android orm
The journey of a developer, like any other professional minimally devoted to learning continuously, inevitably has a continuous learning factor. In my case, this learning curve has been intense and full of weekly novelties. The hot topic of the week has been something that changed completely my view on code and database organization. We are speaking of ORMs or Object-Relational Mapping.
像任何其他专注于持续学习的专业人士一样,开发人员的旅程不可避免地具有持续学习的因素。 就我而言,这种学习曲线非常紧张,每周都有很多新奇之处。 一周的热门话题已经彻底改变了我对代码和数据库组织的看法。 我们所说的是ORM或对象关系映射。
ORM is a computer-science technique for converting data between incompatible data systems, or, in the most common case, querying and manipulating data from a database, by using object-oriented languages. In other words, an ORM works as a “translator” between such systems. Taking the SQL paradigm as an example, it’s translating a database query such as this:
ORM是一种计算机科学技术,用于在不兼容的数据系统之间转换数据,或者在最常见的情况下,通过使用面向对象的语言来查询和操纵数据库中的数据。 换句话说,ORM充当此类系统之间的“翻译器”。 以SQL范例为例,它正在翻译这样的数据库查询:
SELECT * FROM students WHERE name = 'James';
To this (using JavaScript as an example):
为此(以JavaScript为例):
const { myOrm } = require('my-orm-library');const student = myOrm('students').where({ name: 'James });
Long story short, you create and manage your database from objects in your favorite programming language. Instead of querying to create the database and then carrying the data to your code, the ORM does the entire thing from the beginning!
简而言之,您可以使用自己喜欢的编程语言从对象创建和管理数据库。 ORM无需查询创建数据库然后将数据携带到代码中,而是从一开始就完成了所有工作!
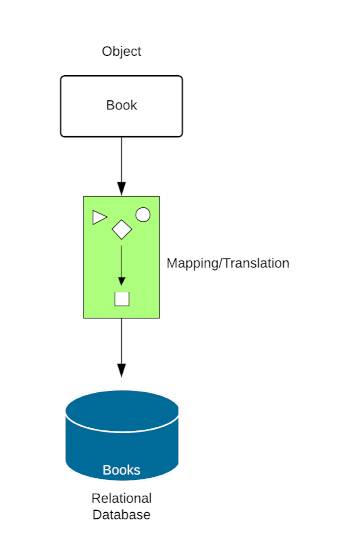
This leads to the main feature of any ORM: the Model-View-Controller pattern, or MVC.
这导致了任何ORM的主要特征:模型-视图-控制器模式或MVC。
And what does the MVC do? It encapsulates a software’s development logic into three intertwined elements, each responsible for its role in an application:
MVC会做什么? 它将软件的开发逻辑封装为三个相互交织的元素,每个元素负责其在应用程序中的角色:
The Model contains the application data, logic, and basic rules of the application.
该模型包含应用程序数据,逻辑和应用程序的基本规则。
View outputs the visual representation of the model in some form.
视图以某种形式输出模型的视觉表示。
Lastly, the Controller manages the interaction and validation between user inputs from the View, performing interactions in the data model objects.
最后,控制器从视图管理用户输入之间的交互和验证,在数据模型对象中执行交互。
In some ORMs, like Ruby’s Rails-based Active Record, the validation and basic rule logic are also managed by the Controller, while the Model takes care of the data modeling and manipulation.
