python 布尔转字符串
Strings and their Methods
字符串及其方法
Python语法(Python Syntax)
Python syntax can be executed by writing directly in the Command Line:
可以通过直接在命令行中编写来执行Python语法:
>>> print(“Hello, World!”)
Hello, World!
Or by creating a python file on the server, using the .py file extension, and running it in the Command Line:
或通过使用.py文件扩展名在服务器上创建python文件,然后在命令行中运行它:
D:\Python\programs>python myfile.py
Python缩进 (Python Indentation)
Indentation refers to the spaces at the beginning of a code line.In other programming languages the indentation in code is just for readability purpose, the indentation in Python is very important.
缩进是指代码行开头的空格。在其他编程语言中,代码中的缩进仅出于可读性目的,Python中的缩进非常重要。
Python uses indentation to indicate a block of code.
Python使用缩进来指示代码块。
>>> if True:
... print("Hello World")
...
Hello World
Python gives an error if you skip the indentation:
如果跳过缩进,Python会给出错误:
>>> if True:
... print("Hello World")
File "<stdin>", line 2
print("Hello World")
^
IndentationError: expected an indented block
>>>
评论 (Comments)
Python has commenting capability for the purpose of in-code documentation. Comments start with a ‘#’, and Python will render the rest of the line as a comment:
Python具有注释功能,可用于代码内文档。 注释以' #'开头,Python将其余的行作为注释呈现:
>>>#This is a comment.
>>>print("Hello, World!")
Hello, world!
Comments can be used to explain Python code. Comments can be used to make the code more readable. Comments can be used to prevent execution when testing code.
注释可用于解释Python代码。 注释可用于使代码更具可读性。 注释可用于在测试代码时阻止执行。
Comments can be placed at the end of a line, and Python will ignore the rest of the line:
注释可以放在一行的末尾,Python将忽略该行的其余部分:
>>>print("Hello, World!") #This is a comment
Comments does not have to be text to explain the code, it can also be used to prevent Python from executing code.
注释不必是文本来解释代码,它也可以用于阻止Python执行代码。
多行注释 (Multi Line Comments)
Python do not have a multiline comment. Since Python will ignore string literals that are not assigned to a variable, you can add a multiline string (triple quotes) in your code, and place your comment inside it:
Python没有多行注释。 由于Python会忽略未分配给变量的字符串文字,因此您可以在代码中添加多行字符串(三引号),并将注释放入其中:
"""
This is a comment
written in
more than just one line
"""
print("Hello, World!")
As long as the string is not assigned to a variable, Python will read the code, but then ignore it, and you have made a multiline comment.
只要未将字符串分配给变量,Python就会读取该代码,但随后将其忽略,并且您已做出多行注释。
Python变量 (Python Variables)
In Python, variables are created when you assign a value to it. Python has no command for declaring a variable.
在Python中,当您为其分配值时会创建变量。 Python没有用于声明变量的命令。
x = 5
y = "Hello, World!"
Here x and y are variables. x is an integer variable and y is an string variable.
这里的x和y是变量。 x是整数变量,y是字符串变量。
创建变量 (Creating Variables)
Variables are containers for storing data values. Variables are reserved memory location to store values. Unlike other programming languages, Python has no command for declaring a variable. A variable is created when we assign a value to it.
变量是用于存储数据值的容器。 变量是保留的存储器位置,用于存储值。 与其他编程语言不同,Python没有用于声明变量的命令。 当我们给变量赋值时,就创建了一个变量。
>>>a = 1000
>>>b = 10.24
>>>c = "Andhra University"
Variables do not need to be declared with any particular type and can even change type after they have been set.
变量不需要用任何特定类型声明,甚至可以在设置变量后更改类型。
>>>c = "Andhra University"
>>>c = 10000
>>>print(c)
1000
String variables can be declared either by using single or double quotes:
可以使用单引号或双引号声明字符串变量:
>>>c = "Andhra University"
#is same as
>>>c = 'Andhra University"
变量名 (Variable Names)
A variable can have a short name (like x and y) or a more descriptive name (age, car_name, total_vol).
