介绍 (Introduction)
Sorting is one of the most basic algorithm types and it appears quite often in interview. Among them, bubble sort is probably the most fundamental one. The naive bubble sort is usually not that efficient, however, it’s important to understand how it works and how it can be optimized.
排序是最基本的算法类型之一,它在面试中经常出现。 其中,气泡排序可能是最基本的一种。 幼稚的气泡排序通常效率不高,但是,了解它的工作方式以及如何对其进行优化非常重要。
天真泡泡排序 (Naive Bubble Sort)
Native bubble sort used a nested loop to iterate through the target array, and swap each pair of adjacent elements if arr[i] < arr[i + 1], so that the largest element always merge to the top(last of the array).
本机冒泡排序使用嵌套循环遍历目标数组,如果arr [i] <arr [i + 1],则交换每对相邻元素,以便最大的元素始终合并到顶部(数组的最后一个) 。
在Python中实现朴素气泡排序 (Implement Naive Bubble Sort in Python)
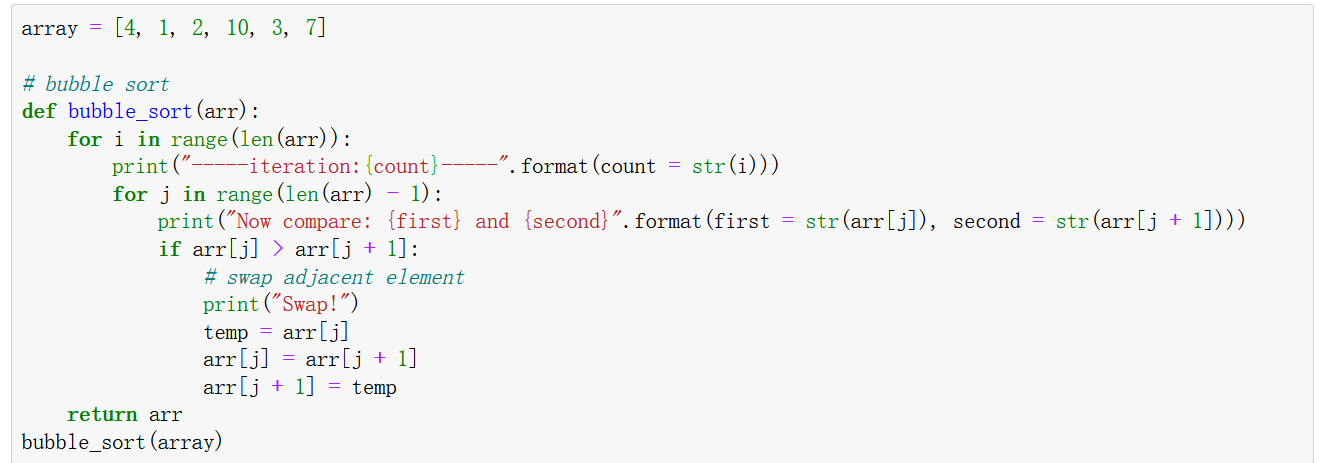
As you’ve seen from the code snippet above, this sorting function operates on the original array instead of creating a new one:
从上面的代码片段中可以看到,此排序功能在原始数组上运行,而不是创建一个新数组:
In the outer loop, we simply iterate through the array while in the inner loop, we compare each pair of element and swap if the first one is larger than the second. Since we already print out some step in the code, now let’s check how the function run own this array:
在外循环中,我们简单地遍历数组,而在内循环中,我们比较每对元素 , 如果第一个 元素 大于第二个 元素 ,则交换。 由于我们已经在代码中打印出了一些步骤,所以现在让我们检查一下该数组如何运行该函数:
-----iteration:0-----
Now compare: 4 and 1
Swap!
Now compare: 4 and 2
Swap!
Now compare: 4 and 10
Now compare: 10 and 3
Swap!
Now compare: 10 and 7
Swap!
-----iteration:1-----
Now compare: 1 and 2
Now compare: 2 and 4
Now compare: 4 and 3
Swap!
Now compare: 4 and 7
Now compare: 7 and 10
-----iteration:2-----
Now compare: 1 and 2
Now compare: 2 and 3
Now compare: 3 and 4
Now compare: 4 and 7
Now compare: 7 and 10
-----iteration:3-----
Now compare: 1 and 2
Now compare: 2 and 3
Now compare: 3 and 4
Now compare: 4 and 7
Now compare: 7 and 10
-----iteration:4-----
Now compare: 1 and 2
Now compare: 2 and 3
Now compare: 3 and 4
Now compare: 4 and 7
Now compare: 7 and 10
-----iteration:5-----
Now compare: 1 and 2
Now compare: 2 and 3
Now compare: 3 and 4
Now compare: 4 and 7
Now compare: 7 and 10
[4, 1, 2, 10, 3, 7]: To sort an array with the length of 6, this function runs 6 iterations and in the end, while all the element is compared and merged to the top (if necessary), the sorting process completed.
