建筑设计师和程序员
谁应该读这个 (Who Should Read This)
This post is intended for those new to Flutter mobile app development and can create new projects and have a basic understanding of concepts such as Flutter’s Stateful and Stateless widgets.
这篇文章是针对Flutter移动应用程序开发的新手,可以创建新项目,并对Flutter的有状态和无状态小部件等概念有基本的了解。
什么是材料设计 (What is Material Design)
Material design is an approach to design that has successfully unified the best traits of user experience and good design.
材料设计是一种设计方法,已成功地统一了用户体验和良好设计的最佳特征。
Developed by Google in 2014, it promotes a uniform app experience across different platforms. Google’s own products like Keep, Meet and Maps, as well as popular apps like WhatsApp and DropBox, follow Material Design guidelines. This can result in apps with unified and distinct identities.
由Google在2014年开发,可在不同平台上促进统一的应用程序体验。 Google自己的产品(例如Keep,Meet和Maps)以及流行的应用程序(例如WhatsApp和DropBox)均遵循Material Design指南。 这可能会导致应用具有统一且不同的身份。
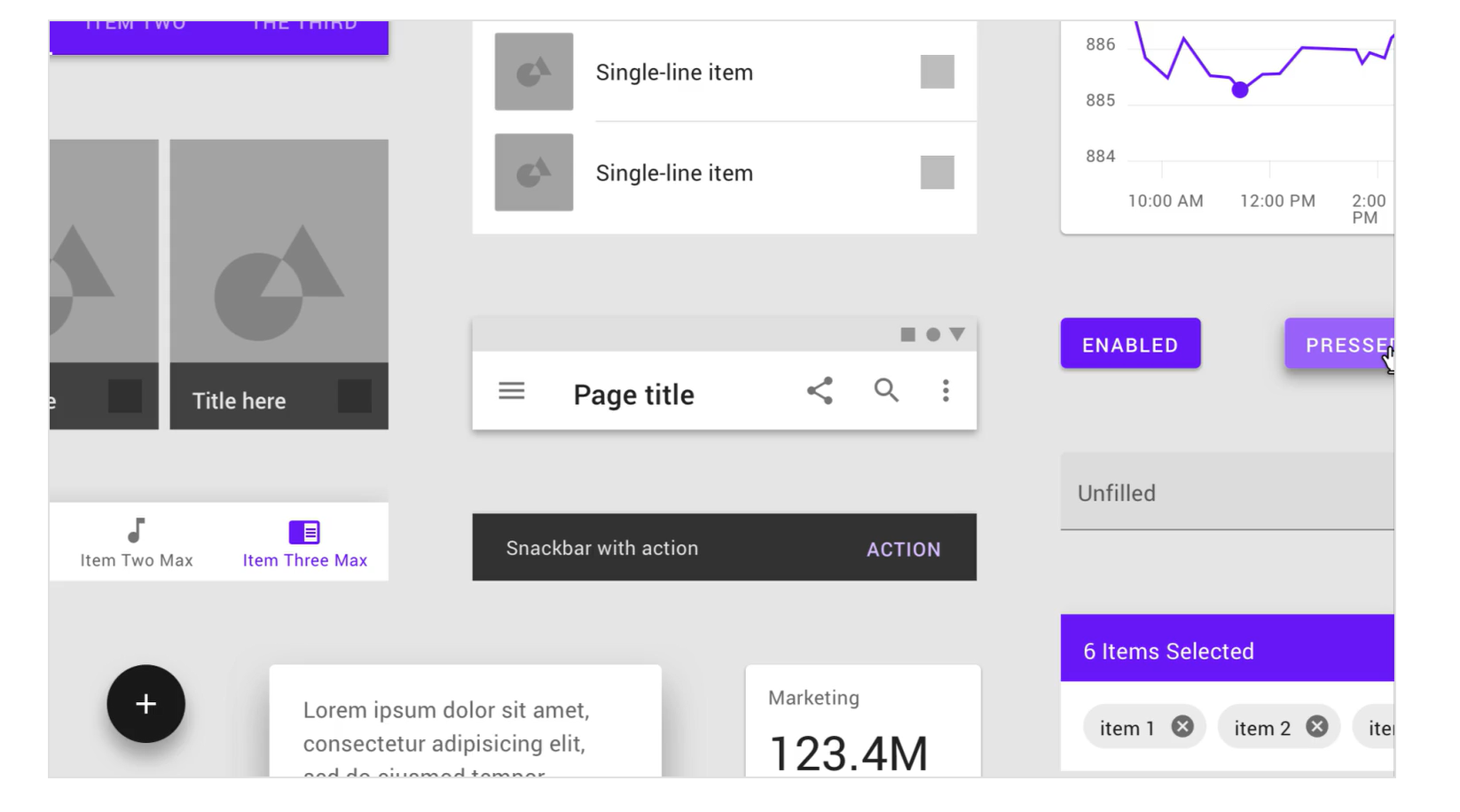
颤振材料库 (Flutter Material Library)
Some of the widgets included in the Flutter Material library are Checkboxes, Radio Buttons, Text Fields, DateTime Pickers and an assortment of Buttons. Standard fare for any UI Framework.
Flutter材质库中包含的一些小部件包括复选框,单选按钮,文本字段,日期时间选择器和各种按钮。 任何UI框架的标准票价。
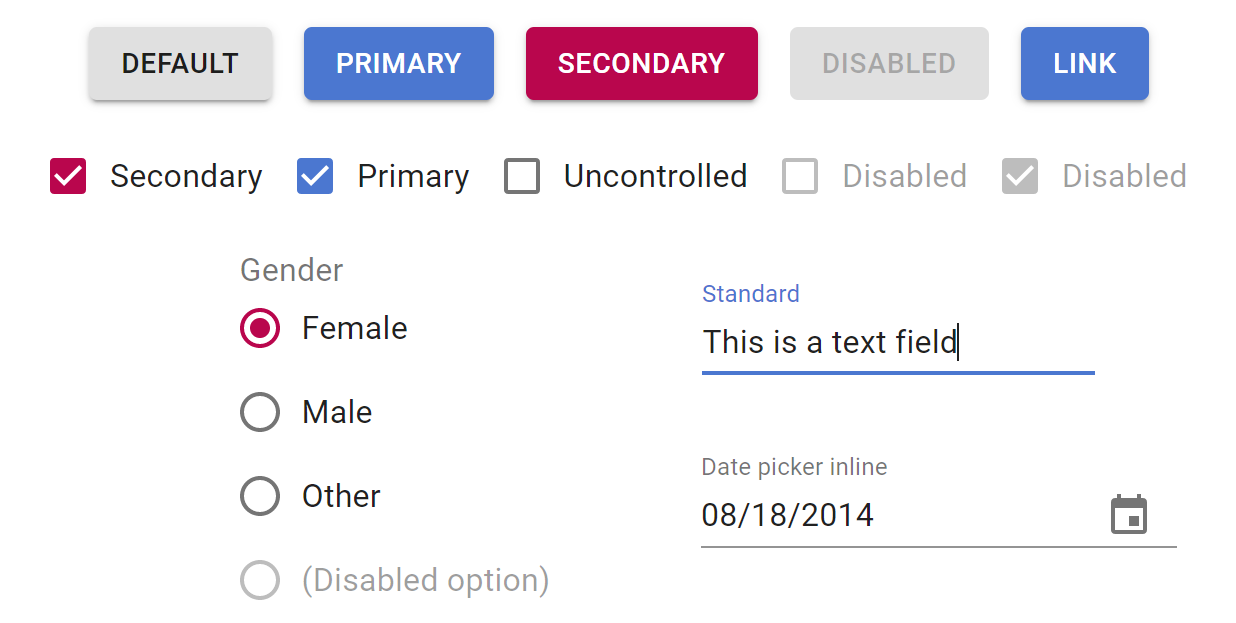
Key to developing apps that follow material design guidelines are the so-called “Convenience” widgets: MaterialApp and Scaffold.