在某些ORM中,例如Ruby的基于Rails的Active Record,验证和基本规则逻辑也由Controller管理,而Model负责数据建模和操作。
A basic diagram of an MVC pattern functioning in an ORM would look like this:
在ORM中起作用的MVC模式的基本示意图如下所示:
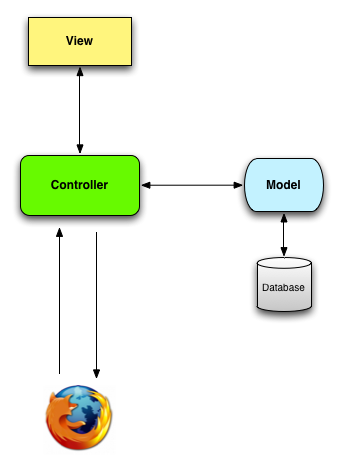
My current (and ongoing, as of September 2020) experience with ORM is Active Record, Ruby’s most popular development library. At the same time that it helped me understand much better object-oriented programming and provided a much cleaner code style, it’s been learning to ride a bike again from scratch. The basic drawback of any ORM is that it acts as a translator, and because of that, speaking in development terms, it’s almost like learning a new programming language entirely. It does compartmentalize and organize your logic in a much better way, but requires a new learning curve, since it is kind of a dialect in the programming language you are working on, and the MVC pattern may not be very familiar for the code newbie — myself included.
我目前(并正在进行,截至2020年9月)的经验是Active Record,这是Ruby最受欢迎的开发库。 同时,它帮助我理解了更好的面向对象编程,并提供了更简洁的代码样式,同时,它还在学习从头开始骑自行车。 任何ORM的基本缺点是它充当翻译器,因此,以开发术语来讲,这就像完全学习一种新的编程语言一样。 它确实可以更好地划分和组织逻辑,但是需要一条新的学习曲线,因为它是您正在使用的编程语言中的一种方言,并且MVC模式对于新手来说可能不是很熟悉-包括我自己。
These are some advantages of using an ORM:
这些是使用ORM的一些优点:
- You may keep writing in the same programming language you are used to and don’t need to take long learning proper SQL statements to build and manipulate your databases. 您可以继续使用与以前相同的编程语言来编写代码,而无需花费很长时间学习适当SQL语句即可构建和操作数据库。
- Since it uses your programming language to abstract database querying, you may switch database systems, as long as both systems are supported by the ORM library. 因为它使用您的编程语言来抽象数据库查询,所以您可以切换数据库系统,只要这两个系统都受ORM库支持即可。
Many ORMs include other advanced features out of the box, such as progressive database migrations (meaning easier database manipulation), seeding, and transactions, among other features. Such features help prevent SQL injections, as well.
许多ORM都具有其他现成的高级功能,例如渐进式数据库迁移(意味着更轻松的数据库操作),种子和事务以及其他功能。 这些功能也有助于防止SQL注入。
- Most ORMs automate the data modeling process, taking fewer steps from the developer to organize the code inside their projects. 大多数ORM可以自动执行数据建模过程,而开发人员却很少采取措施在其项目中组织代码。
- Since the cornerstone of an ORM is writing code in the MVC pattern, your code gets cleaner and more reusable. 由于ORM的基石是以MVC模式编写代码,因此您的代码将变得更干净,更可重用。
Bear in mind, however, that adopting an ORM isn’t unanimity among programmers. When I first Googled the subject, I quickly found a lengthy criticism against ORMs, demonstrated with practical JavaScript examples. Some of the advantages described include:
但是请记住,采用ORM并不是程序员之间的一致意见。 当我第一次搜索该主题时,我很快发现了对ORM的长期批评,并通过实用JavaScript示例进行了演示。 描述的一些优点包括:
- For the same reason, you stick to your current programming language, using an ORM leads you to not learn appropriately how SQL is programmed and structured, leading to technical deficiencies. 出于同样的原因,您仍然使用当前的编程语言,使用ORM会使您无法正确学习SQL的编程和结构方式,从而导致技术缺陷。
ORMs are usually efficient for querying simpler database structures, but that isn’t the case when you need to develop more complex queries. Since the ORM created a new abstraction layer with the object model, this comes with a toll on performance. This article gives a SQL vs. ORM performance comparison to demonstrate such reality, and this repository demonstrates the same issue within Node ORM libraries.
