numpy机器学习
NumPy library is an important foundational tool for studying Machine Learning. Many of its functions are very useful for performing any mathematical or scientific calculation. As it is known that mathematics is the foundation of machine learning, most of the mathematical tasks can be performed using NumPy.
NumPy库是学习机器学习的重要基础工具。 它的许多功能对于执行任何数学或科学计算都非常有用。 众所周知,数学是机器学习的基础,大多数数学任务都可以使用NumPy执行。
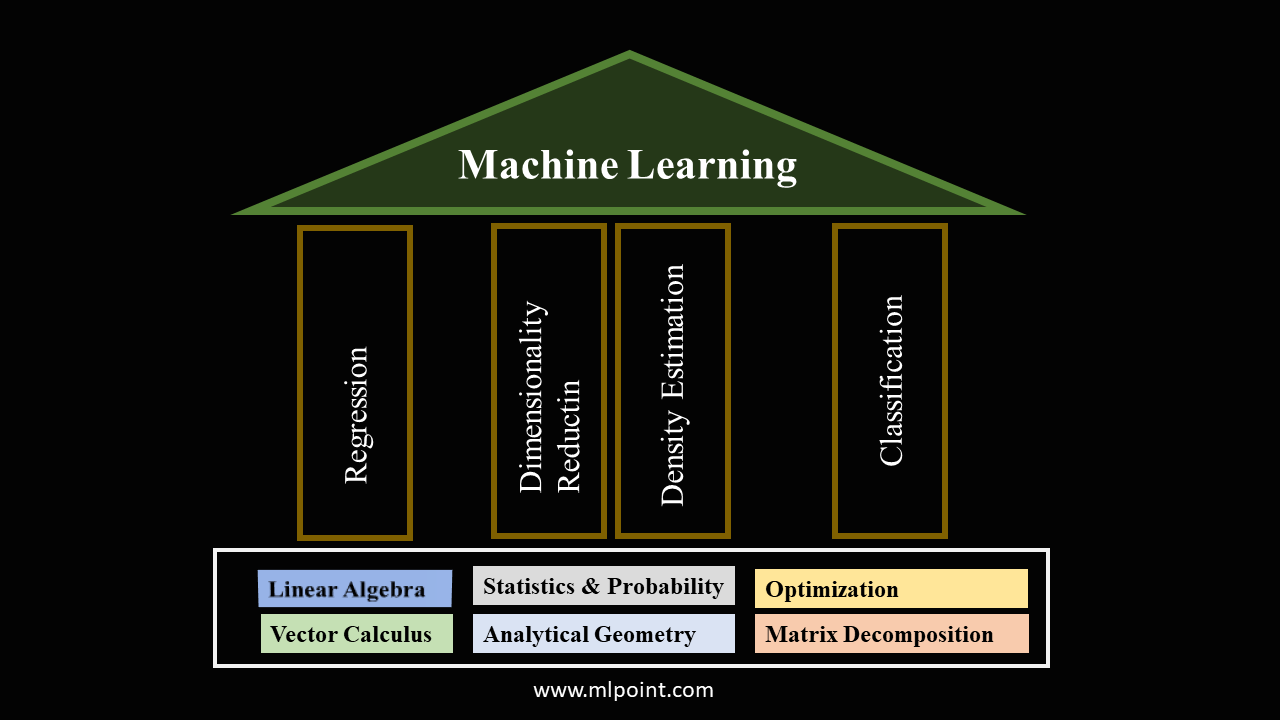
In this story, I will cover the basic concepts of functions in Numpy which are often used.
在这个故事中,我将介绍Numpy中经常使用的函数的基本概念。
1.Introduction to NumPyNumPy stands for ‘Numerical Python’. It is an open-source Python library used to perform various mathematical and scientific tasks. It contains multi-dimensional arrays and matrices, along with many high-level mathematical functions that operate on these arrays and matrices.
1. NumPy简介 NumPy代表“ Numerical Python”。 它是一个开放源代码的Python库,用于执行各种数学和科学任务。 它包含多维数组和矩阵,以及在这些数组和矩阵上运行的许多高级数学函数。
2. Installing NumPyyou can install NumPy with:conda install numpy
orpip install numpy
2.安装NumPy可以通过以下方式安装NumPy: conda install numpy
或pip install numpy
3. How to import NumPy?After installing NumPy, you can now use this library by importing it. To import NumPy use:
3.如何导入NumPy? 安装NumPy之后,您现在可以通过导入使用此库。 要导入NumPy,请使用:
import numpy as np
import numpy as np
4. What is an array?
4.什么是数组?
An array is a data structure consisting of a collection of elements (values or variables), each identified by at least one array index or key.
数组是一种数据结构,由一组元素(值或变量)组成,每个元素均由至少一个数组索引或键标识。
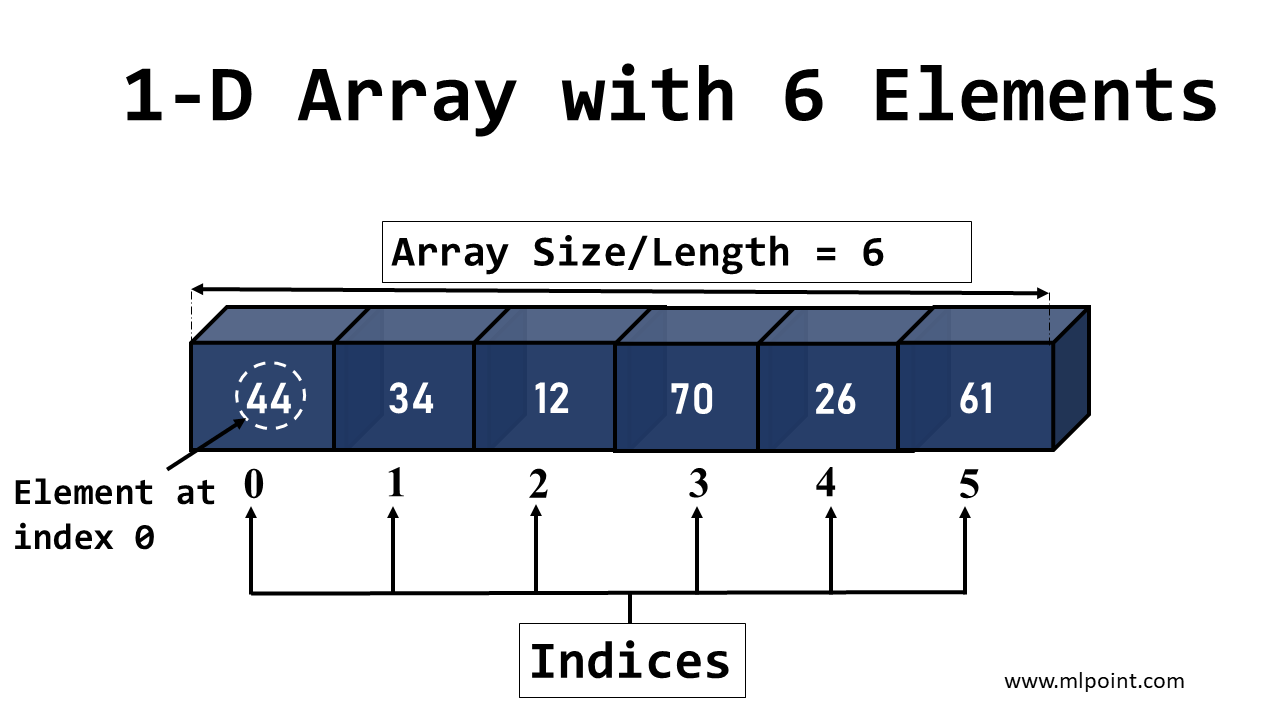
An array is known as the central data structure of the NumPy library. Array in NumPy is called as NumPy Array.
