swift 设置默认
All iOS apps have a built-in data dictionary that stores small amounts of user settings for as long as the app is installed. This system is called UserDefault.
所有iOS应用程序都具有内置的数据字典,该字典在安装应用程序后便会存储少量用户设置。 该系统称为UserDefault。
什么是用户默认值? (What is User Defaults?)
According to Apple’s documentation, UserDefaults is an interface to the user’s default database, where you store key-value pairs persistently across launches of your app.
根据Apple的文档,UserDefaults是用户默认数据库的接口,您可以在其中存储应用程序启动期间的键值对。
UserDefault can save integers, booleans, strings, arrays, dictionaries, dates and more, but you should be careful not to save too much data because it will slow the launch of your app.
UserDefault可以保存整数,布尔值,字符串,数组,字典,日期和更多内容,但是您应注意不要保存太多数据,因为这会减慢应用程序的启动速度。
UserDefaults is just like dictionaries, it consists of keys and values. For example:
UserDefaults就像字典一样,它由键和值组成。 例如:
var dict = [
"Name": "Yafonia",
"Age" : 21,
"Language": "Indonesian"
]
User defaults are saved in a .plist
file, and in this case is in Info.plist
.
用户默认值保存在.plist
文件中,在这种情况下,保存在Info.plist
。
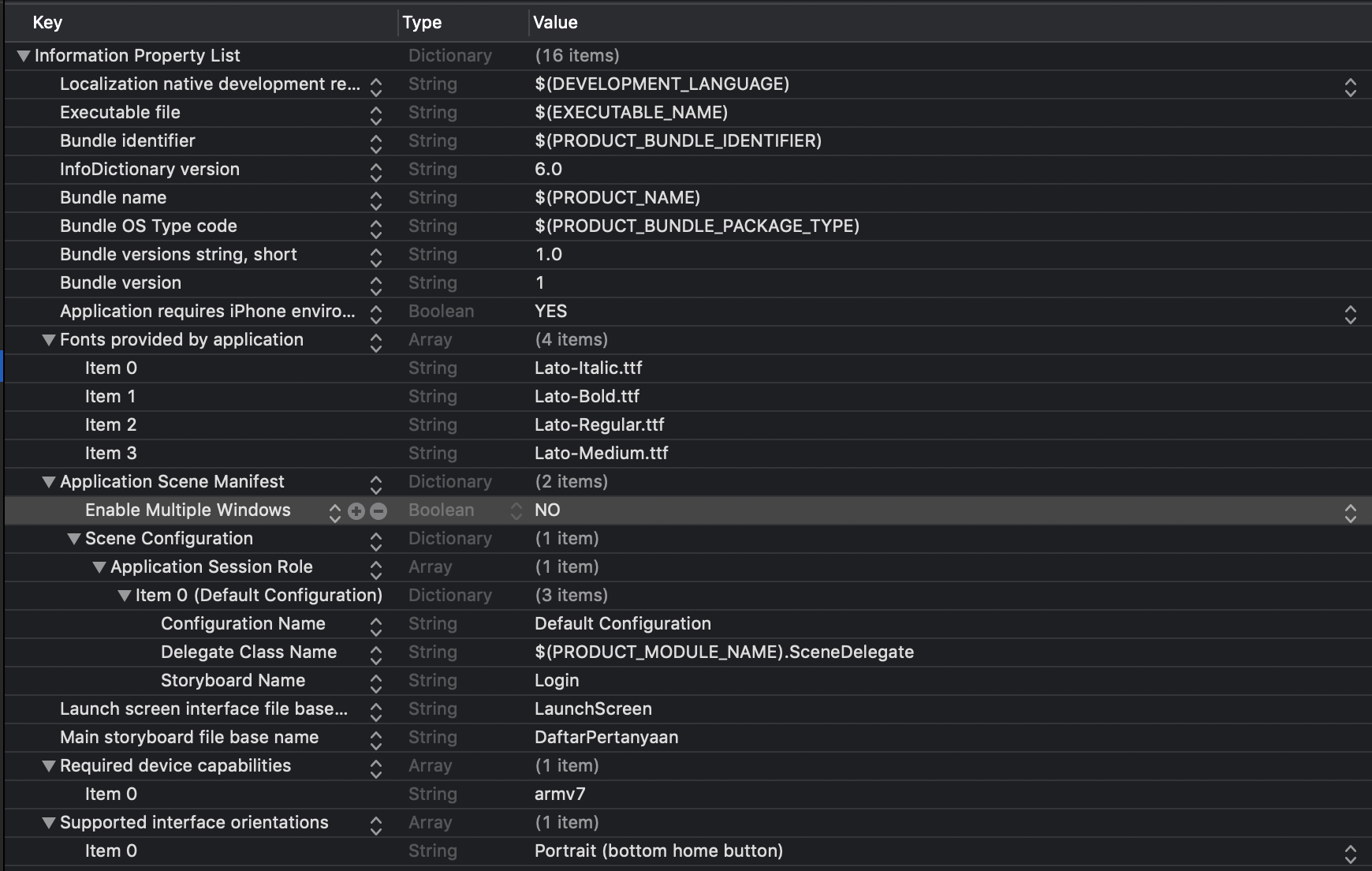
何时使用用户默认值 (When to Use User Defaults)
The user defaults are best used for simple pieces of data. If you need to store multiple objects, you better use the real database. These are several example pieces of data that are saved in UserDefaults:
用户默认值最适合用于简单数据。 如果需要存储多个对象,则最好使用真实数据库。 以下是一些保存在UserDefaults中的示例数据:
- User information, like name, email address, age, occupation 用户信息,例如姓名,电子邮件地址,年龄,职业
- App settings, like user interface language, app color theme or font 应用程序设置,例如用户界面语言,应用程序颜色主题或字体
- Flags (isLoggedIn to check whether the user is logged in or not, etc.) 标志(isLoggedIn检查用户是否已登录等)
以用户默认值保存数据 (Saving Data in User Defaults)
We can save several variable types on UserDefaults:
我们可以在UserDefaults上保存几种变量类型:
Booleans with
Bool
, integers withInt
, floats withFloat
and doubles withDouble
具有
Bool
,具有Int
整数,具有Float
和具有Double
精度数Strings with
String
, binary data withData
, dates with Date, URLs with theURL
type具有
String
,具有Data
二进制数据, 具有日期的日期 ,具有URL
类型的URL
Collection types with
Array
andDictionary
具有
Array
和Dictionary
集合类型
Internally the UserDefaults
class can only store NSData
, NSString
, NSNumber
, NSDate
, NSArray
and NSDictionary
classes.
在内部, UserDefaults
类只能存储NSData
, NSString
, NSNumber
, NSDate
, NSArray
和NSDictionary
类。
For example, in this project, I want to save several account’s information such as email, code, name, token, and UserID. So I set values from loginResponse as the values of the keys (Email, LawyerCode, LawyerName, Token, UserID). All the values are string.
例如,在这个项目中,我要保存几个帐户信息,例如电子邮件,代码,名称,令牌和UserID。 因此,我将loginResponse中的值设置为键的值(Email,LawyerCode,LayererName,Token,UserID)。 所有值都是字符串。
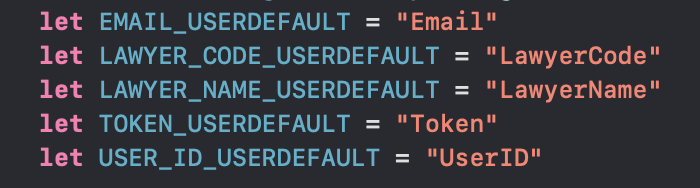
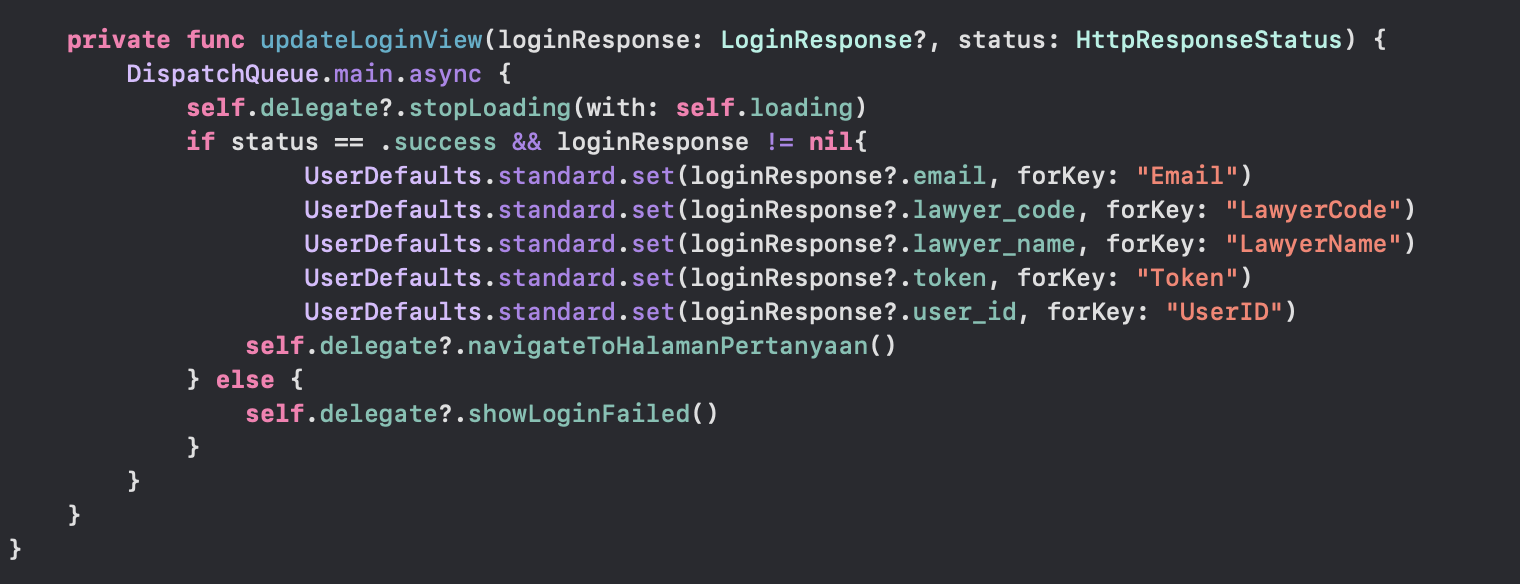
Besides account info, there’s a key named "Token"
that is used for login info. If there’s value on that key, then the user is logged in, vice versa. You can also use flags, for example you can call it "isLoggedIn"
with boolean
values.
除了帐户信息外,还有一个名为"Token"
的密钥,用于登录信息。 如果该键上有值,则用户已登录,反之亦然。 您还可以使用标志,例如,您可以使用boolean
值将其称为"isLoggedIn"
。
以用户默认值获取数据 (Getting Data in User Defaults)
Getting data in User Defaults is as simple as saving it. Let’s see example below.