ORM通常对于查询更简单的数据库结构是有效的,但是当您需要开发更复杂的查询时,情况并非如此。 由于ORM使用对象模型创建了新的抽象层,因此会带来性能损失。 本文给出了SQL与ORM性能的比较,以证明这种情况,并且此存储库还演示了Node ORM库中的相同问题。
- Despite adopting the programming language you are working on, ORMs still bring a new layer to learn, since they come with their patterns, setups, special syntax, and features. This may be particularly time-consuming or confusing for new developers. 尽管采用了您正在使用的编程语言,但ORM仍然带有新的学习层,因为它们附带了它们的模式,设置,特殊语法和功能。 对于新开发者而言,这可能特别耗时或令人困惑。
- ORM database building is still an automated process. If you have solid SQL knowledge, you probably may build better performing queries by yourself. ORM数据库构建仍然是一个自动化过程。 如果您具有扎实SQL知识,则可以自己构建性能更好的查询。
All things said and described regarding ORMs and what they may bring, I have decided to explore a little more on the topic with JavaScript ORM libraries, since JS is the first programming language I have learned as a web developer, and this means I will inevitably bump into projects and systems that adopt such libraries. I am taking some time to describe a little about three of the most popular ORM libraries available and what they may bring to developers.
关于ORM及其可能带来的一切内容,我决定用JavaScript ORM库对该主题进行更多的探讨,因为JS是我作为Web开发人员学习的第一门编程语言,这意味着我将不可避免地碰到采用此类库的项目和系统。 我花一些时间来描述大约三个最流行的ORM库以及它们可能带给开发人员的东西。
Before I go on, I’m only opening a brief parenthesis on how I sorted these libraries first:
在继续之前,我仅在简短括号中说明如何首先对这些库进行排序:
Despite its great popularity, Knex is NOT an ORM. Knex is a SQL query builder that, despite supporting many databases such as Postgres, MySQL, MariaDB, and many others, does not perform Object Related Mapping. That role is still up to the developer, on their own or by utilizing an ORM. By the way, one of the ORMs I’ll present uses Knex as their query builder.
尽管广受欢迎,但Knex 不是ORM。 Knex是一个SQL查询生成器,尽管它支持许多数据库,例如Postgres,MySQL,MariaDB和许多其他数据库,但是却不执行对象相关映射。 该角色仍然由开发人员自行决定或通过使用ORM。 顺便说一下,我将介绍的ORM之一使用Knex作为其查询生成器。
- I have prioritized ORMs that support multiple databases since one of the essential points of adopting such a library is avoiding the hassle of restructuring your entire query building in the event of a database system migration. 我对支持多个数据库的ORM进行了优先排序,因为采用这样的库的要点之一是避免了在数据库系统迁移时重组整个查询构建的麻烦。
1.续集 (1. Sequelize)
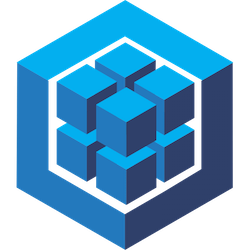
Sequelize is by far the most popular JavaScript ORM library, with over 700,000 weekly downloads, and has been maintained and updated for almost ten years. The constant and consistent response to issues justifies its enormous popularity, making it considered a solid ORM library for JavaScript.
Sequelize是迄今为止最受欢迎的JavaScript ORM库,每周下载量超过700,000,并且已维护和更新了近十年。 对问题的持续且一致的响应证明了其巨大的普及性,使其成为JavaScript的可靠ORM库。
Sequelize supports Postgres, MySQL, MariaDB, SQLite, and Microsoft SQL Server. Being Promise-based, it helps many developers who are used to use this object model quickly getting acquainted with their library. Other features that Sequelize include are, but not limited to:
Sequelize支持Postgres,MySQL,MariaDB,SQLite和Microsoft SQL Server。 基于Promise,它可以帮助许多习惯于使用该对象模型的开发人员快速熟悉他们的库。 Sequelize的其他功能包括但不限于:
- Database synchronization 数据库同步
- Database migrations数据库迁移
- Model validations and hooks模型验证和挂钩
- Data seeding, relations, and even raw queries to the developer’s taste数据种子,关系,甚至原始查询都取决于开发人员的喜好
- Facilitated testing便利的测试
Sequelize features extremely rich and detailed documentation, separated by version to help out legacy development, and includes an API reference. Their documentation may be hard to read sometimes, though.
