介绍 (Introduction)
In redux, we always need middleware to help us to handle the asynchronous operations or other side effects.
在redux中,我们始终需要中间件来帮助我们处理异步操作或其他副作用。
The most popular middlewares are Redux-thunk and Redux-saga.
最受欢迎的中间件是Redux-thunk和Redux-saga 。
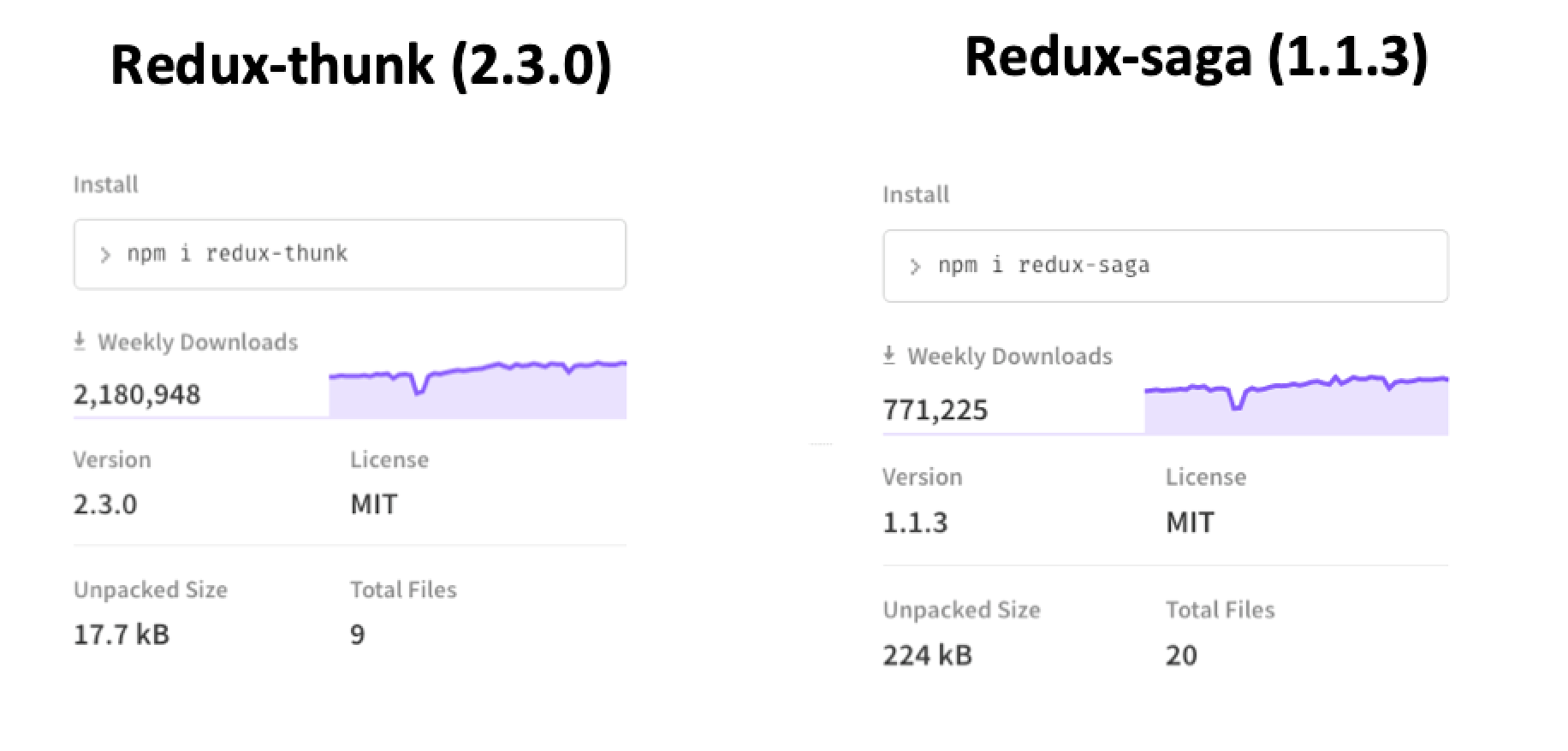
This topic notes the differences between Redux-thunk and Redux-saga for using. We will discuss the following items.
