神秘面纱
W e should load much of the data from the network or external/internal storage from devices. The best case is that we do not need to wait for fetching data from the external environment. But in most cases, there is no choice. We must wait a while for loading data. While waiting it looks much better to show view skeletons to users instead of showing only a white-empty screen. Because they don’t know if it loading or stopped or whatever.
W e应该从网络或从设备的外部/内部存储中加载许多数据。 最好的情况是我们不需要等待从外部环境中获取数据。 但是在大多数情况下,别无选择。 我们必须等待一段时间才能加载数据。 等待时,向用户显示视图骨架比仅显示白屏更好。 因为他们不知道它是加载还是停止还是其他。
This posting is how to implement masked skeletons and shimmering effects like Facebook or LinkedIn to your layouts and recyclerView on Android using AndroidVeil library.
这篇文章介绍了如何使用AndroidVeil库在Android上的布局和recyclerView上实现蒙版骨架和Facebook或LinkedIn等闪烁效果。
Let’s start!
开始吧!
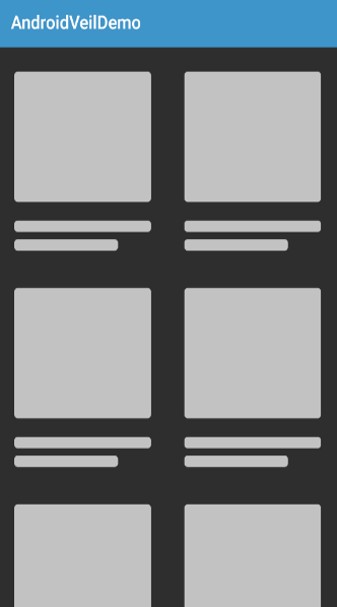
包括在您的项目中 (Including in your project)
Firstly you should add a dependency code to your module’s build.gradle file.
首先,您应该在模块的build.gradle文件中添加一个依赖代码。
dependencies {
implementation “com.github.skydoves:androidveil:1.0.8”
}
The version could be different from the latest release.If you want to get the latest version, you can check it here.
该版本可能与最新版本不同。如果要获取最新版本,可以在此处进行检查。
如何使用 (How to use)
Here is a basic example of implementing a shimmering effect and skeleton to your view or viewGroup in your layout using VeilLayout.
这是一个使用VeilLayout对布局中的view或viewGroup实现闪烁效果和骨架的基本示例。
<com.skydoves.androidveil.VeilLayout
xmlns:app="http://schemas.android.com/apk/res-auto"
android:id="@+id/veilLayout"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:veilLayout_veiled="true" // shows veils initially
app:veilLayout_shimmerEnable="true" // sets shimmer enable
app:veilLayout_baseColor="@android:color/holo_green_dark" // sets shimmer base color
app:veilLayout_highlightColor="@android:color/holo_green_light" // sets shimmer highlight color
app:veilLayout_baseAlpha="0.6" // sets shimmer base alpha value
app:veilLayout_highlightAlpha="1.0" // sets shimmer highlight alpha value
app:veilLayout_dropOff="0.5"// sets how quickly the shimmer`s gradient drops-off
app:veilLayout_radius="6dp" // sets a corner radius of the whole veiled items >
<TextView
android:layout_width="match_parent"
android:layout_height="wrap_content"
android:text="And now here is my secret, a very simple secret"
android:textColor="@android:color/white"
android:textSize="22sp"/>
<!-- Any Views or ViewGroups -->
</com.skydoves.androidveil.VeilLayout>
The VeilLayout can wrap any views or viewGroups and it will make skeletons based on the wrapped view hierarchy and give shimmering effects. The basic principle is the VeilLayout will traverse the wrapped view hierarchy and make masked skeleton layout based on the child view’s width/height sizes and position.
VeilLayout可以包装任何视图或viewGroups,它将基于包装的视图层次结构制作骨架并产生闪烁效果。 基本原理是VeilLayout将遍历包装的视图层次结构,并根据子视图的宽度/高度大小和位置进行蒙版骨架布局。
If we want to wrap a layout resource, we can implement veiled skeletons like below.
如果我们想包装一个布局资源,我们可以像下面这样实现遮遮掩掩的骨架。
veilLayout.setLayout(R.layout.layout_item_test)
And we can change the skeleton color, shimmering color, alpha, dropOff and the radius of the view skeleton using attributes.
我们可以使用属性更改骨架颜色,闪烁颜色,alpha,dropOff和视图骨架的半径。
面纱和面纱 (Veil and UnVeil)
It is really easy to use. Just call veil() and unVeil() functionalities.
真的很容易使用。 只需调用veil()和unVeil()功能即可。
veilLayout.veil()
veilLayout.unVeil()
We can call the veil() method before calling the network request or something costly works. After finish something works, we should call the unVeil() method to reveal the real natural layout which we want to show.
我们可以在调用网络请求之前调用veil()方法,否则可能会花费很多钱。 完成某些工作后,我们应该调用unVeil()方法来显示我们想要显示的真实自然布局。
Here is an example of using Glide and VeilLayout in the ViewHolder (RecyclerView). This case is, every ViewHolder item has an imageView that should be loaded by Glide. And we want to make a veil effect before loading the image and unveil after finish the loading.
