Have you ever worked on a problem where you needed to make a list of all the numbers between two inputs and manipulate them in some sort of way? Recently, I found myself in just this predicament and thought that JavaScript would have a nice and easy built in function to make the process easier, like Ruby does. I was surprised to find that there is nothing similar to the simple built in Ruby Range method, and quite a bit of different ways to accomplish this in JavaScript.
您是否曾经遇到过一个问题,需要列出两个输入之间的所有数字并以某种方式对其进行操作? 最近,我发现自己正处在这种困境中,并认为JavaScript会像Ruby一样,具有一个易于使用的内置函数来使过程更容易。 我很惊讶地发现,没有什么比使用Ruby Range方法内置的简单方法更相似了,并且在JavaScript中有很多不同的方法可以实现此目的。
In Ruby, you can build a new array from ranges by using the built in method .to_a
or the Array
method. If we need to create a range of years from 2010 to 2020, you would do it like (2010..2020).to_a
or Array (2010..2020)
. Inside the parenthesis we are giving Ruby two numbers and two dots, which Ruby interprets as creating an inclusive range from 2010 to 2020.
在Ruby中,可以使用内置方法.to_a
或Array
方法从范围构建新数组 。 如果我们需要创建从2010年到2020年的范围,则可以使用(2010..2020).to_a
或Array (2010..2020)
。 在括号内,我们给Ruby两个数字和两个点,Ruby将其解释为在2010年至2020年之间创建了一个包含范围。
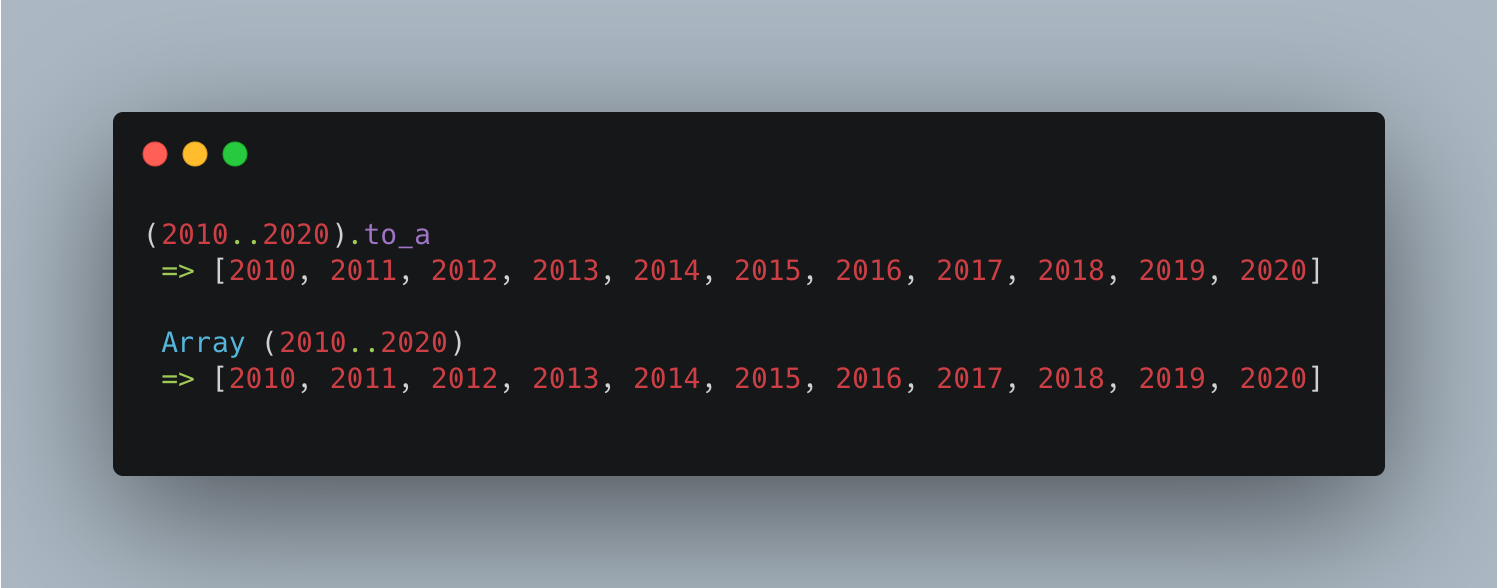
As seen above, and inclusive range creates an array for of all the numbers between and including the ones that invoked it.