本主题记录了Redux-thunk和Redux-saga之间的区别。 我们将讨论以下项目。
- Overview 总览
- Roles and usages 角色和用法
- Libraries 图书馆
- Implementations 实作
总览 (Overview)
定义 (Definitions)
Redux Thunk middleware allows you to write action creators that return a function instead of an action. The thunk can be used to delay the dispatch of an action, or to dispatch only if a certain condition is met.
Redux Thunk 中间件使您可以编写返回函数而不是动作的动作创建者 。 重击程序可用于延迟操作的分发,或者仅在满足特定条件时调度。
Redux-saga (the definitions from redux-saga.js.org)
Redux-saga(来自redux-saga.js.org的定义)
redux-saga
is a library that aims to make application side effects (i.e. asynchronous things like data fetching and impure things like accessing the browser cache) easier to manage, more efficient to execute, easy to test, and better at handling failures.
redux-saga
是一个旨在使应用程序副作用(即,诸如数据获取之类的异步事物和诸如访问浏览器缓存之类的不纯之物)更易于管理,更有效地执行,易于测试以及更好地处理故障的库。
文件结构 (File structures)
The following figure is the figure I made for learning the Redux-thunk and Redux-saga. We can discover a few different things for their structure.
下图是我用来学习Redux-thunk和Redux-saga的图 。 我们可以发现它们结构的一些不同之处。
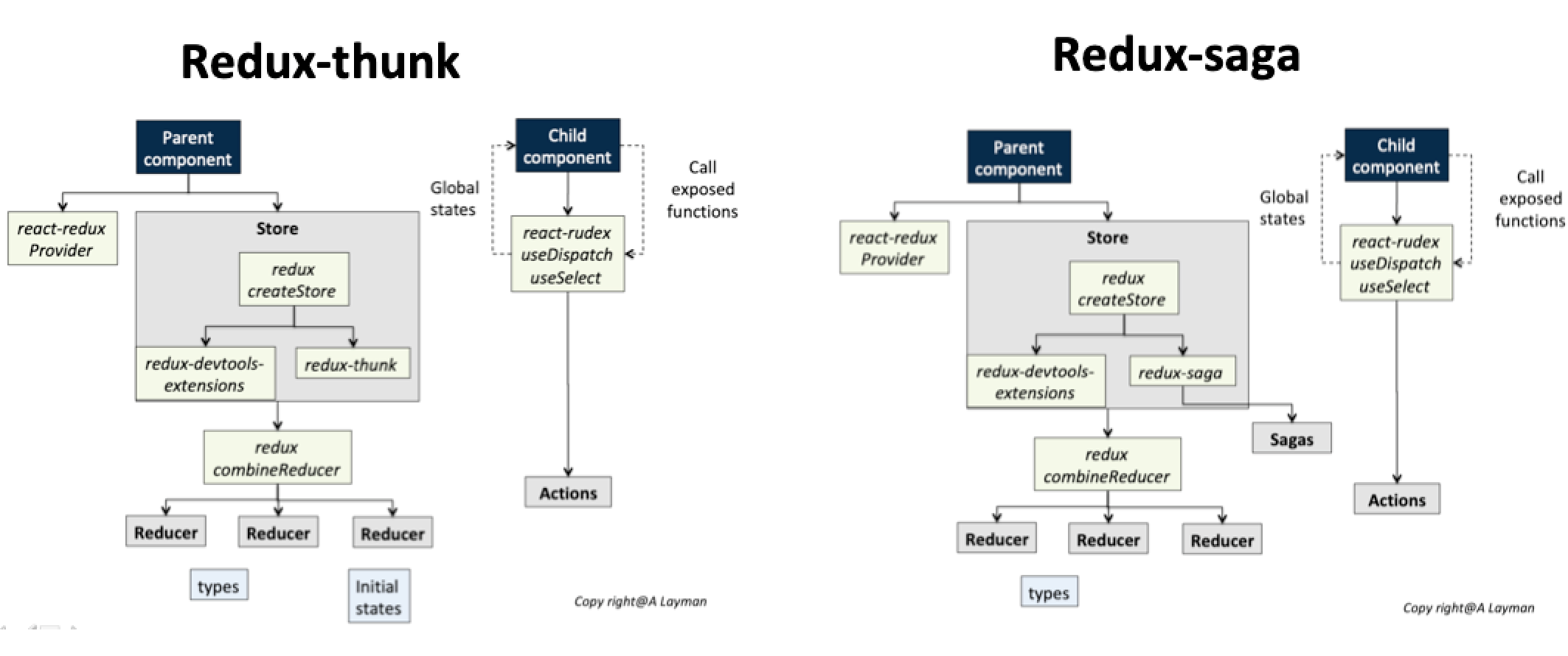
角色和用法 (Roles and usages)
I refer to Redux Saga with React: Fast-track Redux Saga intro course for the example and the source code for the Redux-saga part.
我通过示例参考Redux Saga和React:Redux Saga快速入门入门课程以及Redux-saga部分的源代码。
减速器 (Reducers)
- Reducer decide which state to change depends on the type of actions 减速器根据操作类型决定要更改的状态
For Redux-thunk, we usually have one state for a request(start)
对于Redux-thunk ,我们通常对一个请求有一个状态( 开始)
For Redux-saga, we need two states for a request(start and success)
对于Redux-saga ,我们需要一个请求的两个状态( 开始和成功)
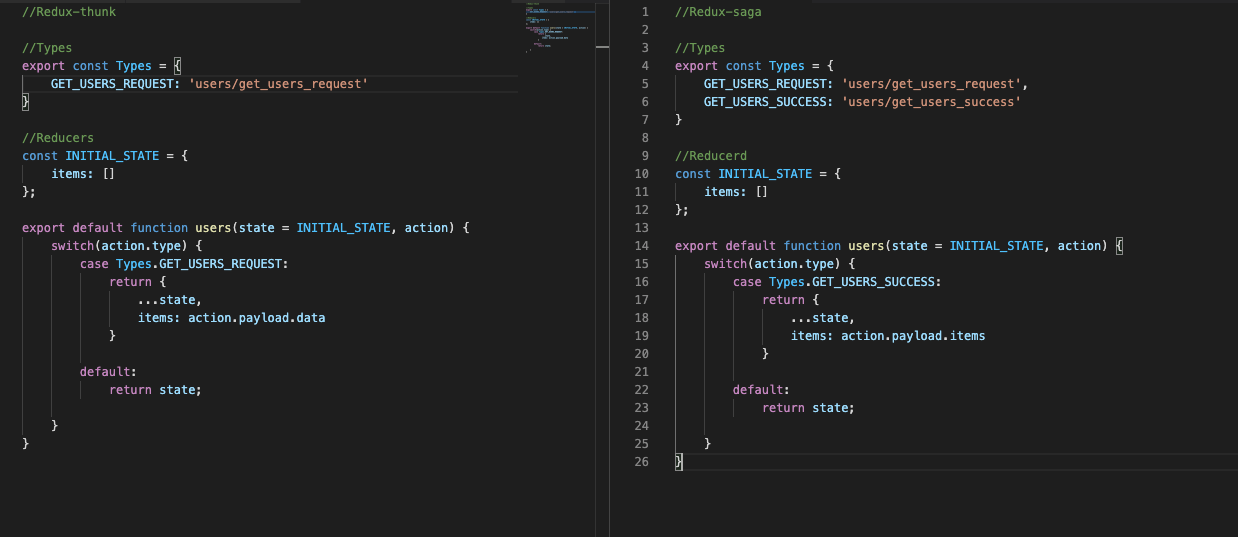