变量可以具有短名称(如x和y)或更具描述性的名称(年龄,car_name,total_vol)。
Rules for Python variables:
Python变量规则:
- A variable name must start with a letter or the underscore character 变量名称必须以字母或下划线字符开头
- A variable name cannot start with a number变量名不能以数字开头
- A variable name can only contain alpha-numeric characters and underscores (A-z, 0–9, and _ )变量名称只能包含字母数字字符和下划线(Az,0–9和_)
- Variable names are case-sensitive (age, Age and AGE are three different variables) 变量名称区分大小写(年龄,年龄和年龄是三个不同的变量)
>>>s = "string"
>>>college_name = "Andhra University"
Here s is a simple variable and college_name is a more descriptive variable.
这里s是一个简单变量,而college_name是一个更具描述性的变量。
将值分配给多个变量 (Assign Value to Multiple Variables)
Python allows you to assign values to multiple variables in one line:
Python允许您在一行中将值分配给多个变量:
>>>a,b,c = 1,2,"Andhra"
>>>print(a)
1
>>>print(b)
2
>>>print(c)
Andhra
And you can assign the same value to multiple variables in one line:
您可以在一行中将相同的值分配给多个变量:
>>>a = b = c = "string"
>>>print(a)
string
>>>print(b)
string
>>>print(c)
string
The Python print
statement is often display the values of the variables. To combine both text and a variable, Python uses the +
character:
Python print
语句通常显示变量的值。 为了结合文本和变量,Python使用+
字符:
>>>x = "I Love"
>>>print(x + "Python")
I Love Python
Python数据类型 (Python Data Types)
In programming, data type is an important concept. Variables can store data of different types, and different types can do different things. Python has the following data types built-in by default, in these categories:
在编程中,数据类型是一个重要的概念。 变量可以存储不同类型的数据,并且不同类型可以执行不同的操作。 在这些类别中,Python默认具有以下内置数据类型:
Text Type:str
文字类型: str
Numeric Types:int
, float
, complex
数值类型: int
, float
, complex
Sequence Types:list
, tuple
, range
序列类型: list
, tuple
, range
Mapping Type:dict
映射类型: dict
Set Types:set
, frozenset
集合类型: set
, frozenset
Boolean Type:bool
布尔类型: bool
Binary Types:bytes
, bytearray
, memoryview
二进制类型: bytes
, bytearray
, memoryview
You can get the data type of any object by using the type()
function:
您可以使用type()
函数获取任何对象的数据类型:
>>>x = 5
>>>print(type(x))
<class 'int'>
Python数字 (Python Numbers)
There are three numeric types in Python:
Python中有三种数值类型:
int
int
float
float
complex
complex
Variables of numeric types are created when you assign a value to them:
在为数字类型的变量赋值时会创建它们:
>>>x = 1100 # int
>>>y = 2234.845 # float
>>>z = 1j # complex
>>>print(type(x))
<class 'int'>
>>>print(type(y))
<class 'float'>
>>>print(type(z))
<class 'complex'>
类型转换 (Type Conversion)
You can convert from one type to another with the int()
, float()
, and complex()
methods:
您可以使用int()
, float()
和complex()
方法将一种类型转换为另一种类型:
>>>x = 1100 # int
>>>y = 2234.845 # float
>>>z = 1j # complex#convert from int to float:
>>>a = float(x)
#convert from float to int:
>>>b = int(y)
#convert from int to complex:
>>>c = complex(x)
>>>print(a)
1100.0>>>print(b)
2234>>>print(c)
1100+0j>>>print(type(a))
<class 'float'>>>>print(type(b))
<class 'int'>>>>print(type(c))
<class 'complex'>
随机数 (Random Number)
Python does not have a random()
function to make a random number, but Python has a built-in module called random
that can be used to make random numbers:
Python没有用于生成随机数的random()
函数,但是Python具有一个称为random
的内置模块,可用于生成随机数:
>>> import random
>>> print(random.randrange(1, 10))
9
Python字符串 (Python Strings)
Strings are sequence of characters. String literals in python are surrounded by either single quotation marks, or double quotation marks. ‘hello’ is the same as “hello”. You can display a string literal usingprint()
function:
字符串是字符序列。 python中的字符串文字被单引号或双引号引起来。 “ hello”与“ hello”相同。 您可以使用print()
函数显示字符串文字:
>>>a = "Hello"
>>>print(a)
多行字符串 (Multiline Strings)
You can assign a multiline string to a variable by using three quotes:
您可以使用三个引号将多行字符串分配给变量:
>>>a = """Lorem ipsum dolor sit amet,
consectetur adipiscing elit,
sed do eiusmod tempor incididunt
ut labore et dolore magna aliqua."""
