javascript 简介
Technical Committee 39 (TC39) is a group of JavaScript experts who work on the standardization of ECMAScript.
技术委员会39( TC39 )是一组JavaScript专家,他们致力于ECMAScript的标准化。
The TC39 process has a number of stages:
TC39流程分为多个阶段:
Stage 0 (Strawperson): Take input for the specification.
阶段0(Strawperson):输入规格说明。
Stage 1 (Proposal): Propose the high-level API for review.
第1阶段(提案):提出高级API进行审查。
Stage 2 (Draft): Precisely describe the syntax and semantics using formal spec language.
第2阶段(草案):使用正式规范语言精确描述语法和语义。
Stage 3 (Candidate): Designated reviewers have signed off on the current spec text.
第3阶段(候选者):指定的审阅者已经签署了当前的规范文本。
Stage 4 (Finished): The addition is ready for inclusion in the formal ECMAScript standard.
第4阶段(完成):可以将其添加到正式的ECMAScript标准中。
After stage 4, proposals will be included in the next revision of ECMAScript. When the spec goes through its yearly ratification as a standard, the proposal is ratified as part of it.
在第4阶段之后,提案将包含在ECMAScript的下一个修订版中。 当规范通过其年度批准作为标准时,该提案将作为其一部分被批准。
Since ES2015, a lot of new ECMAScript syntaxes and features have been introduced. In this article, we present four of them: **
, ??
, ?.
, and |>
.
自ES2015起,引入了许多新的ECMAScript语法和功能。 在本文中,我们介绍其中四个: **
, ??
, ?.
和|>
。
求幂运算符 (The Exponentiation Operator)
The exponentiation operator (**
) is a computation operator that’s used in the format var1 ** var2
. It returns the value of var1
to the power of var2
. It’s equivalent to Math.pow
, except it also accepts BigInt
s as operands.
幂运算符 ( **
)是一种计算运算符,格式为var1 ** var2
。 它将var1
的值返回为var2
的幂。 它等效于Math.pow
,除了它还接受BigInt
作为操作数。
The exponentiation operator (**
) is in ES2016.
幂运算符( **
)在ES2016中。
Here are some examples:
这里有些例子:
The exponentiation operator is right-associative: a ** b ** c
is equal to a ** (b ** c)
. Therefore, line 7 is equivalent to 2 ** (3 ** 3)
.
求幂运算符是右关联的: a ** b ** c
等于a ** (b ** c)
。 因此,第7行等效于2 ** (3 ** 3)
。
BigInt
is in ES2020, so even though it’s shipping in browsers, ESLint doesn’t support it by default. Without the comment at line 8, it’ll show the error “'BigInt' is not defined”
. The comment informs ESLint that BigInt
is a global variable. Therefore, don’t use the no-undef rule to warn.
BigInt
位于ES2020中,因此即使它在浏览器中提供,ESLint默认也不支持它。 如果在第8行没有注释,它将显示错误“'BigInt' is not defined”
。 该注释告知ESLint BigInt
是全局变量。 因此,请勿使用no-undef规则进行警告。
Line 10 throws an error: “Uncaught TypeError: Cannot mix BigInt and other types, use explicit conversions”
.
