An introduction to cloud-based testing on BrowserStack using Selenium in Python.
在Python中使用Selenium对BrowserStack进行基于云的测试的简介。
An introduction to cloud-based testing on BrowserStack using Selenium in Python. Selenium is a library that enables the automation of web browsers. Testing web applications is its most popular use. At the heart of Selenium lies the Selenium WebDriver. WebDriver uses browser automation APIs to control the browser and run tests. This feature empowers the automation of a browser. You can use these features to automate most of your boring stuff online, such as applying to multiple jobs on LinkedIn and AngelList.
在Python中使用Selenium对BrowserStack进行基于云的测试的简介。 Selenium是一个使Web浏览器自动化的库。 测试Web应用程序是其最流行的用途。 Selenium WebDriver是Selenium的核心。 WebDriver使用浏览器自动化API来控制浏览器并运行测试。 此功能可实现浏览器的自动化。 您可以使用这些功能在线上自动执行大多数无聊的工作,例如在LinkedIn和AngelList上申请多个职位。
This article is about using Selenium (Python) to automate a form-filling process, i.e., filling a form multiple times. After that, we step things up a notch using BrowserStack to take this automation process to the cloud. BrowserStack is a cloud web and mobile testing platform that lets developers access browsers on-demand for their testing needs.
本文是关于使用Selenium(Python)来自动执行表单填充过程,即多次填充表单。 在那之后,我们使用BrowserStack将这一自动化过程推向了云端,从而提高了水平。 BrowserStack是一个云Web和移动测试平台,开发人员可以使用该浏览器按需访问其测试需求的浏览器。
Cloud-based automation and testing have several benefits. It offers better collaboration, quicker and cost-effective testing, unlimited availability, and some significant advantages like customisation, parallelisation and support for agile workflows.
基于云的自动化和测试有几个好处。 它提供了更好的协作,更快,更具成本效益的测试,无限的可用性以及一些重要的优势,例如定制,并行化以及对敏捷工作流的支持。
For this article, we will be using a sample Google form.
对于本文,我们将使用示例Google 表单。
The first and the most obvious step would be to install Selenium on your system by using the following command in your terminal:
第一步也是最明显的步骤是在终端中使用以下命令在系统上安装Selenium:
sudo pip3 install selenium
The next step would be to create a BrowserStack account (If you have one already, then that’s great). BrowserStack’s Automate Mobile Plan for one parallel and one user for one year is free for students through the Github Student Developer Pack.
下一步将是创建一个BrowserStack帐户(如果已经拥有一个帐户,那就太好了)。 通过Github Student Developer Pack ,学生可以免费使用BrowserStack的针对一名并行用户和一名用户的自动移动计划(一年)。
You would then need to head to this page on BrowserStack’s website. Here you can select the browser and OS combination of your preference. After you’ve chosen your choice, you would then need to create a new .py file on your system in a code editor. Let’s say you wish to perform this test on Chrome 84 Browser on Mac OS X Catalina. So you should write down the following code in your .py file:
然后,您需要访问BrowserStack网站上的此页面 。 在这里,您可以选择自己喜欢的浏览器和操作系统组合。 选择完毕后,您将需要在系统中的代码编辑器中创建一个新的.py文件。 假设您希望在Mac OS X Catalina上的Chrome 84浏览器上执行此测试。 因此,您应该在.py文件中写下以下代码:
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
from selenium.webdriver.common.desired_capabilities import DesiredCapabilities
import time
desired_cap = {
'browser': 'Chrome',
'browser_version': '84.0 beta',
'os': 'OS X',
'os_version': 'Catalina',
'resolution': '1024x768',
'name': 'Bstack-[Python] Sample Test'
}
driver = webdriver.Remote(
command_executor='Your unique BrowserStack Access Key link',
desired_capabilities=desired_cap)
The first four lines of the code specify the imported libraries. The time library will be used later on in the project. The desired cap dictionary specifies the specifications of the OS and Browser you want to use. The next statement ( webdriver.Remote() ) connects the program to the BrowserStack’s system. The unique string to be passed to the command_executor parameter would be found on this page when you select your Browser and OS preference. The access key is unique to each BrowserStack user, and so is the link.