数组被称为NumPy库的中央数据结构。 NumPy中的数组称为NumPy数组。
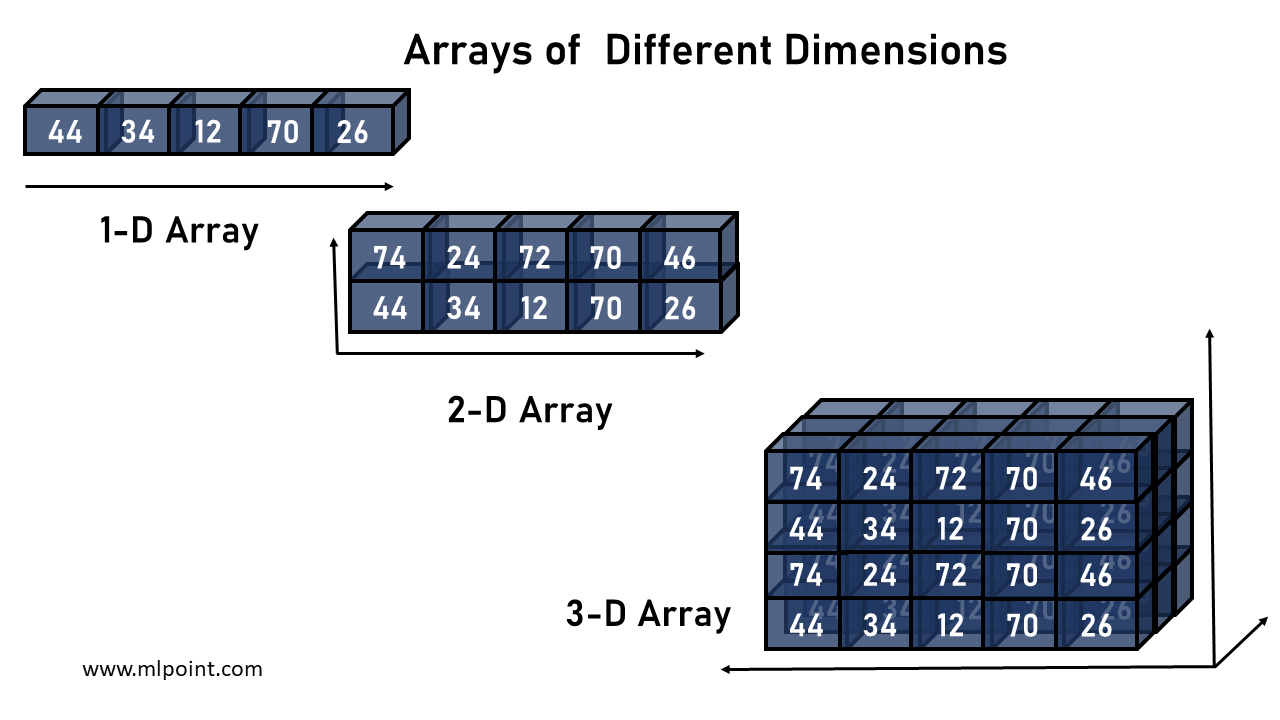
5. What is the difference between Python List and a Numpy Array?
5. Python List和Numpy Array有什么区别?
- Python list can contain elements with different data types whereas Numpy Array‘s elements are always homogeneous(same data types). Python列表可以包含具有不同数据类型的元素,而Numpy Array的元素始终是同质的(相同的数据类型)。
- NumPy arrays are faster and more compact than Python lists. NumPy数组比Python列表更快,更紧凑。
Why NumPy Arrays are faster than Lists?
为什么NumPy数组比列表快?
- NumPy Array uses fixed memory to store data and less memory than Python lists. NumPy Array使用固定内存来存储数据,并且内存少于Python列表。
- Contiguous memory allocation in NumPy Arrays. NumPy数组中的连续内存分配。
6. Creating ArraysNumPy Arrays of different dimensions can be created by using ndarray(n-dimensional array). It is an array class in NumPy.The basic ndarray can be created using the numpy.array()
function in NumPy.
6.创建数组 NumPy可以使用ndarray(n-维数组)创建不同维的数组。 它是NumPy中的一个数组类。可以使用NumPy中的numpy.array()
函数创建基本的ndarray。
numpy.array(object, dtype = None, copy = True, order = None, subok = False, ndmin = 0)
numpy.array(object,dtype = None,copy = True,order = None,subok = False,ndmin = 0)
a)1-Dimensional Array
a)一维数组
import numpy as np#creating 1-dimensional arrays#creating python list
list_1 = [3,2,7]
arr_1 = np.array(list_1,dtype=np.int16)
print(arr_1)#or
arr_1=np.array([3,2,7])
print(arr_1)output:
[3 2 7]#creating array of complex numberarr_complex = np.array([1, 2, 3], dtype = complex)
print(arr_complex)output:
[1.+0.j 2.+0.j 3.+0.j]
b)Multi-Dimensional array
b)多维数组
#creating two-dimensionsal array
list_m = [[1, 2, 3], [4, 5, 6], [7, 8, 9]] #nested lists
arr_m = np.array(list_m,dtype=np.int16)
print(arr_m)
output:
[[1 2 3]
[4 5 6]
[7 8 9]]

you can create arrays with different Data Types (dtypes): Boolean : np.bool_
Char : np.byte
Short : np.short
Integer : np.short
Long : np.int_
Float : np.single
&np.float32
Double :np.double
&np.float64 np.int8
: integer (-128 to 127)np.int16
:integer( -32768 to 32767)np.int32
: integer(-2147483648 to 2147483647)np.int64
:integer( -9223372036854775808 to 9223372036854775807)
您可以创建具有不同数据类型(dtypes)的数组: 布尔值: np.bool_
字符: np.byte
短: np.short
整数: np.short
长: np.int_
浮点数: np.single
和np.float32
Double: np.double
和np.float64 np.int8
:整数(- 128至127) np.int16
:整数(-32768至32767) np.int32
:整数(-2147483648至2147483647) np.int64
:整数(-9223372036854775808至9223372036854775807)
c)ndarray in NumPy also provides many functions to create different types of arrays.i)An Array having all elements 0
c)NumPy中的ndarray 还提供了创建不同类型数组的许多功能。i)具有所有元素的数组0
#Creating an array of all zeros
arr_0 = np.zeros((2,2))
print(arr_0)output:
[[0. 0.]
[0. 0.]]
ii)An Array having all elements 1
ii)具有所有元素1的数组
# Creating an array of all ones
arr_1= np.ones((1,2))
print(arr_1)output:
[[1. 1.]]
iii)An array with a constant
iii)具有常数的数组
# Creating a constant array
arr_c = np.full((2,2), 7)
print(arr_c)output:
[[7 7]
[7 7]]
iv)Creating an array with start value, end value and number of values
iv)创建一个包含开始值,结束值和值数的数组
arr_L = np.linspace(0, 5,7)
print(arr_L)
output:
[0. 0.83333333 1.66666667 2.5 3.33333333 4.16666667
5. ]#observe here dtype('float64')
v) Creating an array with start value, end value and number of values(datatypes int)
v)创建一个包含开始值,结束值和值个数的数组(数据类型为int)
arr_a=np.arange(1, 10,1)
print(arr_a)output:
[1 2 3 4 5 6 7 8 9]#here dtype('int32')
vi)An array filled with random values
vi)充满随机值的数组
arr_r = np.random.random((2,2))
print(arr_r)output:
[[0.05736758 0.58044299]
[0.57759937 0.85564153]]
vii)An array filled with random integers
vii)充满随机整数的数组
arr_1= np.random.randint(10,100,7)
print(arr_1)
output:
[43 15 15 62 31 88 69]
vii) An array filled with random values(which follows Normal Distribution)
vii)充满随机值的数组(遵循正态分布)
arr_n= np.random.randn(10)
print(arr_n)
output:
[ 0.71586489 -0.2929509 0.1952457 1.07499362 0.08089464 -0.18440427
-0.00194278 0.2594934 1.8713812 -0.76195258]
viii)Creating a 2x2 identity matrix
viii)创建2x2身份矩阵
arr_I = np.eye(2) Create a 2x2 identity matrix
print(arr_I)output:
[[1. 0.]
