Even though I’ve been in my coding Bootcamp for only 12 weeks, it feels like it’s been years. The amount of materials that I needed to learn and all the time and effort I’ve put in finally feels like it’s paying off. When I first started boot camp, I didn’t think I’ll be able to become a full-stack developer in 15 weeks, but now that I’m almost at the end of this journey, I’m really proud of what I was able to accomplish and excited about the next step.
即使我在编码训练营中只有12周的时间,但感觉已经好几年了。 最终,我需要学习的材料数量以及我投入的所有时间和精力似乎都正在得到回报。 当我刚开始新手训练营时,我认为15星期内我将无法成为一名全职开发人员,但是现在我快要走完旅程了,我为自己的工作感到自豪能够完成并为下一步感到兴奋。
When I first used an API (with Ruby haha), it was difficult to even understand where the data was coming from and also how to fetch from the URL. Now, I’m able to use the API provided in the public for my advantage and “try” to be creative with the data. For our React project, my partner and I decided to use Google API. It was a little frightening at first when I looked through the documentation, but after some research, I found out that there are so many different NPM(node package modules) that can help me take full advantage of what Google put out there. Hopefully, the steps I took can help some people like me who use Google API for the first time.
当我第一次使用API(使用Ruby haha)时,甚至很难理解数据来自何处以及如何从URL获取。 现在,我可以利用公开提供的API发挥自己的优势,并“尝试”利用数据进行创意。 对于我们的React项目,我和我的合作伙伴决定使用Google API。 刚浏览文档时,这有点令人恐惧,但是经过一番研究,我发现有这么多不同的NPM(节点程序包模块)可以帮助我充分利用Google在其中提供的功能。 希望我采取的步骤可以帮助像我这样的人首次使用Google API。
首先,从Google获取API密钥。 (First, get the API Key from Google.)
At https://console.developers.google.com/apis navigate to Credentials on the tabs and click on + CREATE CREDENTIALS to receive API Key.
在https://console.developers.google.com/apis上,导航至标签上的凭据,然后单击+创建凭据以接收API密钥。
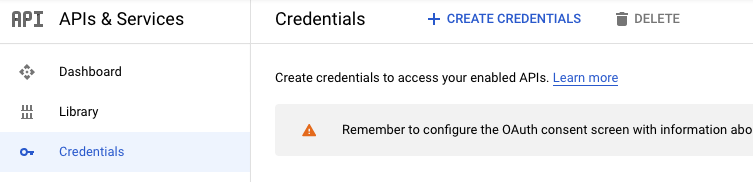
You can use the free API, but there’s also an option to use free trial which is $300 worth of requests for the first 90 days. this gives unlimited access to all Google Cloud Platform.
您可以使用免费的API,但也可以选择使用免费试用版,在开始的90天内,该请求的价值为300美元。 这样就可以无限制地访问所有Google Cloud Platform。
让我们编写代码并渲染地图! (Let’s code and render the map!)
Again, I am using JavaScript and React. Google has so many different libraries, but first I needed to have a map showing up on the Document Object Model(DOM) to work with other libraries. (link for Google Maps).
同样,我正在使用JavaScript和React。 Google有许多不同的库,但是首先我需要在文档对象模型(DOM)上显示一个地图,以便与其他库一起使用。 (Google地图链接) 。
To render Google Maps I used @react-google-maps/api.
为了渲染Google Maps,我使用了@ react-google-maps / api 。
import { GoogleMap, useLoadScript } from '@react-google-maps/api';
After installation, I imported “GoogleMap” and “useLoadScript”.
安装后,我导入了“ GoogleMap”和“ useLoadScript”。
function Map() { const {isLoaded, loadError} = useLoadScript({
googleMapsApiKey: process.env.REACT_APP_GOOGLE_KEY
}); const mapRef = React.useRef();const onMapLoad = React.useCallback((map) => {
mapRef.current = map;
}, []); if(loadError) return "Error";
if(!isLoaded) return "Maps";return (
<GoogleMap mapContainerStyle={containerStyle}
center={center}
zoom={10}
onLoad={onMapLoad}
>
</GoogleMap>
)
}
Basically, using useLoadScript, the Map will be loaded on to the DOM if the googleMapsApi Key is valid. And you have to set up width and height to your map to actually have the map show on your page.
基本上,如果googleMapsApi键有效,则使用useLoadScript将地图加载到DOM上。 而且,您必须为地图设置宽度和高度,才能在页面上实际显示地图。
const containerStyle = {
width: '400px',
height: '400px'
}; // Use any size you want!
Then I also set up the “center” of my map that will show when I first load the map on to the DOM.