如上所示,“包含”和“包含”范围将为包含调用它的数字之间的所有数字创建一个数组。
Ruby also allows us to call the same two methods with three dots, which would create a new array that would exclude the specified highest value.
Ruby还允许我们使用三个点调用相同的两个方法,这将创建一个新数组,该数组将排除指定的最大值。
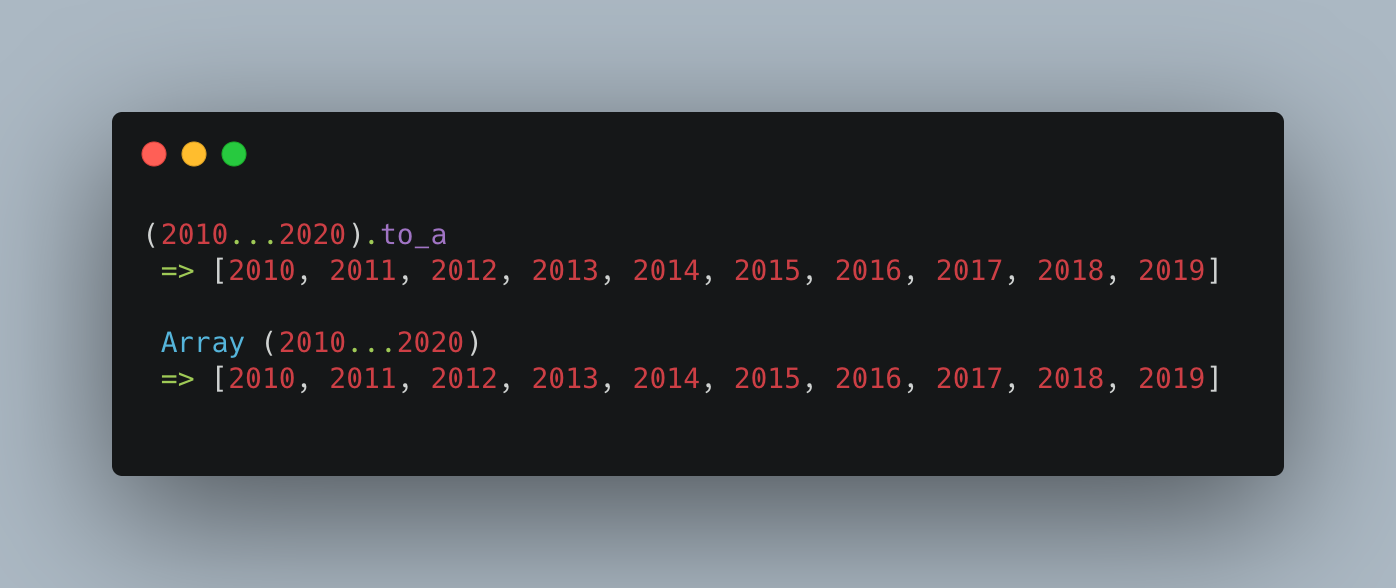
Calling our range method with three dots 2010...2020
creates an array for all the numbers from 2010–2019, and does not include 2020. As you can see, very easy and simple to create a range with specified parameters. In Ruby, the range functionality doesn’t only stop at numbers! Letters, conditionals, and even intervals can all use the built in range methods to produce some easy to read and compute functionality in our code.