第10行引发错误: “Uncaught TypeError: Cannot mix BigInt and other types, use explicit conversions”
。
The following is the table of browser compatibility:
下表是浏览器兼容性表:
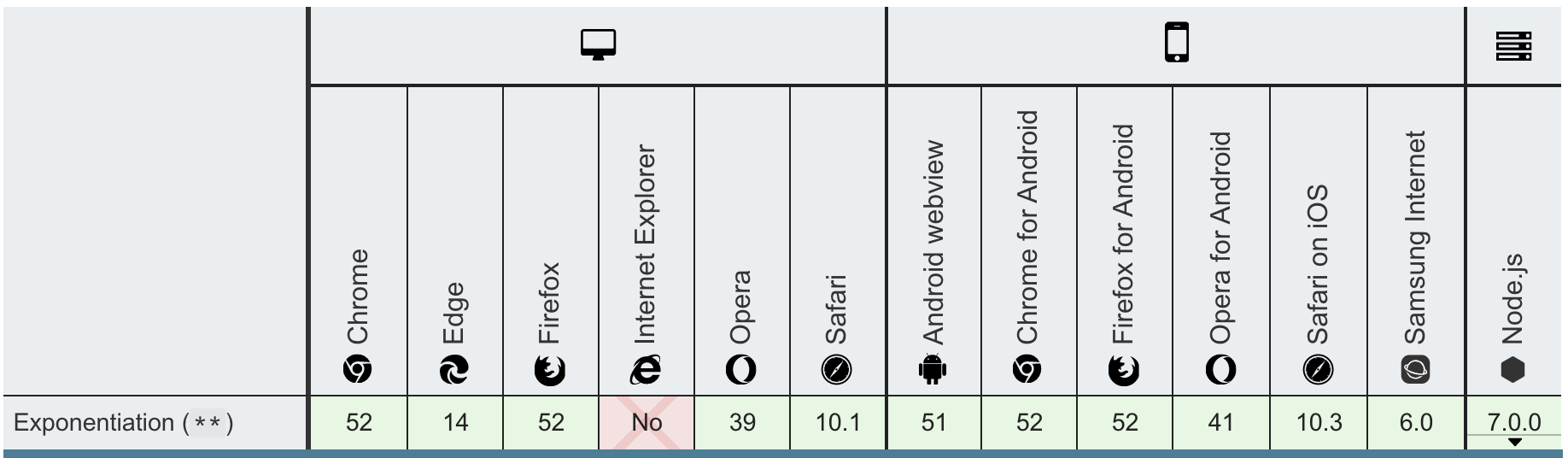
空位合并运算符 (The Nullish Coalescing Operator)
The nullish coalescing operator (??
) is a logical operator that’s used in the format leftExpr ?? rightExpr
. When the leftExpr
is null
or undefined
, it returns rightExpr
. Otherwise, it returns leftExpr
.
空合并运算符 ( ??
)是逻辑运算符,格式为leftExpr ?? rightExpr
leftExpr ?? rightExpr
。 当leftExpr
为null
或undefined
,它将返回rightExpr
。 否则,它返回leftExpr
。
The nullish coalescing operator (??
) is in ES2020.
空合并的运算符( ??
)在ES2020中。
This is an enhancement of the logical OR operator (||
), which is used in the format leftExpr || rightExpr
. When the leftExpr
is falsy, ||
returns rightExpr
. Otherwise, it returns leftExpr
. Sometimes, the expression unexpectedly returns rightExpr
when leftExpr
is a falsy value, such as false
, ‘’
, or 0
.
这是对逻辑OR运算符( ||
)的增强,其格式为leftExpr || rightExpr
leftExpr || rightExpr
。 当leftExpr
错误时, ||
返回rightExpr
。 否则,它返回leftExpr
。 有时,当leftExpr
是一个伪造的值(例如false
, ''
或0
时,表达式会意外返回rightExpr
。
The following are examples:
以下是示例:
The following is the table of browser compatibility:
下表是浏览器兼容性表:
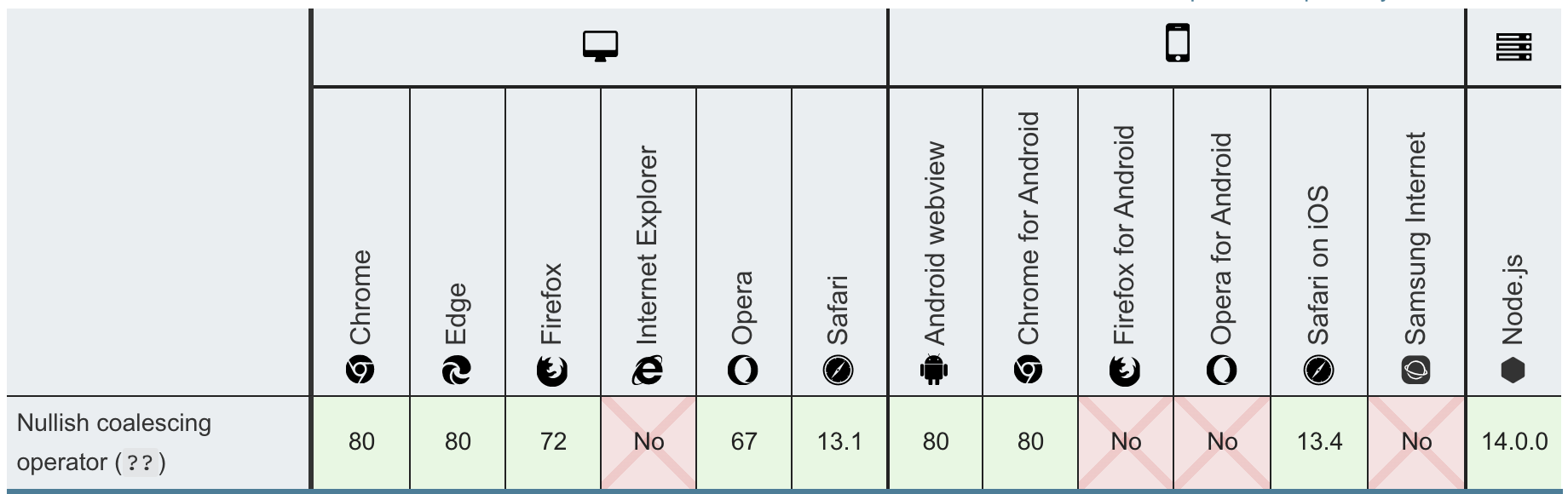
可选链接运算符 (The Optional Chaining Operator)
The optional chaining operator (?.
