最小二乘法解决什么问题
Hello, everyone!
大家好!
It’s been quite some time since I last posted, but I am back with another JavaScript algorithm tutorial. This time we’ll be solving the Minimum Operations problem, which tasks you with finding the minimum number of operations needed to make each element of an array equal. There are a few more specific guidelines to the problem, which are outlined below.
自上次发布以来已经有一段时间了,但是我又回来了另一篇JavaScript算法教程。 这次,我们将解决“ 最小操作数”问题,该任务将为您找到使数组的每个元素相等所需的最小操作数。 有一些针对此问题的更具体的准则,概述如下。
You have an array arr of length n where arr[i] = (2 * i) + 1 for all valid values of i (i.e. 0 <= i < n).In one operation, you can select two indices x and y where 0 <= x, y < n and subtract 1 from arr[x] and add 1 to arr[y] (i.e. perform arr[x] -= 1 arr[y] += 1). The goal is to make all the elements of the array equal. It is guaranteed that all the elements of the array can be made equal using some operations.Given an integer n, the length of the array. Return the minimum number of operations needed to make all the elements of arr equal.
After reading the problem description, I immediately noticed that although we are meant to iterate over an array, there is no array being passed into the function as an argument. This means that our first course of action is to create and populate an array where each element follows the formula outlined in the instructions, arr[i] = (2 * i) + 1
. Let’s declare a variable called arr
and set it equal to an empty array.
阅读问题描述后,我立即注意到,尽管我们打算遍历数组,但没有数组作为参数传递给函数。 这意味着我们的第一个操作步骤是创建并填充一个数组,其中每个元素都遵循指令中概述的公式arr[i] = (2 * i) + 1
。 让我们声明一个名为arr
的变量,并将其设置为一个空数组。
Next we’ll create a for loop
that loops from 0
to n
. Within each iteration of the for loop
we’ll run the formula to populate the array with the intended values.
接下来,我们将创建一个从0
到n
循环的for loop
。 在for loop
每次迭代中for loop
我们将运行公式以使用预期值填充数组。
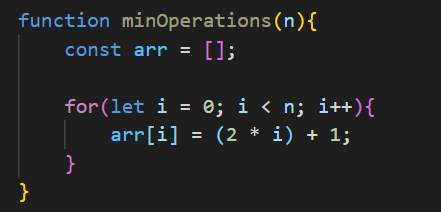
Now that our array is populated, we can begin solving the problem.
现在已经填充了数组,我们可以开始解决问题了。
Our next step will be to create a couple of variables. Our first variable, which we’ll call counter
will keep track of the number of operations our function has made, and two additional variables, front
and end
, will act as pointers that will be initialized to represent the first and last indices of our array. Note: These pointer variables represent the x
and y
variables in the problem description.
我们的下一步将是创建几个变量。 我们的第一个变量(称为counter
将跟踪函数执行的操作数,另外两个变量front
和end
将用作指针,将初始化这些指针以表示数组的第一个索引和最后一个索引。 注意: 这些指针变量代表 问题描述中 的 x
和 y
变量。
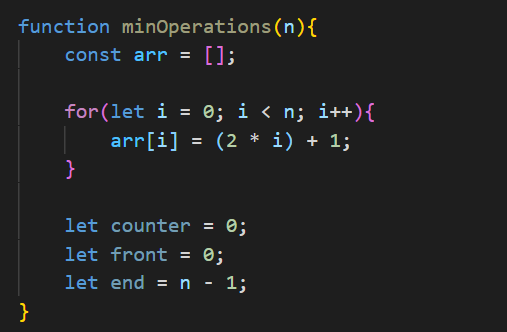
Next we’re going to create a second for loop
to iterate over arr
and compare the elements at the pointer indices, checking if they are equal. If they are not equal, we must operate on the values, incrementing the element at front
’s index and decrementing the element at end
’s index. Additionally, we’ll increment counter
here as well because we just operated on the array. Since we want to increment and decrement until the two elements are equal, we will use a while loop
as our conditional.
