JavaScript has a handful of methods to help search and filter Arrays. The methods vary depending upon if you would like to search using an item
or a predicate
as well as whether you need to return the item(s)
, index
, or a Boolean
.
JavaScript有一些方法可以帮助搜索和过滤数组。 这些方法取决于您是否要使用item
或predicate
进行搜索以及是否需要返回item(s)
, index
或Boolean
。
视频 (Video)
If you prefer to consume this content in video form, then this is for you.
如果您喜欢以视频形式使用此内容,那么这是给您的。
什么是谓词? (What is a Predicate?)
A predicate
is just a fancy word for a function that takes an item
and returns a Boolean
whether the item
passes some condition.
predicate
只是一个函数的奇特词,该函数接受一个item
并在item
是否通过某些条件时返回Boolean
。
The isEven
function is an example of a predicate
that takes a number and returns true
if the number is even.
isEven
函数是predicate
一个示例,该predicate
采用数字,如果数字为偶数,则返回true
。
const isEven = number => number % 2 === 0
搜索多个项目 (Searching for Multiple Items)
。过滤 (.filter)
takes a predicate
and returns an Array of all matching items.
接受一个predicate
并返回所有匹配项的数组。
const numbers = [1, 2, 3, 4, 5]
const isEven = number => number % 2 === 0
const isLessThanZero = number => number < 0numbers.filter(isEven) //=> [2, 4]
numbers.filter(isLessThanZero) //=> []
每个 (.every)
also searches for multiple items, see implementation below.
还搜索多个项目,请参见下面的实现。
查找第一个匹配项 (Find First Matching Item)
。找 (.find)
takes a predicate
and returns the first matching item or undefined
.
接受predicate
并返回第一个匹配项或undefined
。
const numbers = [1, 2, 3, 4, 5]
const isEven = number => number % 2 === 0
const isLessThanZero = number => number < 0numbers.find(isEven) //=> 2
numbers.find(isLessThanZero) //=> undefined
查找匹配项的索引 (Find The Index of Matching Item)
.findIndex (.findIndex)
takes a predicate
and returns the Index or -1
.
接受一个predicate
并返回Index或-1
。
const numbers = [1, 2, 3, 4, 5]
const isEven = number => number % 2 === 0
const isLessThanZero = number => number < 0numbers.findIndex(isEven) //=> 1
numbers.findIndex(isLessThanZero) //=> -1
。指数 (.indexOf)
takes an item
(and optional start
) and returns the Index or -1
.
接受一个item
(和可选的start
)并返回Index或-1
。
const numbers = [1, 2, 3, 4, 5]numbers.indexOf(2) //=> 1
numbers.indexOf(9) //=> -1
.lastIndexOf (.lastIndexOf)
same as indexOf
, but searches in reverse.
与indexOf
相同,但反向搜索。
如果存在则返回一个布尔值 (Return a Boolean if Exists)
Sometimes you only need to know whether or not items match.
有时,您只需要知道项目是否匹配即可。
.includes (.includes)
takes an item
and returns true
if all items match.
接受一个item
,如果所有项目都匹配,则返回true
。
const numbers = [1, 2, 3, 4, 5]numbers.includes(2) //=> true
numbers.includes(9) //=> false
每个 (.every)
takes a predicate
and returns true
if all items match.
接受一个predicate
,如果所有项目都匹配,则返回true
。
const numbers = [1, 2, 3, 4, 5]
const isEven = number => number % 2 === 0
const isGreaterThanZero = number => number > 0numbers.every(isEven) //=> false
numbers.every(isGreaterThanZero) //=> true
。一些 (.some)
takes a predicate
and returns true
if at least one items matches.
接受一个predicate
,如果至少一项匹配,则返回true
。
const numbers = [1, 2, 3, 4, 5]
const isEven = number => number % 2 === 0
const isLessThanThanZero = number => number < 0numbers.some(isEven) //=> true
numbers.some(isLessThanThanZero) //=> false
额外信用 (Extra Credit)
Some of the predicates
also include extra information like the index
and the array
. Read the documentation for each method to discover all of these features.
一些predicates
还包括额外信息,例如index
和array
。 阅读每种方法的文档以发现所有这些功能。
Example:
例:
const numbers = [1, 2, 3, 4, 5]numbers.filter((item, index, array) => {
/* code here */
})
摘要 (Summary)
JavaScript contains many different methods for searching Arrays. Some search for explicit items, while others use a predicate
to match. Some methods return items, while others may return an index or a Boolean.
JavaScript包含许多用于搜索数组的不同方法。 一些搜索显式项目,而另一些使用predicate
进行匹配。 一些方法返回项目,而其他方法则可能返回索引或布尔值。
Be sure to subscribe for more videos like this!
请务必订阅更多这样的视频!
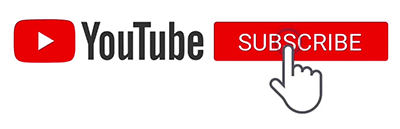
使用Bit共享和管理可重复使用的React组件 (Share & Manage Reusable React Components with Bit)
Use Bit (Github) to share, document, and manage reusable components from different projects. It’s a great way to increase code reuse, speed up development, and build apps that scale.
使用Bit ( Github )共享,记录和管理来自不同项目的可重用组件。 这是增加代码重用,加速开发并构建可扩展应用程序的好方法。
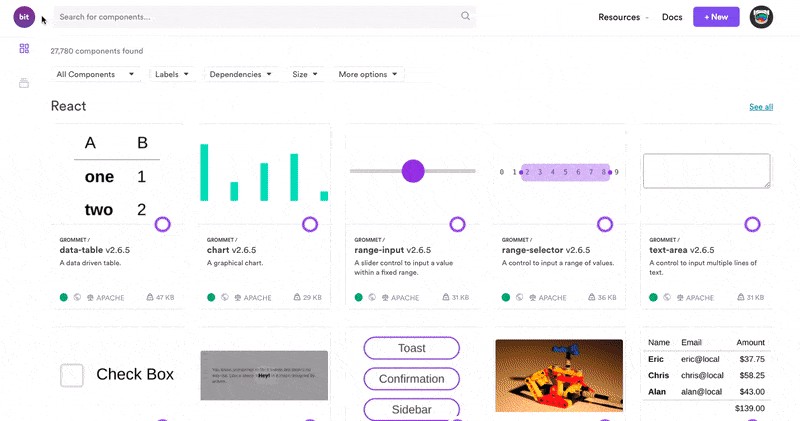
翻译自: https://blog.bitsrc.io/8-methods-to-search-javascript-arrays-fadbce8bea51