) is syntactic sugar to short-circuit with a return value of undefined
if the object chain has a nullish (null
or undefined
) reference in the middle.
如果对象链的中间有一个null
引用( null
或undefined
),则可选的链接运算符 ( ?.
)是语法糖,可以使用返回值undefined
进行短路。
The optional chaining operator (??
) is in ES2020.
可选的链接运算符( ??
)在ES2020中。
a?.b
is equivalent to a && a.b
.
a?.b
等同于a && ab
。
The optional chaining operators can be stacked to make the code concise.
可以堆叠可选的链接运算符以使代码简洁。
a?.b?.c?.d?.e
is equivalent to a && a.b && a.b.c && a.b.c.d && a.b.c.d.e
.
a?.b?.c?.d?.e
等效于a && ab && abc && abcd && abcde
。
The optional chaining can be used for an object, a function, or an array. Here are some examples:
可选链接可用于对象,函数或数组。 这里有些例子:
The following is the table of browser compatibility:
下表是浏览器兼容性表:
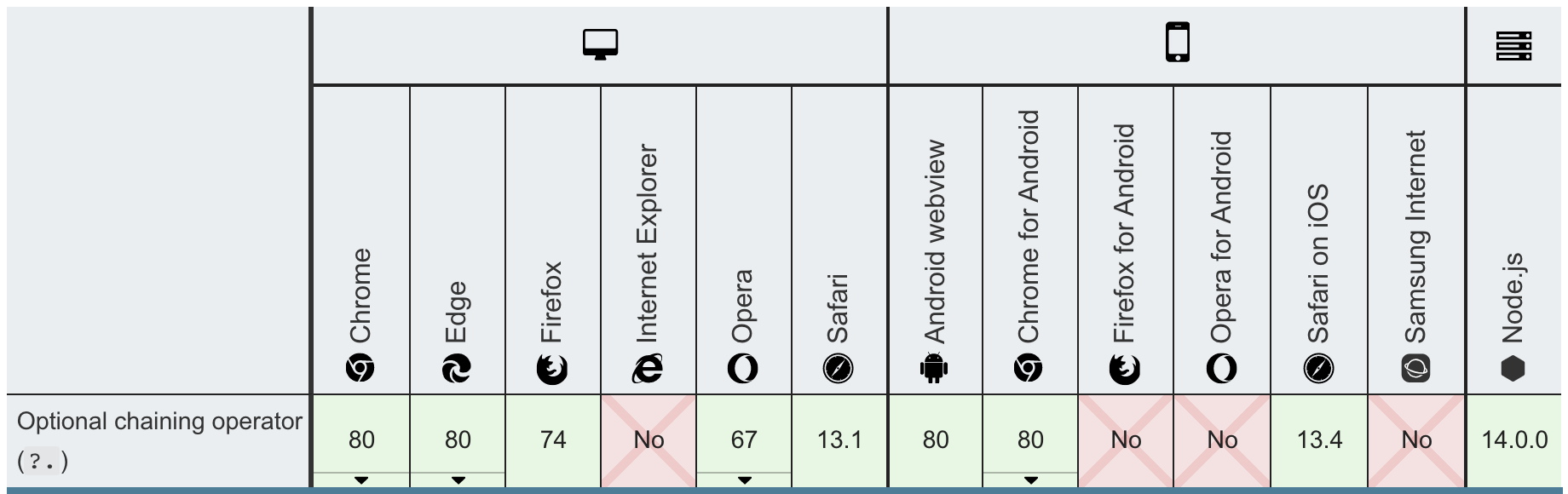
管道运营商 (The Pipeline Operator)
The pipeline operator (|>
) is syntactic sugar that’s used in the format expression |> function
. It creates chained function calls in a readable manner.