开发遵循材料设计准则的应用程序的关键是所谓的“便捷”小部件: MaterialApp和Scaffold。
创建材质设计应用 (Creating a Material Design App)
Before proceeding with our first Material Design project, we need to become acquainted with the MaterialApp and Scaffold widgets.
在继续第一个Material Design项目之前,我们需要熟悉MaterialApp和Scaffold小部件。
MaterialApp小部件 (MaterialApp Widget)
The MaterialApp widget delivers quite a lot of functionality, with support for themes, navigation, and localisation. Below is an example of a minimal Flutter Material App.
MaterialApp小部件提供了大量功能,并支持主题,导航和本地化。 以下是最小Flutter Material App的示例。
import 'package:flutter/material.dart';
main() => runApp(MyApp());
class MyApp extends StatelessWidget {
@override
Widget build(context) {
return MaterialApp(
home: Scaffold(
body: Center(
child: Text('Hello, Flutter'),
),
),
);
}
}
MaterialApp has many named parameters/properties. We’ll touch on some of those later in the post. For now, we set only the home property, specifying the first screen/widget to display — a Scaffold.
MaterialApp具有许多命名的参数/属性。 我们将在后面的文章中介绍其中一些内容。 现在,我们仅设置home属性,指定要显示的第一个屏幕/小部件-Scaffold 。
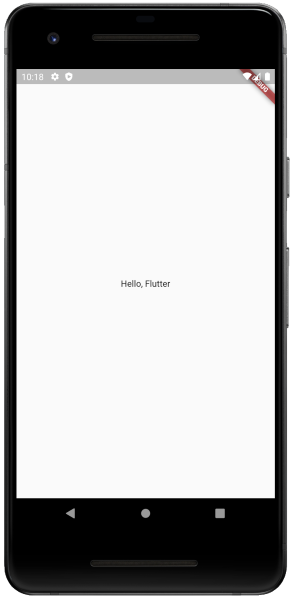
脚手架小部件 (Scaffold Widget)
Something’s missing from our app above. For one, the toolbar that runs across the top is absent. The bottom navigation bar is also missing and one of those floating buttons would spruce things up!
我们上面的应用缺少一些内容。 首先,缺少在顶部运行的工具栏。 底部导航栏也丢失了,其中一个浮动按钮将使事情变得更加精彩!
The Scaffold widget is responsible for implementing this material design visual layout structure and provides an API for showing drawers, snack bars, bottom sheets, app bars and floating buttons.
脚手架小部件负责实现这种材料设计的视觉布局结构,并提供了用于显示抽屉,小吃店,底页,应用栏和浮动按钮的API。
Let’s expand our basic app above to give the Scaffold a white background with a blue AppBar at the top. A blue FloatingActionButton will be positioned at the bottom right corner of the Scaffold.
让我们在上面扩展我们的基本应用程序,为Scaffold提供一个白色背景,并在顶部添加一个蓝色AppBar 。 蓝色的FloatingActionButton将位于支架的右下角。

Create a new Flutter project using VSCode or Flutter CLI and overwrite the file lib/main.dart with the code below. Press F5 to run the app.
使用VSCode或Flutter CLI创建一个新的Flutter项目,并使用以下代码覆盖文件lib / main.dart 。 按F5键运行该应用程序。
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
static const String _title = 'Flutter Code Sample';
@override
Widget build(BuildContext context) {
return MaterialApp(
title: _title,
home: MyStatefulWidget(),
debugShowCheckedModeBanner: false);
}
}
class MyStatefulWidget extends StatefulWidget {
MyStatefulWidget({Key key}) : super(key: key);
@override
_MyStatefulWidgetState createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('To-Do List'), actions: <Widget>[
// action button
IconButton(
icon: Icon(Icons.person),
onPressed: () {
// TODO
},
),
]),
body: Center(child: Text('Log on to view todo items')),
floatingActionButton: FloatingActionButton(
onPressed: () {
// TODO
},
tooltip: 'Add Task',
child: const Icon(Icons.add),
),
);
}
}
An improvement over our first attempt.
