python中map函数
介绍(Introduction)
Map, Filter, and Reduce are typical examples of functional programming. They allow us to write simpler, shorter code, without bothering about loops and branching.
Map,Filter和Reduce是功能编程的典型示例。 它们使我们可以编写更简单,更短的代码,而不必担心循环和分支。
In this tutorial we will see how to use the built-in map and filter functions in python.
在本教程中,我们将看到如何在python中使用内置的map和filter函数。
Map comes built-in with Python (in the __builtins__ module) and need not be imported. Now let’s get a better understanding of how map works.
Map是Python内置的(在__builtins__模块中),不需要导入。 现在,让我们更好地了解地图的工作原理。
地图功能 (Map Function)
The map() function has the following syntax:
map()函数具有以下语法:
map(function, *iterables)
map(function, *iterables)
Where function is the function on which each element in iterables (as many as they are) would be applied on. The asterisk(*) means there can be as many iterables as possible, function has that exact number as required input arguments. Also, the number of arguments to function must be the number of iterables listed.
其中function是可迭代元素中的每个元素(尽可能多)将应用于其上的函数。 星号(*)表示可以有尽可能多的可迭代项,函数具有与所需输入参数相同的确切数字。 同样,函数的参数数必须是列出的可迭代数。
Let’s take a look at few examples to get a better understanding:
让我们看一些示例以更好地理解:
If I have a list of names (which is the iterable here), and all of which are in upper case and I need to convert them to lower case. In normal python programming, it would be something like this:
如果我有一个名称列表(在这里是可迭代的),并且所有名称都是大写的,则需要将它们转换为小写。 在普通的python编程中,将是这样的:
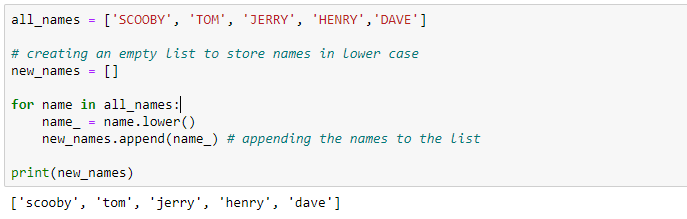
Now let’s see how we could do the same by using map function:
现在,让我们看看如何通过使用map函数来做到这一点:

Using map() syntax above,where function is str.lower and iterables is the list all_names — just one iterable. Also note that we did not call the str.lower function (as: str.upper), as the map function does that for us on each element in our list.
使用上面的map()语法,其中all.names列表是str.lower函数,而iterables是仅可迭代的列表。 还要注意,我们没有调用str.lower函数(如str.upper ),因为map函数在列表中的每个元素上都为我们做到了。
Please note that in this eg., str.lower function requires only one argument by definition and so we passed just one iterable to it. So, if the function you're passing requires two, or three, or n arguments, then you need to pass in two, three or n iterables to it. Lets take a look at another example to understand this :
请注意,在此示例中, str.lower函数根据定义仅需要一个参数,因此我们仅向其传递了一个可迭代的参数。 因此,如果要传递的函数需要两个,三个或n个参数,则需要向其传递两个,三个或n个可迭代对象。 让我们看另一个例子来理解这一点:
If I have a list of numbers containing having four decimal places. And I need to round each element in the list up to its position decimal places, i.e I want to round up the first element in the list to one decimal place, the second element to two decimal places, the third to three decimal places, etc. With map() this is very easy. Let's see how.
如果我有一个包含四个小数位的数字列表。 我需要将列表中的每个元素四舍五入到小数点后一位的位置,即,我想将列表中的第一个元素四舍五入到一个小数位,第二个元素四舍五入到两个小数位,第三个到第三个小数位等等使用map(),这非常容易。 让我们看看如何。

Here, the round() function that takes two arguments -- the number to round up and the number of decimal places to round the number up to. So, since the function requires two arguments, we need to pass in two iterables.
在这里, round()函数需要两个参数-要向上取整的数字和要向上取整的小数位数。 因此,由于该函数需要两个参数,因此我们需要传入两个可迭代对象。
The range(1,7) function acts as the second argument to the round() function. So as map iterates through the list deci_numbers, in the first iteration, the first element of the list, 7.156783 is passed along with the first element of range(1,7), 1 to round, making it effectively become round(7.156783,1). During the second iteration, the second element of the list which is 4.546689 along with the second element of range(1,7), 2 is passed to round making it translate to round(4.546689,2). This will continue until the end of the list is reached.
range(1,7)函数充当round()函数的第二个参数。 因此,当map遍历列表deci_numbers时,在第一次迭代中,将列表的第一个元素7.156783与range(1,7)的第一个元素(即1)传递给round,从而使其有效地变为round(7.156783,1 ) 。 在第二次迭代期间,列表的第二个元素4.546689和range(1,7),2的第二个元素一起传递到round,使其转换为round(4.546689,2) 。 这将一直持续到列表末尾。
Now in case you change the range to (1,4), result = list(map(round,deci_numbers, range(1,4))), you won't get any error even as the length of deci_numbers and the length of range(1,4) differ. Instead, this is what happens: It takes the first element of deci_numbers and the first element of range(1,4) and passes it to round. round evaluates it then saves the result. Then it goes on to the second iteration, takes the second element in the list and the second element of range(1,4), round saves it again. This goes on till the fourth iteration. In the fourth iteration, it tries to take the fourth element of range(1,4) but since range(1,4) does not have a fourth element, Python simply stops and returns the result, which in this case would simply be [7.2,4.55,15.009].
现在,如果您将范围更改为(1,4),结果= list(map(round(deci_numbers,range(1,4)))) ,则即使deci_numbers的长度和的长度也不会出现任何错误。 range(1,4)不同。 相反,这是发生的情况:它接受deci_numbers的第一个元素和range(1,4)的第一个元素并将其传递给round。 一轮求值,然后保存结果。 然后继续进行第二次迭代,获取列表中的第二个元素和range(1,4)的第二个元素,回合将其再次保存。 这一直持续到第四次迭代。 在第四次迭代中,它尝试采用range(1,4)的第四个元素,但是由于range(1,4)没有第四个元素,Python只是停止并返回结果,在这种情况下,其结果将是[ 7.2,4.55,15.009] 。
Using lambda with map function:
将lambda与地图功能配合使用:

Notice here that using lambda and map, I didn’t even need to create a function using the def my_function(). That's how flexible map() is!
请注意,在这里使用lambda和map甚至不需要使用def my_function()创建函数。 那就是map()的灵活度!
结论 (Conclusion)
In this tutorial, with the help of few examples we learnt how to use the built-in map functions in python.
在本教程中,借助几个示例,我们学习了如何在python中使用内置的map函数。
翻译自: https://medium.com/swlh/map-function-in-python-36d9d91cb4ec
python中map函数