[0. 1.]]
ix)Creating Arrays from existing dataThis is useful for converting Python sequence into ndarray.
ix)根据现有数据创建数组,这对于将Python序列转换为ndarray非常有用。
numpy.asarray(a, dtype=None ,order = None)
numpy.asarray(a,dtype = None,order = None)
#converting list to ndarray
import numpy as npx = [1,2,3]
a = np.asarray(x)
print (a)
output:
[1 2 3]# ndarray from tuple
x_1 = (1,2,3)
a_1 = np.asarray(x_1)
print (a_1)
output:
[1 2 3]# ndarray from list of tuples
import numpy as npx_2 = [(1,2,3),(4,5)]
a_2 = np.asarray(x_2)
print (a_2)
output:
[(1, 2, 3) (4, 5)]
7.Important Arrays attributes
7,重要的数组属性
arr.shape :
It returns a tuple listing the length of the array along each dimension.
arr.shape :
它返回一个元组,列出沿每个维度的数组长度。
import numpy as np
arr = np.array([[1,2,3],[4,5,6]])
print (arr.shape)
output:
(2, 3)#resizing the above arraya = np.array([[1,2,3],[4,5,6]])
a.shape = (3,2)
print (a)
output:
[[1 2]
[3 4]
[5 6]]or
a = np.array([[1,2,3],[4,5,6]])
b = a.reshape(3,2)
print (b)
Output:
[[1, 2]
[3, 4]
[5, 6]]
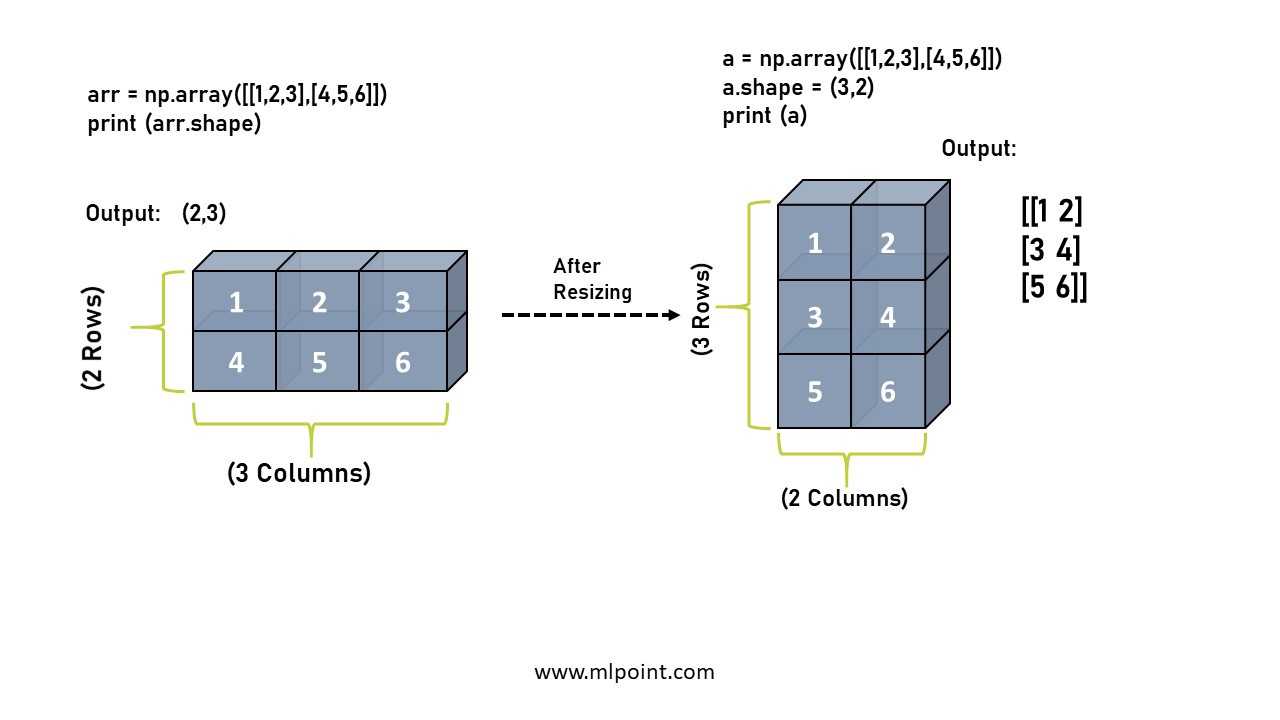
arr.size :
It returns the total number of elements in an array.
arr.size :
它返回数组中元素的总数。
import numpy as np
x = np.array([[3,2,1],[4,6,1]]) print(x.size)
output:6
arr.itemsize:
It returns the number of bytes per element.
arr.itemsize:
返回每个元素的字节数。
import numpy as np
a = np.array([[1,2,3],[4,5,6]]) a.itemsizeoutput:
4
arr.ndim:
This array attribute returns the number of array dimensions.
arr.ndim:
此数组属性返回数组维数。
import numpy as np
a = np.array([[1,2,3],[4,5,6]]) a.ndimoutput:
2
type(arr)
This attribute is used to check the type.
type(arr)
此属性用于检查类型。
#for example -
import numpy as np
arr = np.array([[1,2,3],[4,5,6]])
print (arr)
output:
[[1,2,3]
[4,5,6]]type(arr)
output:numpy.ndarray
arr.dtype:
It is used to check the data type or numeric type of the arrays.
arr.dtype:
用于检查数组的数据类型或数字类型。
#for example -print(arr.dtype)
output:int32
arr.nbytes:
It returns the number of bytes used by the data portion of the array.
arr.nbytes:
它返回数组的数据部分使用的字节数。
import numpy as np
a = np.array([[1,2,3],[4,5,6]]) a.nbytesoutput:24
8.Indexing&SlicingIndexing is used to assign numbers to each element in the NumPy array or we can say it maps to the elements stored in an array. It helps to access an array element by referring to its index number.
8.Indexing&Slicing索引用于为NumPy数组中的每个元素分配数字,或者可以说它映射到存储在数组中的元素。 它通过引用其索引号来帮助访问数组元素。
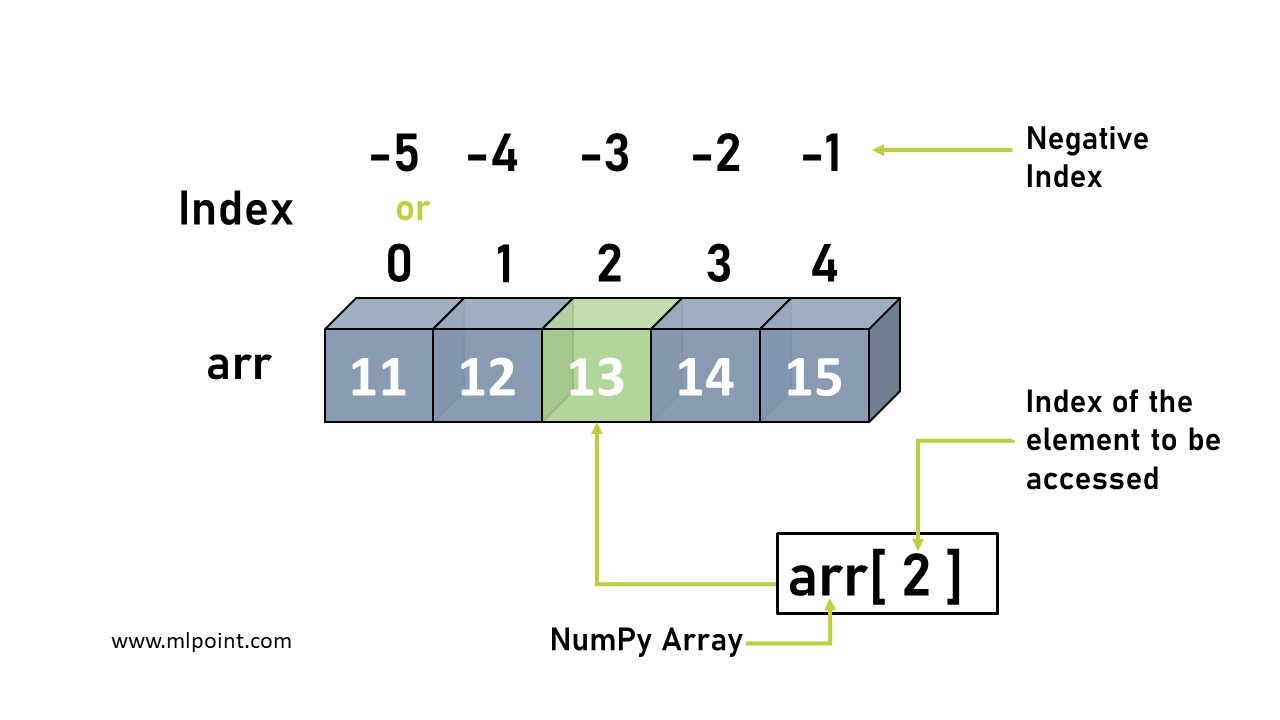
import numpy as np
arr = np.arange(10)
print(arr)
output:
[0 1 2 3 4 5 6 7 8 9]#Example-1print(arr[7])
or
print(arr[-3])
output:
7#Example-2print(arr[0:5])
or
print(arr[0:-4])output:[0 1 2 3 4 5]#Example-3print(arr[-4:-1])output:[6 7 8]
Slicing is an operation used to extract a portion of elements from arrays by specifying lower and upper bound.