对我们的第一次尝试有所改进。
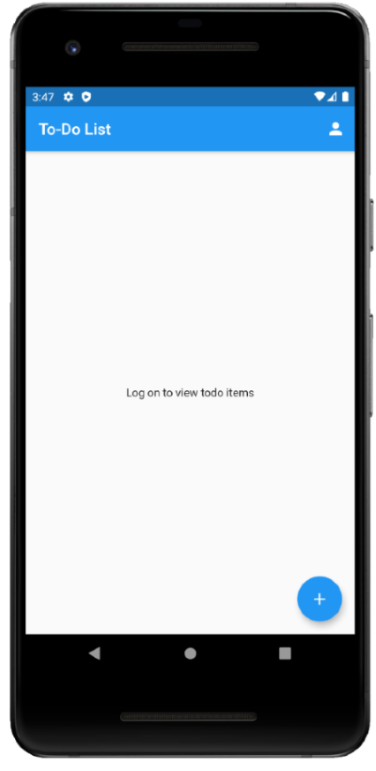
A few things to notice here:
这里需要注意的几件事:
The home parameter of the MaterialApp widget is now assigned a Stateful widget MyStatefulWidget. This widget’s state object defines the Scaffold.
现在为MaterialApp小部件的home参数分配了一个有状态小部件MyStatefulWidget。 这个小部件的状态对象定义了Scaffold 。
The Scaffold widget’s properties appbar, body and floatingActionButton are assigned values. The AppBar widget displays the title of the app using a child Text widget and a single IconButton action widget.
脚手架小部件的属性appbar , body和fatingatingButton被分配了值。 该 AppBar插件播放使用Text子控件和一个IconButton动作插件的应用程序的标题。
The body is assigned a Text widget, placed in a Center widget to centre the text within the Scaffold.
为主体分配了一个文本小部件,该文本小部件放置在“ 中心”小部件中,以使文本在“ 脚手架”中居中。
- The Scaffold widget automatically fills the screen, allowing the Text to be centred vertically and horizontally. 脚手架小部件会自动填充屏幕,使文本可以垂直和水平居中。
The debug banner has been removed by setting the MaterialApp widget’s debugShowCheckedModeBanner parameter to false.
通过将MaterialApp小部件的debugShowCheckedModeBanner参数设置为false,可以删除调试横幅。
- Clicking the floating button and action does not perform any operations…yet 😅 单击浮动按钮并不执行任何操作……operations
让我们导航 (Let’s Navigate)
When the user clicks the login action, we’d like to transition to a new screen. Screen transitions in Flutter are managed by the Navigation widget.