这是在ViewHolder(RecyclerView)中使用Glide和VeilLayout的示例。 在这种情况下,每个ViewHolder项都有一个应由Glide加载的imageView。 我们希望在加载图像之前制作面纱效果,并在加载完成后显示。
itemView.run {
item_user_veilLayout.veil()
Glide.with(context)
.load(githubUser.avatar_url)
.apply(RequestOptions().circleCrop().override(100).placeholder(R.drawable.ic_account))
.listener(object : RequestListener<Drawable> {
override fun onLoadFailed(e: GlideException?, model: Any?, target: Target<Drawable>?, isFirstResource: Boolean): Boolean {
item_user_veilLayout.unVeil()
return false
}
override fun onResourceReady(resource: Drawable?, model: Any?, target: Target<Drawable>?, dataSource: DataSource?, isFirstResource: Boolean): Boolean {
item_user_veilLayout.unVeil()
return false
}
})
.into(item_user_avatar)
}
The Glide provides a listener functionality that listens to success or failure to load an image. So we can use it with the VeilLayout. Just call veil() method initially in the onBindViewHolder
method. And after we could know the status of the image is loaded (success or failure), we should call unVeil() method. You can reference some examples to use of the VeilLayout in the ViewHolder on this repository GitHubFollows.
Glide提供了侦听器功能,可侦听加载映像的成功或失败。 因此,我们可以将其与VeilLayout一起使用。 最初仅在onBindViewHolder
方法中调用veil()方法。 在我们知道图像的状态已加载(成功或失败)之后,我们应该调用unVeil()方法。 您可以在此存储库GitHubFollows上的ViewHolder 中引用一些使用VeilLayout 的 示例 。
VeilRecyclerFrameView (VeilRecyclerFrameView)
Here is an easier way to implement skeletons and shimmering effects to the RecyclerView using the VeilRecyclerFrameView. It is almost similar to use the VeilLayout. We should use VeilRecyclerFameView instead of the RecyclerView in our XML layout file.
这是使用VeilRecyclerFrameView对RecyclerView实施骨架和闪烁效果的一种更简单的方法。 使用VeilLayout几乎类似。 我们应该在XML布局文件中使用VeilRecyclerFameView而不是RecyclerView 。
<com.skydoves.androidveil.VeilRecyclerFrameView
android:id="@+id/veilRecyclerView"
android:layout_width="match_parent"
android:layout_height="match_parent"
app:veilFrame_layout="@layout/item_profile" // sets to make veiling target layout
app:veilFrame_veiled="true" // shows veils initially
app:veilFrame_shimmerEnable="true" // sets shimmer enable
app:veilFrame_baseColor="@android:color/holo_green_dark" // sets shimmer base color
app:veilFrame_highlightColor="@android:color/holo_green_light" // sets shimmer highlight color
app:veilFrame_baseAlpha="0.6" // sets shimmer base alpha value
app:veilFrame_highlightAlpha="1.0" // sets shimmer highlight alpha value
app:veilFrame_radius="8dp" // sets a corner radius of the whole veiled items />
And we can bind our RecyclerView.Adapter and LayoutManager to the like VeilRecyclerFameView below.
并且我们可以将我们的RecyclerView.Adapter和LayoutManager绑定到下面的类似VeilRecyclerFameView 。
veilRecyclerView.setAdapter(adapter) // sets your own adapter
veilRecyclerView.setLayoutManager(LinearLayoutManager(this)) // sets LayoutManager
Now add as many fake views (masked skeleton views) to the VeilRecyclerFameView as we want. The below codes will add some fake masked views to your frame view.
现在,根据需要向VeilRecyclerFameView中添加尽可能多的假视图(蒙版的骨架视图)。 以下代码将在框架视图中添加一些假的蒙版视图。
veilRecyclerView.addVeiledItems(15) // add veiled 15 items
We can even scroll the RecyclerView with shimmering effects on every item.
我们甚至可以滚动RecyclerView,对每一项进行闪烁效果。
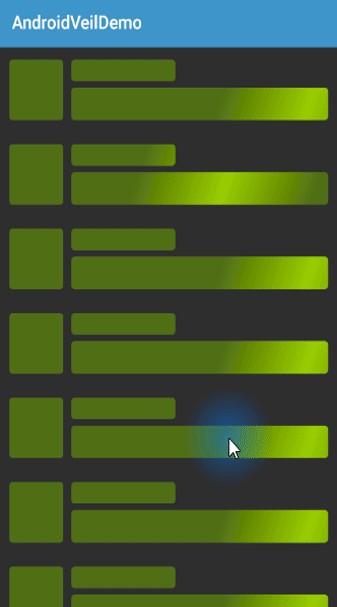
微光 (Shimmer)
This library using shimmer-android by Facebook. Here is the detail shimmer-instruction about shimmer or we can reference the below example.
该库使用Facebook的shimmer-android 。 这是有关微光的详细微光指令 ,或者我们可以参考以下示例。
val shimmer = Shimmer.ColorHighlightBuilder()
.setBaseColor(ContextCompat.getColor(context, R.color.shimmerBase0))
.setHighlightColor(ContextCompat.getColor(context, R.color.shimmerHighlight0))
.setBaseAlpha(1f)
.setHighlightAlpha(1f)
.build()
veilLayout.shimmer = shimmer // sets shimmer to VeilLayout
veilRecyclerView.shimmer = shimmer // sets shimmer to VeilRecyclerView
That’s all!If you want to get more information about the AndroidVeil library, you can check below the GitHub link :) Thank you! Have a nice day! 🤩
仅此而已。如果您想获取有关AndroidVeil库的更多信息,可以在GitHub链接下面查看:)谢谢! 祝你今天愉快! 🤩
神秘面纱