android 自动化构建

Part 1: https://medium.com/@ehsanulfahad/how-i-automated-android-app-building-process-part-1-f010e4c4c644
第1部分: https : //medium.com/@ehsanulfahad/how-i-automated-android-app-building-process-part-1-f010e4c4c644
Ok, we’ve seen the modifications needed in the app file to automate app building process. In this part, we’re going to do some Python coding to automatically change the properties and then build the app.
好的,我们已经看到了需要在应用文件中进行的修改,以使应用构建过程自动化。 在这一部分中,我们将做一些Python编码来自动更改属性,然后构建应用程序。
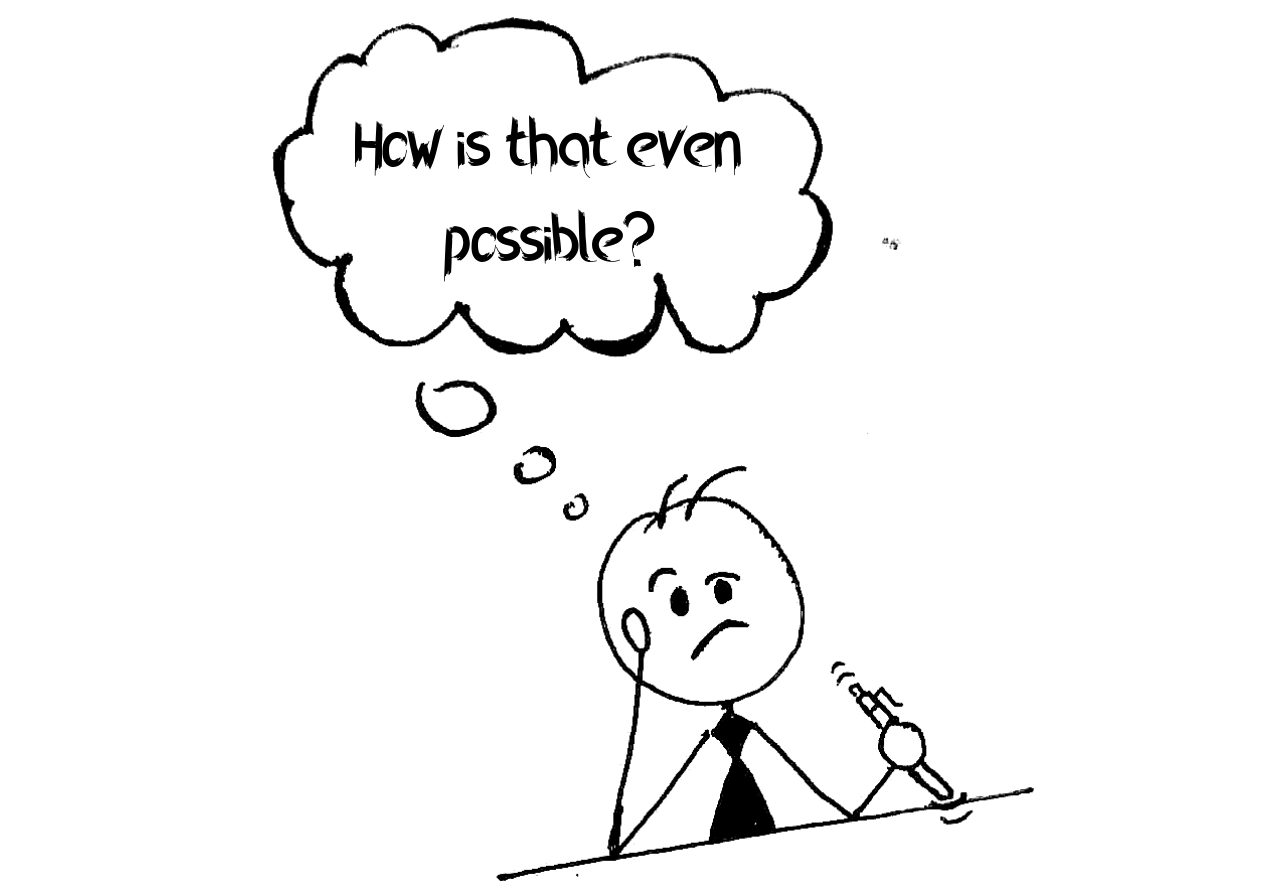
By the way, in my machine, I’m running Python 3.7.5.
顺便说一句,在我的机器上,我正在运行Python 3.7.5 。
We will create one folder and 3 files into the root folder of the project.
我们将在项目的根文件夹中创建一个文件夹和3个文件。

