react生命周期方法
To build a project using React, the first step is to figure out the various Components that are required to bring your project to life! Once you are able to visualize the client-side of your project as a collection of Components, half the battle is won. So it is fair to say that Components form the crux of any React application.
要使用React构建项目,第一步是弄清楚使项目栩栩如生所需的各种组件! 一旦您能够将项目的客户端可视化为一组组件,就成功了一半。 因此可以说组件是任何React应用程序的症结所在。
But how does a collection of Components end up becoming a single page application? This is no different to the way every single website is rendered by your browser i.e, by creating a DOM. But in case of React, the Components are first woven into a Virtual DOM and only the necessary modifications are made to the real DOM. In order to do this, React must constantly keep track of every Component built for the project, and this is where we come across the life cycle of a Component.
但是,如何将一组组件最终变成一个单页应用程序? 这与您的浏览器(即通过创建DOM )呈现每个网站的方式没有什么不同。 但是在使用React的情况下,首先将组件编织到虚拟DOM中,然后仅对实际DOM进行必要的修改。 为了做到这一点,React必须不断跟踪为项目构建的每个Component,这就是我们遇到Component的生命周期的地方。
The following article provides a more elaborate explanation of how a browser renders a web page after creating the DOM. You can check it out if you need some more clarification!
下面的文章提供了有关浏览器在创建DOM之后如何呈现网页的更详尽的解释。 如果需要更多说明,可以检查一下!
A Component undergoes 3 phases in its life cycle. Think of it as milestones along the course of a Component’s life.
组件在其生命周期中经历三个阶段。 将其视为组件生命周期中的里程碑。
Mounting: This is the stage where the Component is inserted into the DOM. This phase is accounted for using the componentDidMount() method.
安装:这是将组件插入DOM的阶段。 此阶段是使用componentDidMount()方法解决的。
Updating: This is the stage in which the Component’s state and props can change, leading to the process of re-rendering the Component with the updated state/props.
更新:这是组件状态和道具可以更改的阶段,从而导致使用更新后的状态/道具重新渲染组件的过程。
Unmounting: This is the final stage of the Component’s life, in which it is removed from the DOM.
卸载:这是组件寿命的最后阶段,在该阶段将其从DOM中删除。
Note that sometimes there is another stage which is considered even before a Component is mounted. This is called the Initialization stage, where the Component’s initial state is set. Hence, it is common to see images that depict 4 stages in a Component’s life cycle.
请注意,有时甚至在安装组件之前,还会考虑另一个阶段。 这称为初始化阶段,在此阶段设置组件的初始状态。 因此,通常会看到描绘组件生命周期中四个阶段的图像。
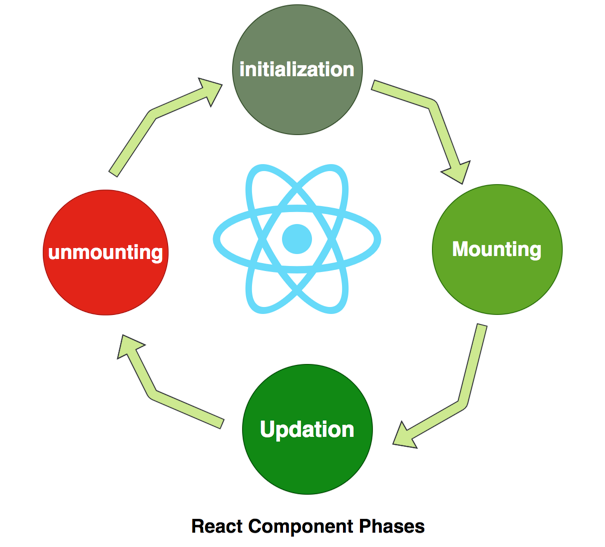
Now let us dive a little deeper into the life cycle methods which can be applied to a Component at various phases. Note that I am deliberately excluding certain deprecated methods.
现在让我们更深入地研究生命周期方法,该方法可以在各个阶段应用于组件。 请注意,我故意排除了某些不推荐使用的方法。
Before we start learning about the different methods available, it’s better to understand the role that they play.
在开始学习可用的不同方法之前,最好先了解它们所扮演的角色。
It is very straight forward too! These methods are like checkpoints along the way. They are invoked only at the very specific phases of a Component’s life cycle. This way, we have more control over a Component’s behavior, which in turn gives us a more flexible approach to building the UI using those Components!
这也非常简单! 这些方法就像整个过程中的检查点一样。 仅在组件生命周期的特定阶段调用它们。 这样,我们就可以更好地控制组件的行为,从而为我们提供了一种使用这些组件构建UI的更加灵活的方法!
Take a look at the image below, which shows the various methods and the points at which they are invoked.
看一下下面的图片,它显示了各种方法以及调用它们的要点。

Let us go over some of the most commonly used life cycle methods, along with examples.