动作 (Actions)
Redux’s actions are exposed functions
Redux的动作是公开的功能
For Redux-thunk, the exposed functions call dispatch to change global states
对于Redux-thunk ,公开的函数调用dispatch来更改全局状态
For Redux-saga, the exposed functions only return JSON
对于Redux-saga ,公开的函数仅返回JSON
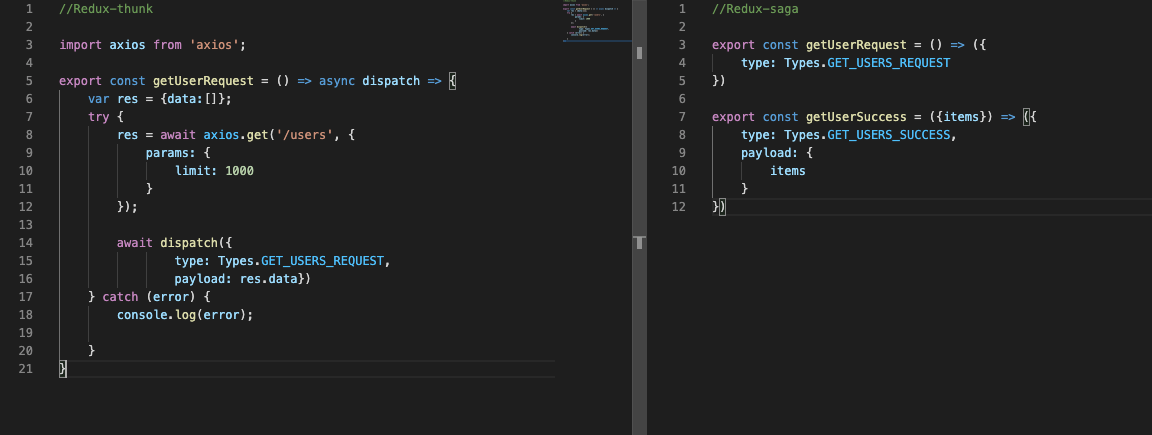
商店 (Store)
For Redux-thunk, we have to create an initial state and integrate it with thunk in the store.
对于Redux-thunk ,我们必须创建一个初始状态并将其与商店中的thunk集成。
For Redux-saga, we have to create sagas with generator functions and integrate them with the saga middleware in the store.
对于Redux-saga ,我们必须创建具有生成器功能的sagas,并将其与商店中的saga中间件集成。
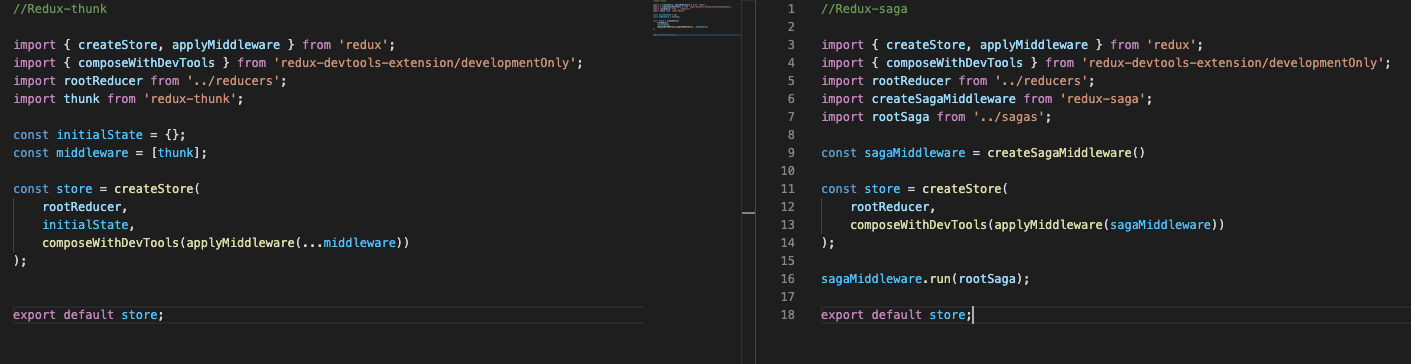
Sagas(仅需要React-saga需求) (Sagas (Only React-saga needs))
For Redux-saga, we have to create generator functions such as watcher and fetch resources here.
