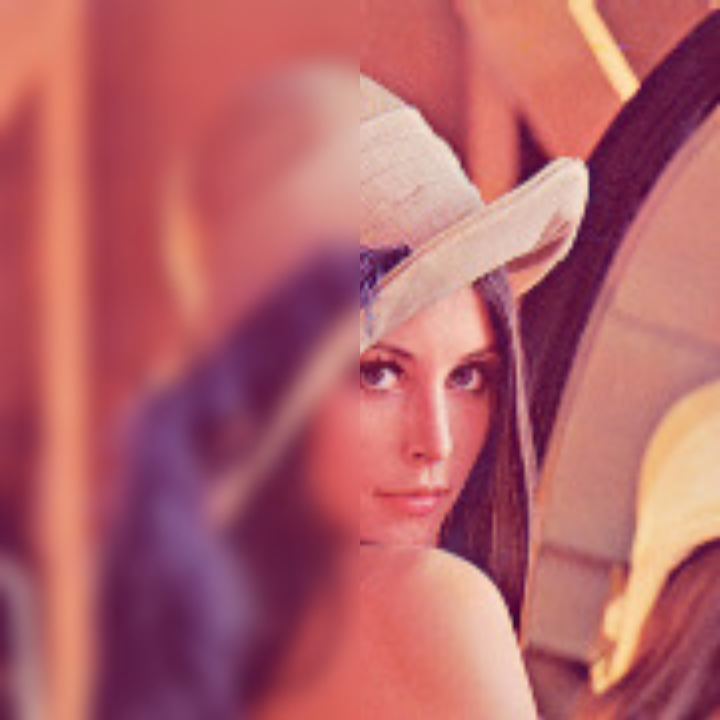
翻译自: https://medium.com/@gonced8/can-you-recover-a-blurred-image-61bbcaa969d5
模糊图像恢复内容