代码的前四行指定了导入的库。 时间库将在项目的后面使用。 所需的上限字典指定您要使用的OS和浏览器的规格。 下一条语句( webdriver.Remote() )将程序连接到BrowserStack的系统。 当选择浏览器和操作系统首选项时,将在此页面上找到要传递给command_executor参数的唯一字符串。 访问密钥对于每个BrowserStack用户都是唯一的,链接也是如此。
After this, you need to add the following python code to your .py file.
之后,您需要将以下python代码添加到.py文件中。
driver.get('https://forms.gle/xX5NEumdXW4Z7SrC6')
The driver.get() statement will open the link provided in the browser. The screenshot of the form is attached below.
driver.get()语句将打开浏览器中提供的链接。 表格的屏幕截图如下。
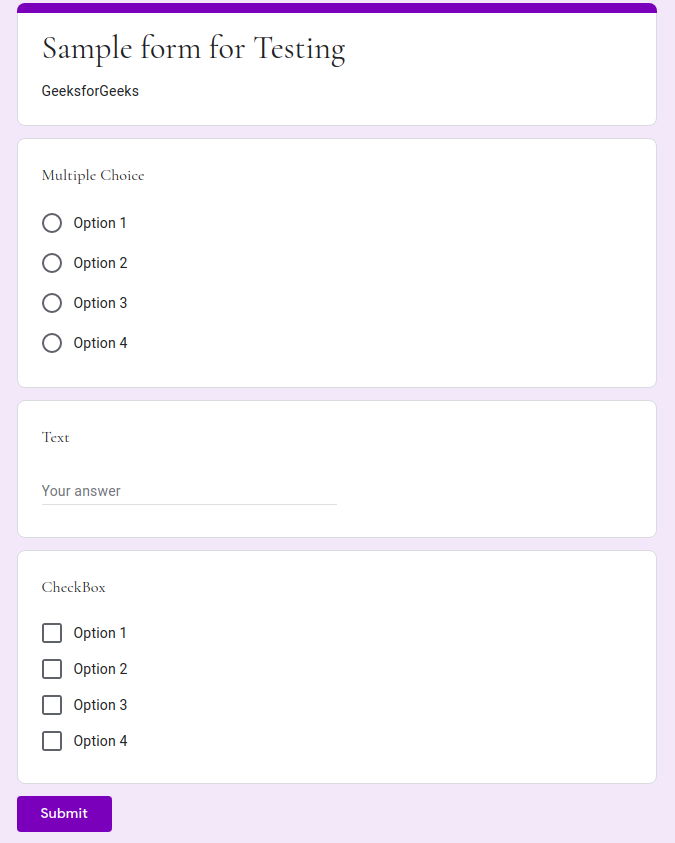
Now your next step would be to select the correct options for each of the questions/fields. There are several ways to do this, but let’s say you use the XPath to choose your desired option. For this, you need to hover over to the option of your choice and press the mouse’ right-click over the radio button/checkbox. Then you would need to select the inspect option. When you right-click on the highlighted element inside the new pane on the right, you would be able to copy the XPath, as shown in the picture below.