companyInfo.json will contain information about the companies. Typically you would want to get the company info from api or pull from database, but here as we don’t have a database, just use this json file for sample info.
companyInfo.json将包含有关公司的信息。 通常,您可能想从api或从数据库中获取公司信息,但是由于这里没有数据库,只需将此json文件用作示例信息即可。
Open the companyInfo.json and write the following code:
打开companyInfo.json并编写以下代码:
Now open the constants.py and write the following code: (The constant names are pretty much self-explanatory)
现在打开constants.py 并编写以下代码:(常量名称几乎是不言自明的)
Now open the makeApp.py. Create some variables at first.
现在打开makeApp.py 。 首先创建一些变量。
Here, app_properties_keys
is basically used for mapping the properties in gradle.properties file with the keys in the companyInfo.json file.
这里, app_properties_keys
基本上是用于在gradle.properties文件与在companyInfo.json文件中的密钥映射的属性。
Next, we’ll automate the modifications of properties inside the gradle.properties file. For that, let’s make a function.
接下来,我们将自动对gradle.properties文件中的属性进行修改。 为此,让我们做一个函数。
This method reads the gradle.properties file line by line. Here, source_item_dict
is a dictionary object. Why? Because it will contain the company info, and if you look in the companyInfo.json file, you’ll see that every company’s information is kept in individual json objects. And in Python, you can think of json objects as dictionaries. That’s why we used the parameter of the function as a dictionary. We have already mapped the necessary property names in the app_properties_keys
. So we are checking if any line starts with any property name, and if so, we replace the entire line, using the values we get from source_item_dict
. But if any property is not present in the gradle.properties file, then we are not adding it. I didn’t feel the necessity of it. But you can take it as a homework: if any property is not present in the gradle.properties file, add it in the file.
此方法逐行读取gradle.properties文件。 在这里, source_item_dict
是一个字典对象。 为什么? 因为它将包含公司信息,并且如果您查看companyInfo.json文件,则会看到每个公司的信息都保存在单独的json对象中。 在Python中,您可以将json对象视为字典。 这就是为什么我们将函数的参数用作字典的原因。 我们已经在app_properties_keys
映射了必要的属性名称。 因此,我们正在检查是否有任何行以任何属性名称开头,如果是,则使用从source_item_dict
获得的值替换整行。 但是,如果gradle.properties文件中没有任何属性,则我们不会添加它。 我觉得没有必要。 但是您可以将其作为作业:如果gradle.properties文件中没有任何属性,请将其添加到文件中。
Now we’ll automate the creation of keystore file and storing the keystore information in the <keystore>.properties file. We will use keytool
command to create keystore file. Let’s write two more methods.
现在,我们将自动创建密钥库文件,并将密钥库信息存储在<keystore> .properties文件中。 我们将使用keytool
命令创建密钥库文件。 让我们再写两个方法。
We have used subprocess
to run keytool
command in Python. Normally you will need Java installed on your machine for using keytool
. You can check out the official documentation for keytool
to know more about its parameters. process.communicate()
returns a tuple, which contains the output and the error. universal_newlines=True
makes sure that the output and error are both strings. process.returncode
is 0 in case of success, and not 0 in case of failure.
我们已经使用subprocess
keytool
在Python中运行keytool
命令。 通常,您需要在计算机上安装Java才能使用keytool
。 您可以查看keytool
的官方文档以了解有关其参数的更多信息。 process.communicate()
返回一个元组,其中包含输出和错误。 universal_newlines=True
确保输出和错误均为字符串。 如果成功,则process.returncode
为0,如果失败,则为0。
Now we have to download the icon in the drawable folder. It’s actually pretty easy. (Actually you should download multiple sizes of icons in the mipmap folders. But we are doing this just for demonstration purpose.)
现在,我们必须将图标下载到drawable文件夹中。 实际上很简单。 (实际上,您应该在mipmap文件夹中下载多种尺寸的图标。但是我们这样做只是出于演示目的。)
Basically we’ve done all the modifications by now. Now the final thing, we’ve to generate release apk. Actually, we’re going to do some more. We will generate the release apk, rename it, and install it on the connected device. Note that, renaming and installing parts are optional. You can ignore them if you want. And also, installing requires adb
installed on your machine.
到目前为止,基本上我们已经完成了所有修改。 现在最后一件事,我们必须生成发行版apk。 实际上,我们将做更多的事情。 我们将生成发行版APK,对其进行重命名,然后将其安装在连接的设备上。 请注意,重命名和安装部件是可选的。 您可以根据需要忽略它们。 而且,安装需要在计算机上安装adb
。
We’ve used ./gradlew assembleRelease
to generate release apk, and adb install <path_to_apk>
to install on connected device. The code is pretty much self-explanatory.
我们已经使用./gradlew assembleRelease
生成发行版apk,并使用adb install <path_to_apk>
安装在连接的设备上。 该代码几乎是不言自明的。
We have written down the functions, now we have to call them. We have to take necessary inputs first. We will take the inputs from command line arguments, then search for the company in the companyInfo.json file. If found, we will start processing. Let’s see the final part.
我们已经写下了函数,现在我们必须调用它们。 我们必须首先采取必要的投入。 我们将从命令行参数中获取输入,然后在companyInfo.json文件中搜索公司 。 如果找到,我们将开始处理。 让我们看看最后一部分。
Now we are done! Finally!!
现在我们完成了! 最后!!
How to run the program? Open terminal, go to the directory where makeApp.py resides, and call
如何运行程序? 打开终端,转到makeApp.py所在的目录,然后调用
python3 makeApp.py <company_id> <keystore_filename> <key_alias> <keystore_password>
if keystore file exists or does not exist.python3 makeApp.py <company_id> <keystore_filename> <key_alias> <keystore_password>
如果密钥库文件存在或不存在)。python3 makeApp.py <company_id> <keystore_filename>
if keystore file exists.python3 makeApp.py <company_id> <keystore_filename>
如果存在密钥库文件)。
You can get the company_id
from the companyInfo.json. I’ve generated 2 sample apps using the Python code, and here’s the screenshots.
您可以从companyInfo.json获取company_id
。 我已经使用Python代码生成了2个示例应用,这是屏幕截图。

In the screenshots, we see that the app name, package name, theme color and icon all are different.
在屏幕截图中,我们看到应用程序名称,程序包名称,主题颜色和图标都不同。
So this is it! You can clone the sample project from https://github.com/Ehsanul-Hoque/App-Automate/tree/master. Hope it helps. Here’s a happy bug for you! Happy coding!
就是这样! 您可以从https://github.com/Ehsanul-Hoque/App-Automate/tree/master克隆示例项目。 希望能帮助到你。 这是给您的快乐虫子! 编码愉快!

翻译自: https://medium.com/@ehsanulfahad/how-i-automated-android-app-building-process-part-2-7b095469db5e
android 自动化构建