对于Redux-saga ,我们必须创建生成器函数(例如watcher)并在此处获取资源。
import { takeEvery, call, fork, put } from 'redux-saga/effects';
import * as actions from '../actions/users';
import * as api from '../api/users';
import axios from 'axios';
export const getUsers = () => {
return axios.get('/users', {
params: {
limit: 1000
}
})
}
function* getUsers() {
try{
const result = yield call(api.getUsers);
yield put(actions.getUserSuccess({
items: result.data.data
}));
} catch(e) {
}
}
function* watchGetUsersRequest() {
yield takeEvery(actions.Types.GET_USERS_REQUEST, getUsers);
}
const usersSagas = [
fork(watchGetUsersRequest)
];
export default usersSagas;
州 (State)
- Create states selectors here 在此处创建状态选择器
//Selector functions
export const selectUsersState = (rootState) => rootState.users.items;
子组件 (Child components)
There are no differences between Redux-thunk and Redux-saga
Redux-thunk和Redux-saga之间没有区别
import React, {useEffect, useState} from 'react'
//import { connect } from 'react-redux';
import { useDispatch, useSelector } from 'react-redux';
import {
getUserRequest
} from '../actions/users';
function App() {
const disPatch = useDispatch();
useEffect(()=> {
disPatch(getUserRequest())
}, [])
return (
<div />
);
}
export default App;
父组件 (Parent components)
There are no differences between Redux-thunk and Redux-saga
Redux-thunk和Redux-saga之间没有区别
import React from 'react';
import ReactDOM from 'react-dom';
import App from './components/App';
import axios from 'axios';
import { Provider } from 'react-redux';
import store from './store/store'
axios.defaults.withCredentials = true;
axios.defaults.baseURL = 'https://rem-rest-api.herokuapp.com/api';
ReactDOM.render(
<Provider store = {store}>
<App />
</Provider>,
document.getElementById('root')
);
图书馆 (Libraries)
Suppose we use create-react-app (CRA)
假设我们使用create-react-app(CRA)
- For redux-thunk, we have to install the following libraries 对于redux-thunk,我们必须安装以下库
npm i -S redux react-redux redux-thunk redux-devtools-extension
- For redux-saga, we have to install the following libraries 对于redux-saga,我们必须安装以下库
npm i -S redux react-redux redux-saga redux-devtools-extension
摘要 (Summary)
Thanks for your patient. I am Sean. I work as a software engineer.
谢谢你的耐心。 我是 肖恩 。 我是一名软件工程师。
This article is my note. Please feel free to give me advice if any mistakes. I am looking forward to your feedback.
这篇文章是我的笔记。 如果有任何错误,请随时给我建议。 期待您的反馈。
- The Facebook page for articles Facebook专页页面
- The latest side project: Daily Learning 最新的辅助项目:日常学习
相关话题 (Related topics)
How to use the two-way binding in Knout.js and ReactJS?
如何在Knout.js和ReactJS中使用双向绑定?
Learn how to use SignalR to build a chatroom application
了解如何使用SignalR构建聊天室应用程序
My reflection of <Effective SQL>:
我对<有效SQL>的反映:
IT & Network:
IT与网络:
Database:
数据库:
Software testing:
软件测试:
Debugging:
调试:
DevOps:
DevOps: