keras 香草编码器
If you’re new to JavaScript, you’re likely starting out with Vanilla JavaScript in order to learn the basics, and to understand how the language works at a core level, before jumping into frameworks. And if you’re new to Vanilla JavaScript, you’re probably realizing just how crucial it is to be able to effectively traverse the DOM when building your site.
如果您不熟悉JavaScript,则可能会从Vanilla JavaScript开始,以便学习基础知识并了解该语言在核心级别的工作方式,然后再跳入框架。 而且,如果您不熟悉Vanilla JavaScript,那么您可能已经意识到,在构建网站时有效地遍历DOM至关重要。
At first you may find this process daunting or at least tedious. And while the tedious nature of traversing the DOM is ubiquitous in Vanilla JavaScript (this is where frameworks like React will eventually make your life much easier), there is no reason that DOM traversal should be scary. To help you along your journey from the pit of despair to the halls of the enlightened, I’m going to detail out a few useful methods to up your DOM traversal game. Get ready.
起初,您可能会发现此过程令人生畏或至少很乏味。 尽管在香草JavaScript中遍历了DOM的乏味特性(这是React这样的框架最终将使您的生活变得更轻松的地方),但没有理由使DOM遍历不会令人恐惧。 为了帮助您从绝望之地到开明的大厅,我将详细介绍一些有用的方法来完善DOM遍历游戏。 做好准备。
To demonstrate this, I’ll use code from a recently completed blogging app my partner Dmitry and I built for our coding bootcamp, created with a Rails backend and Vanilla JS. If you’d like to check out the app, you can do so using this link here, just promise not to judge our StarWars Faker placeholder information too harshly. Without further ado, let’s jump into some methods.
为了说明这一点,我将使用我的合作伙伴Dmitry最近完成的博客应用程序中的代码,我是为我们的代码训练营而构建的,该训练营是由Rails后端和Vanilla JS创建的。 如果您想检出该应用程序,则可以在此处使用此链接进行操作,只是保证不要过于苛刻地判断我们的StarWars Faker占位符信息。 事不宜迟,让我们跳入一些方法。
。火柴() (.matches())
