ios开发防止循环依赖
More and more we see how the iOS community strives for more modularized architectures. And it is obviously not just a fad. The benefits are clearly evident, the most immediate being much shorter compilation times given that you can just focus on the working framework. Furthermore, you are aiming for a cleaner architecture, one much more readable and testable. On top of that, you can just reuse your component in any other application, or exchange it as you wish.
我们越来越多地看到iOS社区如何争取更多的模块化体系结构 。 显然,这不仅仅是一种时尚。 好处显而易见,最直接的好处就是可以缩短编译时间,因为您可以只关注工作框架。 此外,您的目标是建立一种更清晰,更易读和可测试的体系结构。 最重要的是,您可以在任何其他应用程序中重用您的组件,或根据需要交换它。
But same as when creating your object oriented design you rely on the SOLID principles to make it easy to maintain and extend, when constructing your components architecture you always must bear in mind these three points:
但是,与创建面向对象设计时一样,您依靠SOLID原理使其易于维护和扩展,在构造组件架构时,您必须始终牢记以下三点:
APD: The Acyclic Dependencies Principle
APD :非循环依赖原则
SDP: The Stable Dependencies Principle
SDP :稳定依赖原则
SAP: The Stable Abstractions Principle
SAP :稳定抽象原则
In this first article we will focus on the ADP, The Acyclic Dependencies Principle.
在第一篇文章中,我们将重点介绍ADP, 即非循环依赖原则。
非循环依赖原则 (The Acyclic Dependencies Principle)
Allow no cycle in the component dependency graph
组件依赖图中不允许循环
The theory is simple, our dependency graph should not contain any cycle. If there is one cycle, we are just creating a big monolithic component causing exactly what we wanted to avoid in the first place: longer compilation times and other effects caused by a highly coupled architecture, such as the morning after syndrome. How can we avoid that? Let’s look at an example on iOS with the MVVM architecture.
理论很简单,我们的依赖图不应包含任何循环。 如果有一个周期,那么我们只是在创建一个巨大的整体组件,而这正是我们首先要避免的事情:更长的编译时间以及高度耦合的体系结构(例如综合症之后的早晨)所造成的其他影响。 我们如何避免这种情况? 让我们看一下带有MVVM架构的iOS上的示例。
非循环依赖架构 (An Acyclic Dependency Architecture)
Let’s suppose that we modularized the architecture of an IOS application that uses MMVM. Model-View-ViewModel (MVVM) is a structural design pattern that separates objects into three distinct groups: Models, Views and ViewModels. Because we are super impressed with the benefits stated above, we are going to create a Framework for each of them.
假设我们对使用MMVM的IOS应用程序的体系结构进行了模块化。 Model-View-ViewModel( MVVM )是一种结构设计模式,它将对象分为三个不同的组:模型,视图和视图模型。 因为我们对上述好处印象深刻,所以我们将为每个好处创建一个框架 。
Our application is simple, the UI will display distinctive elements depending of the User Permissions in the User Profile, and it will be the responsibility of the ViewModel to discern what to show:
我们的应用程序很简单,UI将根据用户配置文件中的用户权限显示独特的元素,ViewModel负责识别显示的内容:

This is a directed graph, we can follow the dependencies with the arrows. Moreover, there are no cycles in it, it is a Directed Acyclic Graph (DAG). It is awesome.
这是一个有向图,我们可以使用箭头跟随依赖性。 而且,其中没有循环,它是有向无环图(DAG)。 太棒了。
Here we have 4 components, obviating the View or some Routing layer that are not relevant for our case. The UserProfileViewModel depends on the User struct in the Model to get the information. This one at the same time depends on the PermissionsManager to enrich its data with its given permissions.
在这里,我们有4个组成部分,它们消除了与我们的案例无关的View或某些Routing层。 UserProfileViewModel依赖于Model中的User结构来获取信息。 同时,这取决于PermissionsManager以其给定的权限丰富其数据。
错误的行为 (The Misdeed)
Now, the Interaction Designer has come to us with a new feature, we will show a totally different view depending on the User Permissions. That way, they will sell more subscriptions because we show more promotional content directed to a non-paying user.
现在,交互设计器为我们提供了一项新功能,根据用户权限,我们将显示完全不同的视图。 这样,他们将售出更多的订阅,因为我们会显示更多针对非付费用户的促销内容。
Once they let us know this new task, it is time to design our approach. We will create a Factory class that will provide the right View Model depending on the User Permissions. After some deliberation, the “expert” in our team decides that it should be included in the Permissions Manager framework, given that everything related to the permissions is its responsibility. (please forgive this atrocious architecture).
一旦他们告诉我们这项新任务,就该设计我们的方法了。 我们将创建一个Factory类,该类将根据用户权限提供正确的视图模型。 经过仔细考虑,鉴于与权限相关的所有事情都是其责任,我们团队中的“专家”决定将其包括在Permissions Manager框架中。 (请原谅这个残酷的架构)。
Alright, so it is! The factory is implemented in the PermissionsManager, and our architecture looks like this now:
好吧,就是这样! 工厂是在PermissionsManager中实现的,我们的体系结构现在看起来像这样:
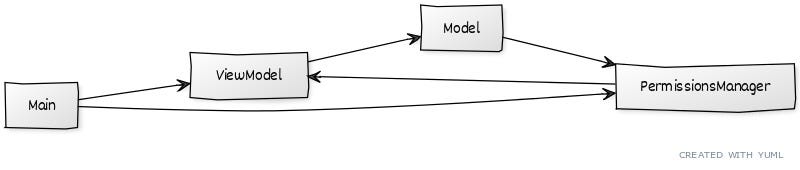
We do not have an acyclic graph anymore, there is a cycle between ViewModel, Model, and PermissionsManager. We are doomed! Instead of three components, we have a big one that should be compiled entirely every time we modify any of them. Besides, given the tight coupling, one change might have consequences in any of them. Not good. Actually awful.