切片是用于通过指定下限和上限从数组中提取元素的一部分的操作。
arr[lower:upper:step]The lower bound element is included but the upper bound element is not included. The step value indicates the stride between the elements.
arr [lower:upper:step]包含下限元素,但不包含上限元素。 步长值指示元素之间的步幅。
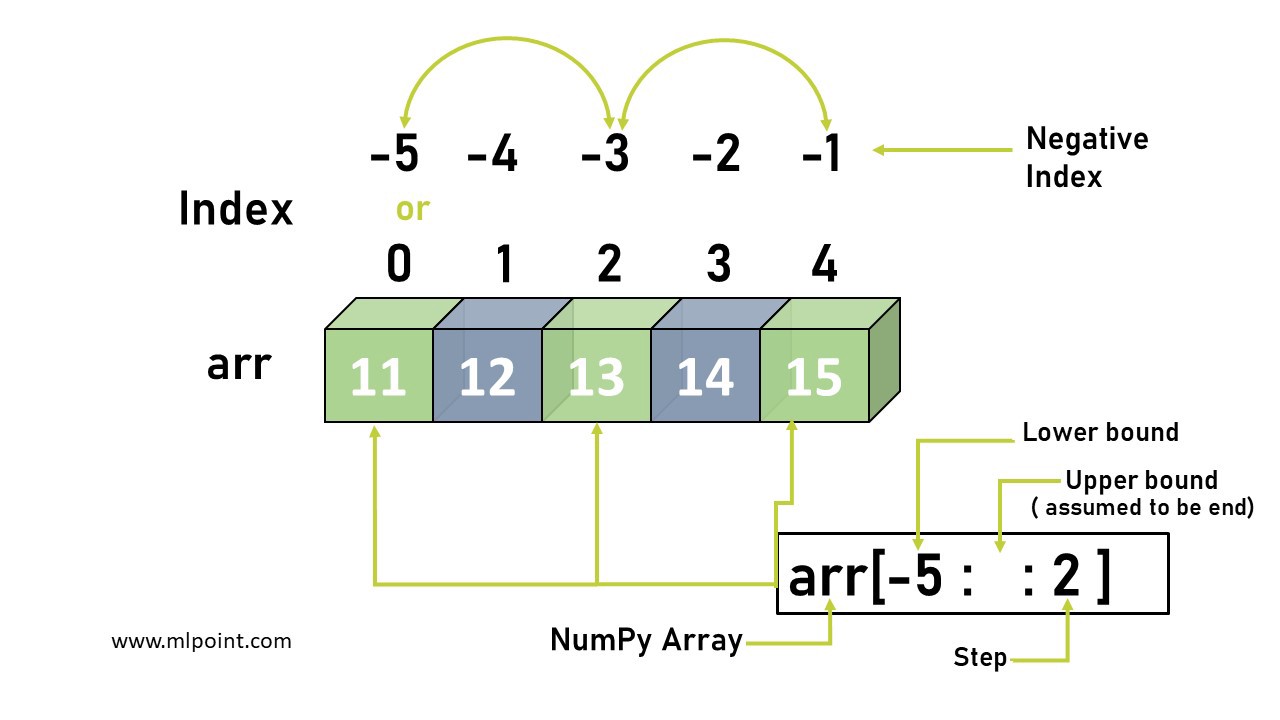
- Slicing 1-Dimensional Array 切片一维数组
import numpy as np
arr = np.arange(10)#Slicing single elementslice_arr = arr[2]
print (slice_arr)output:[2]#Slicing elements in between indexesprint (arr[2:5])orprint (arr[-8:-5]) #using negative indexoutput:[2,3,4]#using slice(lower,upper,step) functions = slice(2,7,2) print (arr[s])
output:[2,4,6]orslice_arr = arr[2:7:2]
print (slice_arr)
output:[2,4,6]
- For slicing specific elements 用于切片特定元素
x= np.array([11,12,13,14,15])
print(np.take(x, [0, 3,4]))
output:
[11 14 15]
- Omitting Indices-Omitted boundaries are assumed to be the beginning or end of the array/list. To understand better you can refer the above figure. 省略索引省略的边界被假定为数组/列表的开始或结尾。 为了更好地理解,您可以参考上图。
arr=np.arange(10)#Example-1print(arr[:5])
output:
[0 1 2 3 4]#Example-2print(arr[5:])
output:
[5 6 7 8 9]#Example-3
print(arr[::2])
output:
[0 2 4 6 8]
Slicing Multi-Dimensional Arrays
切片多维数组
Slicing can be done in multi-dimensional arrays same as the above examples.
切片可以与上述示例相同的多维数组进行。
import numpy as np
arr = np.array([[1,2,3],[3,4,5],[4,5,6]])
print (arr)
output:
[[1 2 3]
[3 4 5]
[4 5 6]]#Slicing first row
print (arr[0:1])
output:
[[1 2 3]]#slicing last 2 rows
print (arr[1:])
output:
[[3 4 5]
[4 5 6]]#slicing three rows and first column
print (arr[0:3,0:1])
output:
[[1]
[3]
[4]]#slicing 4 from above array
print (arr[1:2,1:2])
output:
[[4]]
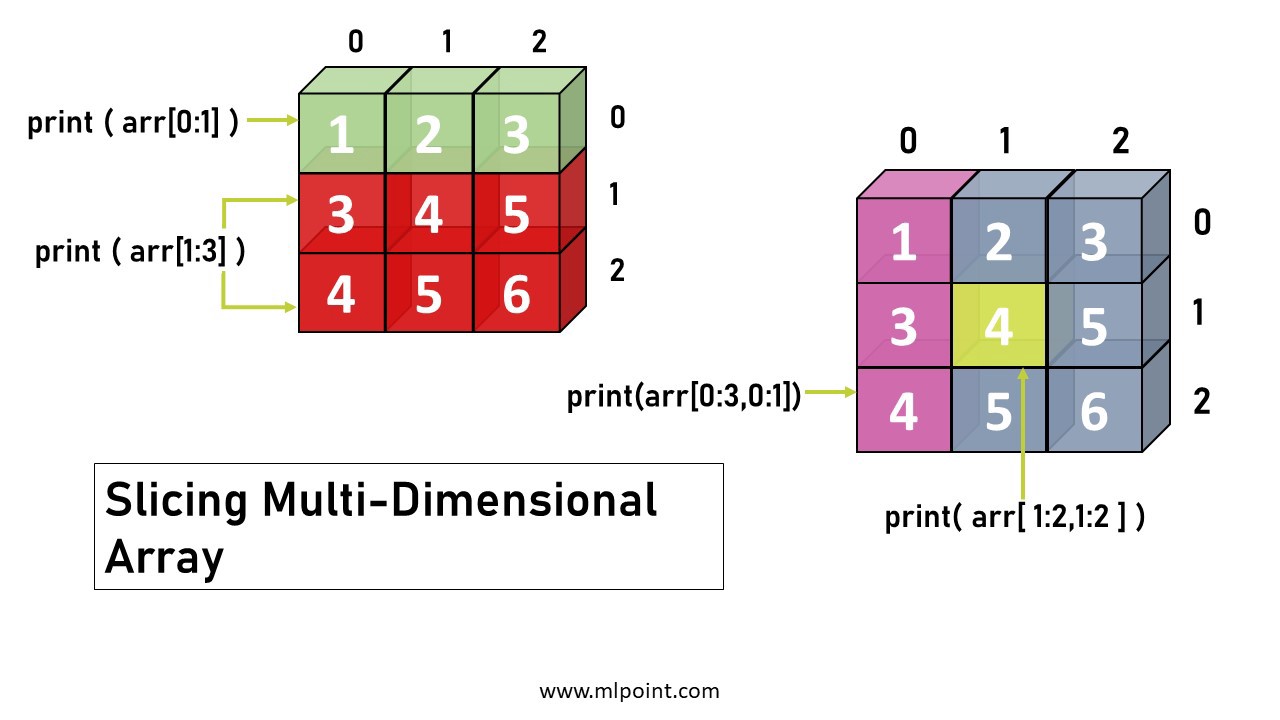
9.Stacking & SplittingStacking is used to join a sequence of same dimension arrays along a new axis.numpy.stack(arrays,axis)
: It returns a stacked array of the input arrays which has one more dimension than the input arrays.