现在,您的下一步将是为每个问题/领域选择正确的选项。 有几种方法可以做到这一点,但是假设您使用XPath选择所需的选项。 为此,您需要将鼠标悬停在您选择的选项上,然后在鼠标单选按钮/复选框上单击鼠标右键。 然后,您需要选择检查选项。 右键单击右侧新窗格内突出显示的元素时,您将能够复制XPath,如下图所示。
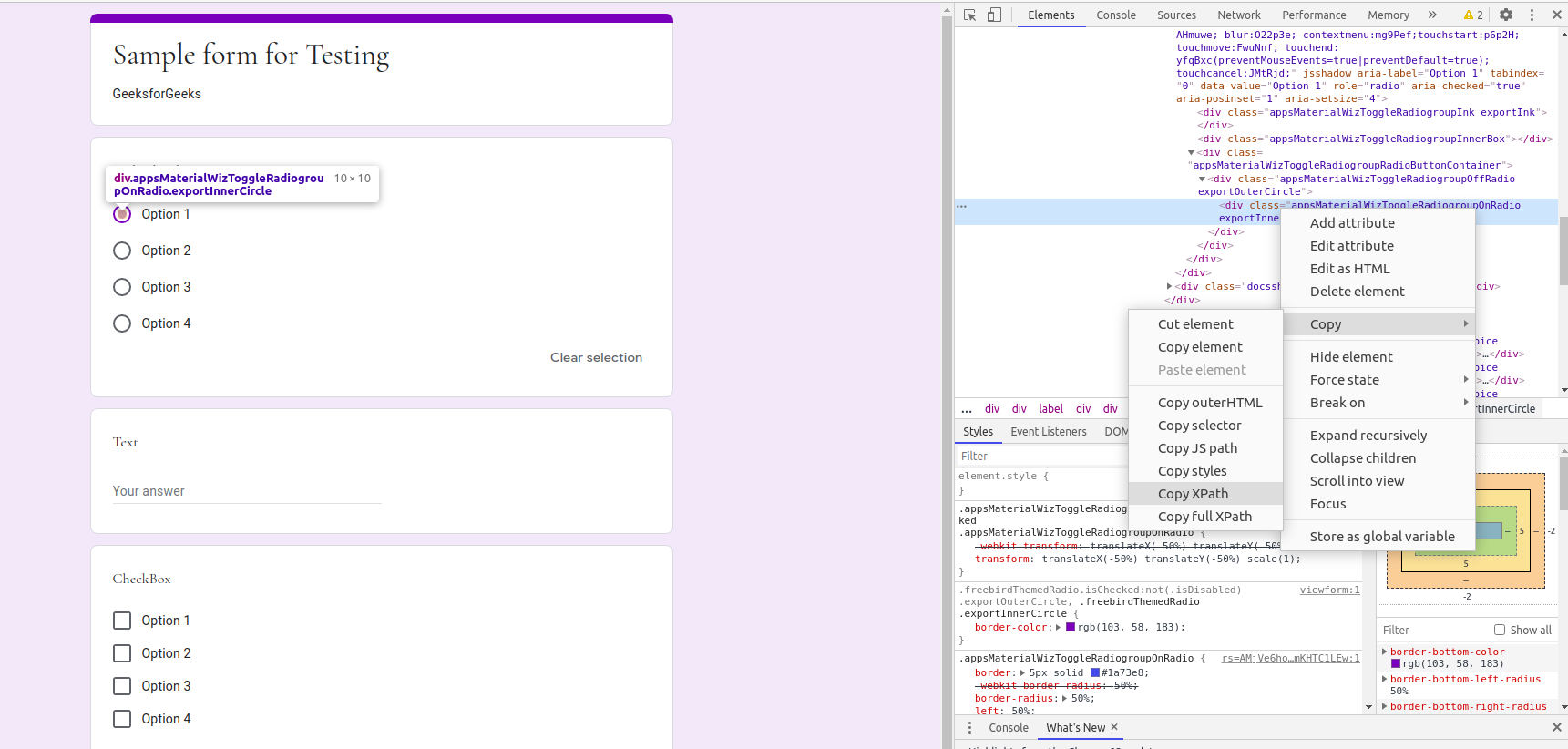
This process works for multiple-choice, text, and checkbox fields. Now you can paste the XPath in the .py file in the form a string as shown below. You would also need the XPath of the Submit button and Submit another response (resubmit) button. The resubmit button makes automation more accessible.
