react 右键
When working with any type of list in React, you will often encounter this warning if you forgot to include a key
prop:
在React中使用任何类型的列表时,如果您忘记包含key
道具,则经常会遇到此警告:
Warning: Each child in a list should have a unique "key" prop
So, why does React tell you to include keys and why is it important?
那么,为什么React会告诉您包括键,为什么它很重要?
In this article, I’ll be explaining the underlying concept of React keys, why they matter, and how to use them correctly. Let’s jump in!
在本文中,我将解释React密钥的基本概念,它们为何重要以及如何正确使用它们。 让我们跳进去吧!
什么是React键? (What Are React Keys?)
Simply put, they are props that are passed in child elements of a list in order to:
简而言之,它们是在列表的子元素中传递的道具,以便:
- Identify which elements are added. 确定要添加的元素。
- Identify which elements are updated. 确定要更新的元素。
- Identify which elements are removed. 确定要删除的元素。
Hence, keys serve as identification for an element just like how passports are used to identify people.
因此,就像护照如何用来识别人一样,钥匙可以作为元素的识别。
为什么我们需要它们? (Why Do We Need Them?)
At this point, you might be wondering why we need keys. After all, we could identify elements by their ID, className, parent/child, index, props, etc. The answer is because of React’s Diffing Algorithm.
在这一点上,您可能想知道为什么我们需要钥匙。 毕竟,我们可以通过元素的ID,className,父/子,索引,道具等来标识元素。答案是因为React的Diffing Algorithm 。
扩散算法:简要说明 (Diffing Algorithm: A Brief Explanation)
A React app is made up of a tree of components. Whenever there’s a prop or state change in any component, React re-renders its components into its virtual DOM. The diffing algorithm compares the new virtual DOM with the old DOM at each level of the component tree, starting from the root node.
React应用由一棵组件树组成。 每当任何组件中的属性或状态发生变化时,React都会将其组件重新呈现到其虚拟DOM中。 差异算法从根节点开始在组件树的每个级别将新的虚拟DOM与旧的DOM进行比较。
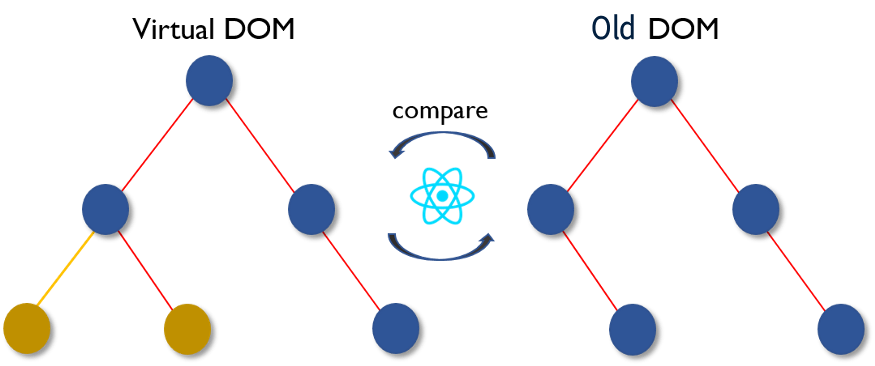
The algorithm finds the minimum number of operations required to update the real DOM. This is how it does it:
该算法找到更新实际DOM所需的最少操作数。 这是怎么做的:
1.按类型比较节点(即<div>
与<span>
) (1. Compare node by types (i.e. <div>
vs. <span>
))
If they are different, destroy and build a new component from scratch.
如果它们不同,则从头开始销毁并构建一个新组件。
// virtual DOM
<div><MyComponent/></div>// real DOM
<span><MyComponent/></span>
In this case, this results in <MyComponent/>
being destroyed and rebuilt.
在这种情况下,这会导致<MyComponent/>
被销毁并重建。
2.如果节点具有相同的类型,则按属性进行比较 (2. If nodes have the same type, compare by attributes)
If they are different, only update the attributes.
如果它们不同,则仅更新属性。
// virtual DOM
<div className="after" title="stuff" />// real DOM
<div className="before" title="stuff" />
In this case, this results in an update of className
to after
.