用三个点2010...2020
调用范围方法会为2010-2019年的所有数字创建一个数组,并且不包括2020。如您所见,创建具有指定参数的范围非常容易。 在Ruby中,范围功能不仅止于数字! 字母,条件,甚至间隔都可以使用内置的范围方法在我们的代码中产生一些易于阅读和计算的功能。
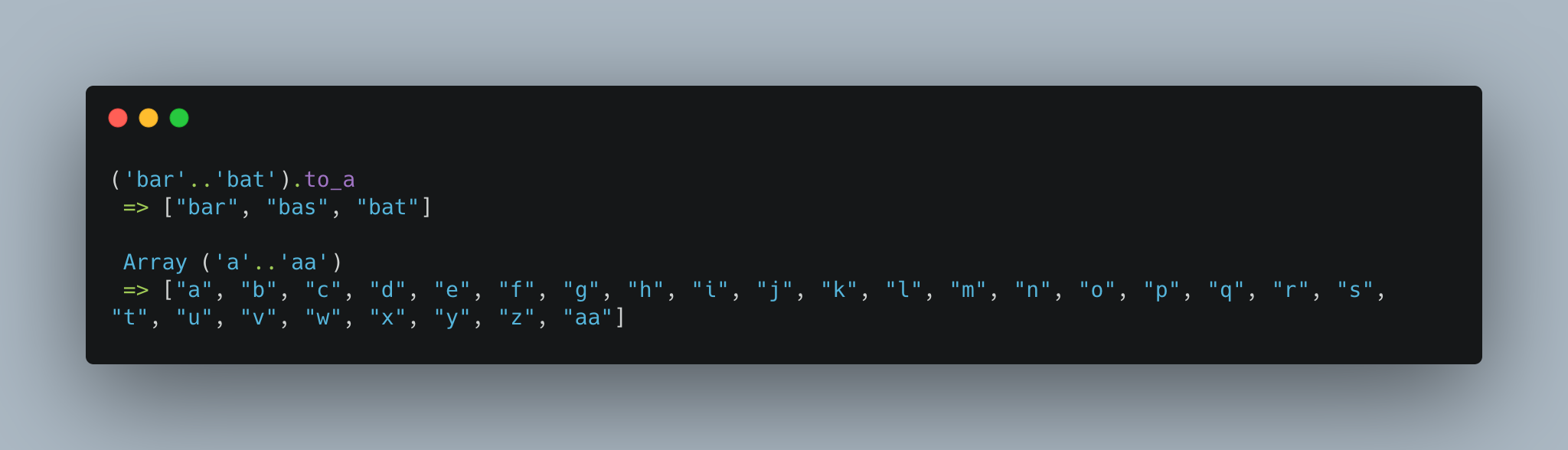
Now, back to our JavaScript range issue. JavaScript does not have a two/three dot notation for creating ranges, however we could use the Ruby examples and create our own. In Ruby, the .to_a
and the Array
methods are built-in methods for creating new Arrays. So our JavaScript code could use the same foundation, and create a new Array to store all our numbers in. Next, using a for
loop, we could loop through the numbers and push them into the array until we reach our higher number and break the loop.
现在,回到我们JavaScript系列问题。 JavaScript没有用于创建范围的二/三点表示法,但是我们可以使用Ruby示例并创建自己的示例。 在Ruby中, .to_a
和Array
方法是用于创建新Array
的内置方法。 因此,我们JavaScript代码可以使用相同的基础,并创建一个新的数组来存储所有数字。接下来,使用for
循环,我们可以遍历数字并将其推入数组,直到达到更高的数字并打破循环。
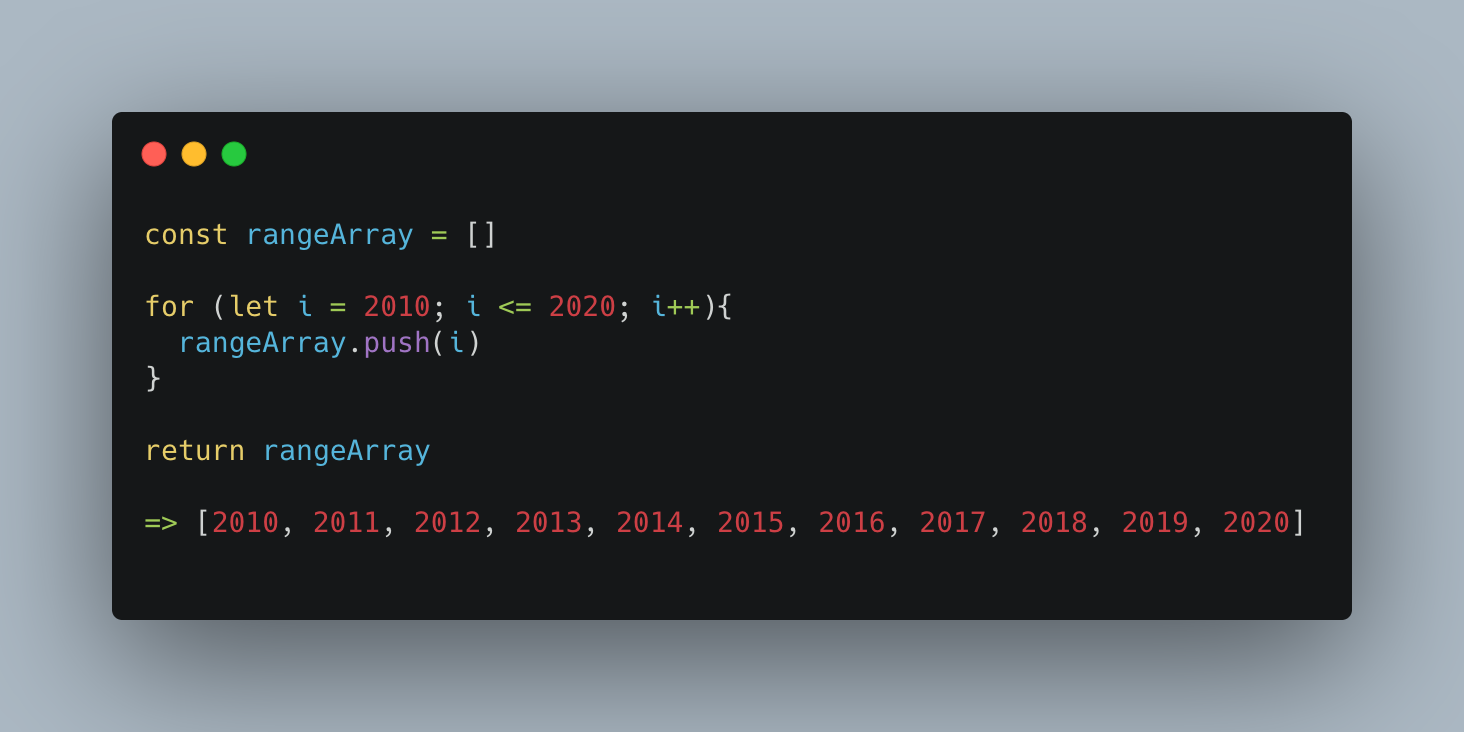
If we wanted to make our JavaScript code act like a Ruby exclusive range, our second argument in the for
loop would change to i < 2020