在“用户默认设置”中获取数据就像保存数据一样简单。 让我们看下面的例子。
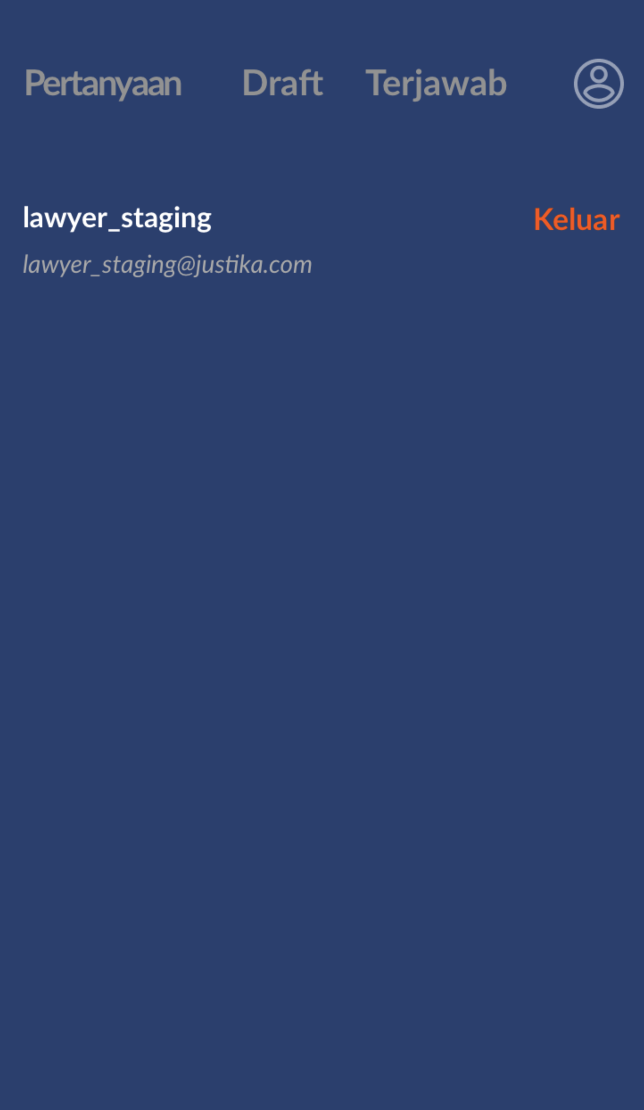
For this page above, I need account’s name (ex: lawyer_staging) and email (ex: lawyer_staging@justika.com), which is saved in User Defaults with the key "Email"
and "LawyerName"
. So here’s what we’re going to do:
对于上面的此页面,我需要帐户名称(ex:律师_staging)和电子邮件(ex:律师_staging@justika.com),将其保存在“用户默认值”中,键为"Email"
和"LawyerName"
。 所以这是我们要做的:
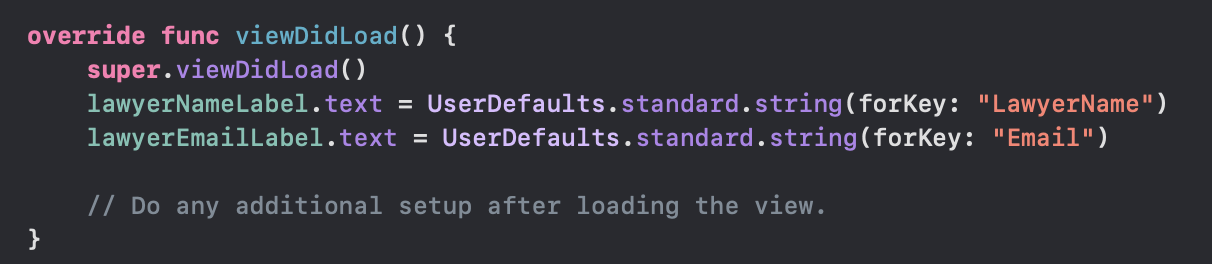
To show the account’s name and email you can just set the label’s text to the data you get from UserDefaults. Yes, it’s as simple as that!
要显示帐户的名称和电子邮件,您只需将标签的文本设置为从UserDefaults获得的数据即可。 是的,就是这么简单!
重置用户默认设置中的数据 (Reset Data in User Defaults)
Maybe you’ve been wondering, since I don’t use flags for login info, how would it be when user is not logged in?
也许您一直在想,既然我不使用标志作为登录信息,那么当用户未登录时会怎样呢?
If the user’s logged out, then we can reset the keys and values on the User Default. How do we do that?
如果用户已注销,那么我们可以在“用户默认设置”上重置键和值。 我们该怎么做?

So, when the user clicks “Keluar” button, we can reset all the User Defaults’ key and values for login info. When a user is logged in, the key and the values are set again in User Defaults.
因此,当用户单击“ Keluar”按钮时,我们可以重置所有“用户默认设置”键和登录信息值。 登录用户后,将在“用户默认值”中再次设置键和值。
用户默认语言上的用户界面语言 (User Interface Language on User Defaults)
As mentioned above, we also can save app settings like user interface language on User Defaults. For example, fonts info is saved in User Defaults.
如上所述,我们还可以将用户界面语言之类的应用设置保存在“用户默认值”上。 例如,字体信息保存在“用户默认设置”中。
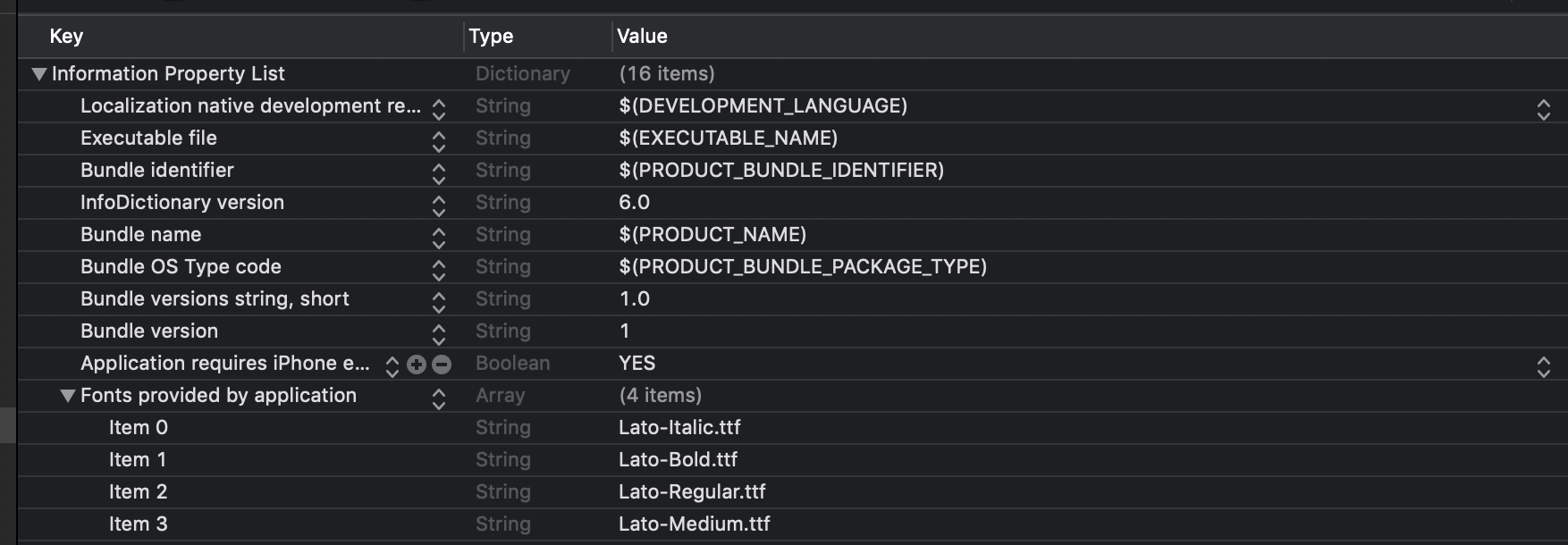
On the image above, you can see that there’s a key called "Fonts provided by application"
and that’s where we save the fonts.
在上图中,您可以看到有一个名为"Fonts provided by application"
的键,这是我们保存字体的地方。
结论 (Conclusion)
We can easily use User Defaults for saving simple pieces of data such as user’s information, app settings, and flags in the form of string, boolean, integers, arrays, dictionaries, dates, and more.
我们可以轻松地使用“用户默认值”以字符串,布尔值,整数,数组,字典,日期等形式保存简单的数据,例如用户信息,应用程序设置和标志。
翻译自: https://levelup.gitconnected.com/user-defaults-in-swift-dfe228f684c6
swift 设置默认