react中使用构建缓存
第0章—资源(Chapter 0 — Resources)
Full Course: Electron & React JS: Build Native Chat App with Javascript
全课程: Electron&React JS:使用Javascript构建本机聊天应用程序
Youtube Guide: https://youtu.be/VCl8li22mrA
YouTube指南: https : //youtu.be/VCl8li22mrA
Github Repo: https://github.com/Jerga99/electron-react-boilerplate
Github回购: https : //github.com/Jerga99/electron-react-boilerplate
第1章-什么是Electron JS? (Chapter 1 — What is Electron JS?)
In short? Electron JS is a framework for creating native applications with web technologies like Javascript, Html & CSS. Yes — you heard that right, you can use HTML to create awesome native applications that can run across multiple platforms including macOS, Windows, and Linux. Many popular applications like Visual Studio Code, Slack, Discord, Twitch, and WhatsApp are created in Electron.
简而言之? Electron JS是一个使用Java,Html和CSS等网络技术创建本机应用程序的框架。 是的-没错,您可以使用HTML创建可在多种平台(包括macOS,Windows和Linux)上运行的出色本机应用程序。 在Electron中创建了许多流行的应用程序,例如Visual Studio Code,Slack,Discord,Twitch和WhatsApp。
那么它是怎样工作的? (So how does it work?)
First, open your coding editors and get ready for programming. Let’s create a very simple application. We will start with coding and then look at the theoretical part.
首先,打开您的编码编辑器,准备进行编程。 让我们创建一个非常简单的应用程序。 我们将从编码开始,然后看理论部分。
- Create an empty folder for your application 为您的应用程序创建一个空文件夹
Initialize npm inside of this folder, run:
npm init -y
在此文件夹中初始化npm,运行:
npm init -y
Install electron, run:
npm install --save-dev electron
安装电子,运行:
npm install --save-dev electron
Specify start script in package.json to:
"start": electron .
在package.json中将启动脚本指定为:
"start": electron .
Upon running
npm start
Electron will try to run the file specified in themain
option of package.json运行
npm start
Electron将尝试运行package.json的main
选项中指定的文件Specify
"main": main.js
指定
"main": main.js
Create
main.js
with the following content :console.log("Hello World");
创建具有以下内容的
main.js
:console.log("Hello World");
{
"name": "your-electron-app",
"version": "1.0.0",
"description": "",
"main": "main.js",
"devDependencies": {
"electron": "^10.1.2",
},
"scripts": {
"start": "electron .",
},
"author": "",
"license": "ISC"
}
console.log("Hello World");
On npm start
electron will run main.js
file, you should see “Hello World” in the terminal console. One interesting thing here is that the application will keep running and will be loaded in the memory of your computer. It’s up to the user to shut it down. Now we need to create a graphical user interface(GUI) to interact with our application. For this, we will use pure HTML and JS.
在npm start
electron将运行main.js
文件,您应该在终端控制台中看到“ Hello World”。 这里有趣的一件事是该应用程序将继续运行并将被加载到您的计算机内存中。 由用户决定将其关闭。 现在,我们需要创建一个图形用户界面(GUI)与我们的应用程序进行交互。 为此,我们将使用纯HTML和JS。
第二章浏览器窗口 (Chapter 2— Browser Window)
In Electron we recognize two main processes: the Main and Renderer process. The process which runs package.json’s main.js
script is the main process. The main process can create a GUI in the form of a web page. Each “web page” runs its own renderer process. Did I use the term “web page”? Yes, Electron is built on top of the same source code as the Google Chrome browser, called Chromium. Again, let’s code a simple browser window, and then we will get into an explanation.
在Electron中,我们认识到两个主要过程: Main和Renderer过程。 运行package.json的main.js
脚本的过程是主要过程。 主过程可以创建网页形式的GUI。 每个“网页”都运行自己的渲染器过程。 我是否使用过“网页”一词? 是的,Electron是基于与Google Chrome浏览器(称为Chromium)相同的源代码构建的。 再次,让我们编写一个简单的浏览器窗口的代码,然后我们将进行解释。
Open main.js
file, and type the following code:
打开main.js
文件,然后输入以下代码:
const { BrowserWindow, app } = require('electron');
function createWindow() {
const win = new BrowserWindow({
width: 1200,
height: 800,
backgroundColor: "white",
webPreferences: {
nodeIntegration: false,
worldSafeExecuteJavaScript: true,
contextIsolation: true
}
})
win.loadFile('index.html')
}
app.whenReady().then(createWindow);
Function createWindow
will create browser window 1200x800px with white background.
函数createWindow
将创建具有白色背景的1200x800px浏览器窗口。
Normally you would be able to access Electron and Node API in the rendered process (the browser window) but by writing nodeIntegration: false
we are disabling it (which considered a good security practice). We will access these APIs differently.
通常,您可以在呈现的过程(浏览器窗口)中访问Electron和Node API,但是通过编写nodeIntegration: false
我们将其禁用(这是一种很好的安全做法)。 我们将以不同的方式访问这些API。
In normal browsers, web pages usually run in a sandboxed environment and are not allowed access to native resources. Electron users, however, have the power to use Node.js APIs in web pages, allowing lower-level operating system interactions. After the browser window is initialized it tries to run the index.html file. The createWindow
function is then executed when the promise of app.whenReady
is resolved. This will happen as soon as the Electron is fully initialized.
在普通浏览器中,网页通常在沙盒环境中运行,并且不允许访问本机资源。 但是,电子用户可以在网页中使用Node.js API,从而可以进行较低级别的操作系统交互。 浏览器窗口初始化之后,它将尝试运行index.html文件。 解决app.whenReady
的承诺后,将执行createWindow
函数。 一旦电子完全初始化,就会发生这种情况。
The last thing missing is theindex.html
file. Let’s create one:
最后缺少的是index.html
文件。 让我们创建一个:
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8" />
<meta http-equiv="Content-Security-Policy" content="script-src 'self'" />
<title>Electron Chat</title>
</head>
<body>
<div id ="root">
<h1>Hello World</h1>
</div>
</body>
</html>
Just standard HTML displaying Hello world.
只是标准HTML显示Hello world 。
Now let’s run npm start
and this is what you should see:
现在让我们运行npm start
,这是您应该看到的内容:
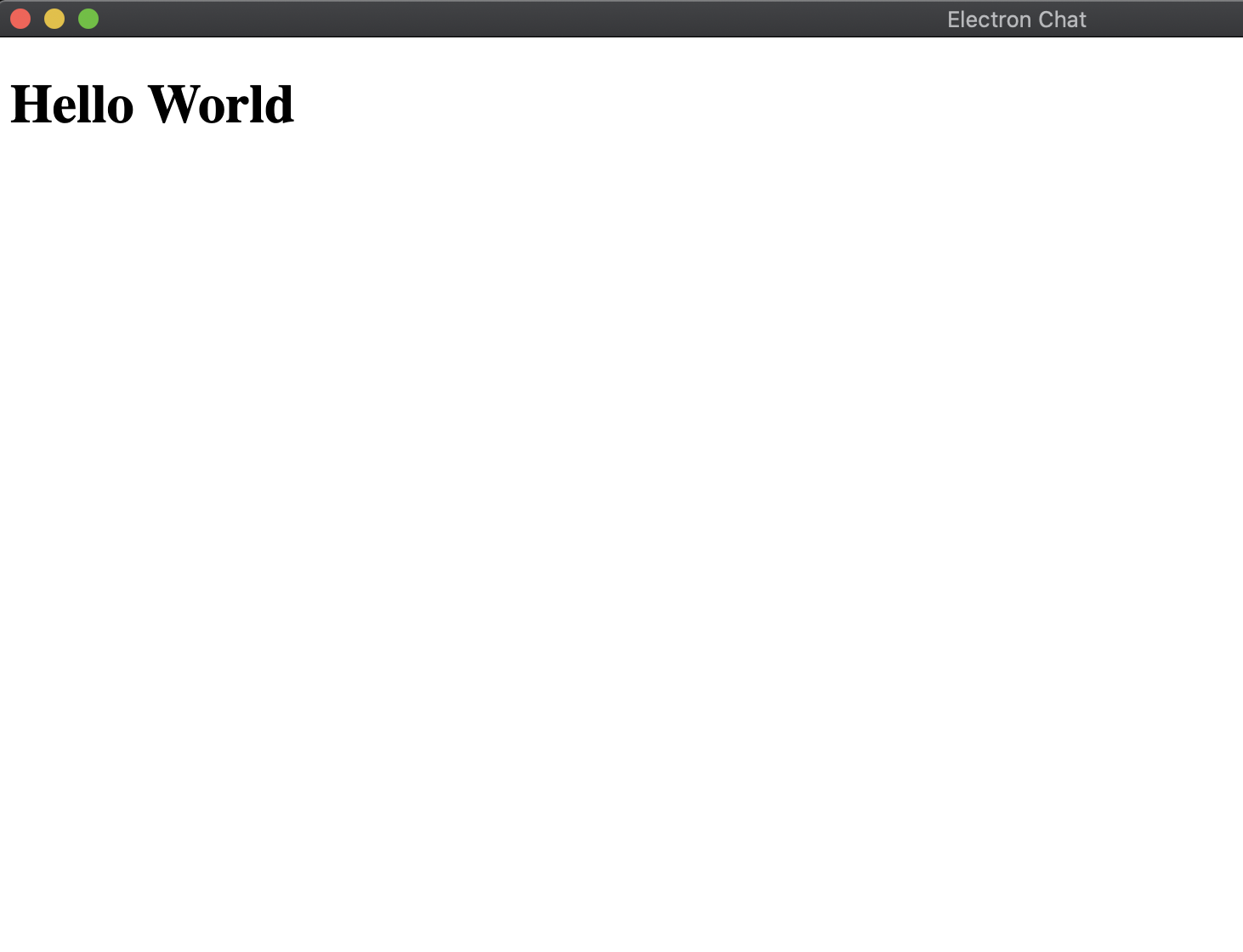
Congrats, you have just created a native application with the use of pure HTML and JS!
恭喜,您刚刚使用纯HTML和JS创建了本机应用程序!
第3章—这是Web应用程序吗? (Chapter 3— Is This a Web App?)
How do you access a normal web page? Typically, you’ll open Google Chrome (or any other browser) and type in the URL of the web page that you want to visit. Let’s say we are visiting medium.com. The browser will make a request to the medium.com server and after some time you will receive a response from the server with an HTML document and usually a bunch of JS, CSS files. The browser will take all of this content, render it on the screen, and the Medium application is displayed.
您如何访问普通网页? 通常,您将打开Google Chrome(或任何其他浏览器),然后输入要访问的网页的URL。 假设我们正在访问medium.com。 的 浏览器将向medium.com服务器发出请求,一段时间后,您将收到来自服务器的响应,其中包含HTML文档以及通常是一堆JS,CSS文件。 浏览器将获取所有这些内容,然后将其呈现在屏幕上,并显示Medium应用程序。
Electron combines all of these steps into one. First, the application is running locally on your computer (the same as Google Chrome). Upon running the Electron app, a browser window is created with the content of the HTML, JS & CSS we have specified to load beforehand. Similar to the Google Chrome browser (which can run natively on your computer), Electron app can do the same.
电子将所有这些步骤组合为一个步骤。 首先,该应用程序在您的计算机上本地运行(与Google Chrome相同)。 运行Electron应用程序后,将创建一个浏览器窗口,其中包含我们指定要预先加载HTML,JS和CSS的内容。 与Google Chrome浏览器(可以在您的计算机上本地运行)相似,Electron应用程序也可以做到这一点。
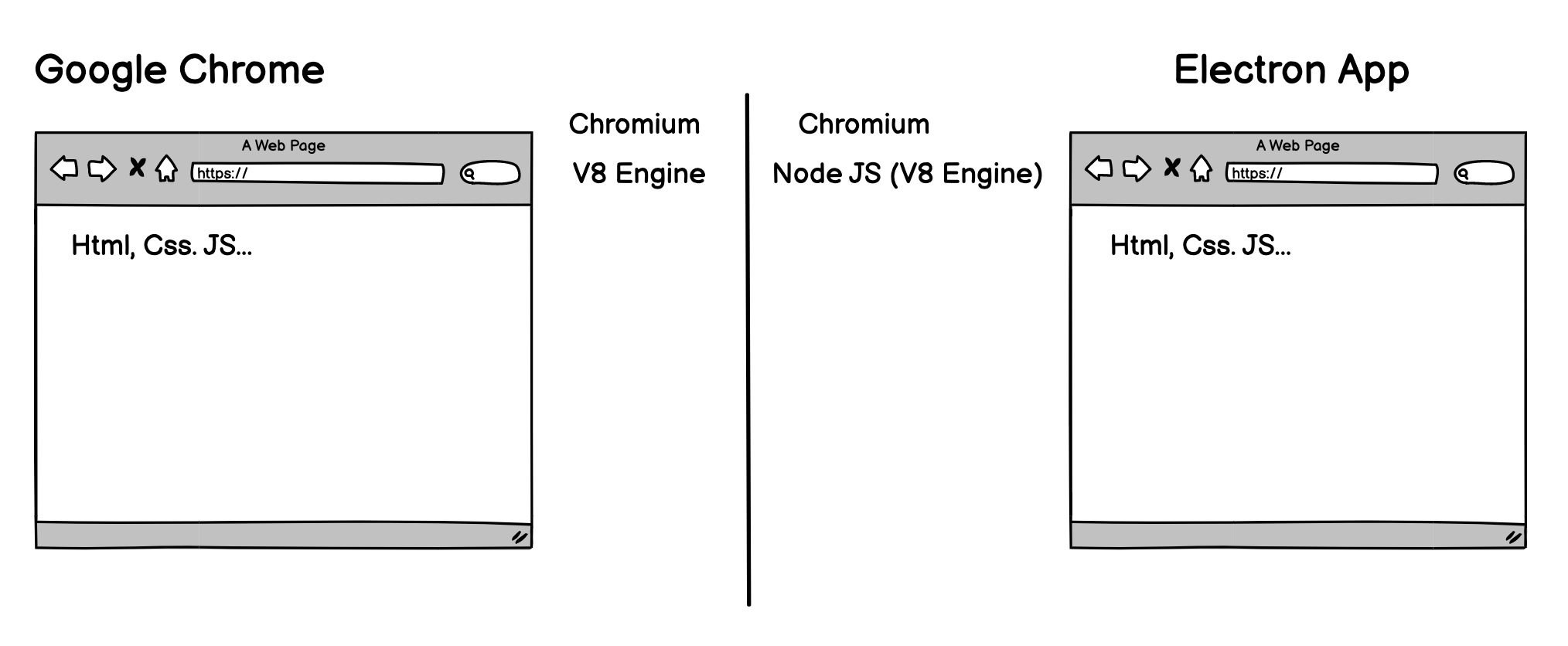
Chrome and Electron have a lot of in common. Chromium is a web engine for rendering the UI — in other words, a full Chrome-like web browser. Chromium can parse HTML, create a DOM tree, render the view, and all of this fancy stuff. That’s why we can use HTML in Electron.
Chrome和电子有很多共同点。 Chromium是用于呈现UI的网络引擎,换句话说,是一个完整的类似Chrome的网络浏览器。 Chromium可以解析HTML,创建DOM树,渲染视图以及所有这些奇特的东西。 这就是为什么我们可以在Electron中使用HTML。