. While this is great, our use for continuously returning a range from 2010 to 2020 is rather limited. Our next change would be to write this to accept arguments, so that the code could perform a variety of tasks, and not just for this past decade. Writing this to be more dynamic would involve making a function that takes in two arguments for our range numbers.
如果我们想使我们JavaScript代码像Ruby专用范围那样工作,则for
循环中的第二个参数将更改为i < 2020
。 虽然这很棒,但我们用于连续返回2010年至2020年范围的用途非常有限。 我们的下一个更改是将其编写为接受参数,以便代码可以执行各种任务,而不仅仅是过去十年。 要使其更具动态性,则需要编写一个函数,该函数为我们的范围号接受两个参数。
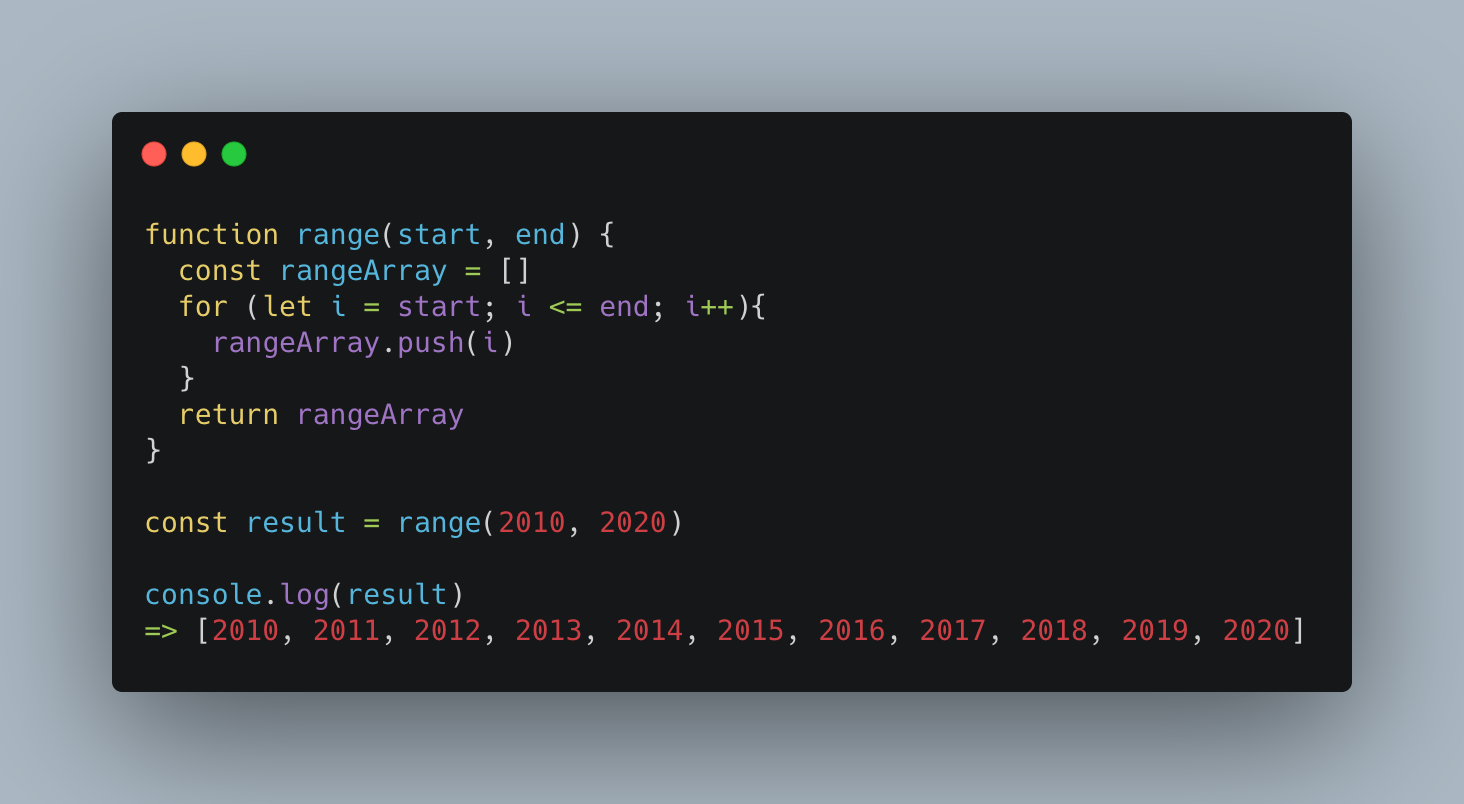
In the above code, 2010
was changed to start
and 2020
to end
, allowing us to create ranges for whichever numbers we need. Now, our JavaScript range function is complete! And only a few more lines of code than our Ruby code…
在上述代码中,将2010
更改为start
,将2020
更改为end
,从而使我们能够为所需的数字创建范围。 现在,我们JavaScript范围功能已经完成! 而且仅比我们的Ruby代码多几行代码…
Now, with our code above it super easy to read and understand what is happening. So let’s change it up a bit and make it more concise and complicated:
现在,有了上面的代码,超级容易阅读和理解正在发生的事情。 因此,让我们对其进行一些修改,使其更加简洁和复杂:
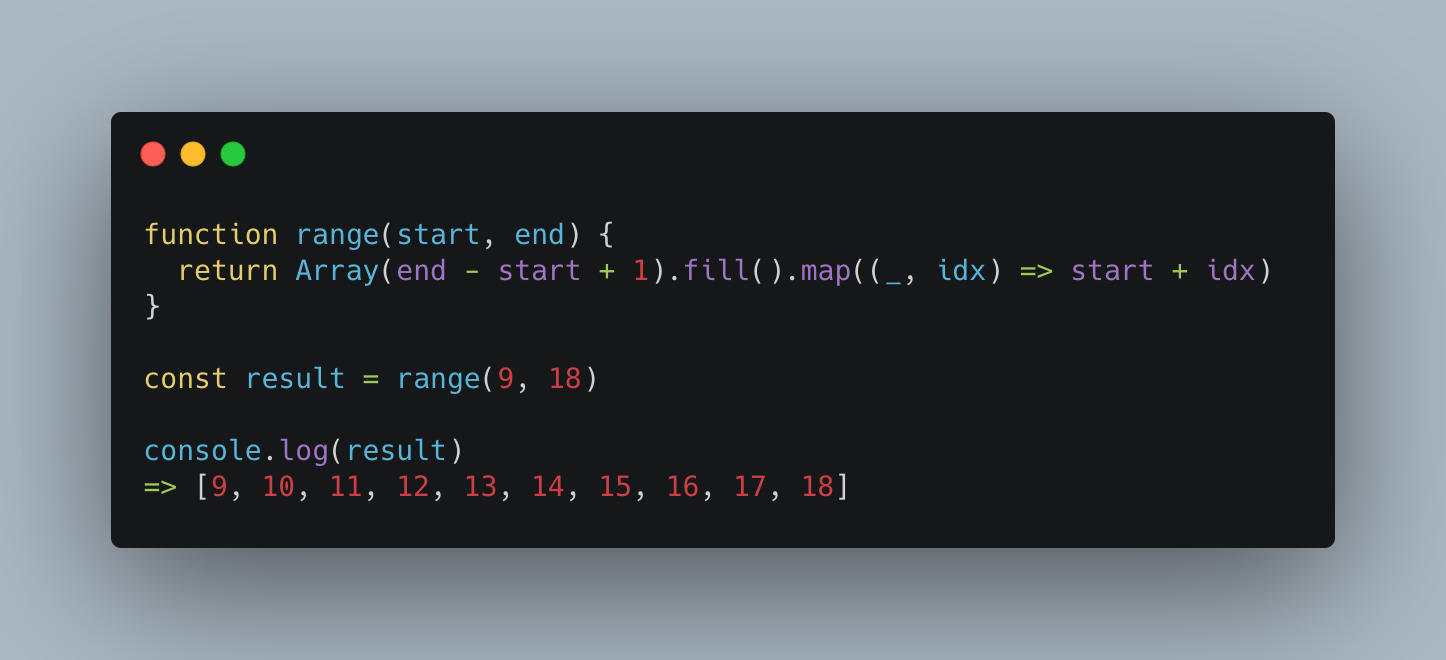