当用户单击登录操作时,我们想要切换到新屏幕。 Flutter中的屏幕过渡由“ 导航”小部件管理。
导航与路线 (Navigation & Routes)
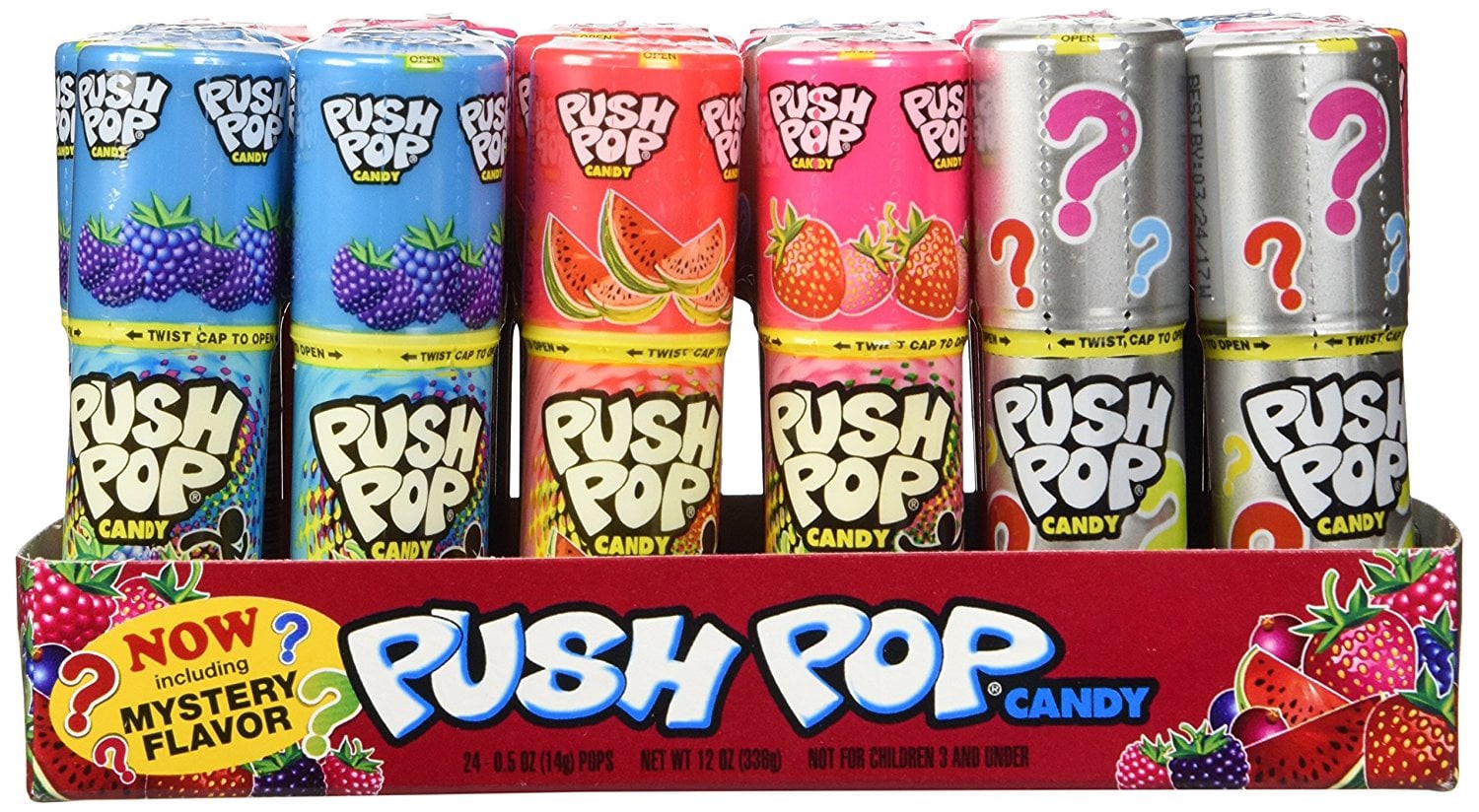
Screens in the Flutter world are called routes. The routes are arranged in a Stack and the Navigator manages the stack using the methods Navigator.push() and Navigator.pop().
Flutter世界中的屏幕称为路线。 路由安排在堆栈中,并且Navigator使用Navigator.push()和Navigator.pop()方法管理堆栈。
导航到另一个屏幕 (Navigating to the Another screen)
Let’s demonstrate the switching from one route to another by building a new screen/widget which we will navigate to when the user clicks the Login action on our AppBar.