然后,我还设置了地图的“中心”,该中心将在我第一次将地图加载到DOM时显示。
const center = {
lat: 40.7128,
lng: -74.0060,
} // This is New York City
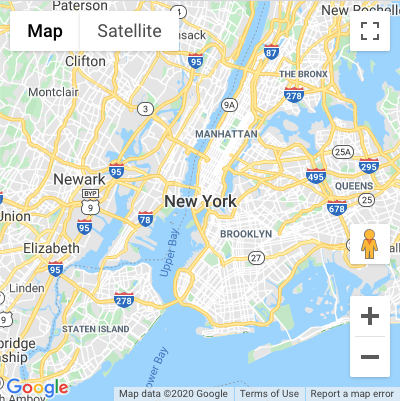
Just like that, Google Maps will be loaded. Pretty simple huh? But hold on, try clicking on the map, something is wrong. Google map usually allows us to place markers when we click on a certain location, but our map isn’t able to do that! It is because we didn’t add any onClick event on our map. This part is relatively simple as well thanks to our npm!
这样,将加载Google Maps。 很简单吧? 但是请稍等,尝试单击地图,这是错误的。 Google地图通常允许我们在单击某个位置时放置标记,但是我们的地图无法做到这一点! 这是因为我们没有在地图上添加任何onClick事件。 由于我们的npm,这部分也相对简单!
让我们编码并渲染标记! (Let’s code and render a marker!)
import { GoogleMap, useLoadScript, Marker } from '@react-google-maps/api';
Now let’s write up some code to make this work!!
现在,让我们编写一些代码来完成这项工作!!
//hook set up mark
const [markers, setMarkers] = React.useState([]);//function that will run when you click
//if you want more than one marker, u can always use spread operator in setMarkers.const onMapClick = React.useCallback((event) => {
setMarkers(() => [{
lat: event.latLng.lat(),
lng: event.latLng.lng(),
time: new Date()
}]);
}, []);//Update your GoogleMap<GoogleMap
mapContainerStyle={containerStyle}
center={center}
zoom={10}
onClick={onMapClick}
onLoad={onMapLoad}
> {markers.map((marker) => (
<Markerkey={marker.time.toISOString()}
position={{lat: marker.lat, lng: marker.lng}}
>
</Marker>
))}
</GoogleMap>
event.latLng.lat() and lng: event.latLng.lng() in our onMapClick is able to locate and set values of latitude and longitude for your marker. I put in time so that I can put a unique key for each Marker. You can style your marker by adding an icon into your Marker component, and also add an onClick event on to the marker.
event .latLng.lat()和lng:onMapClick中的event .latLng.lng()能够找到并设置标记的纬度和经度值。 我投入了时间,以便可以为每个标记放置唯一的键。 您可以通过在标记组件中添加图标来对标记进行样式设置,还可以在标记上添加onClick事件。
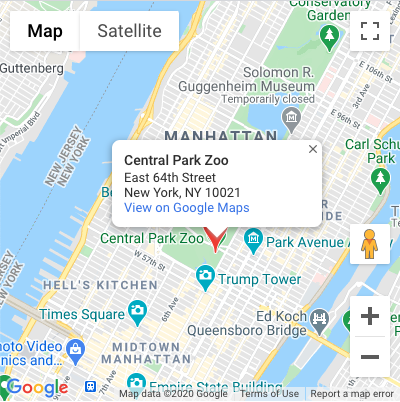
<Markerkey={marker.time.toISOString()}
position={{lat: marker.lat, lng: marker.lng}}
icon={{
url:IMAGE YOU WANT,
scaledSize: new window.google.maps.Size(50,50),
origin: new window.google.maps.Point(0,0),
anchor: new window.google.maps.Point(25,25),
}}
>
</Marker>
Following these steps will allow you to render a map on the DOM and whenever you click a location on the map, it will use its latitude and longitude to place a marker on the map. You will need to use other Google API such as Places, Directions, Routes to add other functions to your app. Hopefully, I can write another blog post about those API.
执行以下步骤将允许您在DOM上呈现地图,并且每当单击地图上的某个位置时,它将使用其纬度和经度在地图上放置标记。 您将需要使用其他Google API(例如位置,路线,路线)将其他功能添加到您的应用中。 希望我能写一篇关于这些API的博客文章。
If you are still struggling to have a map show up on the page follow this youtube guide https://www.youtube.com/channel/UCWPY8W-FAZ2HdDiJp2RC_sQ. He tells you everything you need to know.
如果您仍要在页面上显示地图,请遵循此youtube指南https://www.youtube.com/channel/UCWPY8W-FAZ2HdDiJp2RC_sQ 。 他告诉您您需要知道的一切。
Pricing Information to use Google Maps:https://cloud.google.com/maps-platform/pricing
使用Google Maps的定价信息: https : //cloud.google.com/maps-platform/pricing
翻译自: https://medium.com/weekly-webtips/working-with-google-api-38d57d6a23e4