>>>print(a)
Or three single quotes:
或三个单引号:
>>>a = '''Lorem ipsum dolor sit amet,
consectetur adipiscing elit,
sed do eiusmod tempor incididunt
ut labore et dolore magna aliqua.'''
>>>print(a)
字符串是数组 (Strings are Arrays)
Like many other popular programming languages, strings in Python are arrays of bytes representing unicode characters. However, Python does not have a character data type, a single character is simply a string with a length of 1. Square brackets can be used to access elements of the string.
像许多其他流行的编程语言一样,Python中的字符串是表示unicode字符的字节数组。 但是,Python没有字符数据类型,单个字符就是长度为1的字符串。方括号可用于访问字符串的元素。
>>>str = "Hello, World"
>>>print(str[1]) #prints a value at index 1
e
This is the format of how the string “Hello, World” is stored as a array. The space involved in the string also counts.
这是字符串“ Hello,World”如何存储为数组的格式。 字符串中涉及的空间也很重要。
index----> 0 1 2 3 4 5 6 7 8 9 1011
H e l l o , w o r l d
-12...............-3-2-1
Therefore, str[1] is e.
因此,str [1]为e。
Python also supports negative indexing.
Python还支持负索引。
>>>str[-1] #Prints last character
d
A slice Operator is used in python to display some of the characters or substring.
python中使用切片运算符来显示某些字符或子字符串。
Synatx of slice operator: str [ start : end ]start should be always less than end (start< end) where in slice operator the start is included and end is excluded.
slice运算符的语法:str [start:end] start始终应小于end(start <end),其中slice运算符中包括start,但不包括end。
>>>str[2:5]
llo#Using Negative Indexing
>>>str[-4:-1]
orl
As I have already mentioned above, 2 will be included and 5 will be excluded. therefore, the characters at the index 2 to 5–1 will be printed.That means the characters at str[2],str[3],str[4] are printed. In the same way slice operator deals with negative indexing.
正如我上面已经提到的,将包括2个,将排除5个。 因此,将打印索引2到5–1处的字符。这意味着将打印str [2],str [3],str [4]处的字符。 以相同的方式,切片运算符处理负索引。
弦长 (String Length)
To get the length of a string, use the len()
function.
要获取字符串的长度,请使用len()
函数。
>>>a = "Hello, World!"
>>>print(len(a))
13
字符串方法 (String Methods)
Python has a set of built-in methods that you can use on strings.
Python有一组可用于字符串的内置方法。
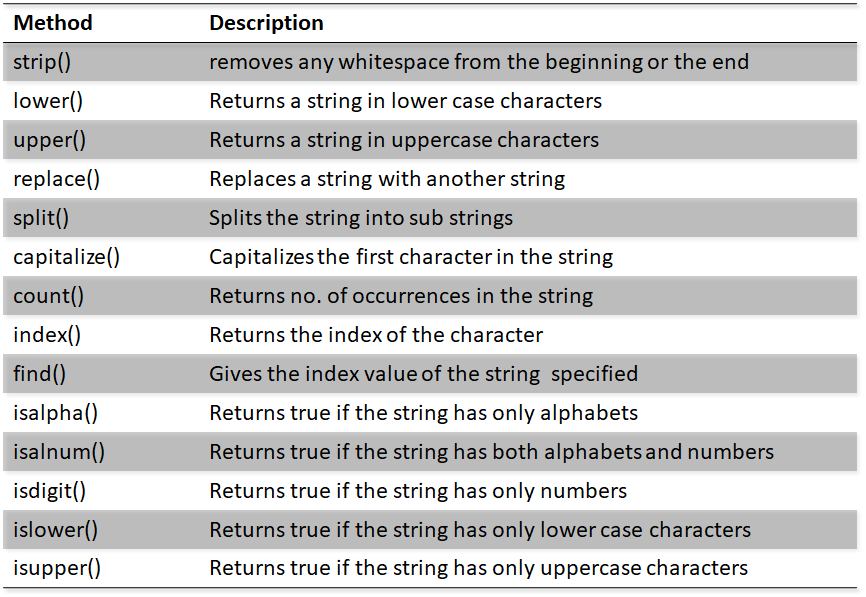
Let us check the usage of these methods.