9.堆叠和拆分堆叠用于沿着新轴连接一系列相同尺寸的数组。 numpy.stack(arrays,axis)
:它返回输入数组的堆叠数组,该数组的维数比输入数组大。
import numpy as np
arr_1 = np.array([1, 2, 3])
arr_2 = np.array([2, 3, 4])arr_stack=np.stack((arr_1,arr_2),axis=0)
print(arr_stack)
print(arr_stack.shape)
output:
[[1 2 3]
[2 3 4]](2,3)arr_stack=np.stack((arr_1,arr_2),axis=1)
print(arr_stack)
print(arr_stack.shape)
output:
[[1 2]
[2 3]
[3 4]](3,2)
The axis parameter specifies the index of the new axis in the dimensions of the result.
axis参数指定结果尺寸中新轴的索引。
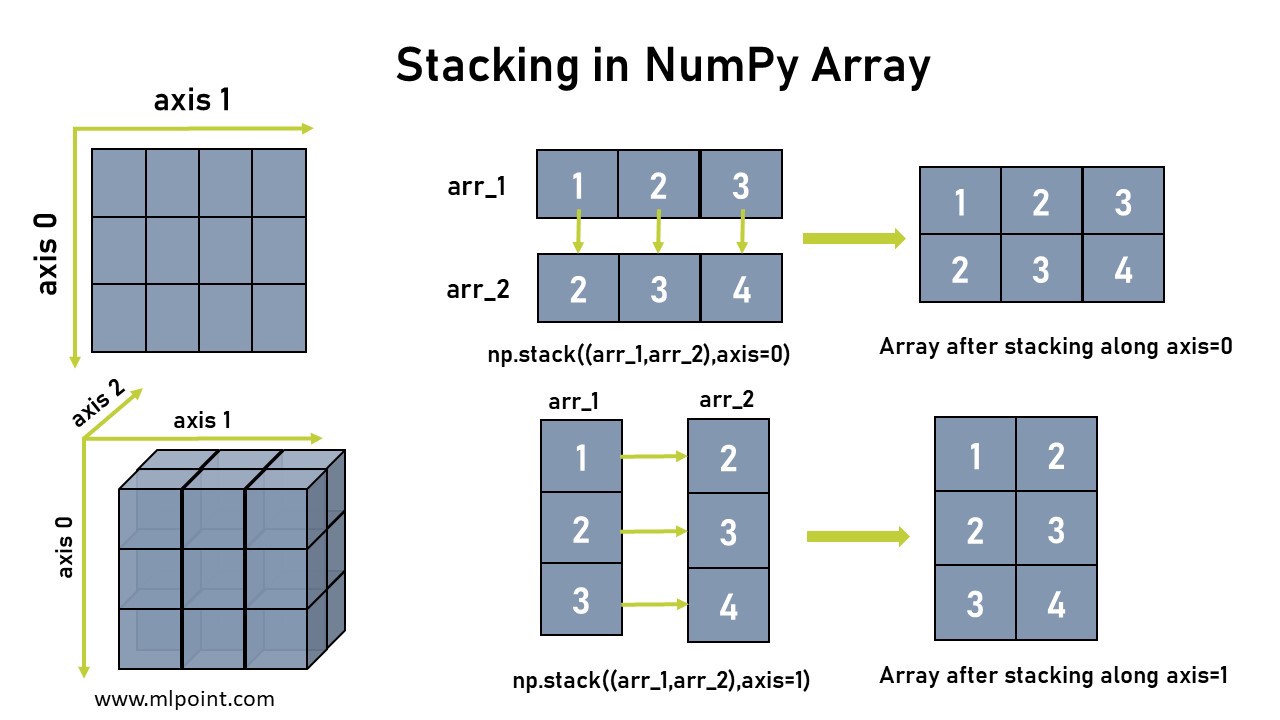
numpy.vstack(tup)
: Join arrays in sequence vertically (row-wise). The array formed by stacking will be at least 2-D. The arrays to be stacked must have the same shape along all but the first axis. 1-D arrays must have the same length.
numpy.vstack(tup)
:按顺序垂直(行numpy.vstack(tup)
连接数组。 通过堆叠形成的阵列将至少是二维的。 除了第一个轴外,要堆叠的阵列必须具有相同的形状。 一维数组必须具有相同的长度。
numpy.hstack(tup)
: Join arrays in sequence horizontally(column-wise). The arrays to be stacked must have the same shape along all but the second axis, except 1-D arrays which can be any length.
numpy.hstack(tup)
:水平(按列)按顺序连接数组。 除第二个轴外,要堆叠的阵列必须具有相同的形状,一维阵列可以是任意长度。
# Generate random arrays
arr_1 = np.random.randint(10, size=(2, 2))
print(" arr_1\n", arr_1)arr_2 = np.random.randint(10, size=(2, 2))
print(" arr_2\n", arr_2)output:
arr_1
[[0 2]
[7 6]]
arr_2
[[3 7]
[1 4]]# Stack arr_2 under arr_1
a=np.vstack((arr_1, arr_2))
print(a)
output:
[[0 2]
[7 6]
[3 7]
[1 4]]# Stacking horizontally
b=np.hstack((arr_1, arr_2))
print(b)
output:
[[0 2 3 7]
[7 6 1 4]]
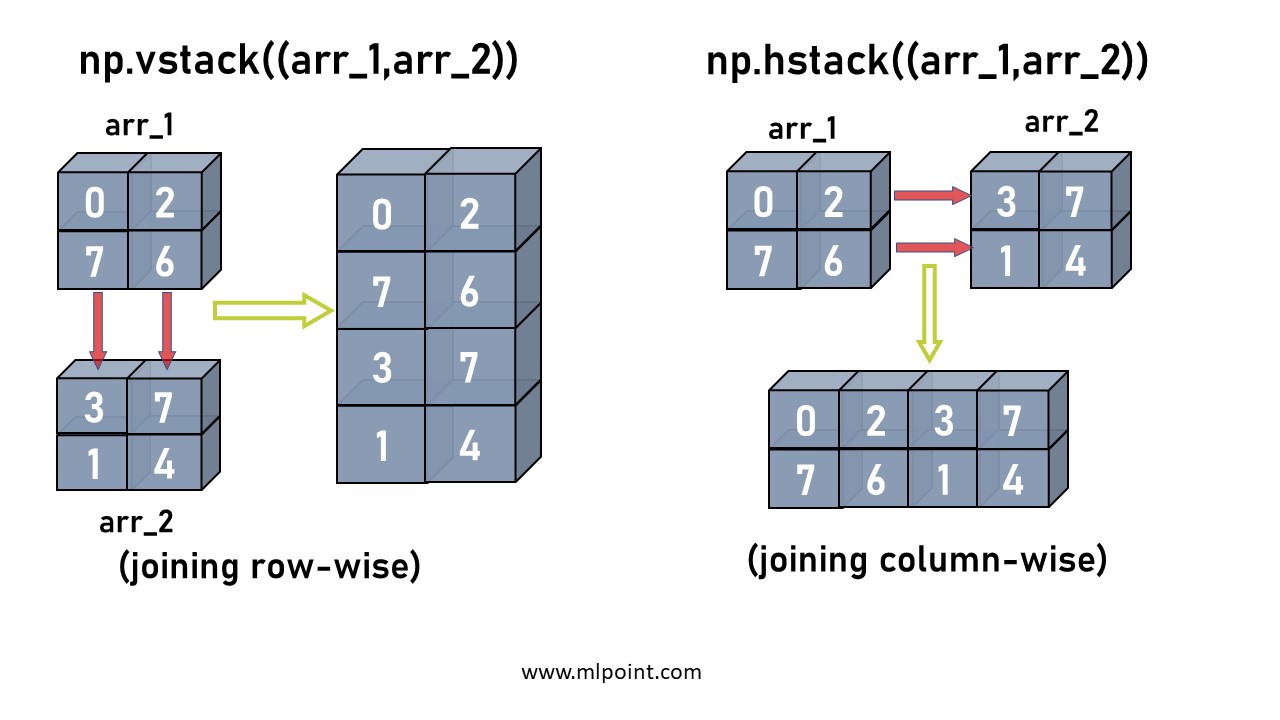
numpy.dstack(tup)
: Join arrays in sequence depth wise (along the third axis). The array formed by stacking will be at least 3-D. The arrays to be stacked must have the same shape along all but the third axis. 1-D or 2-D arrays must have the same shape.