我们不再有一个非循环图, 在ViewModel,Model和PermissionsManager之间存在一个循环 。 我们完了! 除了有三个组成部分外,我们还有一个很大的组成部分,每次我们对它们中的任何一个进行修改时都应完全编译。 此外,鉴于紧密的联系,任何改变都可能对他们造成影响。 不好。 真可怕
打破它! (Break it!)
Once we are aware of the dreadful consequences of the last addition, it is time to break the cycle. To accomplish that we have two different strategies:
一旦我们意识到了最后一次添加的可怕后果, 就该打破循环了 。 为此,我们有两种不同的策略:
应用依赖倒置原则(DIP) (Apply the Dependency Inversion Principle (DIP))
Depend on abstractions, not concretions, you asshole.
混蛋取决于抽象而不是混凝土。
We will create a protocol revealing the functions that provides the Permissions to the User Model. The Permissions Manager will implement that protocol, but will be hidden to the model, thus inverting the dependency between the Model and the PermissionsManager:
我们将创建一个协议,以揭示向用户模型提供权限的功能。 权限管理器将实现该协议,但是将对模型隐藏,从而反转了模型与PermissionsManager之间的依赖关系:
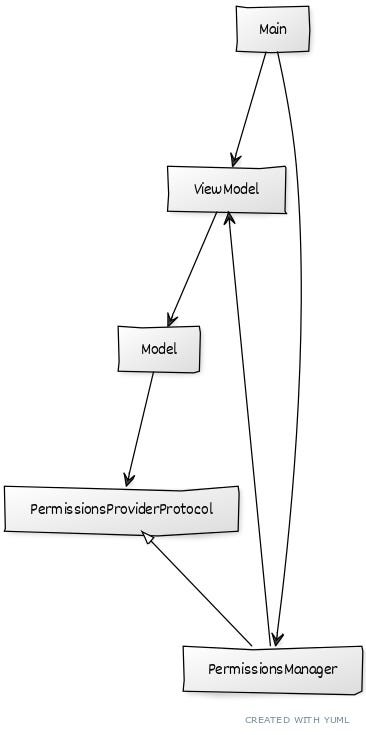
It might look odd having a component with only a protocol on it and no executable code, but this is a necessary and common practice in other languages such as Java and C#. Robert C. Martin, Clean Architecture.
组件上只有一个协议而没有可执行代码,这看起来很奇怪,但这是其他语言(例如Java和C#)的必要和普遍做法。 Robert C. Martin,《清洁建筑》。
在它们之间创建一个组件。 (Create a component between them.)
To break it, we could create a component between the ViewModel and the PermissionsManager. The ViewModelFactory depends on both ViewModel and PermissionsManager, hence breaking the previous cycle:
为了打破它,我们可以在ViewModel和PermissionsManager之间创建一个组件。 ViewModelFactory同时依赖于ViewModel和PermissionsManager,因此打破了上一个周期:

You can combine both strategies to end up with the cleanest approach, one that ensures you a good maintainability and flexibility when adding new features to the project.
您可以将这两种策略结合起来,以最干净的方法结束,这种方法可以确保在向项目添加新功能时具有良好的可维护性和灵活性。
结语 (Wrapping it up)
Now that modularizing our apps is in vogue and with good reason, we should be aware that before adding new components we have to stop and think. Are we improving our architecture? Are we actually achieving the goals we strive for with our separate modules? Or are we just having the same monolithic structure although with fancier looks? We should be open to this reflection not only at design time, but also when refactoring the current solution if it shows the most common pitfalls of a non-clean architecture.
既然我们的应用程序模块化正在流行并且有充分的理由,我们应该意识到在添加新组件之前,我们必须停下来思考。 我们正在改善我们的架构吗? 我们实际上是否通过单独的模块实现了我们追求的目标? 还是我们看起来更像奇特的整体结构? 我们不仅应该在设计时考虑这种反思,还应该在重构当前解决方案(如果它显示了非干净架构的最常见缺陷)时也要开放。
If you want to know more, I refer you to the bible on these matters, Clean Architecture: A Craftsman’s Guide to Software Structure and Design (Robert C Martin) There you will find more examples about this and the other principles I mentioned above.
如果您想了解更多信息,我将向您介绍有关这些问题的圣经,《 清洁体系结构:软件结构和设计的工匠指南》(罗伯特·C·马丁),您将在此找到更多有关此以及我上面提到的其他原理的示例。
And of course, in case you have more questions or suggestions please drop a comment below or send me a message.
当然,如果您有其他问题或建议,请在下面留言或给我发送消息。
Break the cycle, man!
打破循环,伙计!
翻译自: https://medium.com/axel-springer-tech/the-acyclic-dependencies-principle-on-ios-7804e6b1bbf9
ios开发防止循环依赖