Sequelize具有极其丰富和详细的文档,按版本进行分隔以帮助进行遗留开发,并包含API参考。 但是,有时它们的文档可能很难阅读。
If you value development stability, you probably want to stick with Sequelize as your JavaScript ORM of choice.
如果您重视开发的稳定性,那么您可能希望坚持使用Sequelize作为您JavaScript ORM。
2. TypeORM (2. TypeORM)
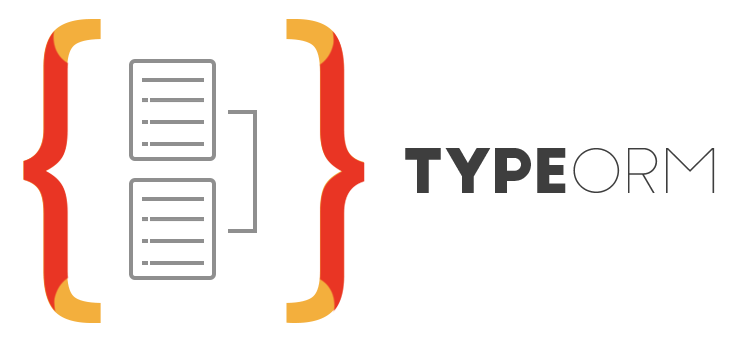
TypeORM is a relatively new library, compared to other ORM counterparts, having its first release four years ago. Nonetheless, its compatibility with both TypeScript and modern JavaScript (ES5 onwards) made it increasingly popular among developers, by having almost half the weekly downloads as its most popular and usual counterpart, described above.
与其他ORM同类库相比,TypeORM是一个相对较新的库,它于4年前首次发布。 尽管如此,它与TypeScript和现代JavaScript(自ES5起)的兼容性使得它在开发人员中越来越受欢迎,如上所述,它每周都有近一半的下载量是最受欢迎和最常用的下载方式。
Looking at TypeORM’s documentation almost felt like looking at a Saturday morning ad, since it enjoys boasting a wide array of features and compatibilities. Here’s part of such list, from their landing documentation page:
查看TypeORM的文档几乎就像看一个星期六的早晨广告一样,因为它具有多种功能和兼容性。 这是此类列表的一部分,来自其着陆文档页面:
- DataMapper or Active Record pattern support DataMapper或Active Record模式支持
- Connection configuration in JSON, XML, YML and ENV formatsJSON,XML,YML和ENV格式的连接配置
- Connection pooling连接池
- Entity management实体管理
- Multiple database connections多个数据库连接
- Support for many frameworks, browsers, and platforms such as React Native支持许多框架,浏览器和平台,例如React Native
Support to a wide array of database systems, including MongoDB, which is a NoSQL (non-relational) database, something which most ORMs do not support
支持各种各样的数据库系统,包括MongoDB,它是NoSQL (非关系)数据库,大多数ORM不支持该数据库
As you travel through their documentation, you quickly get tempted to throw all other options under the bus and immediately adopt TypeORM as your ORM library of choice. However, after some research, I quickly realized that my feeling that I was looking at a Saturday ad wasn’t flawed, after all. A recent comparison between TypeORM and Sequelize points out two jarring problems in TypeORM: poorly detailed documentation (despite their excellent presentation), and lack of native data seeding. While Sequelize supports native data seeding, TypeORM requires an external plugin to do so, extending dependency requirements and potentially generating side effects for testing.