numpy.dstack(tup)
:按深度顺序(沿第三个轴)连接数组。 通过堆叠形成的阵列将至少是3-D。 除第三条轴外,要堆叠的阵列必须具有相同的形状。 一维或二维阵列必须具有相同的形状。
#continuing above commands#stacking depth wise
c=np.dstack((arr_1, arr_2))
print(c)
output:[[[0 3]
[2 7]][[7 1]
[6 4]]]
numpy.column_stack(tup)
: It works the same as hstack and joins arrays in sequence horizontally(column-wise).
numpy.column_stack(tup)
:它与hstack相同,并按水平顺序(列方式)连接数组。
numpy.row_stack(tup)
: It works the same as vstack and joins arrays in sequence vertically(row-wise).
numpy.row_stack(tup)
:它与vstack相同,并按垂直(行)顺序连接数组。
# row_stack()
a=np.row_stack((arr_1, arr_2))
print(a)
output:
[[0 2]
[7 6]
[3 7]
[1 4]]# column_stack()
b=np.column_stack((arr_1, arr_2))
print(b)
output:
[[0 2 3 7]
[7 6 1 4]]
Splitting is used to split an array into multiple sub-arrays. It can be done using following NumPy functions.
拆分用于将一个数组拆分为多个子数组。 可以使用以下NumPy函数来完成。
numpy.split(array,Indices_or_sections,axis)
: This function used to split an array into multiple sub-arrays of equal size. It returns a list of sub-arrays.
numpy.split(array,Indices_or_sections,axis)
:此函数用于将数组拆分为大小相等的多个子数组。 它返回一个子数组列表。
x = np.arange(9.0)
np.split(x, 3)output:
[array([0., 1., 2.]), array([3., 4., 5.]), array([6., 7., 8.])]
numpy.array_split(array,Indices_or_sections,axis)
: This function is also used to split an array into multiple sub-arrays of equal or near-equal size.
numpy.array_split(array,Indices_or_sections,axis)
:此函数还用于将数组拆分为大小相等或近似相等的多个子数组。
x = np.arange(8.0)
np.array_split(x, 3)output:[array([0., 1., 2.]), array([3., 4., 5.]), array([6., 7.])]x = np.arange(7.0)
np.array_split(x, 3)output:
[array([0., 1., 2.]), array([3., 4.]), array([5., 6.])]#all splitted arrays are not equal in size
numpy.hsplit(array,Indices_or_sections,axis)
: This function is also used to split an array into multiple sub-arrays but horizontally (column-wise). hsplit
is equivalent to split
with axis=1.
numpy.hsplit(array,Indices_or_sections,axis)
:此函数还用于将一个数组拆分为多个子数组,但水平(列方向)。 hsplit
等效于使用axis=1.
进行split
axis=1.
arr_1 = np.arange(16.0).reshape(4, 4) arr_1
output:array([[ 0., 1., 2., 3.],
[ 4., 5., 6., 7.],
[ 8., 9., 10., 11.],
[12., 13., 14., 15.]])np.hsplit(arr_1, 2)output:[array([[ 0., 1.],
[ 4., 5.],
[ 8., 9.],
[12., 13.]]),
array([[ 2., 3.],
[ 6., 7.],
[10., 11.],
[14., 15.]])]np.hsplit(arr_1, np.array([3, 6]))output:[array([[ 0., 1., 2.],
[ 4., 5., 6.],
[ 8., 9., 10.],
[12., 13., 14.]]),
array([[ 3.],
[ 7.],
[11.],
[15.]]),
array([], shape=(4, 0), dtype=float64)]
numpy.vsplit(array,Indices_or_sections,axis)
: This function is also used to split an array into multiple sub-arrays but vertically (row-wise). hsplit
is equivalent to split
with axis=0.
numpy.vsplit(array,Indices_or_sections,axis)
:此函数还用于将一个数组拆分为多个子数组,但垂直(行)。 hsplit
等效于使用axis=0.
进行split
axis=0.
arr_1= np.arange(16.0).reshape(4, 4)
arr_1output:
array([[ 0., 1., 2., 3.],
[ 4., 5., 6., 7.],
[ 8., 9., 10., 11.],
[12., 13., 14., 15.]])np.vsplit(arr_1, 2)
output:[array([[0., 1., 2., 3.],
[4., 5., 6., 7.]]), array([[ 8., 9., 10., 11.],
[12., 13., 14., 15.]])]np.vsplit(arr_1, np.array([3, 6]))output:[array([[ 0., 1., 2., 3.],
[ 4., 5., 6., 7.],
[ 8., 9., 10., 11.]]), array([[12., 13., 14., 15.]]), array([], shape=(0, 4), dtype=float64)]
11.Basic Maths with NumPy
第11章NumPy基础数学
- Addition,Subtraction,Multiplication and Division between two arrays. 两个数组之间的加法,减法,乘法和除法。
import numpy as np
arr_3 = np.array([1, 2, 3, 4])
arr_4 = np.array([2, 4, 6, 8])# Add values
print(arr_3 + arr_4)
ornp.add(arr_3 ,arr_4)
output:
[ 3, 6, 9, 12]# Subtract
print(arr_3 - arr_4)
ornp.subtract(arr_3 ,arr_4)output:
[-1, -2, -3, -4]# Multiply
print(arr_3 * arr_4)or
np.multiply(arr_3 ,arr_4)output:
[ 2, 8, 18, 32]# Divide
print(arr_3 / arr_4)ornp.divide(arr_3,arr_4)output:
[0.5, 0.5, 0.5, 0.5]
- Operation on array using sum() & cumsum() function. 使用sum()和cumsum()函数对数组进行操作。
#Random 2 by 3 digit 2D array between 0 to 100
arr_6 =np.random.randint(100, size=(2, 3))
print(arr_6)
output:
[[62, 96, 50],
[87, 26, 42]]# Sum of values in array
a=arr_3.sum()
print(a)
output:
10# Sum columns
c=arr_6.sum(axis=0)
print(c)
output:
[149 122 92]# Cumulative sum of rows
b=arr_6.cumsum(axis=1)
print(b)
output:
[[ 62 158 208]
[ 87 113 155]]
- Minimum and Maximum value from an array 数组的最小值和最大值
arr=np.array([[62, 96, 50],
[87, 26, 42]])
# Min of each row
print(arr.min(axis=1))
output:
[52 1]# Max of each column
print(arr.max(axis=0))
output:[87 96 50]
- Absolute Value of every elements 每个元素的绝对值
print(np.absolute([-1, -2]))
output:
[1, 2]
- Exponent/Power , Square Root and Cube Root functions 指数/幂,平方根和立方根函数
arr_5 = np.array([[1, 2], [3, 4]])
arr_6 = np.array([[2, 4], [6, 9]])# Return values in 1st array to powers defined in 2nd arrayprint(np.power(arr_5, arr_6))
output:
[[ 1, 16],
[ 729, 262144]]arr_3 = np.array([1, 2, 3, 4])#Square Root
print(np.sqrt(arr_3))
output:
[1. 1.41421356 1.73205081 2. ]#Cube Root
print(np.cbrt(arr_3))
output:[1. 1.25992105 1.44224957 1.58740105]
- Exponential of all elements in array 数组中所有元素的指数
arr_3 = np.array([1, 2, 3, 4])#Exponential of all elements in arrayprint(np.exp(arr_3))
output:
[ 2.71828183 7.3890561 20.08553692 54.59815003]
- Logarithmic Functions 对数函数
print(np.log(arr_3))
output:
[0. 0.69314718 1.09861229 1.38629436]print(np.log2(arr_3))
output:
[0. 1. 1.5849625 2. ]print(np.log10(arr_3))
output:
[0. 0.30103 0.47712125 0.60205999]
- Greatest common divisor 最大公约数
print(np.gcd.reduce([9, 12, 15]))
output:
3
- Least Common Multiple 最小公倍数
print(np.lcm.reduce([9, 12, 15]))
output:
180
- Round down and Round up of elements of an array 向下舍入和向上舍入数组的元素
# Round down
print(np.floor([1.2, 2.5]))output:
[1. 2.]# Round up
print(np.ceil([1.2, 2.5]))
output:
[2. 3.]