让我们通过构建一个新的屏幕/小部件来演示从一条路线到另一条路线的切换,当用户单击AppBar上的Login(登录)操作时,我们将导航到该屏幕/小部件。
import 'package:flutter/material.dart';
void main() => runApp(MyApp());
class MyApp extends StatelessWidget {
static const String _title = 'Flutter Code Sample';
@override
Widget build(BuildContext context) {
return MaterialApp(
title: _title,
home: MyStatefulWidget(),
debugShowCheckedModeBanner: false);
}
}
class MyStatefulWidget extends StatefulWidget {
MyStatefulWidget({Key key}) : super(key: key);
@override
_MyStatefulWidgetState createState() => _MyStatefulWidgetState();
}
class _MyStatefulWidgetState extends State<MyStatefulWidget> {
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: const Text('To-Do List'), actions: <Widget>[
// action button
IconButton(
icon: Icon(Icons.person),
onPressed: () {
Navigator.push(
context,
MaterialPageRoute(builder: (context) => LoginRoute()),
);
},
),
]),
body: Center(child: Text('Log on to view todo items')),
floatingActionButton: FloatingActionButton(
onPressed: () {
// TODO
},
tooltip: 'Add Task',
child: const Icon(Icons.add),
),
);
}
}
class LoginRoute extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(
title: Text("This is the login route"),
),
body: Center(
child: RaisedButton(
onPressed: () {
// Navigate back to first route when tapped.
Navigator.pop(context);
},
child: Text('Go back!'),
),
),
);
}
}
Some important points to note:
需要注意的重要事项:
Line 51: We’ve added a new StatelessWidget called LoginRoute. It displays a button, which, when pressed, will cause the app to navigate back to the home screen
第51行:我们添加了一个名为LoginRoute的新StatelessWidget 。 它显示一个按钮,当按下该按钮时,它将导致该应用导航回到主屏幕
Line 31: The Login action button’s OnPressed callback now switches to a new route, using the navigator.push() method. The push() method requires a route to add to the stack of routes managed by Navigator. Here we’re using MaterialPageRoute to create a new route for our second screen.
第31行:现在,使用navigator.push()方法,Login操作按钮的OnPressed回调将切换到新路由。 push()方法需要一条路由才能添加到Navigator管理的路由堆栈中。 在这里,我们使用MaterialPageRoute为第二个屏幕创建新的路线。
Line 62: Returning to the home screen is a little easier. We simply need to pop the last route off the stack. This is achieved using the pop() method.
第62行:返回主屏幕要容易一些。 我们只需要弹出最后一条路由即可。 这是使用pop()方法实现的。
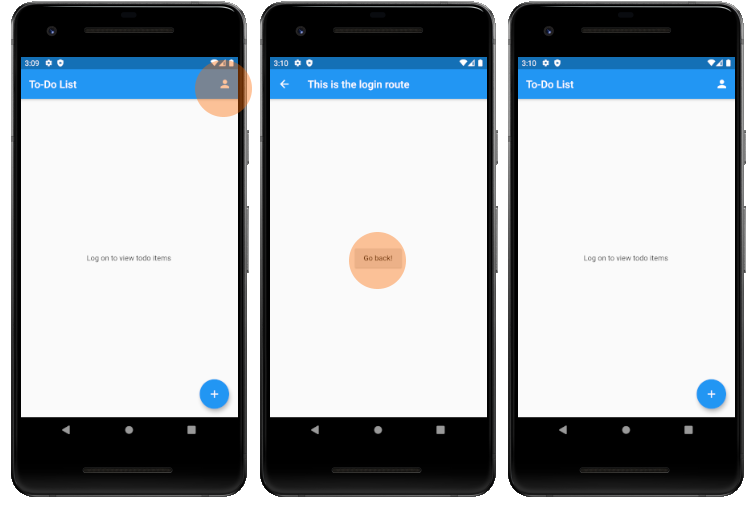
摘要 (Summary)
That concludes our introduction to the Flutter MaterialApp library. We’ve covered how to quickly construct a Material Design themed app using the MaterialApp and Scaffold widgets and utilised the Navigator widget to transition between screens in your Flutter app.
到此结束我们对Flutter MaterialApp库的介绍。 我们已经介绍了如何使用MaterialApp和Scaffold小部件快速构建以Material Design为主题的应用程序,并介绍了如何使用Navigator小部件在Flutter应用程序之间切换屏幕。
翻译自: https://medium.com/@carey.kenneth/building-material-design-apps-in-flutter-fe21298f44e3
建筑设计师和程序员