python 使用try
尝试/除非在python中返回(try/except with return in python)
User code can raise built-in exceptions. Python defines try/except to handle exceptions and proceed with the further execution of program without interruption.
用户代码可以引发内置异常。 Python定义了try / except来处理异常并继续执行程序而不会中断。
Let’s quickly get to an example of a basic try/except clause
让我们快速来看一个基本的try / except子句的示例
try / except语句(try/except statements)
Assuming the file is unavailable, executing the below code will give the output as shown below.
假设文件不可用,执行以下代码将给出如下所示的输出。
try:
f = open("testfile.txt")
...except FileNotFoundError as e:
print(f" Error while reading file {e} ")Output:
Error while reading file [Errno 2] No such file or directory: 'testfile.txt'
In practical use cases such as connecting to a db or opening a file object, we may need to perform teardown operations such db closure/file closure irrespective of the block getting executed. So finally
is one such block which can be reserved for these operations as it gets executed always. Let’s looks at an example.
在实际使用情况下,例如连接到db或打开文件对象,我们可能需要执行拆解操作,例如db闭合/文件闭合,而与执行块无关。 因此, finally
一个是这样的块,因为它总是被执行,所以可以保留给这些操作。 让我们看一个例子。
try / except / finally语句 (try/except/finally statements)
try:
f = open("testfile.txt")
except FileNotFoundError as e:
print(f" Error while reading file {e} ")
finally:
print(" Closing the file ")
f.close()
So what could possibly go wrong here? Why should we be cautious?
那么,这里可能出什么问题了? 我们为什么要谨慎?
Well, one could easily put foot in their mouth when they use return statements with try/except/finally in Python. Let’s carefully take one step at a time to understand the usage of return statements during exception handling.
好吧,当他们在Python中使用try / except / finally的return语句时,可以轻松地站起来。 让我们一次仔细地迈出一步,以了解异常处理期间return语句的用法。
1.使用try / except返回的用法 (1. Usage of return with try/except)
def test_func():
try:
x = 10
return x
except Exception as e:
x = 20
return x
finally:
x = 30
return xprint(test_func())Output:
30
If you think the output of the above code is 10, I am afraid you are wrong. It’s pretty normal to make that assumption because we tend to think that the moment there is a return statement in a function, then it returns(exits) from the function.Well, that may not be true in this case.
如果您认为上述代码的输出是10,恐怕您错了。 做出这种假设是很正常的,因为我们倾向于认为函数中存在return语句的那一刻,它就会从函数中返回(退出),那么在这种情况下可能就不正确了。
From the docs,
从文档中
If a
finally
clause is present, the finally clause will execute as the last task before thetry
statement completes. Thefinally
clause runs whether or not thetry
statement produces an exception.如果存在
finally
子句,则try
子句完成之前,finally子句将作为最后一个任务执行。 无论try
语句是否产生异常,finally
子句都会运行。If the try statement reaches a
break
,continue
orreturn
statement, thefinally
clause will execute just prior to thebreak
,continue
orreturn
statement’s execution.如果try语句到达
break
,continue
或return
语句,则finally
子句将在break
,continue
或return
语句执行之前执行。If a
finally
clause includes areturn
statement, the returned value will be the one from thefinally
clause’sreturn
statement, not the value from thetry/except
clause’sreturn
statement.如果
finally
子句包含return
语句,则返回的值将是finally
子句的return
语句中的值,而不是try/except
子句的return
语句中的值。