This is a nice once for anyone who tires of typing out three equals signs (c’mon JavaScript). The .matches method allows you to check whether the element you use it on, would be selected by the string you’ll eventually pass in the parenthesis, essentially if they match. The example below is used in the MDN page for this method, and shows how it works nicely:
对于那些讨厌输入三个等号的人来说,这是一个不错的机会(请来JavaScript)。 .matches方法允许您检查使用该元素的元素是否由您最终将在括号中传递的字符串选择,如果它们匹配的话。 下面的示例在MDN页面中用于此方法,并显示了它如何正常工作:
var result = element.matches(selectorString);
I find that I’m often using this method when setting conditionals for EventListeners, to make sure an event only happens when a specific element is selected and no other. I’ve put an example from my blogging app below:
我发现为EventListeners设置条件时经常使用此方法,以确保仅在选择了特定元素时才发生事件,而没有其他事件发生。 我在下面的博客应用中举了一个例子:
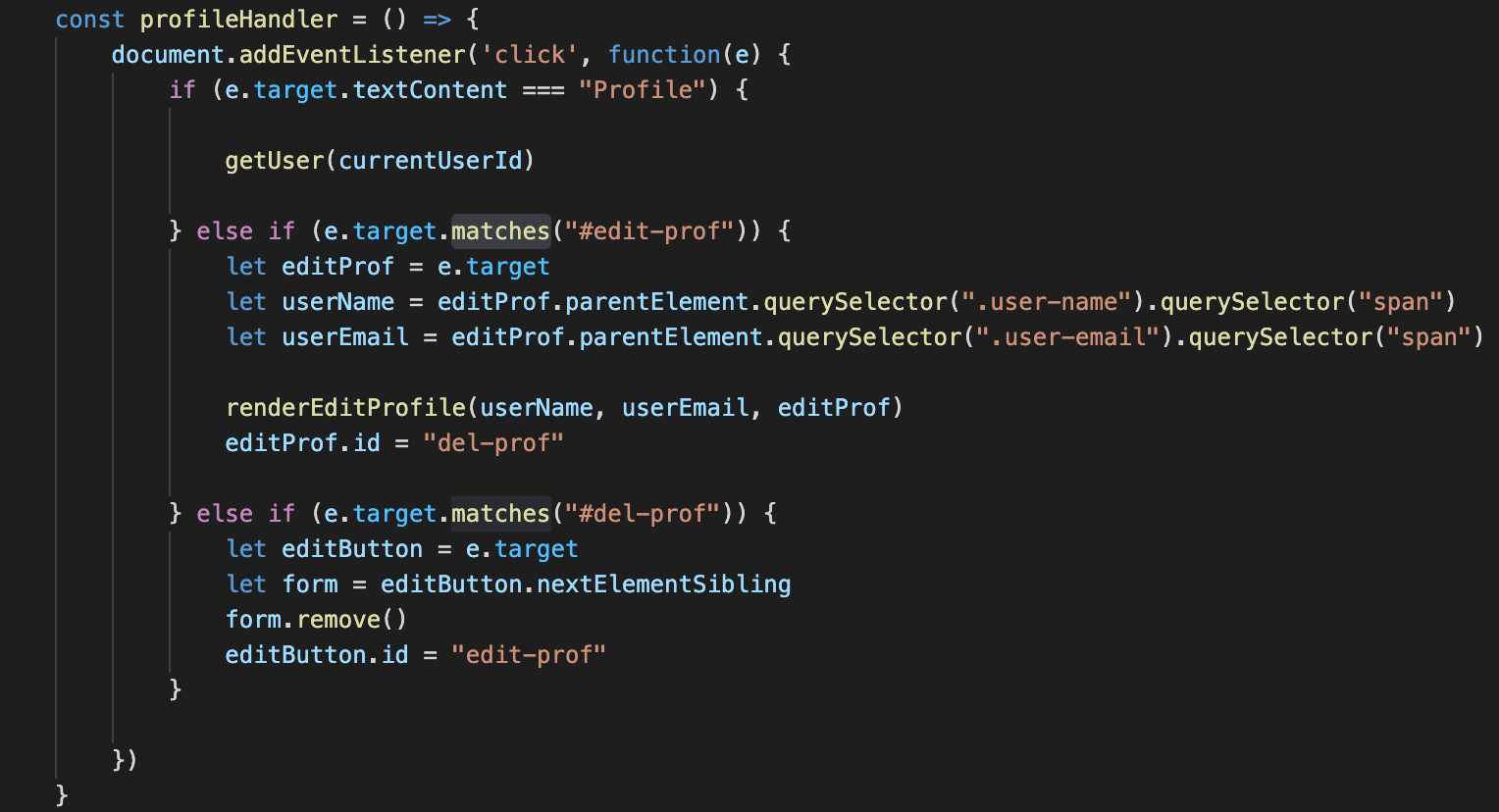
I included the whole function above, because it shows how .matches works in comparison to the triple equals as well as itself. For an EventListener like this, I use .matches to make sure that the target of my clicks is not only the correct element, but the correct class of the element in order to run my subsequent functions. I am toggling the same element’s class after each click, which is either creating or deleting a form. You can see the final result in the gif below, and it’s all thanks to .matches():
我在上面包括了整个函数,因为它显示了.matches与三重等于及其自身相比的工作方式。 对于这样的EventListener,我使用.matches来确保单击的目标不仅是正确的元素,而且是元素的正确类,以便运行我的后续功能。 每次单击后,我都在切换相同元素的类,即创建或删除表单。 您可以在下面的gif中查看最终结果,这全都归功于.matches():
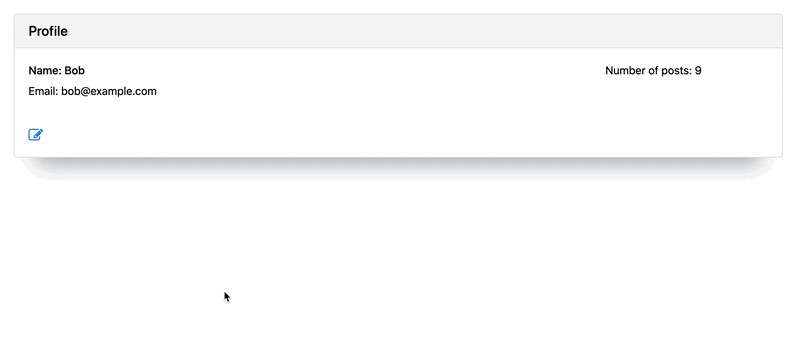
One last note about .matches(). It only works for selectors, so you can’t throw variable names into the parenthesis. It will not work, believe me, I have tried. Now, onto the next method!
关于.matches()的最后一点说明。 它仅适用于选择器,因此您不能将变量名放在括号中。 相信我,这已经行不通了。 现在,进入下一个方法!
.closest() (.closest())
By now, you probably have some basic understanding of .querySelector(). It is one of the first methods you learn upon being introduced to Vanilla JavaScript and DOM traversal and helps you locate the nearest/first child element of the element you call the method on. Since it so well covered and widely-used, we won’t cover it much more here. BUT, what if you’d like to traverse the DOM to find the nearest parent element. How does one do that? The answer my friends, lies in the .closest() method.
到目前为止,您可能已经对.querySelector()有了一些基本的了解。 这是在介绍Vanilla JavaScript和DOM遍历后学习的第一种方法,并且可以帮助您找到调用该方法的元素的最近/第一个子元素。 由于它涵盖的范围很广且使用广泛,因此我们在这里不再赘述。 但是,如果您想遍历DOM以找到最接近的父元素怎么办。 怎么做到的? 我的朋友们的答案在于.closest()方法。
Once again, MDN has our backs with a pretty solid demonstration of the structure of .closest. I’ve put it below:
再次, MDN对.closest的结构进行了非常扎实的演示。 我把它放在下面:
var closestElement = targetElement.closest(selectors);
As this demonstration shows, it essentially performs the same actions as .querySelector(), but instead of looking down into the children of the DOM tree, it looks up at the parent elements. But why not simply use .parentElement the more intrepid of you might ask? Wouldn’t that also help find the parentElement? This is true in some circumstances, but not all, and it is not always the most efficient solution. Another example from my application below will illustrate this difference.
如本演示所示,它实际上执行与.querySelector()相同的操作,但是它不查找DOM树的子级,而是查找父级元素。 但是,为什么不使用.parentElement ,您可能会问得更强悍呢? 那也不会有助于找到parentElement吗? 在某些情况下(并非全部),这是正确的,并且它并不总是最有效的解决方案。 下面我的应用程序中的另一个示例将说明这种差异。
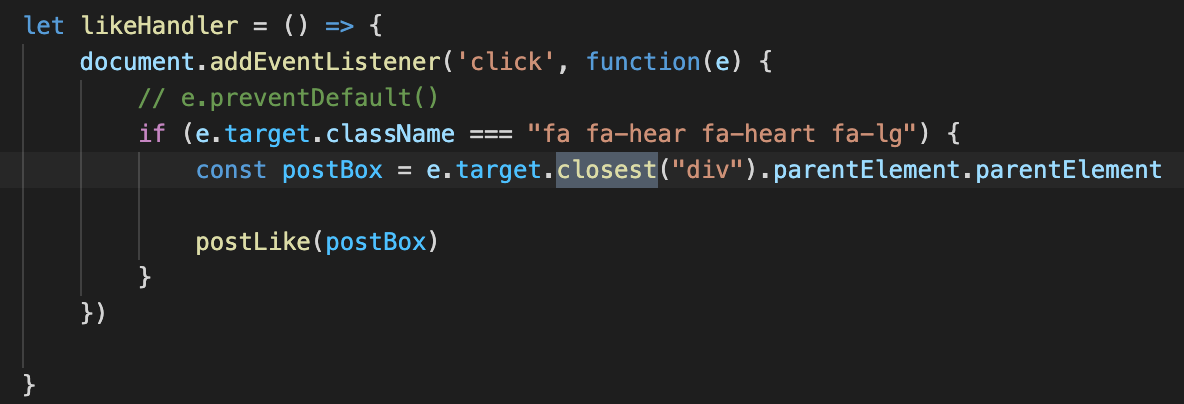
In this example .closest is being used to select the nearest div parent element. The “targets” in this instance could actually be more than one thing, a heart emoji, and a small border around it. To make sure that the user has the same experience no matter where they click on the heart, I used .closest to ensure that the result will be the same div. I then stacked parentElement methods on each other to show how inefficient using .parentElement can be, especially if you are moving multiple levels up the DOM tree. I would have been better off using .closest() again! Here is the simple like function in action, and it works if I click the heart perfectly, or just miss it:
在此示例中,.closest用于选择最接近的div父元素。 在这种情况下,“目标”实际上可能不止一件事,一个心形表情符号,以及围绕它的小边框。 为了确保用户无论在何处单击心脏都具有相同的体验,我使用.closest来确保结果将是相同的div。 然后,我彼此堆叠了parentElement方法,以展示使用.parentElement的效率如何,尤其是在将DOM树上的多个级别向上移动时。 我本来最好再使用.closest()! 这是运行中的简单“赞”函数,如果我完美地单击心脏或者只是错过心脏,它会起作用:
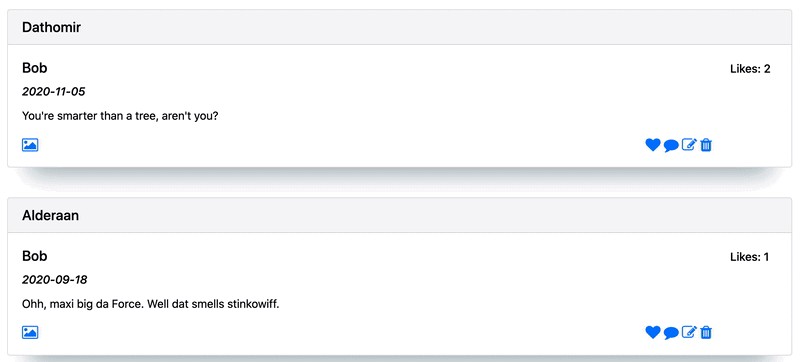
Amazing right? With just a few simple methods, Vanilla JavaScript is already feeling easier. Who needs React? Well, React is pretty awesome, but at the very least this should help you improve your ability to traverse the DOM with less headaches.
对不对? 仅使用一些简单的方法,Vanilla JavaScript就已经变得更容易了。 谁需要React? 好的,React非常棒,但是至少这应该可以帮助您以更少的麻烦来提高遍历DOM的能力。
To recap, .matches() is an excellent method to use in place of the triple equals. So long as you are looking for selectors, it should save you same headaches and some keystrokes when looking for a match on the DOM. And the .closest() is a fantastic way to traverse the DOM when you are looking for a parent element. It is essentially the opposite of .querySelector() as far as basic functionality goes.
回顾一下,.matches()是一种出色的方法,可以代替三等号。 只要您正在寻找选择器,当在DOM上寻找匹配项时,它就可以避免您同样的头痛和一些击键。 .closest()是在查找父元素时遍历DOM的一种绝妙方法。 就基本功能而言,它基本上与.querySelector()相反。
Happy coding!
编码愉快!
翻译自: https://medium.com/dev-genius/traversing-the-dom-with-vanilla-javascript-3ce8f025682f
keras 香草编码器