react 打字机效果
Make a front-end with react in 10 minutes.
在10分钟内做出React。
When I was kicking off with this stack I had a lot of naive questions that never got answered until I was forced into working on some giant monolithic project for a while.
当我从这个堆栈开始时,我有很多天真的问题,直到我被迫从事某个大型整体项目一段时间后,这些问题才得到解答。
Where do my CSS files go?
我CSS文件在哪里?
How do I modularize things?
如何对事物进行模块化?
What is my repo supposed to look like?
我的仓库应该是什么样的?
I’ll prevent you from having those questions. For this tutorial, install vscode, npm, node, typescript.
我将阻止您提出这些问题。 对于本教程,请安装vscode,npm,node,打字稿。
Navigate to a directory. Type this:
导航到目录。 输入:
npx create-react-app application-name --template typescriptcd application namecode .
Our default repo looks like this.
我们的默认存储库如下所示。
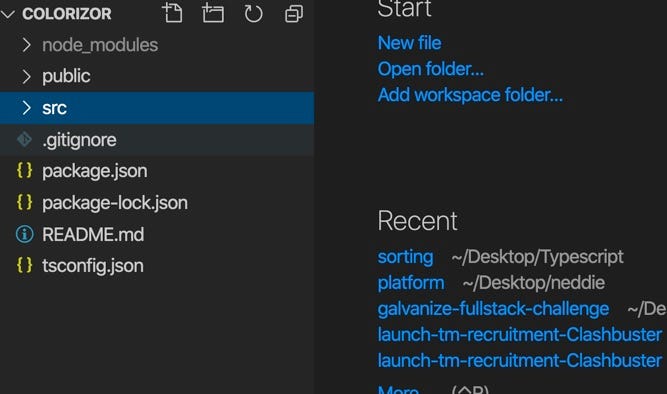
Navigate into the src directory.
导航到src目录。
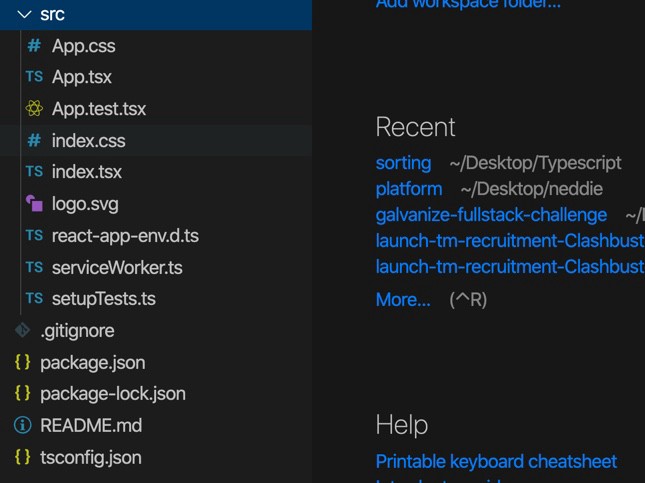
Look at all that bloatware. Using the npx create react app tool does a lot of nice things for us. It sets up our environment and automatically builds all these files, and by default, it will even set up some testing stuff.
看看所有的膨胀软件。 使用npx create react应用程序工具对我们来说有很多好处。 它设置了我们的环境并自动构建了所有这些文件,默认情况下,它甚至还会设置一些测试内容。
Once we get to this point it is important to take ownership of your project and change it however you want to make it organized. What I’m going to do is delete these files.
一旦达到这一点,重要的是要获得项目的所有权并进行更改,但是要使其组织起来。 我要做的是删除这些文件。
App.css
App.test.tsx
index.css
logo.svg
serviceWorker.ts
setupTests.ts
That stuff is useful, but there are three reasons why we don’t need it.
这些东西很有用,但是有三个原因使我们不需要它。
- If you don’t know anything about tests you’re probably better off setting them aside until you invest in setting them up properly. 如果您对测试一无所知,则最好将它们搁置一旁,直到您投资适当地设置它们为止。
- Tests are mandatory for enterprise projects, but You won’t need any tests (or any other scaling solutions like redux) unless your project grows to a certain point. If your project has less than ~15 components, Redux/tests might cause more trouble than they are worth. 测试对于企业项目是必不可少的,但是除非您的项目发展到一定程度,否则您将不需要任何测试(或任何其他伸缩解决方案,例如redux)。 如果您的项目少于15个组件,则Redux /测试可能会造成超出其价值的麻烦。
- I like to delete the CSS files because I prefer to organize them differently. 我喜欢删除CSS文件,因为我喜欢以不同的方式组织它们。
Now, our repo looks like this.
现在,我们的仓库看起来像这样。
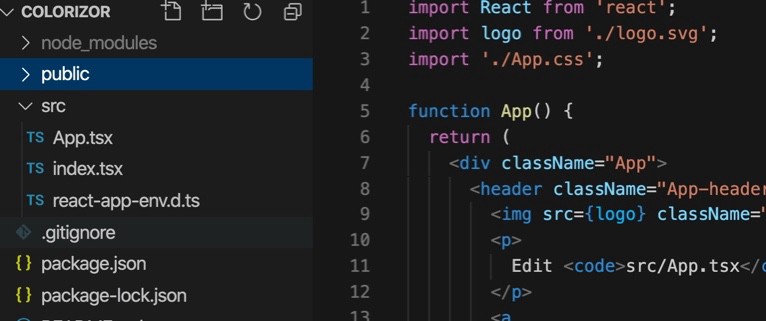
Click on App.tsx and delete everything inside of our App.tsx return statement and our logo import until it looks like this.
单击App.tsx并删除App.tsx返回语句和徽标导入中的所有内容,直到看起来像这样。
Navigate into your public directory. It looks like this:
导航到您的公共目录。 看起来像这样:
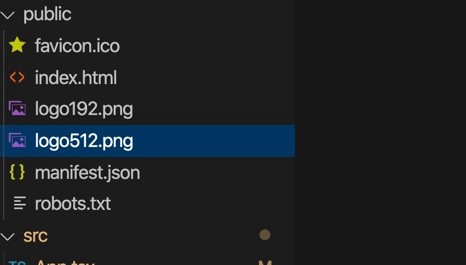
Delete both of those logo.png files and open up your index.html.
删除这两个logo.png文件,然后打开index.html。
There are a couple of little quality of life things we want to change in our index.html.
我们希望在index.html中改变一些生活质量低下的东西。
This is what your index.html file looks like and I’ve highlighted the things that you should consider.
这就是您的index.html文件的外观,并且我着重强调了您应考虑的事项。
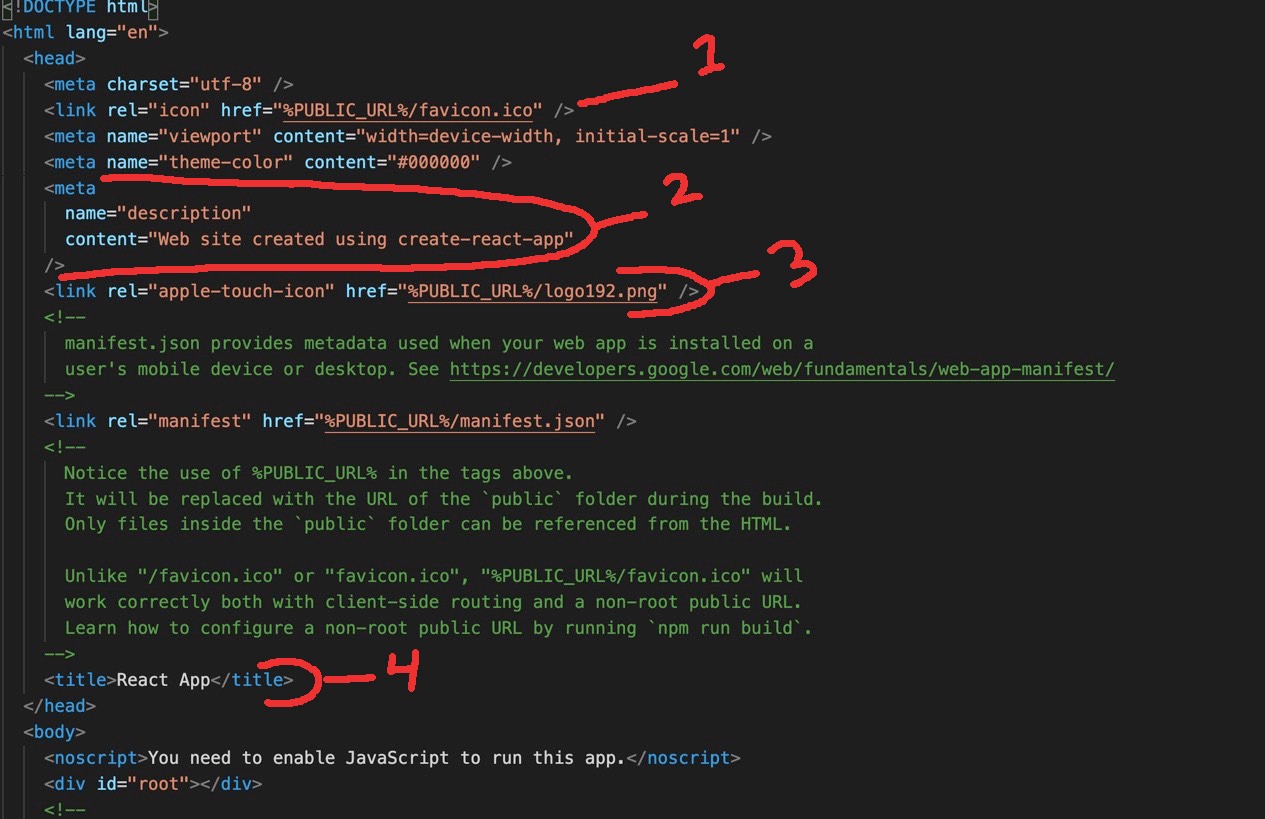
- Whatever photo/icon is being linked is what will appear on your chrome browser tab 链接的任何照片/图标都将出现在Chrome浏览器标签上
- Whatever string is in the content section will show up when you link your website in a lot of major social media locations (like discord) if you don’t change it, it will say “Web site created using create-react-app” when you try to share the link to your new website to your friends. I’d recommend changing it to something sporty like “Taylor’s personal website” 当您在许多主要的社交媒体位置(例如不和谐)上链接您的网站时,无论内容部分中的字符串是什么,如果您不进行更改,它将显示“使用create-react-app创建的网站”您尝试将新网站的链接分享给您的朋友。 我建议将其更改为类似“ Taylor的个人网站”之类的运动内容
- Whatever is linked here will show up on IOS devices. 此处链接的所有内容都会在IOS设备上显示。
Whatever is in the <title></title> tag is what will appear on your chrome browser tab.
<title> </ title>标记中的内容将显示在chrome浏览器标签上。
根据需要更改所有这些。 (Change all of those as you wish.)
让我们谈谈React (Let’s talk about react)
Now, we’ve deleted all of the bloatware and we have a barebones empty react app with which to make stuff.
现在,我们已经删除了所有的膨胀软件,并且有了一个空的React应用程序来制作东西。
Let’s have a discussion about how to make stuff appear in our browser window using react.
让我们讨论一下如何使用react使内容出现在浏览器窗口中。
Everything that appears in our browser is either an HTML document or it’s something that compiles down to HTML. Everything that changes how our HTML looks is either CSS or something that compiles down to CSS.
浏览器中显示的所有内容都是HTML文档,或者是可以编译为HTML的内容。 改变我们HTML外观的一切都是CSS或编译为CSS的东西。
Everything that dynamically changes our HTML is Javascript or something that compiles down to Javascript.
动态更改我们HTML的所有内容都是Javascript或编译为Javascript的内容。
Typescript compiles down to Javascript. JSX is a sub-domain that compiles down to HTML. TSX compiles down to JSX and therefore down to HTML. If we decided to use any CSS-preprocessors like SASS, that would all compile down to CSS, but for this tutorial, I’m going to use regular CSS.
Typescript会编译为Javascript。 JSX是可编译为HTML的子域。 TSX编译为JSX,因此编译为HTML。 如果我们决定使用SASS之类CSS预处理器,那么它们都将编译为CSS,但是在本教程中,我将使用常规CSS。
React is a component-based rendering delivery system. You might have seen some other such systems because it’s the world’s favorite type of rendering framework right now (look at Vue, Angular, Unity etc.) They’re all component-based systems.
React是一个基于组件的渲染交付系统。 您可能已经看过其他一些这样的系统,因为它是当前世界上最受欢迎的渲染框架类型(请查看Vue,Angular,Unity等)。它们都是基于组件的系统。
The way we organize our components is the same way we would organize our HTML in a legacy vanilla JS project. The major difference is with legacy JS we have a monolithic HTML file that may or may not be separated into segments.
组织组件的方式与在原始Vanilla JS项目中组织HTML的方式相同。 主要区别在于,与旧版JS相比,我们拥有一个整体HTML文件,该文件可能会也可能不会分成几部分。
The difference with a component-based system is we atomize our HTML segments into components that all have their own specific JS sections and CSS references.
与基于组件的系统的不同之处在于,我们将HTML段细分为所有具有各自特定的JS部分和CSS引用的组件。
For example, here is my personal website except I’ve put a red checkmark at each div that is it’s own modulized component. Each check represents a whole react module with its own functionality.
例如,这是我的个人网站,只不过我在每个div上都添加了一个红色的选中标记,它是它自己的调制组件。 每个检查代表一个具有自己功能的整个react模块。
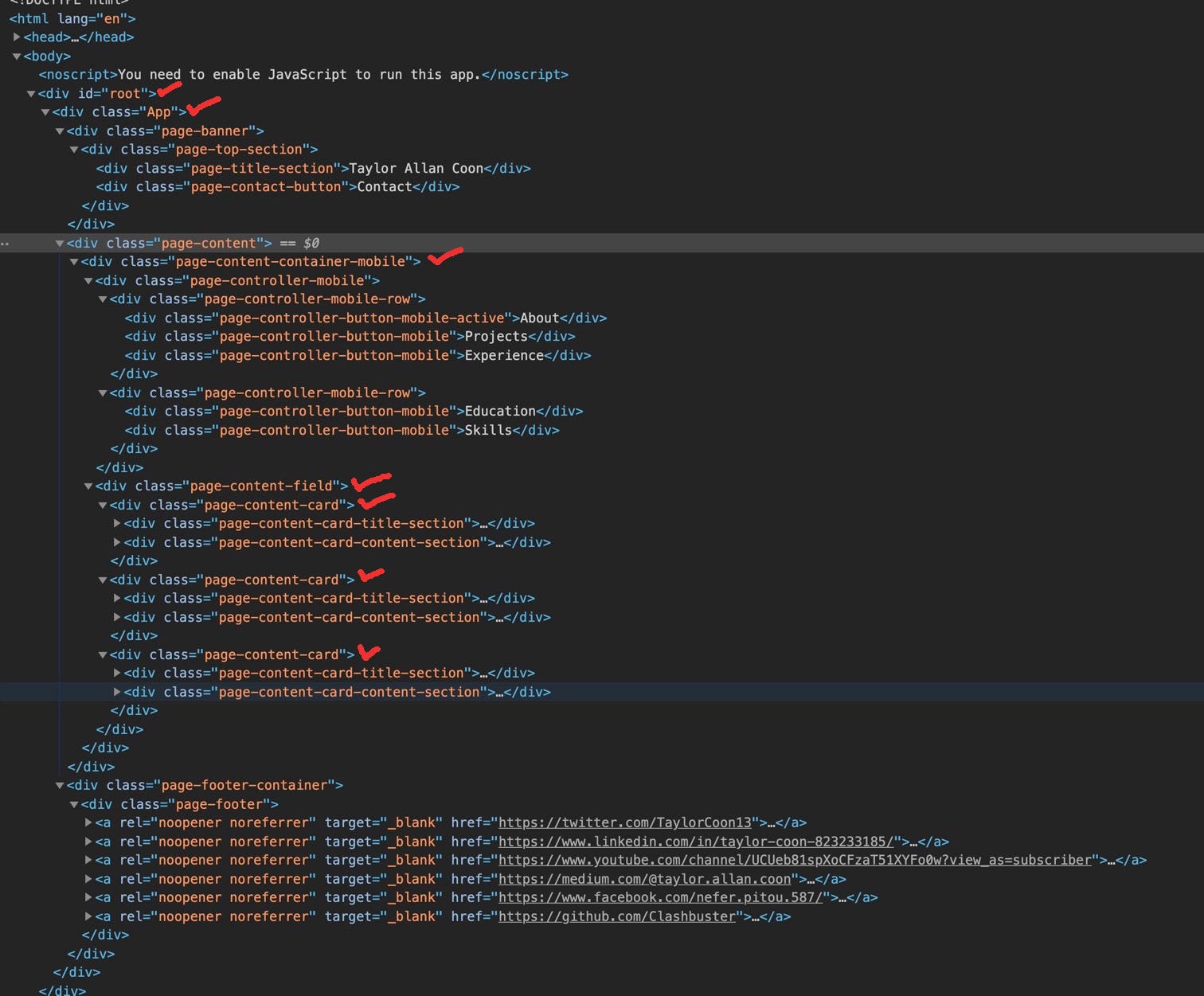
In order to render whatever we want in React all we have to do is reference specific components and inject them into our return statements for each module.
为了在React中呈现我们想要的一切,我们要做的就是引用特定的组件,并将它们注入到每个模块的return语句中。
I’m going to use React hooks and function components only because I think they’re way easier, lighter, and cleaner than state components. Also, Javascript is a functional language and it just seems right.
我将使用React钩子和函数组件仅仅是因为我认为它们比状态组件更容易,更轻便,更干净。 另外,Javascript是一种功能语言,看起来似乎是正确的。
What I’m going to do is find an open-source and free web-API with some data using this link.
我要做的是使用此链接找到带有一些数据的开源和免费的Web API。
Out of that list, I chose the bored API. I’m going to query it and make a small randomized list of activities I can do when I’m bored.
从该列表中,我选择了无聊的API。 我要查询它,并在无聊时做一些随机的活动。
I’m going to make a very small and simple application that will demonstrate the fundamentals of React. With this knowledge, you can expand indefinitely.
我将制作一个非常小而简单的应用程序,以演示React的基础知识。 有了这些知识,您可以无限扩展。
组织- (Organization —)
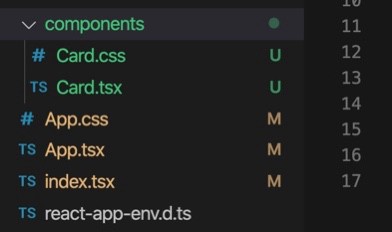
The first thing we can see is I have organized my repository to keep components in a separate folder. Additionally, every component references its own CSS file and the CSS file is coupled with the module inside of the directory.If I had several more components or several more front-end pages, I would organize these even deeper. Where each page has its own directory and each component on each page has its own directory inside of each page directory.
我们可以看到的第一件事是,我已经组织好了我的存储库,以将组件保存在单独的文件夹中。 此外,每个组件都引用自己CSS文件,并且CSS文件与目录内的模块耦合在一起。如果我拥有更多组件或更多前端页面,我将对它们进行更深入的组织。 每个页面都有自己的目录,每个页面上的每个组件在每个页面目录中都有自己的目录。
每个模块的作用 (What each Module does)
Generally, Each module will only have boiler-plate code for whatever it is specifically handling, don’t mix this up. Here is what each module will generally look like.
通常,每个模块仅包含具体处理的样板代码,请勿混淆。 这是每个模块的大致外观。
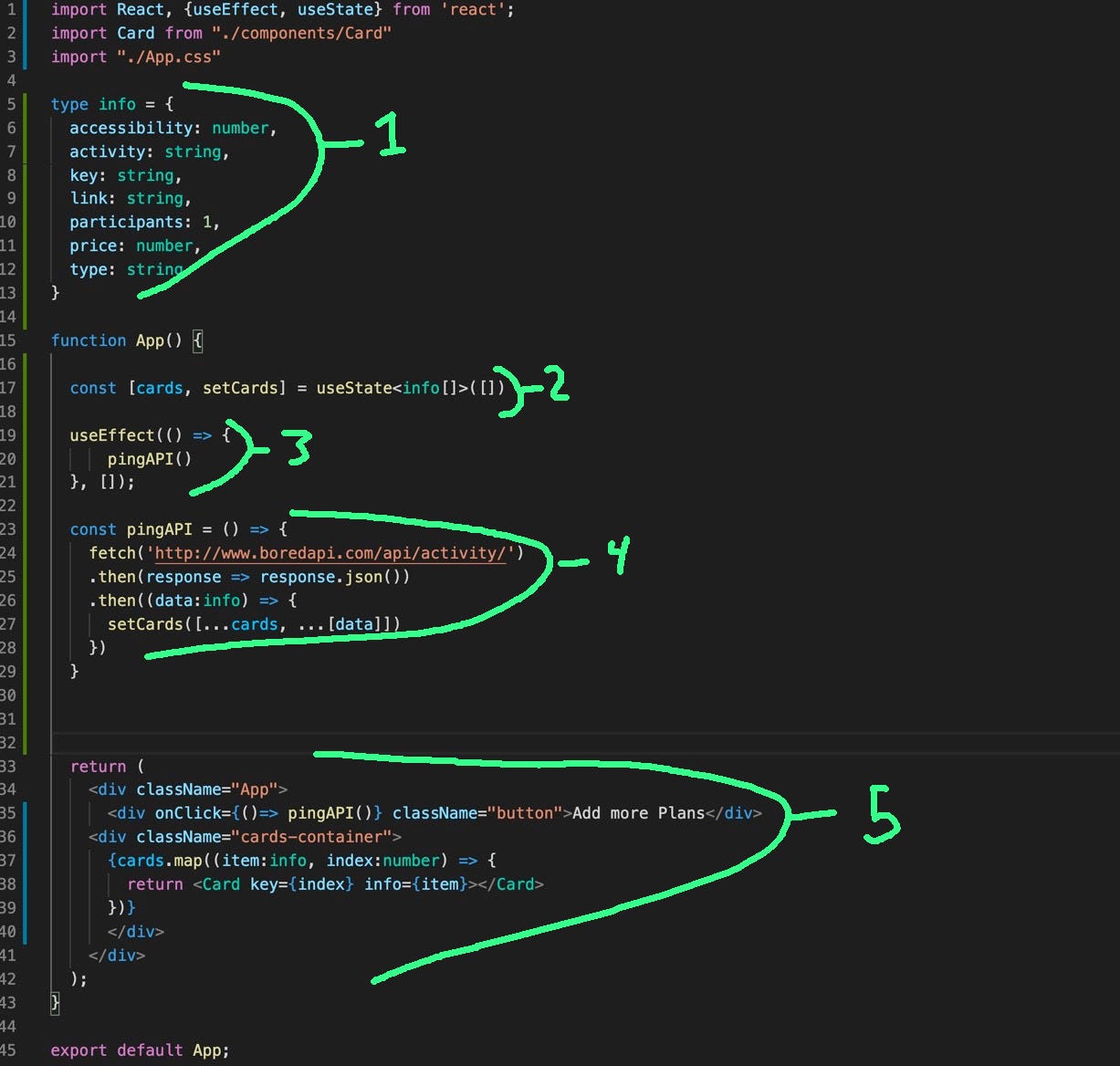
- Some Type declarations to keep the typescript compiler happy. 一些Type声明使Typescript编译器满意。
- State management is all about managing something that will “change state.” When something about this component changes, the state of this component has changed. I have created a single state variable with react hooks. Since the only thing about this page that will change is the number of tasks I have fetched, it’s the only variable I need. 状态管理就是管理“改变状态”的事情。 当有关此组件的某些更改时,此组件的状态也已更改。 我已经创建了一个带有状态钩子的状态变量。 由于此页面上唯一会改变的是我已获取的任务数,因此这是我唯一需要的变量。
- Initial fetch so that the page starts with one card. 初始读取,以便页面以一张卡片开始。
- handler for fetching that updates state when it is called. 用于在调用状态时获取更新状态的处理程序。
- This is the return statement for our module which contains all of the HTML material that will shop up in this component. Considering this is the parent component, it contains all of the cards as well. Since the Cards are their own component, I am referencing the cards in the imports and creating as many as I need depending on how many items are in the state variable. 这是我们模块的return语句,其中包含将在此组件中购买的所有HTML材料。 考虑到这是父组件,它也包含所有卡。 由于Cards是它们自己的组件,因此我将引用导入中的Cards并根据状态变量中的项目数创建所需的数量。
在我CSS中 (Inside my CSS)
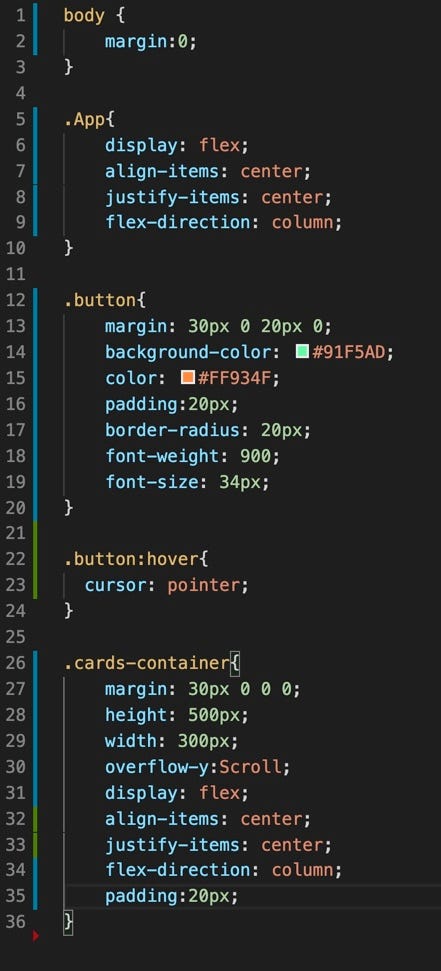
This photo contains 100% of the CSS used on my parent app component. Notice that it is not very long. We like that. If anybody wanted to come later and fix my code they would only have to read through ~30 lines of code.
这张照片包含我的父应用程序组件上使用CSS的100%。 请注意,它不是很长。 我们喜欢那样。 如果有人想稍后再修复我的代码,他们只需要阅读大约30行代码即可。
They would know exactly where to look because if there are any visual bugs they can see it relevant to a specific component on the page and navigate to this directory.
他们会确切地知道在哪里寻找内容,因为如果有任何视觉错误,他们可以看到与页面上特定组件相关的内容,并导航到该目录。
All the other CSS is kept in my Card.css file inside of the Card directory.
所有其他CSS都保存在Card目录中我的Card.css文件中。
最终产品 (The final product)
And there we have it. A basic React front end with typescript with good organizational habits.
我们终于得到它了。 一个基本的React前端,带有良好的组织习惯的打字稿。
Enjoy that weekend!
周末愉快!
翻译自: https://levelup.gitconnected.com/typescript-make-a-front-end-with-react-in-10-minutes-94f034200b5b
react 打字机效果