12.Trigonometry Functions in NumPy
12,NumPy中的三角函数
numpy.sin()
:Sine (x) Function
numpy.sin()
:正弦(x)函数
numpy.cos()
:Cosine(x) Function
numpy.cos()
:余弦(x)函数
numpy.tan()
:Tangent(x)Function
numpy.tan()
:Tangent(x)函数
numpy.sinh()
:Hyperbolic Sine (x) Function
numpy.sinh()
:双曲正弦(x)函数
numpy.cosh()
:Hyperbolic Cosine(x) Functionv
numpy.cosh()
:双曲余弦(x)函数numpy.cosh()
numpy.tanh()
:Hyperbolic Tangent(x)Function
numpy.tanh()
:双曲正切(x)函数
numpy.arcsin()
:Inverse Sine Function
numpy.arcsin()
:反正numpy.arcsin()
函数
numpy.arccos()
:Inverse Cosine Function
numpy.arccos()
:反余弦函数
numpy.arctan()
: Inverse Tangent Function
numpy.arctan()
:反正切函数
numpy.pi
: pi value
numpy.pi
:pi值
numpy.hypot(w,h)
:For calculating Hypotenuse c = √w² + h²
numpy.hypot(w,h)
:用于计算斜边c =√w²+h²
numpy.rad2deg()
: Radians to degrees
numpy.rad2deg()
:弧度
numpy.deg2rad()
: Degrees to radians
numpy.deg2rad()
:弧度
- Plotting trigonometric functions 绘制三角函数
import numpy as np
import matplotlib.pyplot as plt# Generate array of 200 values between -pi & pi
t_arr = np.linspace(-2*np.pi, 2*np.pi, 200)fig = plt.figure()
ax = plt.axes()
ax.grid(color='black', linestyle='-', linewidth=2,alpha=0.1)# Plotting Sin(X)
plt.plot(t_arr, np.sin(t_arr),color='black') # SIN
plt.title("sin(x)")
# Display plot
plt.show()
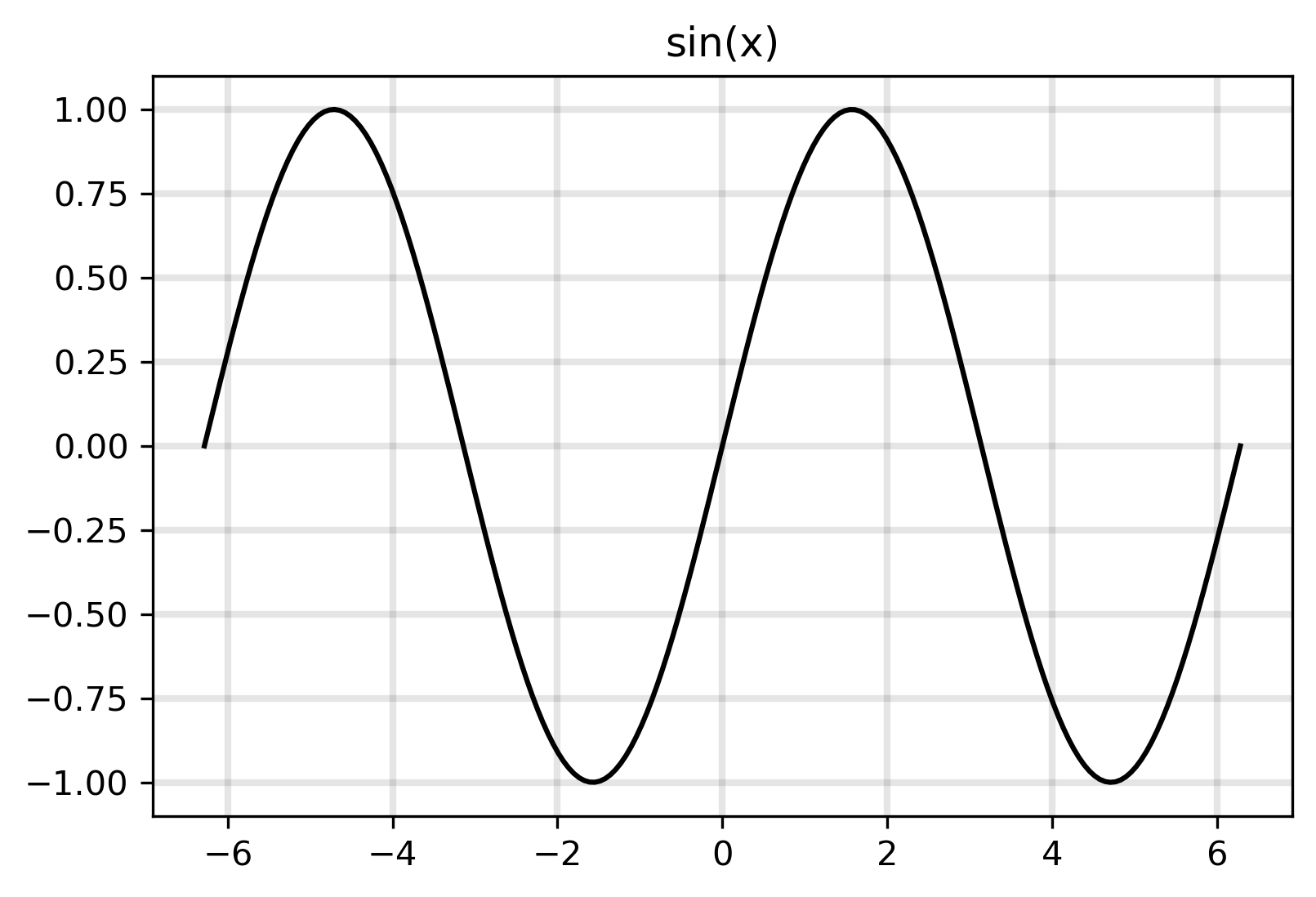
#plotting cos(x)
plt.plot(t_arr, np.cos(t_arr),color='darkorange') # COS
plt.title("cos(x)")

#plotting tan(x)
plt.plot(t_arr, np.tan(t_arr),color='skyblue') # TAN
plt.title("tan(x)")
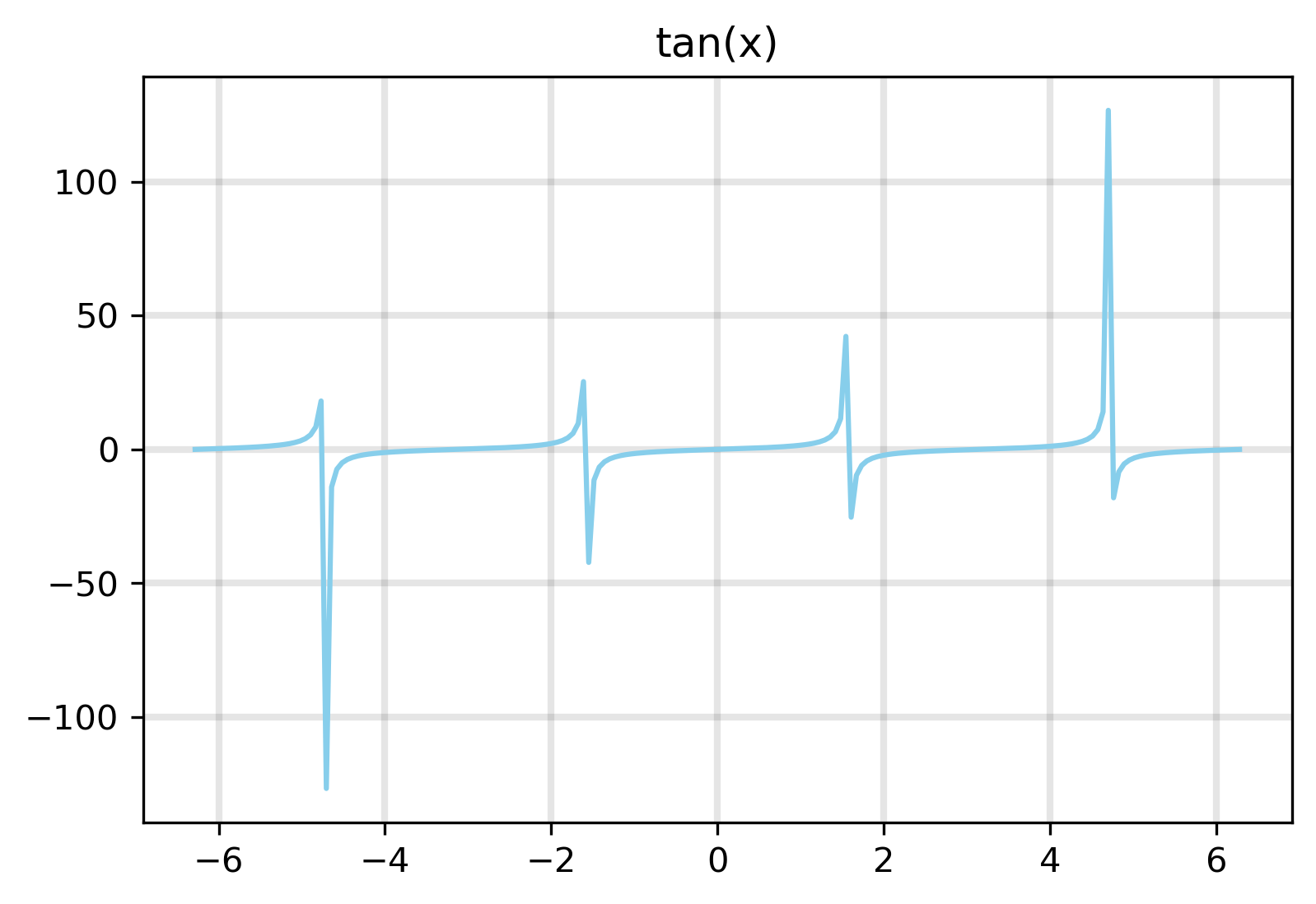
- Plotting all plots in a single graph 在单个图形中绘制所有图
plt.plot(t_arr, np.sin(t_arr),color='black',label='sin(x)') # SIN
plt.plot(t_arr, np.cos(t_arr),color='darkorange',label='cos(x)') # COS
plt.plot(t_arr, np.tan(t_arr),color='skyblue',label='tan(x)') # TAN
plt.legend(loc='best')
plt.show()

- Inverse trigonometric functions 反三角函数
# Provides inverse
print(np.arcsin(1))
print(np.arccos(1))
print(np.arctan(0))
output:
1.5707963267948966
0.0
0.0
- Radians to degrees & Degrees to radians 弧度到度和度到弧度
# Radians to degrees
print(np.rad2deg(np.pi))
output:
180.0
# Degrees to radians
print(np.deg2rad(180))
output:
3.141592653589793
13.Important Statistics Functions in NumPy
13,NumPy中重要的统计功能
numpy.mean()
: This function is used to calculate the mean of data.
numpy.mean()
:此函数用于计算数据均值。
numpy.average()
: To calculate the average of any data.
numpy.average()
:计算任何数据的平均值。
numpy.median()
: To calculate the median.
numpy.median()
:计算中位数。
numpy.mode()
: To calculate the mode of any data.
numpy.mode()