在阅读他们的文档时,您很快会被诱惑将所有其他选项扔到总线下,并立即采用TypeORM作为您选择的ORM库。 但是,经过一番研究,我很快意识到,我在看星期六广告的感觉毕竟没有错。 最近在TypeORM和Sequelize之间进行的比较指出了TypeORM中的两个棘手问题:详尽的文档(尽管其表现出色)和缺乏本地数据种子。 尽管Sequelize支持本机数据播种,但TypeORM需要使用外部插件来这样做,从而扩展了依赖性要求并可能产生测试副作用。
Though it seems to be the ideal ORM of choice for TypeScript developers, many pros tend to advise caution when using TypeORM.
尽管对于TypeScript开发人员来说,它似乎是理想的ORM选择,但许多专业人士在使用TypeORM时往往建议谨慎。
3.书架 (3. Bookshelf)
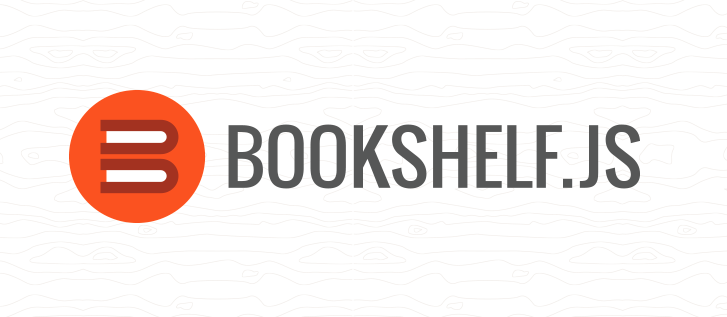
Bookshelf is a distant third option for developers, featuring close to 100,000 weekly downloads. It’s a Node.js built on the Knex SQL builder and supports the Postgres, MySQL, and SQLite SQL databases. What makes Bookshelf stand out compared to the other two mentioned libraries is their support to callbacks, becoming a potential choice for developers who favor functional programming over Sequelize promise-based modeling (also supported by Bookshelf) or TypeORM’s class-based modeling.
对于开发人员而言,书架是遥不可及的第三选择,每周下载量接近100,000。 它是在Knex SQL构建器上构建的Node.js,并支持Postgres,MySQL和SQLite SQL数据库。 与其他提到的两个库相比,Bookshelf脱颖而出的原因在于它们对回调的支持,对于那些偏爱基于Sequelize基于promise的建模(也受Bookhelf支持)或TypeORM的基于类的建模的开发人员,它们成为潜在的选择。
Other features present in Bookshelf are:
书架中的其他功能包括:
- Support to the most common SQL relations (one-to-one, one-to-many, many-to-many) 支持最常见SQL关系(一对一,一对多,多对多)
- Polymorphic associations 多态关联
- Eager (nested of not) relation loading渴望(不需要)关系加载
Bookshelf seems to be a simpler library compared to TypeORM or Sequelize, but their support to callbacks may stand out to some developers, so it’s always nice to present a different choice to work with.
与TypeORM或Sequelize相比,书架似乎是一个更简单的库,但是它们对回调的支持在某些开发人员中可能会脱颖而出,因此,提出一个不同的选择总是很不错的。
好吧,那你呢,乔奥? (Okay, but what about YOU, Joao?)
您到底要潜入哪个JavaScript ORM兔子洞? (Which JavaScript ORM rabbit hole are you diving in, anyway?)