在这种情况下,这导致将className
更新为after
。
列表呢? (What About Lists?)
For lists, React will recurse on both their children simultaneously, find any differences, then patch them to the real DOM if there are any.
对于列表,React会同时递归两个孩子,找到任何差异,然后将它们修补到真实的DOM(如果有)。
// virtual DOM
<ul>
<li>first</li>
<li>second</li>
<li>third</li>
</ul>
// real DOM
<ul>
<li>first</li>
<li>second</li>
</ul>
In this case, this results in the <li>third</li>
being added after <li>second</li>
.
在这种情况下,这会导致在<li>second</li>
之后添加<li>third</li>
<li>second</li>
。
So far, so good. But now, instead of adding an element at the bottom of the list, what if we add a new element at the beginning?
到现在为止还挺好。 但是现在,如果不在列表底部添加元素,而是在开始处添加新元素呢?
// virtual DOM
<ul>
<li>zero</li>
<li>first</li>
<li>second</li>
</ul>
// real DOM
<ul>
<li>first</li>
<li>second</li>
</ul>
This example will result in React re-rendering every single <li>
to the real DOM because it doesn't realize that it can simply add <li>zero</li>
to the beginning of the list.
这个示例将导致React将每个<li>
重新呈现为真实DOM,因为它没有意识到它可以简单地将<li>zero</li>
到列表的开头。
This inefficiency can cause problems, especially in larger apps. Hence, keys provide a simple solution to this issue.
这种低效率可能会导致问题,尤其是在大型应用程序中。 因此,密钥为该问题提供了一种简单的解决方案。
正确使用密钥的方法:id (The Right Way to Use Keys: id)
You can easily add keys to list elements like so:
您可以轻松地将键添加到列表元素,如下所示:
<li key="1">first</li>
<li key="2">second</li>
<li key="3">third</li>
Keys should be a unique identifier so that each element can be identified properly. Therefore, it is recommended to use some uniquely generated id
as the key. You can even assign keys to a dynamically rendered list:
键应该是唯一的标识符,以便可以正确识别每个元素。 因此,建议使用一些唯一生成的id
作为密钥。 您甚至可以将键分配给动态呈现的列表:
const todoItems = todos.map((todo) =>
<li key={todo.id}>
{todo.text}
</li>
);
错误的键使用方式:索引 (The Wrong Way to Use Keys: index)
Using index
as your key will result in issues when certain changes are made to the list. See this demo I made to illustrate the issue.
当对列表进行某些更改时,使用index
作为键将导致问题。 请参阅我制作的演示来说明问题。
Notice that when adding a new element to the student list, the Notes
property is not mapped correctly because the list’s index is the key.
请注意,将新元素添加到学生列表时, Notes
属性未正确映射,因为列表的索引是键。
As a result, the property’s values (greenroots
and mycodinghabits
) will always be at index 0 and 1, even if their corresponding list elements have changed their position in the list.
结果,该属性的值( greenroots
和mycodinghabits
)将始终位于索引0和1,即使它们的相应列表元素已更改其在列表中的位置。
Let’s see what it looks like if we use Student ID
as the key instead.
让我们来看看它是什么样子,如果我们使用Student ID
作为键来代替。
Here’s a side-by-side comparison.
这是一个并行的比较。
结论 (Conclusion)
The main purpose of keys is to help React differentiate and distinguish elements from each other, increasing its performance when diffing between the virtual and real DOM. To use keys, simply add the prop inside an element such as <li>
.
密钥的主要目的是帮助React在元素之间进行区分和区分,从而在区分虚拟DOM和真实DOM时提高其性能。 要使用键,只需将prop添加到元素中,例如<li>
。
Unique IDs are the best value to assign to keys. You can only use index
as the key if the list is static (cannot be changed) and the elements in it have no id
property.
唯一ID是分配给键的最佳值。 如果列表是静态的(无法更改)并且列表中的元素没有id
属性,则只能将index
用作键。
Thank you for reading. I hope this article has been useful. Please comment below if you have any questions.
感谢您的阅读。 希望本文对您有所帮助。 如有任何疑问,请在下面评论。
翻译自: https://medium.com/better-programming/why-react-keys-matter-an-introduction-136b7447cefc
react 右键