:计算任何数据的模式。
numpy.var()
: To calculate the variance of any data.
numpy.var()
:计算任何数据的方差。
numpy.std()
: To calculate the standard deviation.
numpy.std()
:计算标准偏差。
numpy.percentile()
: To calculate percentile values.
numpy.percentile()
:计算百分位值。
numpy.corrcoef()
: To calculate the correlation coefficient.
numpy.corrcoef()
:计算相关系数。
Examples:
例子:
arr_1 = np.arange(1, 6)
print(arr_1)
output:
[1 2 3 4 5]#mean
print(np.mean(arr_1))
output:
3.0#median
print(np.median(arr_1))
output:
3.0#average
print(np.average(arr_1))
output:
3.0# Standard Deviation
print(np.std([4, 6, 3, 5, 2]))
output:
1.4142135623730951#variance
print(np.var([4, 6, 3, 5, 2]))
output:
2.0
14.Linear Algebra with NumPy
14,带NumPy的线性代数
- Vector Transpose 矢量转置
import numpy as np
import matplotlib.pyplot as plt
from mpl_toolkits.mplot3d import Axes3D
v3=[ 4, 6 , 7 ]
v2= [ 4 ,7 ,9 ]v3t = np.transpose(v3)
print(v3t)
output:
[ 4, 6 , 7 ]
- Vector Addition & Subtraction 向量加法和减法
#taking 2 vectors of same dimention
v1 = np.array([ 2 ,6 ])
v2 = np.array([ 5 ,7 ])v3 = v1+v2 #Additionprint(v3)output:
[ 7, 13]v4 = v2-v1 #Subtraction
print(v4)
output:
[3, 1]
- Vector Multiplication 向量乘法
# VECTOR SCALAR PRODUCT
v1 = np.array([ 9 ,2 ])
c= 0.7
v2= c*v1
print(v2)
output:
[6.3, 1.4]# dot product
v1 = np.array([ 4 ,3 ,9 ])
v2 = np.array([ 1 ,2 ,3 ])#method 1
D_P1 = sum( np.multiply(v1,v2) )
print(D_P1)
output:
37#method 2
D_P2 = np.dot( v1,v2 )
print(D_P2)
output:
37#method 3
D_P3= np.matmul( v1,v2 )
print(D_P3)
output:
37
- Hadamard Multiplication 哈达玛乘法
# create vectors
w1 = [ 1, 3, 5 ]
w2 = [ 3, 4, 2 ]w3 = np.multiply(w1,w2)
print(w3)
output:
[ 3 12 10]
- Vector Cross Product 矢量叉积
v1 = np.array([3,9,9])
v2 = np.array([2,3,4])
v3 = np.cross (v1,v2)
print (v3)
output:
[ 9 6 -9]
- Vector Length 向量长度
# vector
v1 = np.array([ 3,4,6 ])# example method 1
vl = np.sqrt( sum( np.multiply(v1,v1)) )or# method 2: take the norm
vl = np.linalg.norm(v1)print(vl)
output:
7.810249675906654
There are many more functions are available in NumPy which can be used to solve problems of linear algebra easily.
NumPy中提供了更多功能,可轻松解决线性代数问题。
NumPy is the basis of many other libraries in python, it very vast and difficult to cover full NumPy functions in a single blog. This blog will give you a glance of NumPy library which covers most used functions. So that's the end of this blog.
NumPy是python中许多其他库的基础,它非常庞大且难以在单个博客中涵盖完整的NumPy函数。 该博客将使您一览NumPy库,其中涵盖了最常用的功能。 到此为止。
Happy Coding 😊
快乐编码😊
翻译自: https://medium.com/mlpoint/numpy-for-machine-learning-211a3e58b574
numpy机器学习