结果 (Result)
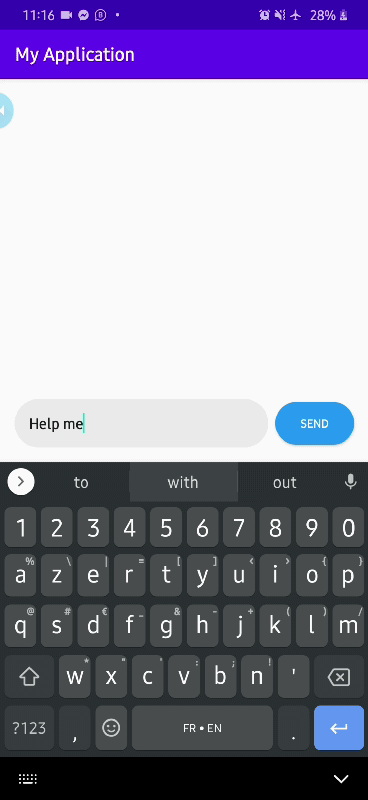
介绍 (Introduction)
Groupie is a simple, flexible library for complex RecyclerView layouts.
Groupie是一个简单,灵活的库,用于复杂的RecyclerView布局。
Groupie lets you treat your content as logical groups and handles change notifications for you — think sections with headers and footers, expandable groups, blocks of vertical columns, and much more. It makes it easy to handle asynchronous content updates and insertions and user-driven content changes. At the item level, it abstracts the boilerplate of item view types, item layouts, viewholders, and span sizes.
Groupie使您可以将内容视为逻辑组,并为您处理更改通知-考虑具有页眉和页脚,可扩展组,垂直列块等等的部分。 它使处理异步内容更新和插入以及用户驱动的内容更改变得容易。 在项目级别,它抽象了项目视图类型,项目布局,视图持有人和跨度大小的样板。
In this article, we will see how to create a small chat interface using the Groupie library that you can find on the following link
在本文中,我们将看到如何使用Groupie库创建一个小型聊天界面,您可以在以下链接中找到该库
组态 (Configuration)
To be able to use groupie, it is necessary to add certain elements in your build.gradle file.
为了能够使用groupie,必须在build.gradle文件中添加某些元素。
- Activate databinding 激活数据绑定
- Activate android extension 激活Android扩展
3. Adding Groupie dependencies
3.添加Groupie依赖项
implementation "com.xwray:groupie:2.7.0"
implementation "com.xwray:groupie-databinding:2.7.0"
implementation "com.xwray:groupie-kotlin-android-extensions:2.7.0"
应用程序用户界面 (Application UI)
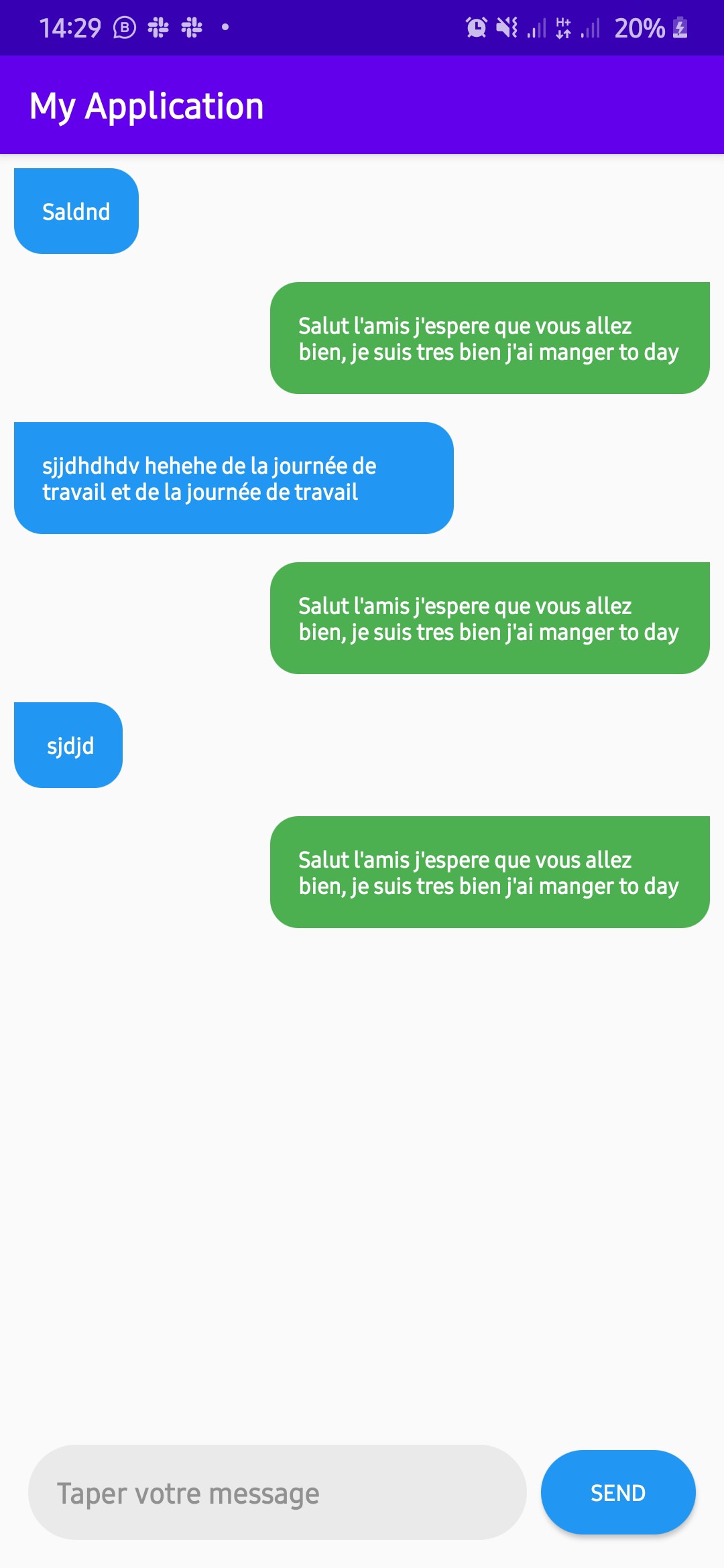
As our project is configured, we now need to create the graphical interface of our application, we will start by creating a layout that will be used to display the send message which is green has the following code.