让我们来看一些最常用的生命周期方法,以及一些示例。
constructor(): This is used only if you have a class-based Component and it serves the purpose of initializing the state of a Component. In case of functional Components, the useState() hook is used to do the same.
constructor():仅当您具有基于类的Component且用于初始化Component的状态时,才使用此方法。 对于功能性组件, useState()挂钩用于执行相同操作。
Consider an example in which you are creating a Component to store Todo tasks.
考虑一个示例,其中您将创建一个组件来存储Todo任务。
ComponentDidMount(): As seen from the image in the previous section, this is invoked after a Component is inserted into the DOM for the first time. This has a variety of uses, one of which can be to update state after a Component is mounted, like the example shown below.
ComponentDidMount() :从上一节中的图像可以看出,这是在第一次将Component插入DOM后调用的。 这具有多种用途,其中之一可以是在安装组件后更新状态,如以下示例所示。
render(): This is the method that is responsible for inserting a Component into the DOM. This is invoked every time a Component’s state/props is updated.
render() :这是负责将Component插入DOM的方法。 每次更新组件的状态/属性时都会调用此方法。
Now let us take a look at the life cycle methods which are invoked during the updating phase of a Component.
现在让我们看一下在组件更新阶段调用的生命周期方法。
shouldComponentUpdate(): This is invoked immediately after a Component’s state or props is updated. Although most changes are dealt with using the componentDidUpdate() method, this is often a more immediate way of dealing with the change. To take a look at a possible scenario where this comes in handy, you can go through the following article.
shouldComponentUpdate():这在组件的状态或道具更新后立即调用。 尽管大多数更改是使用componentDidUpdate()方法处理的,但这通常是处理更改的更直接的方法。 要查看在这种情况下派上用场的可能情况,可以阅读以下文章。
componentDidUpdate(): This is the method invoked after re-rendering an updated Component. This method can give you the information about a Component’s previous state and previous props. A fair warning to give before you start using this method is to never directly set the state of a Component within it. Doing that will change the Component’s state, futher triggering a componentDidUpdate() and so on.
componentDidUpdate() :这是在重新渲染更新的Component之后调用的方法。 此方法可以为您提供有关组件的先前状态和先前道具的信息。 在开始使用此方法之前,应给出的合理警告是,切勿直接在其中设置Component的状态。 这样做将更改Component的状态,进一步触发componentDidUpdate()等等。
componentDidUpdate(): This is the method invoked after re-rendering an updated Component. This method can give you the information about a Component’s previous state and previous props. A fair warning to give before you start using this method is to never directly set the state of a Component within it. Doing that will change the Component’s state, futher triggering a componentDidUpdate() and so on. This article provides some safe use cases for this life cycle method.
componentDidUpdate() :这是在重新渲染更新的Component之后调用的方法。 此方法可以为您提供有关组件的先前状态和先前道具的信息。 在开始使用此方法之前,应给出的合理警告是,切勿直接在其中设置Component的状态。 这样做将更改Component的状态,进一步触发componentDidUpdate()等等。 本文提供了此生命周期方法的一些安全用例。
getSnapshotBeforeUpdate(): This is used only when the developer requires more data about the DOM before the Component was updated and re-rendered. Although this is seldom used, the following article does a very good job of providing an explanation for an important use case.
getSnapshotBeforeUpdate():仅当开发人员需要更多数据时才使用 有关组件更新和重新呈现之前的DOM的信息。 尽管很少使用它,但以下文章在为重要用例提供解释方面做得很好。
getDerivedStateFromProps(): Again, this is a method that is seldom used. I have never come across a scenario which required the use of this specific method, and the team at React seems to agree!
getDerivedStateFromProps():同样,这是一种很少使用的方法。 我从来没有遇到过需要使用这种特定方法的场景,React的团队似乎也同意!
Finally, the only method to deal with the unmounting of a Component.
最后,这是处理组件卸载的唯一方法。
componentWillUnmount(): This is invoked just before a Component is removed from the DOM. This is where you can perform any cleanups that need to be done such as invalidating timers, canceling network requests, removing event listeners, and so on.
componentWillUnmount():这是在从DOM中删除组件之前调用的。 在这里,您可以执行任何需要清除的操作,例如使计时器无效,取消网络请求,删除事件侦听器等。
These are the methods that you are most likely to come across. But as I mentioned earlier, I have omitted certain methods that are deprecated or are set to be deprecated in the very near future. So, in case you run into a legacy system which uses some of the deprecated life cycle methods, you may need to do a bit of Googling!
这些是您最有可能遇到的方法。 但是,正如我前面提到的,我省略了某些方法,这些方法在不久的将来将不建议使用或设置为不建议使用。 因此,如果您遇到了使用某些过时的生命周期方法的旧系统,则可能需要做一些谷歌搜索!
Lastly, in case you are working with a functional Component, and are having trouble with implementing some of the methods discussed, you can go through the following article.
最后,如果您正在使用功能性组件,并且在实现所讨论的某些方法时遇到麻烦,则可以阅读以下文章。
翻译自: https://medium.com/@rajat_m/understand-life-cycle-methods-in-react-js-71a5464867cf
react生命周期方法