接下来,我们将创建第二个for loop
以遍历arr
并比较指针索引处的元素,检查它们是否相等。 如果它们不相等,则必须对这些值进行运算,使front
的索引处的元素递增,而end
的索引处的元素递减。 另外,我们也将在此处递增counter
,因为我们只是在数组上进行操作。 由于我们要递增和递减直到两个元素相等,因此我们将使用while loop
作为条件。
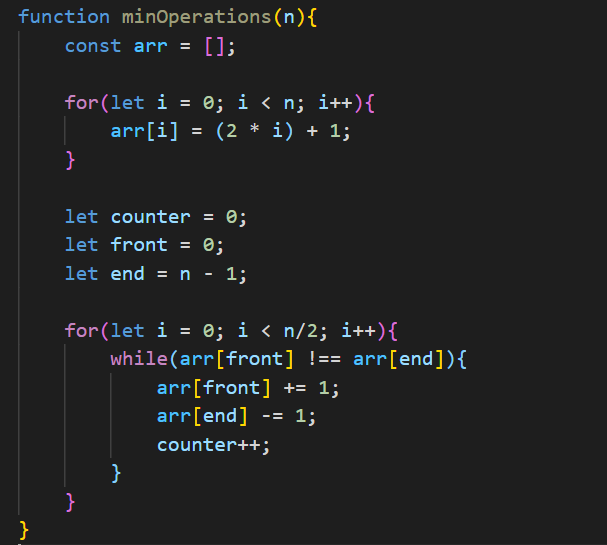
Once the two elements are equal, our function will exit the while loop
and we’ll need to check the next two elements. To do this, we will increment front
and decrement end
by one.
一旦两个元素相等,我们的函数将退出while loop
,我们需要检查接下来的两个元素。 要做到这一点,我们将增加front
和递减end
的一个。

The next iteration of our for loop
will begin and if the two elements are not equal, then the while loop
and corresponding code will run again. This process will continue until the for loop
has completed the last iteration and all elements have been made equal. All that’s left to do after this is return counter
outside of the for loop
to output the total number of operations the function had to make in order to achieve total equality.
for loop
的下一个迭代将开始,如果两个元素不相等,则while loop
和相应的代码将再次运行。 该过程将继续进行,直到for loop
完成最后一次迭代且所有元素均相等为止。 此后剩下要做的就是在for loop
外的return counter
,以输出该函数为实现完全相等而必须执行的操作总数。
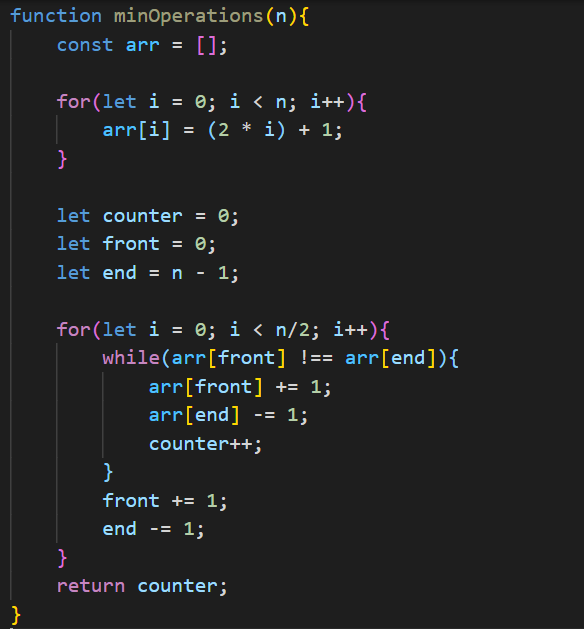
结论 (Conclusion)
When I first encountered the Minimum Operations problem, I found the description to be a bit difficult to understand. While reading it a second time, I began to identify and break the problem down into parts (creating and populating an array, comparing elements, adjusting values, etc.) and noticed similarities between this problem and others I’ve solved before.
当我第一次遇到最低操作问题时,我发现描述有些难以理解。 在第二遍阅读时,我开始将问题识别并分解为多个部分(创建和填充数组,比较元素,调整值等),并注意到该问题与我之前解决的其他问题之间的相似之处。
Hopefully when you first read the problem description you were able to identify a few possible ways to approach the solution, such as the use of pointers to compare two variables at once. If you did not come up with that strategy initially, that’s alright! You now are equipped to handle problems like this in the future.
希望当您初次阅读问题描述时,您能够找到几种解决方案的可能方法,例如使用指针一次比较两个变量。 如果您最初没有提出该策略,那没关系! 您现在已准备好将来处理此类问题。
翻译自: https://medium.com/@briandsalemi/how-to-solving-the-minimum-operations-question-3a6d43edf54a
最小二乘法解决什么问题