管道运算符 ( |>
)是语法糖,用于格式expression |> function
。 它以可读的方式创建链接的函数调用。
The pipeline operator (|>
) is in TC39 stage 1.
管道运算符( |>
)处于TC39阶段1。
value |> function1 |> function2 |> function3 |> function4 |> function5
is equivalent to function5(function4(function3(function2(function1(value)))))
.
value |> function1 |> function2 |> function3 |> function4 |> function5
等效于function5(function4(function3(function2(function1(value)))))
。
Here are examples:
以下是示例:
Advanced examples are defined in GitHub. It’s worth reading about.
高级示例在GitHub中定义。 值得一读。
Currently, you’re not able to run these examples. It’s experimental, and there’s no browser support.
当前,您无法运行这些示例。 这是实验性的,没有浏览器支持。
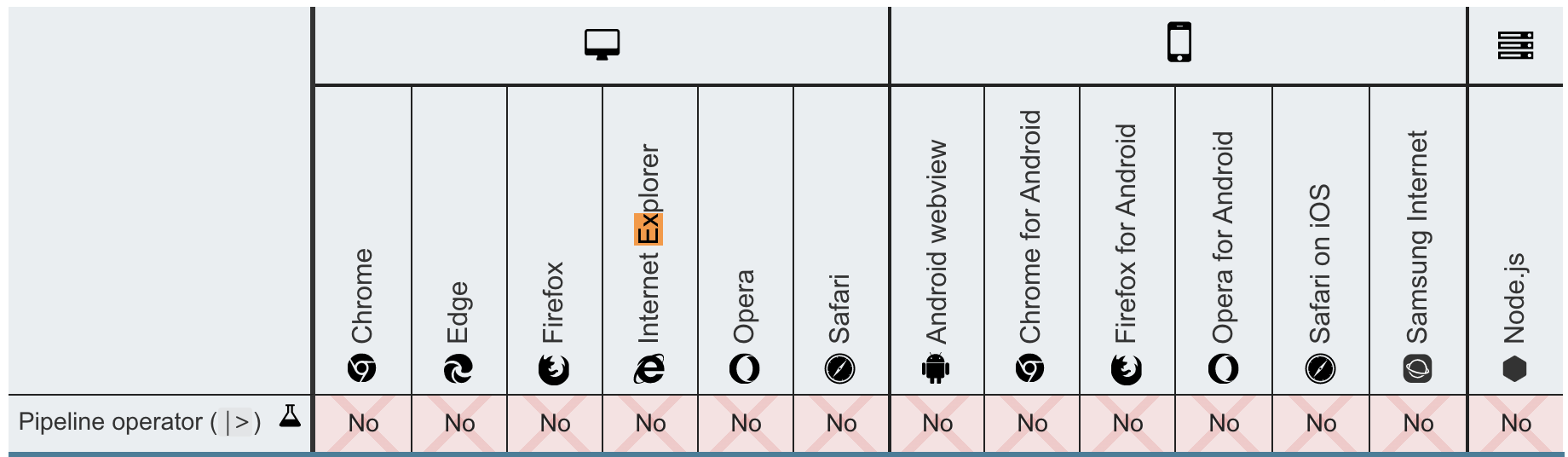
结论 (Conclusion)
Programming languages evolve over time. It’s a challenge to keep up with them. Meanwhile, it’s fun to see them improve to be more concise and more powerful.
编程语言会随着时间而发展。 跟上他们的步伐是一个挑战。 同时,很高兴看到它们不断改进以变得更加简洁和强大。
In 2021, there will be new syntaxes and new features in ECMAScript.
2021年,ECMAScript中将有新的语法和新功能。
Stay tuned!
敬请关注!
Thanks for reading. I hope this was helpful. You can see my other Medium publications here.
谢谢阅读。 我希望这可以帮到你。 您可以在这里查看我的其他Medium出版物。
javascript 简介