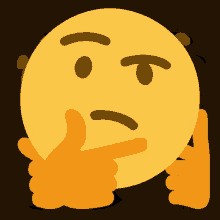
I will probably look at this post (my first development-related post ever, by the way) in a couple of years and take a peal of good laughter, or at least reflect deeply after reading this last section. As I stated at the beginning of this article, as a new developer, I feel like a kid in their 10-year birthday party getting all the cool toys every ten minutes. Incredibly amazed by the next toy I get to play, even if I don’t fully understand yet how it works. I enjoy it because it’s cool to play with, only to be amazed by the next toy available. It has been my life as a developer in a nutshell for the last three months. This may be awesome most of the time but perhaps overwhelming sometimes. It has been the case in this new world of Object-Related Mapping, and the Model-View-Controller pattern that ensues.
几年后,我可能会看一下这篇文章(顺便说一句,这是我有史以来与发展有关的第一篇文章),并发出一声欢笑,或者至少在阅读了最后一部分后会有所反思。 正如我在本文开头所说的那样,作为一名新开发人员,我觉得自己像个孩子,参加他们的10岁生日聚会,每隔10分钟就会得到所有漂亮的玩具。 即使我还不完全了解它的工作原理,我也对下一个玩具感到惊讶。 我喜欢它,因为它很酷,只能被下一个可用的玩具惊呆。 简而言之,这是我过去三个月作为开发人员的生活。 在大多数情况下,这可能很棒,但有时可能会让人不知所措。 在对象相关映射的新世界和随之而来的模型-视图-控制器模式中就是这种情况。
I have also quickly figured out that ORMs are a more controversial and opinionated topic than I had expected. Though many developers value it as a tool to simplify their code style and development, many other developers see ORMs as a remedy that might do more harm to the patient (in this case, the database model and structure) than good. Being a new developer, such general insight honestly surprised me.
我还很快发现,ORM是一个比我预期的更具争议性和自觉性的话题。 尽管许多开发人员将其视为简化代码样式和开发的工具,但许多其他开发人员将ORM视为对患者(在本例中为数据库模型和结构)造成的危害大于弊的补救措施。 作为一名新开发人员,这种普遍的见解使我感到惊讶。
I also tend to value stability and community support when dealing with programming languages, libraries, and tools. After exploring these three JavaScript ORM documentation and researching over the developer community perception, experience, and comparisons, my ORM of choice will definitely be Sequelize, despite being more verbose than the other two competitors. It will take me some time to get comfortable with using (and mastering!) its use, and the MVC pattern in general within JavaScript (I am getting to know it with Active Record and Rails), but learning is always a continuous process, and doing so as a developer isn’t an exception.
在处理编程语言,库和工具时,我也倾向于重视稳定性和社区支持。 在浏览了这三个JavaScript ORM文档并研究了开发人员社区的看法,经验和比较之后,尽管比其他两个竞争对手更冗长,但我选择的ORM肯定是Sequelize。 我将需要一些时间来适应使用(和掌握!)它的使用以及JavaScript中的MVC模式(我通过Active Record和Rails逐渐了解它),但是学习总是一个连续的过程,并且作为开发人员这样做也不例外。
If you wish to get to know better about ORM and its corresponding JavaScript libraries, I suggest some links I have navigated to better know the topic:
如果您想更好地了解ORM及其相应JavaScript库,建议您浏览一些链接以更好地了解以下主题:
Object-Relational Mapping, Wikipedia.
对象关系映射,维基百科。
ORM explained in 1 minute, John Hillegass.
ORM在1分钟内解释了John Hillegass。
What is an ORM and Why You Should Use it, Bits and Pieces.
什么是ORM以及为什么要使用它,点点滴滴。
Why you should avoid ORMs (with examples in Node.js), LogRocket.
为什么要避免使用ORM(使用Node.js中的示例) , LogRocket。
To ORM or not to ORM, Eli Bendersky.
到ORM还是不到ORM , Eli Bendersky。
Top Node.js SQLite ORM Libraries (with statistics), Openbase.
顶级Node.js SQLite ORM库(带有统计信息) , Openbase。
翻译自: https://medium.com/@jgoncalvesjr/diving-a-little-into-the-javascript-orm-rabbit-hole-fa4418253388
android orm