[4,1,2,10,3,7]:要对长度为6的数组进行排序,此函数将运行6次迭代,最后,将所有元素进行比较并合并到顶部(如有必要),排序过程完成。
It’s pretty obvious that this bubble sort function has a time complexity of O(n²) since it runs on a nested loop base.
很明显,由于该气泡排序函数在嵌套循环库上运行,因此其时间复杂度为O(n²)。
Is there a way to improve the performance a little bit?
有什么办法可以改善性能吗?
优化气泡排序 (Optimized Bubble Sort)
One way to optimize bubble sort is to skip some of the swaps: If the swaps didn’t occur in an entire iteration, doesn’t that mean all the elements in this array is already in order?
优化冒泡排序的一种方法是跳过某些交换:如果交换不是在整个迭代中发生的,这是否意味着该数组中的所有元素都已经排列好了?
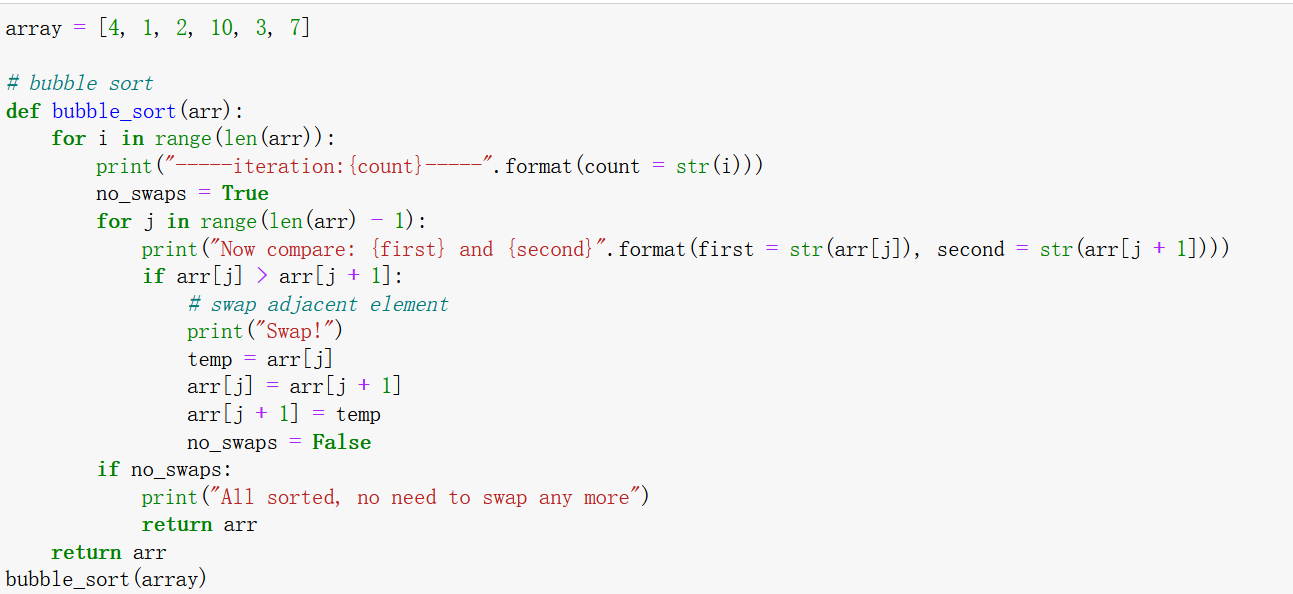
In the optimized version of bubble sort, a flag variable no_swaps is declared, in each iteration, if no swap happens, the function will stop and return the current array.
在冒泡排序的优化版本中,声明了一个标志变量no_swaps,在每次迭代中,如果没有交换发生,该函数将停止并返回当前数组。
Now let’s see how the function run on the same arry:
现在,让我们看看函数如何在相同的位置运行:
-----iteration:0-----
Now compare: 4 and 1
Swap!
Now compare: 4 and 2
Swap!
Now compare: 4 and 10
Now compare: 10 and 3
Swap!
Now compare: 10 and 7
Swap!
-----iteration:1-----
Now compare: 1 and 2
Now compare: 2 and 4
Now compare: 4 and 3
Swap!
Now compare: 4 and 7
Now compare: 7 and 10
-----iteration:2-----
Now compare: 1 and 2
Now compare: 2 and 3
Now compare: 3 and 4
Now compare: 4 and 7
Now compare: 7 and 10
All sorted, no need to swap any more
As you can see from above, it stops in only three iteration as by then the arrya is already sorted.
从上面可以看到,它只停止了三个迭代,此时arrya已被排序。