配置好项目后,我们现在需要创建应用程序的图形界面,首先要创建一个布局,该布局将用于显示绿色的发送消息,其代码如下。
The above code produces the following result
上面的代码产生以下结果

The interface only contains a TextView for which the background attribute is associated with the file bg_receive_message.xml which allowed us to round the borders of the TextView, here is the content of the file bg_receive_message.xml
该界面仅包含一个TextView,其background属性与文件bg_receive_message.xml关联,该文件允许我们舍入TextView的边框,以下是文件bg_receive_message.xml的内容
Just repeat the same procedure to create the second layout that will display the received message or just download the source code of the project at this link.
只需重复相同的过程以创建第二个布局即可显示收到的消息,或者在此链接下载项目的源代码。
When parsing the item_message_send.xml file, you will notice that a message variable of type Message has been declared in it and the content of the text property has been assigned to the application’s unique TextView
解析i tem_message_send.xml文件时,您会注意到其中已声明类型为Message的消息变量,并且text属性的内容已分配给应用程序的唯一TextView
class Message(
val text: String = "",
val sendBy: String = ""
)
The main GUI contains a RecyclerView, a button, and an EditText, the following code defines the components of the GUI.
主GUI包含一个RecyclerView,一个按钮和一个EditText,以下代码定义了GUI的组件。
让我们潜入Groupie (Let dive in Groupie)
With Groupie we don’t need the ViewHolder that we often create when we want to link the data to the RecyclerView, all we need to do is create a class that will represent an element of our RecyclerView and to do that we just have to do something like this
使用Groupie,我们不需要将数据链接到RecyclerView时经常创建的ViewHolder,我们要做的就是创建一个代表RecyclerView元素的类,而要做的就是像这样的东西
In our case, we created two classes, the ReceiveMessageItem class, and SendMessageItem the two classes inherited from the BindableItem class and this supports databinding, that’s why the layout of the item_message_send.xml file supports databinding.
在我们的案例中,我们创建了两个类,分别是ReceiveMessageItem类和SendMessageItem,这两个类都是从BindableItem类继承的,并且这两个类都支持数据绑定,这就是为什么tem_message_send.xml文件的布局支持数据绑定的原因。
The type parameter of the BindableItem class must be a class that inherits from the ViewBinding class, like the ItemMessageSend class generated from the item_message_send.xml file.
BindableItem类的类型参数必须是从ViewBinding类继承的类,例如从item_message_send.xml文件生成的ItemMessageSend类。
The SendMessageItem class has a Message property which is then passed to the layout in the bind method.
SendMessageItem类具有Message属性,然后将其传递给bind方法中的布局。
The getLayout method only returns the id of the relevant layout. In our case, it is the layout item_message_send or item_message_receive in the case of the ItemMessageReceive class.
getLayout方法仅返回相关布局的ID。 在我们的情况下,对于ItemMessageReceive类,它是布局item_message_send或item_message_receive 。
将适配器连接到RecyclerView (Connecting the Adapter to the RecyclerView)
With Groupie we need to create an Adapter for the RecyclerView and even less for the ViewHolder, Groupie offers us directly a GroupAdapter class and a GroupieViewHolder class that we just have to use.
使用Groupie,我们需要为RecyclerView创建一个Adapter,为ViewHolder创建更少的适配器,Groupie直接为我们提供了GroupAdapter类和我们仅需使用的GroupieViewHolder类。
If you notice very well you will see when in MainActivity a property has been created this way.
如果您注意到的很好,您将看到在MainActivity中以这种方式创建属性的时间。
private val messageAdapter = GroupAdapter<GroupieViewHolder>()
Then we use this property as an adaptation of RecyclerView.
然后,我们将此属性用作RecyclerView的改编。
In the populateData method you can notice that depending on the value of the sendBy property you add in the adapter either an object of type SendMessageItem or ReceiveMessageItem.
在populateData方法中,您会注意到,根据您在适配器中添加的sendBy属性的值,可以创建类型为SendMessageItem或ReceiveMessageItem的对象。
And in setOnClickListener we use SendMessageItem and then we call a method that simulates the arrival of a new message using coroutines
在setOnClickListener中,我们使用SendMessageItem ,然后调用使用协程模拟新消息到达的方法
The file project on this link
该链接上的文件项目
If you have any kind of feedback, feel free to connect with me on Twitter.
如果您有任何反馈意见,请随时在 Twitter上 与我联系 。
翻译自: https://medium.com/swlh/android-chat-interface-using-recyclerview-and-groupie-9c1a67e28e3e