此过程适用于多项选择,文本和复选框字段。 现在,您可以将XPath以字符串形式粘贴在.py文件中,如下所示。 您还需要“ 提交”按钮的XPath和“ 提交另一个响应 (重新提交)”按钮。 重新提交按钮使自动化更容易访问。
radioOption3 ='//*[@id="mG61Hd"]/div[2]/div/div[2]/div[1]/div/div/div[2]/div[1]/div/span/div/div[3]/label/div/div[1]/div/div[3]'
checkBox2 = '//*[@id="mG61Hd"]/div[2]/div/div[2]/div[3]/div/div/div[2]/div[1]/div[2]/label/div/div[1]/div[2]'
checkBox3 = '//*[@id="mG61Hd"]/div[2]/div/div[2]/div[3]/div/div/div[2]/div[1]/div[3]/label/div/div[1]/div[2]'
text = '//*[@id="mG61Hd"]/div[2]/div/div[2]/div[2]/div/div/div[2]/div/div[1]/div/div[1]/input'
submit = '//*[@id="mG61Hd"]/div[2]/div/div[3]/div[1]/div/div/span/span'
resubmit = '/html/body/div[1]/div[2]/div[1]/div/div[4]/a'
Now, you are left with the final step, implementing the loop for automation. Let’s say you want to fill the form 100 times. So you run a while loop 100 times, incrementing variable i each time. Inside the while loop, you use find_element_by_xpath and click() attribute to implement your functionality. For the text field, the send_keys method does the job. The time.sleep(1) waits for 1 second after submitting the form to avoid any unwanted error. The following code uses try and except for the same purpose (as a precaution). If there is no error, the tool will go on to submit another response in the same window. If any statement generates an error, it will close that window, and start with a new one. The driver.quit() statement at the end stops/completes the process of automation.
现在,您将剩下最后一步,实现自动化循环。 假设您要填写100次表格。 因此,您运行一次while循环100次,每次都增加变量i 。 在while循环内,您可以使用find_element_by_xpath和click()属性来实现您的功能。 对于文本字段, send_keys方法完成该工作。 提交表单后, time.sleep(1)等待1秒钟,以避免任何不必要的错误。 下面的代码使用尝试和除了同样的目的(作为预防措施)。 如果没有错误,该工具将继续在同一窗口中提交另一个响应。 如果有任何语句产生错误,它将关闭该窗口,并从一个新窗口开始。 最后的driver.quit()语句停止/完成了自动化过程。
i=0
while i<100:
driver.find_element_by_xpath(radioOption3).click()
driver.find_element_by_xpath(checkBox2).click()
driver.find_element_by_xpath(checkBox3).click()
text_box=driver.find_element_by_xpath(text)
text_box.send_keys("The text I need")
driver.find_element_by_xpath(submit).click()
i=i+1
time.sleep(1)
try:
driver.find_element_by_xpath(resubmit).click()
except:
driver.close()
driver = webdriver.Remote(command_executor='Your unique BrowserStack link',desired_capabilities=desired_cap)
driver.get('https://forms.gle/xX5NEumdXW4Z7SrC6')
driver.quit()
Now all you need to do is go to your terminal and use the following command to run the program.
现在,您所需要做的就是转到终端并使用以下命令运行该程序。
python3 filename.py
Now you can move to your BrowserStack Dashboard and see your program fill the form 100 times.
现在,您可以移至BrowserStack仪表板,并看到您的程序填写100次。
Selenium is a powerful tool. The code is easy to implement, and it makes testing and automation a lot easier. BrowserStack provides an easy-to-use powerful interface which makes cloud-based testing a lot easier.
Selenium是一个强大的工具。 该代码易于实现,并且使测试和自动化变得更加容易。 BrowserStack提供了易于使用的强大界面,这使得基于云的测试变得更加容易。
I will also soon be publishing it on GeeksforGeeks.
我还将很快将其发布在 GeeksforGeeks上 。
翻译自: https://medium.com/@singhsuryansh12/automation-using-browserstack-and-selenium-f3f01c0360e1