V8 is the name of the JavaScript engine that powers Google Chrome. It’s the thing that takes our JavaScript and executes it while browsing with Chrome. The Electron application uses Node.js and Node.js internally, using a V8 engine. That’s the reason why we can use JS in Electron application + thanks to Node we can access the lower API of our operating system, which is not allowed on a typical web page.
V8是支持Google Chrome浏览器JavaScript引擎的名称。 这是需要我们JavaScript并在使用Chrome浏览器时执行它的东西。 Electron应用程序通过V8引擎在内部使用Node.js和Node.js。 这就是为什么我们可以在Electron应用程序中使用JS的原因+借助Node,我们可以访问操作系统的较低API,这在典型的网页上是不允许的。
那么这是一个网络应用程序吗? (So is this a web app?)
No, but it’s very very similar. What you are creating in Electron is a native application that uses web technologies(HTML, JS, CSS, and so on) with the ability to access “lower” operating system interactions (including the ability to access a file system, power state changes of a computer, and much more). You can create any native application not much different from applications that you use on your computer in your daily life.
不,但是非常相似。 您在Electron中创建的是一个使用Web技术(HTML,JS,CSS等)的本机应用程序,该技术能够访问“较低”的操作系统交互(包括访问文件系统,电源状态更改的能力)。电脑等等)。 您可以创建与本地计算机上使用的应用程序没有太大不同的任何本机应用程序。
结论 (Conclusion)
That’s it for this part of the tutorial. In the next chapter, I will show you how to integrate the React Library into your Electron app. If Electron is something that interests you then feel free to check my full course:
这是本教程的一部分。 在下一章中,我将向您展示如何将React Library集成到您的Electron应用程序中。 如果您对Electron感兴趣,那么可以随时查看我的全部课程:
Full Course: Electron & React JS: Build Native Chat App with Javascript
全课程: Electron&React JS:使用Javascript构建本机聊天应用程序
Youtube Guide: https://youtu.be/VCl8li22mrA
YouTube指南: https : //youtu.be/VCl8li22mrA
Github Repo: https://github.com/Jerga99/electron-react-boilerplate
Github回购: https : //github.com/Jerga99/electron-react-boilerplate
Have a nice day!
祝你今天愉快!
Cheers,
干杯,
Filip
菲利普
react中使用构建缓存