So as you guessed it right, the output of the above code will be 30.
因此,您猜对了,上述代码的输出为30。
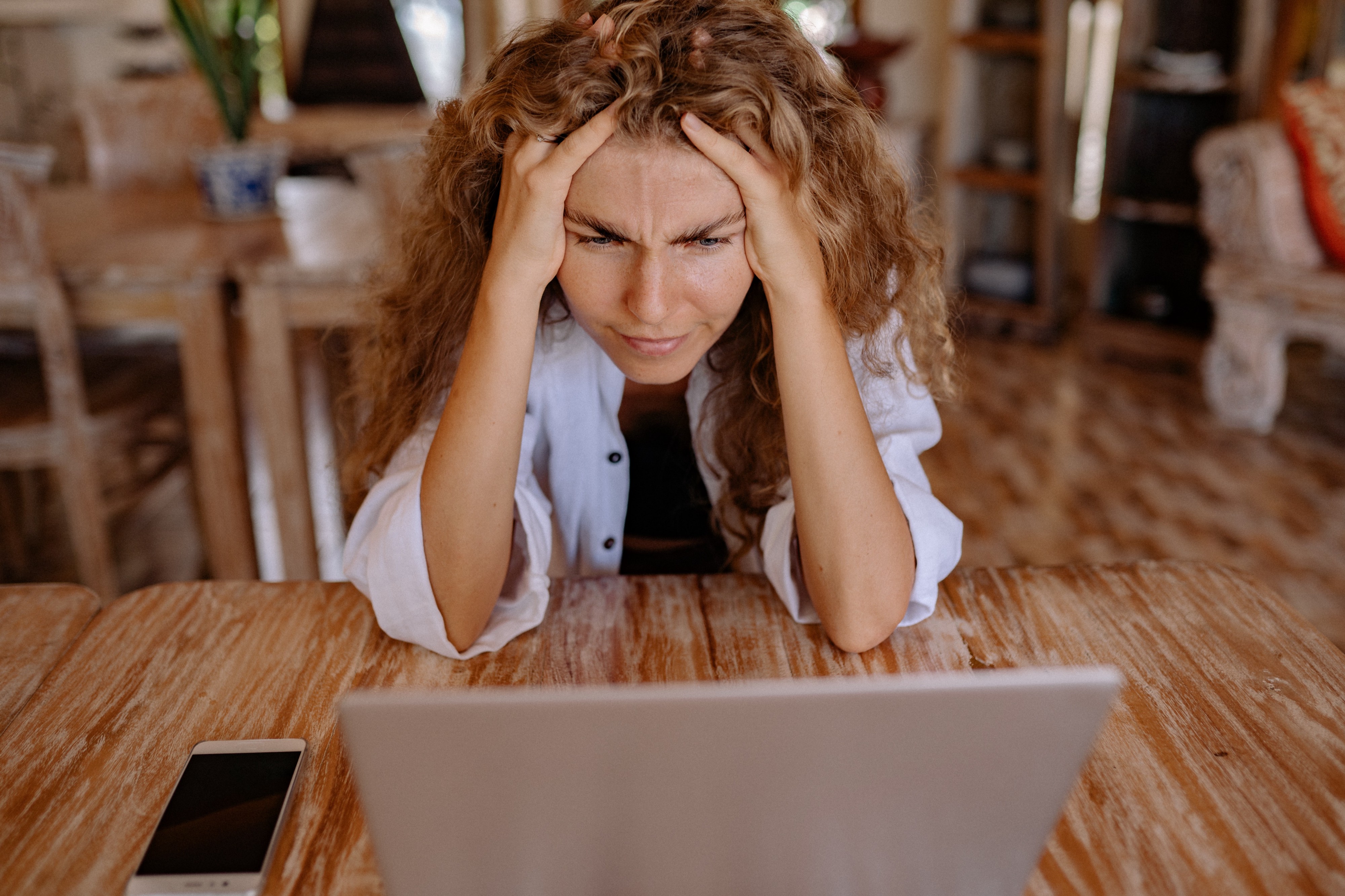
Now, what happens if an exception is raised in the aforementioned code.
现在,如果在上述代码中引发异常,会发生什么情况。
2.例外情况下退货的使用 (2. Usage of return with exceptions)
def test_func():
try:
x = 10
raise Exception
except Exception as e:
print(f" Raising exception ")
x = 20
return x
finally:
x = 30
return xprint(test_func())
Output:
Raising exception
30
So, again the output value of x will be 30. We should remember the fact that a finally statement gets executed ALWAYS.
因此,x的输出值将再次为30。我们应该记住一个事实,那就是始终执行finally语句。
To have a clearer idea of the execution flow, let’s add print statements in every block.
为了更清楚地了解执行流程,让我们在每个块中添加打印语句。
def test_func():
try:
x = 10
print(f" Inside try block ")
return x
except Exception as e:
x = 20
print(f" Inside except block ")
return x
finally:
x = 30
print(f" Inside finally block ")
return xprint(test_func())
Output:
Inside try block
Inside finally block
30
This would have given an idea on the execution flow.Now that we have a good understanding of how try/except/finally works with return statements, let’s try to squeeze in another clause.
这样可以对执行流程有所了解,现在我们对try / except / finally如何使用return语句有了很好的了解,让我们尝试挤压另一个子句。
An else clause can be added along with try/except and the
else
clause will get executed if thetry
block does not raise an exception.可以将else子句与try / except一起添加,如果
try
块未引发异常,则else
子句将被执行。
3.使用try / else / final返回的用法 (3. Usage of return with try/else/finally)
def test_func():
try:
x = 10
print(f" Inside try block ")
return x
except Exception as e:
x = 20
print(f" Inside except block ")
return x
else:
print(f" Inside else block ")
x = 40
return x
finally:
x = 30
print(f" Inside finally block ")
return x
print(test_func())Output:Inside try block
Inside finally block
30
So, why didn’t the else clause get executed here though the try block did not raise any exception. Note the return
statement in the try
block.The else block never got executed because the function returned even before the execution reached the else clause.
因此,尽管try块没有引发任何异常,为什么else子句没有在这里执行。 注意try
块中的return
语句。 else块从未执行过,因为该函数甚至在执行到达else子句之前就已返回。
Now remove the return statement in the try
block and execute the above code again.
现在,在try
块中删除return语句,然后再次执行上述代码。
def test_func():
try:
x = 10
print(f" Inside try block ")
except Exception as e:
x = 20
print(f" Inside except block ")
return x
else:
print(f" Inside else block ")
x = 40
return x
finally:
x = 30
print(f" Inside finally block ")
return x
print(test_func())Output:
Inside try block
Inside else block
Inside finally block
30
Summary:
概要:
- Use extra caution when adding return in try/except/finally clauses 在try / except / finally子句中添加return时要格外小心
The
finally
clause runs whether or not thetry
statement produces an exception.无论
try
语句是否产生异常,finally
子句都会运行。If a
finally
clause includes areturn
statement, the returned value will be the one from thefinally
clause’sreturn
statement如果
finally
子句包含return
语句,则返回的值将是finally
子句的return
语句中的值- An else clause will get executed if the try block does not raise an exception如果try块未引发异常,则将执行else子句
python 使用try