Above, instead of creating a new array, using a for
loop to iterate through our numbers, and pushing those numbers into the array to be returned once the loop is complete, we did the same process in one line.
上面我们没有创建一个新的数组,而是使用for
循环遍历我们的数字,并将这些数字推入数组中,以便在循环完成后返回,而我们在一行中执行了相同的过程。
Using the Array constructor, we specified the length of the return array as (end — start + 1)
. The newly created Array will have the correct length property, but nothing is actually in it. Trying to run .map() won’t work because there’s nothing to iterate over despite the length being the correct value.
使用Array构造函数,我们将返回数组的长度指定为(end — start + 1)
。 新创建的数组将具有正确的length属性,但实际上没有任何内容。 尝试运行.map()无效,因为尽管长度是正确的值,也没有要迭代的内容。
To make the array contain elements we call the .fill() on it. Without any parameters it populates all the values of the array’s length, turning it from a weird empty array with a length to an array whose individual elements are each undefined
. This means we can iterate over them with map.
为了使数组包含元素,我们称为。 在其上填充 ()。 没有任何参数,它将填充数组长度的所有值,将其从具有长度的奇怪的空数组变为其各个元素均未undefined
的数组。 这意味着我们可以使用map遍历它们。
Lastly, .map() takes in a callback function which takes up to 3 parameters (previous value, index, and the original array) and fills in the array with the return value for each value. In our example, we use the callback function with the first two parameters because we need to use the index of the array for addition (which we cannot access the second parameter unless the first parameter is stated in the callback). Using a placeholder for the previous value _
, we completely ignore it and for each element in the array, we add the elements index to the start
argument.
最后, .map ()接收一个回调函数,该函数最多包含 3个参数(先前值,索引和原始数组),并使用每个值的返回值填充该数组。 在我们的示例中,我们将回调函数与前两个参数一起使用,因为我们需要使用数组的索引进行加法运算(除非在回调函数中声明了第一个参数,否则我们无法访问第二个参数)。 对上一个值_
使用占位符,我们将完全忽略它,并且对于数组中的每个元素,我们将元素索引添加到start
参数。
In the example above, our code substituted with numbers would like like this: return Array(18 — 9 + 1).fill().map((_, index) => [9 + 0], [9 + 1], [9 + 2] ... [9 + 8], [9 + 9]))
在上面的示例中,我们的代码用数字替换是这样的: return Array(18 — 9 + 1).fill().map((_, index) => [9 + 0], [9 + 1], [9 + 2] ... [9 + 8], [9 + 9]))
翻译自: https://medium.com/swlh/creating-a-range-in-javascript-e2303e05979b