让我们检查这些方法的用法。
>>>name = "python programming">>>print(name) #prints the string
python programming>>>print(name.upper()) #prints the sting in uppercase
PYTHON PROGRAMMING>>>print(name.lower()) #prints the string in lowercase
python programming>>>print(name.islower()) #checks if string is lowercase or not
TRUE>>>print(name.isupper()) # checks if string is uppercase or not
FALSE>>>print(name.split(" ")) #Splits the string by space
['python', 'programming']>>>print(name.capitalize()) #First character in the string becomes capital
Python programming#Counts number of times the character is repeated in the string
>>>print(name.count('p'))
2#Counts the character in the string from specified index
>>>print(name.count('p',5))
1#Counts the character in the string in between the specified index
>>>print(name.count('p',5,10))
1#replaces the character in the string
>>>print(name.replace("p", "J"))
'Jython Jrogramming'#returns the index of the character in the string
>>>print(name.index('p'))
0#Returns the index of the character in the string in from specified #index
>>>print(name.index('p',5))
7#Returns the index of the character in the string in between specified indexes
>>> print(name.index('p',5,10))
7#checks if string consists of alphabets and numbers
>>>print(name.isalnum())
FALSE#
>>>print(name.isdigit())
FALSE
Special Method format() is used to replace the strings in empty braces.
特殊方法format()用于替换空括号中的字符串。
For example:
例如:
>>>query = "I love {} and {}"
>>>print(query.format("python","hadoop"))
I love python and hadoop
Let us see about Booleans..
让我们来看看布尔值。
Python布尔值 (Python Booleans)
In programming you often need to know if an expression is True
or False
. You can evaluate any expression in Python, and get one of two answers, True
or False
. When you compare two values, the expression is evaluated and Python returns the Boolean answer:
在编程中,您通常需要知道表达式是True
还是False
。 您可以评估Python中的任何表达式,并获得两个答案之一,即True
或False
。 比较两个值时,将对表达式求值,Python返回布尔值答案:
>>>print(10 > 9)
True
>>>print(10 == 9)
False
>>>print(10 < 9)
False
The bool()
function allows you to evaluate any value, and give you True
or False
in return,
bool()
函数可让您评估任何值,并为您提供True
或False
,
多数价值观是真实的 (Most Values are True)
Almost any value is evaluated to True
if it has some sort of content.
如果具有某种内容,则几乎所有值都将评估为True
。
Any string is True
, except empty strings.
除空字符串外,任何字符串均为True
。
Any number is True
, except 0
.
除0
外,任何数字均为True
。
Any list, tuple, set, and dictionary are True
, except empty ones.
任何列表,元组,集合和字典都是True
,空列表除外。
>>>print(bool("Hello"))
True
>>>print(bool(15))
True
>>>bool("abc")
True
>>>bool(123)
False
>>>bool(["apple", "cherry", "banana"])
False
一些值是错误的 (Some Values are False)
In fact, there are not many values that evaluates to False
, except empty values, such as ()
, []
, {}
, ""
, the number 0
, and the value None
. And of course the value False
evaluates to False
.
实际上,除了空值(例如()
, []
, {}
, ""
,数字0
和值None
()
之外,没有多少可以计算为False
的值。 当然,值False
计算结果为False
。
>>>bool(False)
False
>>>bool(None)
False
>>>bool(0)
False
>>>bool("")
False
>>>bool(())
False
>>>bool([])
False
>>>bool({})
False
python 布尔转字符串